POJ3126:Prime Path
kuangbin专题一:F题
Description
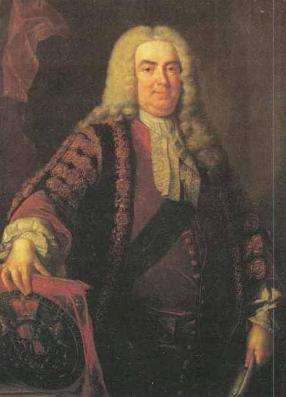
— It is a matter of security to change such things every now and then, to keep the enemy in the dark.
— But look, I have chosen my number 1033 for good reasons. I am the Prime minister, you know!
— I know, so therefore your new number 8179 is also a prime. You will just have to paste four new digits over the four old ones on your office door.
— No, it’s not that simple. Suppose that I change the first digit to an 8, then the number will read 8033 which is not a prime!
— I see, being the prime minister you cannot stand having a non-prime number on your door even for a few seconds.
— Correct! So I must invent a scheme for going from 1033 to 8179 by a path of prime numbers where only one digit is changed from one prime to the next prime.
Now, the minister of finance, who had been eavesdropping, intervened.
— No unnecessary expenditure, please! I happen to know that the price of a digit is one pound.
— Hmm, in that case I need a computer program to minimize the cost. You don't know some very cheap software gurus, do you?
— In fact, I do. You see, there is this programming contest going on... Help the prime minister to find the cheapest prime path between any two given four-digit primes! The first digit must be nonzero, of course. Here is a solution in the case above.
1033The cost of this solution is 6 pounds. Note that the digit 1 which got pasted over in step 2 can not be reused in the last step – a new 1 must be purchased.
1733
3733
3739
3779
8779
8179
Input
One
line with a positive number: the number of test cases (at most 100).
Then for each test case, one line with two numbers separated by a blank.
Both numbers are four-digit primes (without leading zeros).
Output
One line for each case, either with a number stating the minimal cost or containing the word Impossible.
Sample Input
3 1033 8179 1373 8017 1033 1033
Sample Output
6 7 0
题意:给定两个素数n和m,要求把n变成m,每次变换时只能变一个数字,即变换后的数与变换前的数只有一个数字不同,
并且要保证变换后的四位数也是素数。求最小的变换次数;如果不能完成变换,输出Impossible。
无论怎么变换,个位数字一定是奇数(个位数字为偶数肯定不是素数),这样枚举个位数字时只需枚举奇数就行;
而且千位数字不能是0。所以可以用广搜,枚举各个数位上的数字,满足要求的数就加入队列,直到变换成功。
因为是广搜,所以一定能保证次数最少。
/* queue队列:
调用头文件:
#include<queue>
using namespace std;
详细用法(部分):
queue<Type> k; ------ 定义一个queue的变量(定义时已经初始化) 例如: queue<int> k;
k.empty() ------ 查看是否为空范例,是的话返回1,不是返回0
k.push(i) ------ 从已有元素后面增加元素i(队伍大小不预设)
k.pop() ------ 清除第一个元素
k.front() ------ 显示第一个元素 例如n = k.front();
k.back() ------ 显示最后一个元素
k.size() ------ 输出现有元素的个数
*/
#include <cstdio> #include <iostream> #include <cstring> #include <algorithm> #include <cmath> #include <queue> using namespace std ; #define maxn 100000 #define LL long long bool prime[maxn] ; bool visit[maxn] ; int pre[maxn] ; int num1 , num2 ; struct node { int num ; int step ; }; node st ; int result ; void init(){//线性筛法(O(n)) for(int i=2 ; i<maxn ; i++){ prime[i] = true ; } prime[0] = prime[1] = false ; int total = 0 ; for(int i=2 ; i< maxn ; i++){ if(prime[i]){ pre[total ++ ] = i ; } for(int j = 0 ; j< total && i*pre[j] < maxn ; j++ ){ prime[i * pre[j]] = false ; if(i%pre[j] == 0 ){ break ; } } } } void BFS(){ queue<node> Q ; Q.push(st) ; while(!Q.empty()){ node q = Q.front() ; Q.pop() ; if(q.num == num2){ printf("%d " , q.step) ; return; } node turn ; // 个位 for(int i=1 ; i<=9 ; i+=2){ turn.num = q.num/10*10 + i ; turn.step = q.step + 1 ; if(!visit[turn.num]&&prime[turn.num]){ visit[turn.num] = 1 ; Q.push(turn) ; } } // 十位 for(int i=0 ; i<=9 ; i++){ turn.num = q.num/100*100 + q.num%10 + i *10 ; turn.step = q.step + 1 ; if(!visit[turn.num]&&prime[turn.num]){ visit[turn.num] = 1 ; Q.push(turn) ; } } // 百位 for(int i=0 ; i<=9 ; i++){ turn.num = q.num/1000*1000 + q.num % 100 + i * 100 ; turn.step = q.step + 1 ; if(!visit[turn.num]&&prime[turn.num]){ visit[turn.num] = 1 ; Q.push(turn) ; } } // 千位 for(int i=1 ; i<=9 ; i++){ turn.num = q.num % 1000 + i * 1000 ; turn.step = q.step + 1 ; if(!visit[turn.num]&&prime[turn.num]){ visit[turn.num] = 1 ; Q.push(turn) ; } } } printf("Impossible ") ;//失误之下 发现POJ的这道题 没有样例要求输出 Impossible return; } int main(){ init() ; int t ; scanf("%d" , &t) ; while(t--){ scanf("%d %d" , &num1 , &num2) ; st.num = num1 ; st.step = 0 ; result = 0 ; memset(visit , 0 , sizeof(visit)) ; visit[num1] = 1 ; BFS() ; } return 0 ; }