完整的Django请求生命周期:
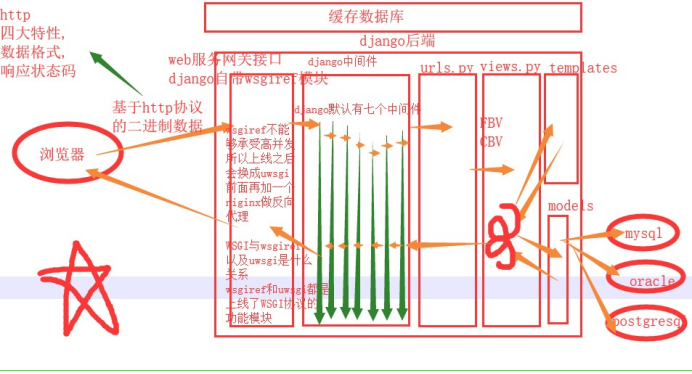
django中间件:
"""
请求来的时候,需要先经过中间件才能到达django后端:(urls,views,templates,models)
响应走的时候也需要经过中间件才能到达web服务网关接口
"""
django默认的七个中间件:
MIDDLEWARE = [
'django.middleware.security.SecurityMiddleware',
'django.contrib.sessions.middleware.SessionMiddleware',
'django.middleware.common.CommonMiddleware',
'django.middleware.csrf.CsrfViewMiddleware',
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django.contrib.messages.middleware.MessageMiddleware',
'django.middleware.clickjacking.XFrameOptionsMiddleware',
]
中间件执行顺序:
#请求来的时候,从上往下依次执行每个中间件中的process_request()方法,如果该中间件中没有process_request方法,将直接跳过执行下一个中间件。
#响应走的时候,从下往上依次执行每个中间件中的process_response()方法,如果该中间件中没有process_response()方法,将直接跳过执行下一个中间件。
自定义Django中间件:
"""
django中间件可以用来做什么?
1.网站全局的身份校验,访问频率限制,权限校验...只要是涉及到全局的校验你都可以在中间件中完成
2.django的中间件是所有web框架中 做的最好的
"""
#django中间件提供了五个用户可以自定义的方法
"""
如何自定义我们自己的中间件?
1.如果你想让你写的中间件生效,就必须要先继承MiddlewareMixin
2.在注册自定义中间件的时候,一定要确保路径不要写错
"""
class MyMdd(MiddlewareMixin):
def process_request(self,request):
print('我是第一个中间件里面的process_request方法')
def process_response(self,request,response):
print('我是第一个中间件里面的process_response方法')
return response
def process_view(self,request,view_func,view_args,view_kwargs):
print(view_func)
print(view_args)
print(view_kwargs)
print('我是第一个中间件里面的process_view方法')
def process_exception(self, request, exception):
print('我是第一个中间件里面的process_exception')
def process_template_response(self, request, response):
print('我是第一个中间件里面的process_template_response')
return response
# settings中注册Django中间件
'mymiddleware.mdd.MyMdd',
掌握:
# 1.process_request()方法
"""
规律:
1.请求来的时候 会经过每个中间件里面的process_request方法(从上往下)
2.如果方法里面直接返回了HttpResponse对象 那么会直接返回 不再往下执行
基于该特点就可以做访问频率限制,身份校验,权限校验
"""
# 2.process_response()方法
"""
规律:
1.必须将response形参返回 因为这个形参指代的就是要返回给前端的数据
2.响应走的时候 会依次经过每一个中间件里面的process_response方法(从下往上)
"""
了解:
# 3.process_view()
"""触发条件:在路由匹配成功执行视图函数之前 触发"""
# 4.process_exception()
"""触发条件:当你的视图函数报错时 就会自动执行"""
# 5.process_template_response()
"""触发条件:当你返回的HttpResponse对象中必须包含render属性才会触发"""
def index(request):
print('我是index视图函数')
def render():
return HttpResponse('render属性')
obj = HttpResponse('index')
obj.render = render # 给obj添加属性,属性名为render,属性值为render函数
return obj
"""
总结:你在书写中间件的时候 只要形参中有repsonse 你就顺手将其返回 这个reponse就是要给前端的消息
"""
csrf跨站请求伪造:
# 钓鱼网站:
"""
通过制作一个跟正儿八经的网站一模一样的页面,骗取用户输入信息 转账交易
从而做手脚转账交易的请求确确实实是发给了中国银行,账户的钱也是确确实实
少了唯一不一样的地方在于收款人账户不对
"""
# 内部原理:
"""
在让用户输入对方账户的那个input上面做手脚
给这个input不设置name属性,在内部隐藏一个事先写好的name和value属性的input框
这个value的值 就是钓鱼网站受益人账号
"""
# 防止钓鱼网站的思路:
"""
网站会给返回给用户的form表单页面 偷偷塞一个随机字符串
