第一种 :springboot+mybatis+pageHelper
第二种: 利用 listMap.subList(firstIndex, lastIndex) ,listMap是数组,firstIndex,是开始元素,lastIndex是结束元素但不包括lastIndex。 (本人目前用的比较多)
第三种:利用数据库自身的limit关键字 limit
select * from table limit (pageNum-1)*pageSize,pageSize
第一种
1.概念:PageHelper 是一款好用的开源免费的 Mybatis 第三方物理分页插件,可以支持多个数据库,应用mybatis
2.引入依赖:
<!-- 这个pagehelper好像适用于spring,对springboot不适用,给个提醒-->
<!--<dependency>-->
<!--<groupId>com.github.pagehelper</groupId>-->
<!--<artifactId>pagehelper</artifactId>-->
<!--<version>5.0.3</version>-->
<!--</dependency>-->
<!--分页插件-->
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.2.3</version>
</dependency>
3.application.yml
server:
port: 8088
spring:
datasource:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/mybatis?serverTimezone=Asia/Shanghai&useUnicode=true&characterEncoding=utf-8&useSSL=false&allowMultiQueries=true
username: root
password: zhang
mybatis:
mapper-locations: classpath:mapper/*.xml
type-aliases-package: com.yiyezhiqiu.jwt.jwt.domain
configuration:
map-underscore-to-camel-case: true
logging:
level:
com:
yiyezhiqiu:
jwt:
jwt:
dao: debug
4.数据库表:
5.domain层
5.1.Goods实体类
package com.yiyezhiqiu.jwt.jwt.domain;
import lombok.Data;
import java.math.BigDecimal;
@Data
public class Goods {
private int id;
private String goodsType;
private BigDecimal goodsPrice;
private Double goodsWeight;
private String goodsShape;
}
5.2 分页传入参数实体类
package com.yiyezhiqiu.jwt.jwt.domain;
import lombok.Data;
/**
* 分页实体类
*/
@Data
public class PageHelpParam {
/**
* 当前页
*/
private String currentPageParam ;
/**
* 当前页条数
*/
private String pageSizeParam;
/**
* 模糊查询参数fuzzy
*/
private String fuzzy;
/**
* 模糊查询参数 nextFuzzy
*/
private String nextFuzzy;
}
6.service层
6.1 接口:IGoodsService
package com.yiyezhiqiu.jwt.jwt.service;
import com.yiyezhiqiu.jwt.jwt.domain.PageHelpParam;
import java.util.List;
import java.util.Map;
public interface IGoodsService {
