[POJ1177]Picture
试题描述
A number of rectangular posters, photographs and other pictures of the same shape are pasted on a wall. Their sides are all vertical or horizontal. Each rectangle can be partially or totally covered by the others. The length of the boundary of the union of all rectangles is called the perimeter.
Write a program to calculate the perimeter. An example with 7 rectangles is shown in Figure 1.
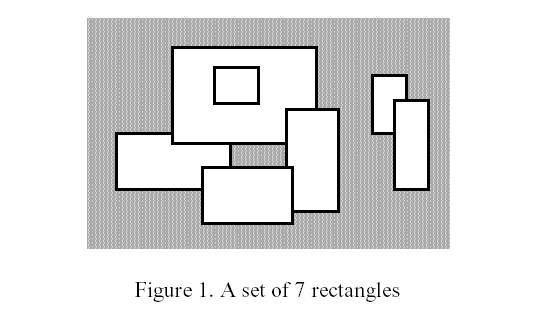
The corresponding boundary is the whole set of line segments drawn in Figure 2.
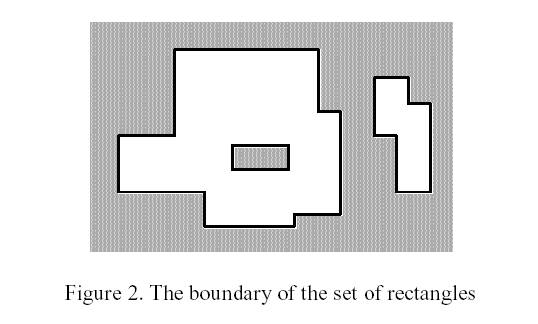
The vertices of all rectangles have integer coordinates.
输入
Your program is to read from standard input. The first line contains the number of rectangles pasted on the wall. In each of the subsequent lines, one can find the integer coordinates of the lower left vertex and the upper right vertex of each rectangle. The values of those coordinates are given as ordered pairs consisting of an x-coordinate followed by a y-coordinate.
0 <= number of rectangles < 5000
All coordinates are in the range [-10000,10000] and any existing rectangle has a positive area.
输出
Your program is to write to standard output. The output must contain a single line with a non-negative integer which corresponds to the perimeter for the input rectangles.
输入示例
7 -15 0 5 10 -5 8 20 25 15 -4 24 14 0 -6 16 4 2 15 10 22 30 10 36 20 34 0 40 16
输出示例
228
数据规模及约定
见“输入”
题解
可以发现水平方向的边与竖直方向的边是独立的,那么我们可以分别做两次扫描线。
不难发现扫描线运行时要进行区间修改操作,所以会想到线段树。会发现单纯地打懒标记像一般的题那样做是不行的,这里引入一个新的维护方法,在这里,我们不用标记下传;每次区间的修加就是对对应区间的节点打上一个标记,区间删就是对对应区间的节点回收那个标记,标记是静态的,不能下传。
显然对答案的贡献一定是在某个矩形的上下边界时产生的,也就是说只用在扫描线扫到需要加或需要删的操作时才累计答案。对于加操作,我们计加之后与加之前相差为 t,那么把答案累加 t;删操作同理。
#include <iostream> #include <cstdio> #include <cstdlib> #include <cstring> #include <cctype> #include <algorithm> using namespace std; int read() { int x = 0, f = 1; char c = getchar(); while(!isdigit(c)){ if(c == '-') f = -1; c = getchar(); } while(isdigit(c)){ x = x * 10 + c - '0'; c = getchar(); } return x * f; } #define maxn 20010 #define maxr 5010 int n; struct Rec { int x1, y1, x2, y2; Rec() {} Rec(int _1, int _2, int _3, int _4): x1(_1), y1(_2), x2(_3), y2(_4) {} } rs[maxr]; struct Line { int l, r, x; Line() {} Line(int _1, int _2, int _3): l(_1), r(_2), x(_3) {} bool operator < (const Line& t) const { return x < t.x; } } ad[maxr], mi[maxr]; int ca, cm, ans; int cntv[maxn<<2], sumv[maxn<<2]; void update(int L, int R, int o, int ql, int qr, int v) { int M = L + R >> 1, lc = o << 1, rc = lc | 1; if(ql <= L && R <= qr) { cntv[o] += v; if(cntv[o]) sumv[o] = R - L + 1; else if(L == R) sumv[o] = 0; else sumv[o] = sumv[lc] + sumv[rc]; return ; } if(ql <= M) update(L, M, lc, ql, qr, v); if(qr > M) update(M+1, R, rc, ql, qr, v); sumv[o] = cntv[o] ? R - L + 1 : sumv[lc] + sumv[rc]; return ; } void solve() { sort(ad + 1, ad + ca + 1); sort(mi + 1, mi + ca + 1); memset(sumv, 0, sizeof(sumv)); memset(cntv, 0, sizeof(cntv)); int ka = 1, km = 1; for(int i = 1; i <= 20001; i++) { while(ka <= ca && ad[ka].x == i) { int tmp = sumv[1]; update(1, 20001, 1, ad[ka].l, ad[ka].r - 1, 1); ans += sumv[1] - tmp; // printf("add[%d, %d]: %d <- %d ", ad[ka].l, ad[ka].r - 1, sumv[1], tmp); ka++; } while(km <= cm && mi[km].x == i) { int tmp = sumv[1]; update(1, 20001, 1, mi[km].l, mi[km].r - 1, -1); ans += tmp - sumv[1]; // printf("del[%d, %d]: %d <- %d ", mi[km].l, mi[km].r - 1, sumv[1], tmp); km++; } } return ; } int main() { n = read(); for(int i = 1; i <= n; i++) { int x1 = read() + 10001, y1 = read() + 10001, x2 = read() + 10001, y2 = read() + 10001; rs[i] = Rec(x1, y1, x2, y2); ad[++ca] = Line(y1, y2, x1); mi[++cm] = Line(y1, y2, x2); } solve(); ca = cm = 0; for(int i = 1; i <= n; i++) { int x1 = rs[i].x1, x2 = rs[i].x2, y1 = rs[i].y1, y2 = rs[i].y2; ad[++ca] = Line(x1, x2, y1); mi[++cm] = Line(x1, x2, y2); } solve(); printf("%d ", ans); return 0; }