请求到来的时候 会先比对随机字符串是否一致 如果不一致 直接拒绝(403)
"""
# 该随机字符串有以下特点:
"""
1.同一个浏览器每一次访问都不一样
2.不同浏览器绝对不会重复
"""
解决方案:
1.post表单提交:
# 1.form表单发送post请求时,携带token,在表单上方书写(不再需要注释掉那一行中间件)
{% csrf_token %}
2.ajax发post请求:
# 1.现在页面上写{% csrf_token %},利用标签查找 获取到该input键值信息
{'username':'yyj','csrfmiddlewaretoken':$('[name=csrfmiddlewaretoken]').val()}
$('[name=csrfmiddlewaretoken]').val()
# 2.直接书写'{{ csrf_token }}'
{'username':'yyj','csrfmiddlewaretoken':'{{ csrf_token }}'}
{{ csrf_token }}
# 3.你可以将该获取随机键值对的方法 写到一个js文件中,之后只需要导入该文件即可
"""
新建一个js文件,存放以下代码,之后导入即可。(设置为静态文件,添加到settings中的STATICFILES_DIRS文件列表,之后在你想使用的html页面中进行引入即可)
"""

function getCookie(name) {
var cookieValue = null;
if (document.cookie && document.cookie !== '') {
var cookies = document.cookie.split(';');
for (var i = 0; i < cookies.length; i++) {
var cookie = jQuery.trim(cookies[i]);
// Does this cookie string begin with the name we want?
if (cookie.substring(0, name.length + 1) === (name + '=')) {
cookieValue = decodeURIComponent(cookie.substring(name.length + 1));
break;
}
}
}
return cookieValue;
}
var csrftoken = getCookie('csrftoken');
function csrfSafeMethod(method) {
// these HTTP methods do not require CSRF protection
return (/^(GET|HEAD|OPTIONS|TRACE)$/.test(method));
}
$.ajaxSetup({
beforeSend: function (xhr, settings) {
if (!csrfSafeMethod(settings.type) && !this.crossDomain) {
xhr.setRequestHeader("X-CSRFToken", csrftoken);
}
}
});
JS文件
问题:
"""
1.当你网站全局都需要校验csrf的时候,有几个不需要校验该如何处理
2.当你网站全局不校验csrf的时候,有几个需要校验又该如何处理
"""
# 装饰器的导入
from django.utils.decorators import method_decorator
from django.views.decorators.csrf import csrf_exempt,csrf_protect
# FBV装饰时直接给函数使用装饰器:@csrf_exempt和@crsf_protect
# @csrf_exempt和@crsf_protect装饰器在给CBV装饰的时:
# 如果是csrf_protect 那么有三种方式:
# 第一种方式:
@method_decorator(csrf_protect,name='post')
class MyView(View):
pass
# 第二种方式
@method_decorator(csrf_protect)
def post(self,request):
return HttpResponse('post')
# 第三种方式
@method_decorator(csrf_protect)
def dispatch(self, request, *args, **kwargs):
res = super().dispatch(request, *args, **kwargs)
return res
def get(self,request):
return HttpResponse('get')
# 如果是csrf_exempt 只有两种方式(只能给dispatch装):
# 第二种可以不校验的方式
@method_decorator(csrf_exempt,name='dispatch')
class MyView(View):
@method_decorator(csrf_exempt) # 第一种可以不校验的方式
def dispatch(self, request, *args, **kwargs):
res = super().dispatch(request, *args, **kwargs)
return res
def get(self,request):
return HttpResponse('get')
def post(self,request):
return HttpResponse('post')
"""
总结:装饰器中只有csrf_exempt是特例,其他的装饰器在给CBV装饰的时候 都可以有三种方式,即类似于csrf_protect的三种方式.
