linux 进程间通信系列3,使用socketpair,pipe
1,使用socketpair,实现进程间通信,是双向的。
2,使用pipe,实现进程间通信
使用pipe关键点:fd[0]只能用于接收,fd[1]只能用于发送,是单向的。
3,使用pipe,用标准输入往里写。
疑问:在代码2里不写wait函数的话,父进程不能结束,但是在代码3里也没有写wait函数,父进程却可以结束???
1,使用socketpair:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <wait.h>
int main(){
int sv[2];
pid_t pid;
char buf[128];
memset(buf, 0, sizeof(buf));
if(socketpair(AF_UNIX, SOCK_STREAM, 0, sv) != 0){
perror("socketpair");
return 1;
}
pid = fork();
if(pid < 0){
perror("fork");
return 1;
}
if(pid == 0){
close(sv[0]);
read(sv[1], buf, sizeof(buf));
printf("child process : data from parant process [%s]
", buf);
exit(0);
}
else {
int status;
close(sv[1]);
write(sv[0], "HELLO", 5);
printf("parent process : child process id %d
", pid);
wait(&status);
}
return 0;
}
github源代码
2,使用pipe:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <wait.h>
int main(){
int p[2];
pid_t pid;
char buf[128];
memset(buf, 0, sizeof(buf));
if(pipe(p) != 0){
perror("pipe");
return 1;
}
pid = fork();
if(pid < 0){
perror("fork");
return 1;
}
if(pid == 0){
close(p[1]);
read(p[0], buf, sizeof(buf));
printf("child process : data form parent process [%s]
", buf);
exit(0);
}
else{
close(p[0]);
write(p[1], "aaaa", 4);
printf("parent process : child process is %d
", pid);
int status;
wait(&status);
}
return 0;
}
github源代码
3,使用pipe,用标准输入往里写。
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <wait.h>
int main(){
int p[2];
pid_t pid;
char buf[1024];
memset(buf, 0, sizeof(buf));
if(pipe(p) != 0){
perror("pipe");
return 1;
}
pid = fork();
if(pid < 0){
perror("fork");
return 1;
}
if(pid == 0){
printf("child process : my_id=%d
",getpid());
close(p[0]);
//把标准输出给管道1了
dup2(p[1], fileno(stdout));
char *argv[ ]={"ls", "/home/ys/cpp/network"};
//利用ls命令,往标准输出里,输入文件夹里文件的的名字,标准输出又连接到了上面开的管道1里。
if(execve("/bin/ls", argv, NULL) < 0){
perror("exec");
return 1;
}
exit(0);
}else{
int n;
FILE* filep;
close(p[1]);
printf("parent process : child process id=%d
", pid);
//先打开管道1
filep = fdopen(p[0], "r");
if(filep == NULL){
perror("fdopen");
return 1;
}
//再从管道1里读取数据
while(fgets(buf, sizeof(buf), filep) != NULL){
printf("get:%s
", buf);
}
int status;
wait(&status);
}
return 0;
}
github源代码
c/c++ 学习互助QQ群:877684253
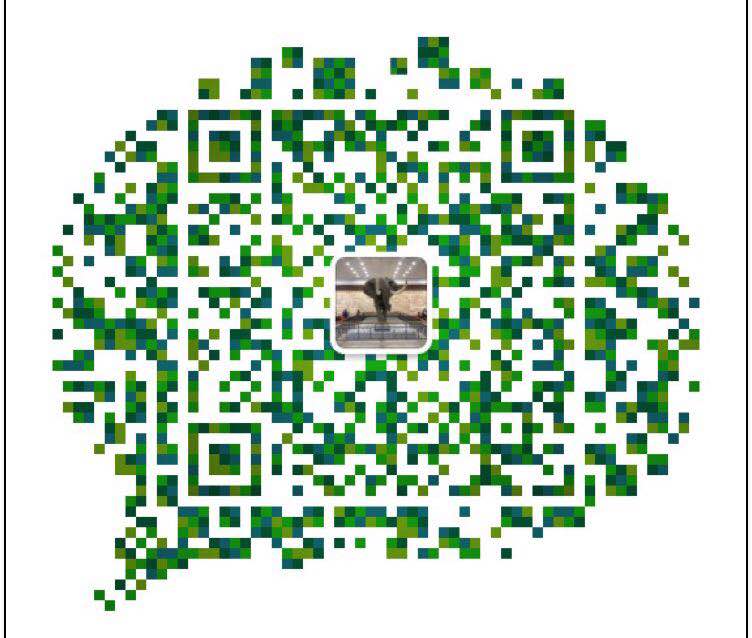
本人微信:xiaoshitou5854