public Map findPageHelp(PageHelpParam pageHelpParam);
}
6.2.实现类:GoodsServiceImpl
package com.yiyezhiqiu.jwt.jwt.service.impl;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import com.yiyezhiqiu.jwt.jwt.dao.GoodsDao;
import com.yiyezhiqiu.jwt.jwt.domain.Goods;
import com.yiyezhiqiu.jwt.jwt.domain.PageHelpParam;
import com.yiyezhiqiu.jwt.jwt.service.IGoodsService;
import com.yiyezhiqiu.jwt.jwt.service.IUsersService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.util.CollectionUtils;
import org.springframework.util.StringUtils;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* 实现分页
*/
@Service
@Slf4j
public class GoodsServiceImpl implements IGoodsService {
@Autowired
IGoodsService goodsService;
@Autowired
GoodsDao goodsDao;
@Override
public Map findPageHelp(PageHelpParam pageHelpParam) {
String currentPageParam = pageHelpParam.getCurrentPageParam();
String pageSizeParam = pageHelpParam.getPageSizeParam();
log.info("currentPageParam:"+currentPageParam);
log.info("pageSizeParam:"+pageSizeParam);
int currenPage = 1;
int pageSize = 10;
if(StringUtils.isEmpty(currentPageParam)){
currenPage = 1;
}else{
currenPage = Integer.parseInt(currentPageParam);
}
if(StringUtils.isEmpty(pageSizeParam)){
pageSize = 10;
}else{
pageSize = Integer.parseInt(pageSizeParam);
}
//分页条件查询参数
PageHelper.startPage(currenPage,pageSize);//页数和每页大小
/**
* 获得要分页的集合,
* 1.这里获得集合,如果你要给参数到数据库,比如模糊查询,就可以在PageHelpParam中添加相关字段(在实现了分页后,做了下模糊查询)
* 2.这里是返回某个表的实体类,当然你也可以用一个vo类,也就是不同表字段返回在一个vo类中使用
*/
String fuzzy = pageHelpParam.getFuzzy();
String nextFuzzy = pageHelpParam.getNextFuzzy();
Map<String,Object> mapParam = new HashMap<>();
if(null != fuzzy && fuzzy != ""){
mapParam.put("fuzzy",fuzzy);
}
if(null != nextFuzzy && fuzzy != ""){
mapParam.put("nextFuzzy",nextFuzzy);
}
List<Goods> goodsList = goodsDao.findAll(mapParam);
//这里可以看下PageInfo为啥会和PageHelper有关系,因为startPage会返回page,PageInfo中使用page,这就是联系,可以去看源码