"""
auth模块
# 查询用户
from django.contrib import auth
# 必须要用authenticate()方法,因为数据库中的密码字段是密文的,而你获取的用户输入的是明文
user_obj = auth.authenticate(username=username,password=password)
#models.User.objects.filter(username=username,password=password).first()
# 记录用户状态
auth.login(request,user_obj) # 将用户状态记录到session中
# request.session['user'] = user_obj
# 判断用户是否登录
print(request.user.is_authenticated) # 判断用户是否登录 如果是你们用户会返回False
# 用户登录之后,获取用户对象
print(request.user) # 如果没有执行auth.login那么拿到的是匿名用户
#校验用户是否登录
from django.contrib.auth.decorators import login_required
@login_required(login_url='/xxx/') # 局部配置
def index(request):
pass
# 全局配置 settings文件中
LOGIN_URL = '/xxx/'
# 验证密码是否正确
request.user.check_password(old_password)
# 修改密码
request.user.set_password(new_password)
request.user.save() # 修改密码的时候 一定要save保存 否则无法生效
# 退出登陆
auth.logout(request) # request.session.flush()
# 注册用户
# User.objects.create(username =username,password=password) # 创建用户名的时候 千万不要再使用create 了
# User.objects.create_user(username =username,password=password) # 创建普通用户
User.objects.create_superuser(username =username,password=password,email='123@qq.com') # 创建超级用户 需要填写邮箱.
自定义auth_user表:
from django.contrib.auth.models import AbstractUser
"""
第一种:使用一对一关系(不考虑)
第二种方式:使用类的继承
"""
class Userinfo(AbstractUser):
# 千万不要跟原来表中的字段重复,只能添加新的字段
phone = models.BigIntegerField()
avatar = models.CharField(max_length=32)
# 去Django的settings文件中,配置使用自定义的表,而不再使用auth_user表
AUTH_USER_MODEL = 'app01.Userinfo' # '应用名.类名'
"""
执行数据库迁移命令(需要重新设置新的数据库)
所有的auth模块功能 全部都基于你创建的表
而不再使用auth_user
"""
插拔式原理:
#插拔式思想的应用:Django中间件。
#核心方法:importlib和反射getattr来完成。
# |—notify文件夹
|—__init__.py
|—email.py
|—msg.py
|—wechat.py
# |—settings.py
# |—start.py
# __init__.py文件
import settings
import importlib
def send_all(content):
for path_str in settings.NOTIFY_LIST: # 1.拿出一个个的字符串 'notify.email.Email'
module_path, class_name = path_str.rsplit('.', maxsplit=1) # 2.从右边开始 按照点切一个 ['notify.email','Email']
module = importlib.import_module(module_path) # from notity import msg,email,wechat
cls = getattr(module, class_name) # 利用反射 一切皆对象的思想 从文件中获取属性或者方法 cls = 一个个的类名
obj = cls() # 类实例化生成对象
obj.send(content) # 对象调方法
# wechat.py文件
class WeChat(object):
def __init__(self):
pass # 发送微信需要的代码配置
def send(self,content):
print('微信通知:%s'%content)
# email.py文件
class Email(object):
def __init__(self):
pass # 发送邮件需要的代码配置
def send(self,content):
print('邮件通知:%s'%content)
# settings.py文件
NOTIFY_LIST = [
'notify.email.Email',
'notify.msg.Msg',
# 'notify.wechat.WeChat',
'notify.qq.QQ',
]
# start.py文件
import notify
notify.send_all('通知内容:恭喜您中奖了!')