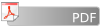
Problem D: Airport Express |
In a small city called Iokh, a train service, Airport-Express, takes residents to the airport more quickly than other transports. There are two types of trains in Airport-Express, the Economy-Xpress and the Commercial-Xpress. They travel at different speeds, take different routes and have different costs.
Jason is going to the airport to meet his friend. He wants to take the Commercial-Xpress which is supposed to be faster, but he doesn't have enough money. Luckily he has a ticket for the Commercial-Xpress which can take him one station forward. If he used the ticket wisely, he might end up saving a lot of time. However, choosing the best time to use the ticket is not easy for him.
Jason now seeks your help. The routes of the two types of trains are given. Please write a program to find the best route to the destination. The program should also tell when the ticket should be used.
Input
The input consists of several test cases. Consecutive cases are separated by a blank line.
The first line of each case contains 3 integers, namely N, S and E (2 ≤ N ≤ 500, 1 ≤ S, E ≤ N), which represent the number of stations, the starting point and where the airport is located respectively.
There is an integer M (1 ≤ M ≤ 1000) representing the number of connections between the stations of the Economy-Xpress. The next M lines give the information of the routes of the Economy-Xpress. Each consists of three integers X, Y and Z (X, Y ≤ N, 1 ≤ Z ≤ 100). This means X and Y are connected and it takes Z minutes to travel between these two stations.
The next line is another integer K (1 ≤ K ≤ 1000) representing the number of connections between the stations of the Commercial-Xpress. The next K lines contain the information of the Commercial-Xpress in the same format as that of the Economy-Xpress.
All connections are bi-directional. You may assume that there is exactly one optimal route to the airport. There might be cases where you MUST use your ticket in order to reach the airport.
Output
For each case, you should first list the number of stations which Jason would visit in order. On the next line, output "Ticket Not Used" if you decided NOT to use the ticket; otherwise, state the station where Jason should get on the train of Commercial-Xpress. Finally, print the total time for the journey on the last line. Consecutive sets of output must be separated by a blank line.
Sample Input
4 1 4 4 1 2 2 1 3 3 2 4 4 3 4 5 1 2 4 3
Sample Output
1 2 4 2 5
题意:去机场有两种方法,一个是经济线一个是商业线。线路、速度、价格都不同样,你有一张商业票。能够坐一站商业线。而其它时候仅仅能做经济线,换乘时间不计算,你的任务是找一条去机场最快的线路。
思路:枚举商业线的起点和终点,然后分别从我们的起点和终点最短路。然后找出最优解就能够了
#include <iostream> #include <cstdio> #include <vector> #include <cstring> #include <algorithm> #include <queue> using namespace std; const int MAXN = 505; const int INF = 0x3f3f3f3f; struct Edge { int from, to, dist; }; struct HeapNode { int d, u; bool operator< (const HeapNode rhs) const { return d > rhs.d; } }; struct Dijkstra { int n, m; // 点数和边数 vector<Edge> edges; //边列表 vector<int> G[MAXN]; // 每一个点出发的边编号(0開始) bool done[MAXN]; // 是否已标记 int d[MAXN]; //s 到各个点的距离 int p[MAXN]; //最短路中上一个点,也能够是上一条边 void init(int n) { this->n = n; for (int i = 0; i < n; i++) G[i].clear(); edges.clear(); } void AddEdge(int from, int to, int dist) { edges.push_back((Edge){from, to, dist}); m = edges.size(); G[from].push_back(m-1); } void dijkstra(int s) { priority_queue<HeapNode> Q; for (int i = 0; i < n; i++) d[i] = INF; d[s] = 0; memset(done, 0, sizeof(done)); Q.push((HeapNode){0, s}); while (!Q.empty()) { HeapNode x = Q.top(); Q.pop(); int u = x.u; if (done[u]) continue; done[u] = true; for (int i = 0; i < G[u].size(); i++) { Edge &e = edges[G[u][i]]; if (d[e.to] > d[u] + e.dist) { d[e.to] = d[u] + e.dist; p[e.to] = e.from; Q.push((HeapNode){d[e.to], e.to}); } } } } void getPath(vector<int> &path, int s, int e) { int cur = e; while (1) { path.push_back(cur); if (cur == s) return ; cur = p[cur]; } } }; int n, m, k, s, e; int x, y, z; vector<int> path; int main() { int first = 1; while (scanf("%d%d%d", &n, &s, &e) != EOF) { if (first) first = 0; else printf(" "); s--, e--; Dijkstra ans[2]; ans[0].init(n); ans[1].init(n); scanf("%d", &m); while (m--) { scanf("%d%d%d", &x, &y, &z); x--, y--; ans[0].AddEdge(x, y, z); ans[0].AddEdge(y, x, z); ans[1].AddEdge(x, y, z); ans[1].AddEdge(y, x, z); } ans[0].dijkstra(s); ans[1].dijkstra(e); scanf("%d", &k); path.clear(); int Min = ans[0].d[e]; int flagx = -1, flagy = -1; while (k--) { scanf("%d%d%d", &x, &y, &z); x--, y--; if (Min > ans[0].d[x] + z + ans[1].d[y]) { Min = ans[0].d[x] + z + ans[1].d[y]; flagx = x, flagy = y; } if (Min > ans[1].d[x] + z + ans[0].d[y]) { Min = ans[1].d[x] + z + ans[0].d[y]; flagx = y, flagy = x; } } if (flagx == -1) { ans[0].getPath(path, s, e); reverse(path.begin(), path.end()); for (int i = 0; i < path.size()-1; i++) printf("%d ", path[i]+1); printf("%d ", path[path.size()-1]+1); printf("Ticket Not Used "); printf("%d ", Min); } else { ans[0].getPath(path, s, flagx); reverse(path.begin(), path.end()); ans[1].getPath(path, e, flagy); for (int i = 0; i < path.size()-1; i++) printf("%d ", path[i]+1); printf("%d ", path[path.size()-1]+1); printf("%d ", flagx+1); printf("%d ", Min); } } return 0; }