总结两道题,都是和数组有关。
Spiral Matrix(LC54 Medium):
Given a matrix of m x n elements (m rows, n columns), return all elements of the matrix in spiral order.
For example,
Given the following matrix:
[ [ 1, 2, 3 ], [ 4, 5, 6 ], [ 7, 8, 9 ] ]
You should return [1,2,3,6,9,8,7,4,5]
.
class Solution(object): def spiralOrder(self, matrix): """ :type matrix: List[List[int]] :rtype: List[int] """ result=[] if not matrix: return [] if len(matrix[0])==1: for i in range(len(matrix)): result.append(matrix[i][0]) else: while matrix: result+=matrix.pop(0)# first line if matrix and matrix[0]: for i in range(len(matrix)): result.append(matrix[i].pop()) if matrix: result+=matrix.pop()[::-1] if matrix and matrix[0]: for i in matrix[::-1]: result.append(i.pop(0)) return result
这道题是给定了一个螺旋的matrix,然后再按顺序返回
主要技巧在于使用pop,pop(0)表示弹出第一个元素,pop()默认弹出最后一个元素
然后按照螺旋的走势,把pop出来的元素append到result里
期中,matrix.pop()[::-1]中的[::-1]表示在matrix最后一行,从最后一个元素开始pop,直到最后一行所有元素pop出去,不需要循环,所以不能用append,只能用+
例子:
[ [ 1, 2, 3 ], [ 4, 5, 6 ], [ 7, 8, 9 ] ]
1. 先把第一行所有元素从左到右pop出去,因为只需要一直pop(0)就行,所以不需要循环,所以不能用append,用+
2. 然后按照螺旋的顺序,应该是最右边,从上到小,6,9。这里的操作必须是在matrix和matrix[0]不为空时。这里用了循环,pop()每行最后一个元素,用append
3. 接下来是8,7.要考虑matrix不为空,因为是从matrix最后一行,从最后一个元素pop
4. 最后是4,5.因为是最后两个元素,所以其他元素应该已经被pop出去了,所以这里相当于是从matrix最后一行开始从左到右pop(0),循环,用append
Spiral Matrix II(LC59)
Given an integer n, generate a square matrix filled with elements from 1 to n2 in spiral order.
For example,
Given n = 3
,
You should return the following matrix:
[ [ 1, 2, 3 ], [ 8, 9, 4 ], [ 7, 6, 5 ] ]
class Solution(object): def generateMatrix(self, n): """ :type n: int :rtype: List[List[int]] """ result=[] left=n*n+1 while left>1: left,right=left-len(result),left result=[range(left,right)]+zip(*result[::-1]) return result
1. 这道题的思路很巧妙,从最大的那个数:n*n开始加入result,先顺时针旋转数组,然后在前面加元素,直到1
2. 旋转数组用了zip,zip(*result[::-1]),返回的是tuple (zip: https://www.cnblogs.com/frydsh/archive/2012/07/10/2585370.html)
例子:
1. zip:
zip(*xyz)==zip(zip(x,y,z))
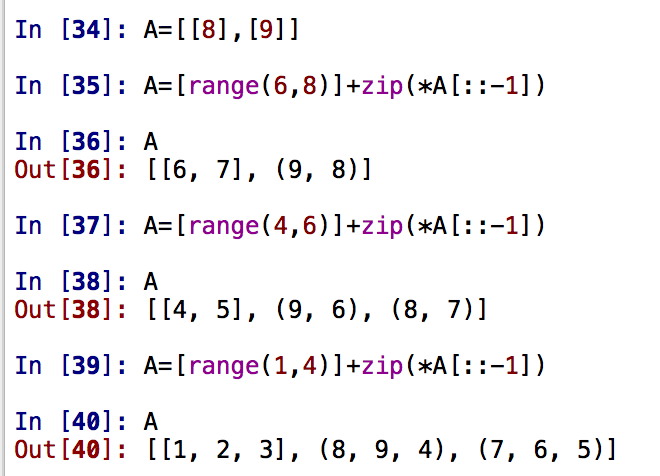