PageInfo<Goods> pageInfo = new PageInfo<Goods>(goodsList);
List<Goods> goods = new ArrayList<>();
if(!CollectionUtils.isEmpty(goodsList)){
for(Goods good:pageInfo.getList()){
goods.add(good);
}
}
Map<String,Object> map = new HashMap<>();
map.put("total",pageInfo.getTotal());
map.put("currentPage",pageInfo.getPageNum());
map.put("pageSize", pageInfo.getPageSize());
Map<String ,Object> maps = new HashMap<>();
maps.put("pageInfo",map);
maps.put("goods",goods);
return maps;
}
}
7.controller
package com.yiyezhiqiu.jwt.jwt.controller;
import com.github.pagehelper.PageHelper;
import com.yiyezhiqiu.jwt.jwt.annotation.OtherPermit;
import com.yiyezhiqiu.jwt.jwt.domain.PageHelpParam;
import com.yiyezhiqiu.jwt.jwt.service.IGoodsService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import java.util.Map;
@RestController
@RequestMapping("/goods")
public class GoodsController {
@Autowired
IGoodsService goodsService;
@RequestMapping(value = "/findGoods",method = RequestMethod.GET)
public Map findGoodsPageHelper(PageHelpParam pageHelpParam){
Map<String,Object> map = goodsService.findPageHelp(pageHelpParam);
return map;
}
}
8.GoodsMapping.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.yiyezhiqiu.jwt.jwt.dao.GoodsDao">
<!--这里
IFNULL(A,B) 意思是如果A不为空,则为A,否则为B
CONCAT(多个IFNULL)实现一个框就可以分别对多个字段进行模糊查询
-->
<select id="findAll" resultType="com.yiyezhiqiu.jwt.jwt.domain.Goods">
select * from goods
<where>
<if test= "fuzzy != null">
IFNULL(goods_type ," ") like "%"#{fuzzy}"%"
</if>
<if test="nextFuzzy != null">
and CONCAT(IFNULL(goods_price," "),IFNULL(goods_shape," ")) like "%"#{nextFuzzy}"%"
</if>
</where>
</select>
</mapper>
9.postman测试:
9.1:
当只为分页时,不模糊筛选
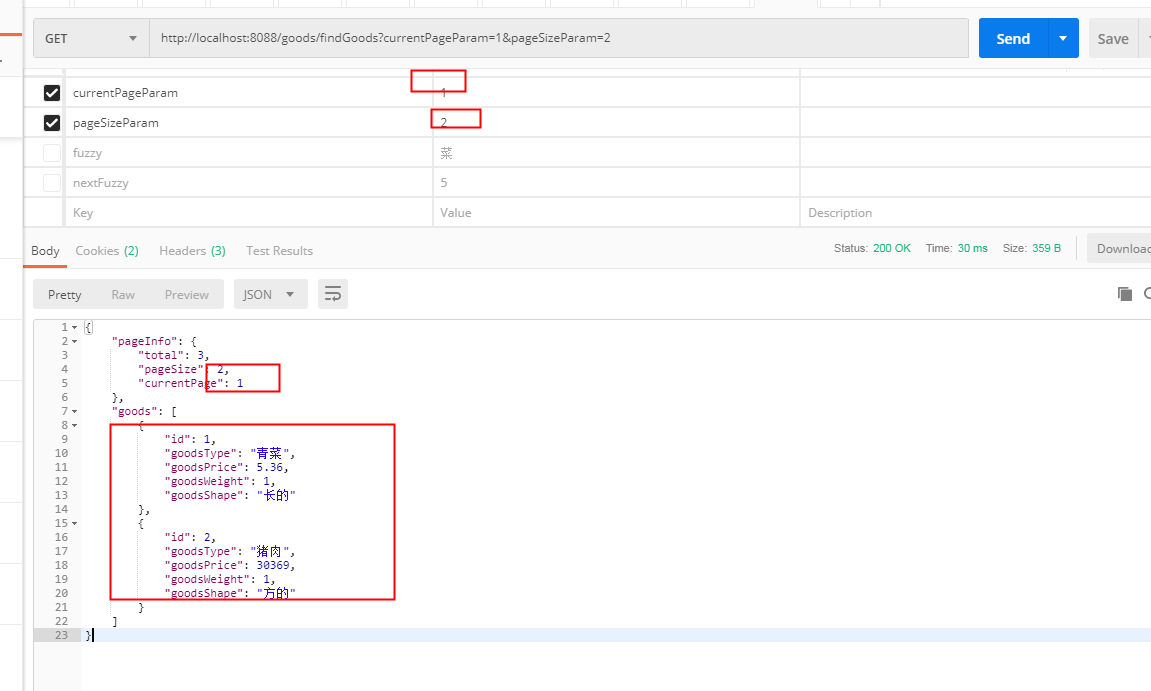
9.2:
当为输入goods_type单个参数筛选时
9.3 :
当可以是goods_weight 或者goods_shape筛选时
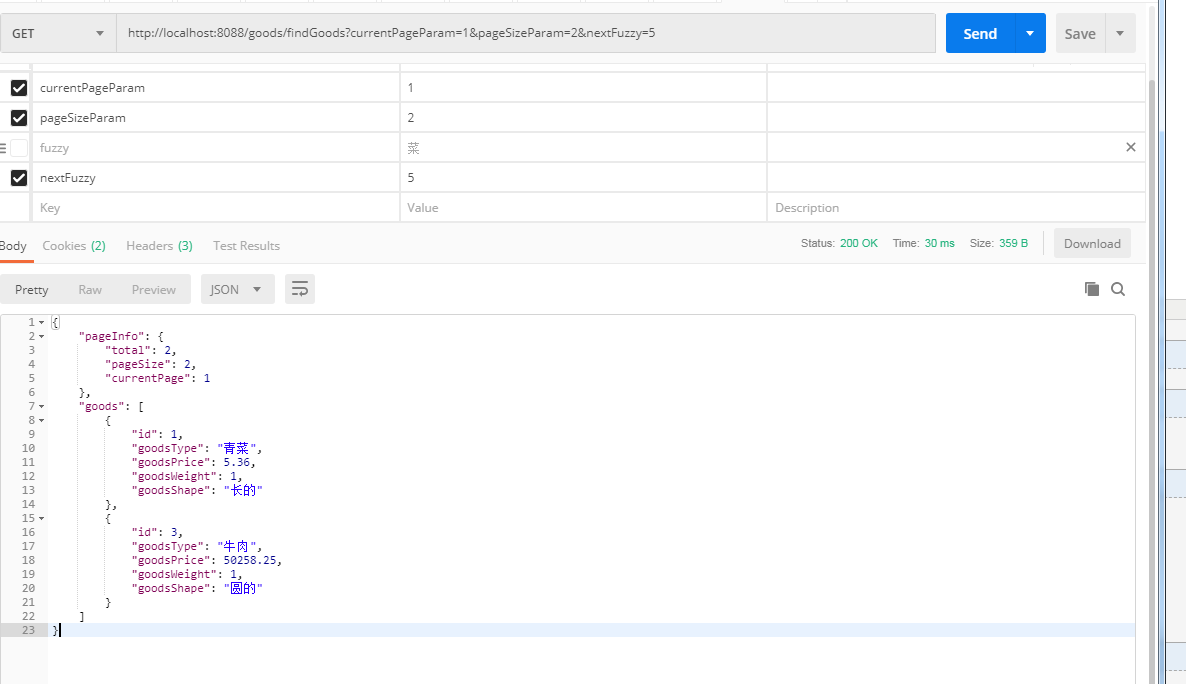
github地址:https://github.com/yiyeluowuchen/springboot-jwt-token.git