Flip Game
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 48663 | Accepted: 20724 |
Description
Flip game is played on a rectangular 4x4 field with two-sided pieces placed on each of its 16 squares. One side of each piece is white and the other one is black and each piece is lying either it's black or white side up. Each round you flip 3 to 5 pieces, thus changing the color of their upper side from black to white and vice versa. The pieces to be flipped are chosen every round according to the following rules:
Consider the following position as an example:
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
- Choose any one of the 16 pieces.
- Flip the chosen piece and also all adjacent pieces to the left, to the right, to the top, and to the bottom of the chosen piece (if there are any).
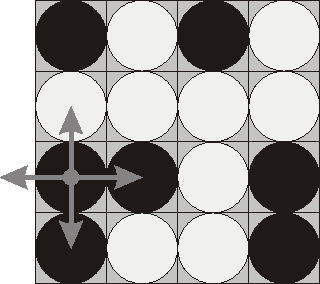
bwbw
wwww
bbwb
bwwb
Here "b" denotes pieces lying their black side up and "w" denotes pieces lying their white side up. If we choose to flip the 1st piece from the 3rd row (this choice is shown at the picture), then the field will become:
bwbw
bwww
wwwb
wwwb
The goal of the game is to flip either all pieces white side up or all pieces black side up. You are to write a program that will search for the minimum number of rounds needed to achieve this goal.
Input
The input consists of 4 lines with 4 characters "w" or "b" each that denote game field position.
Output
Write to the output file a single integer number - the minimum number of rounds needed to achieve the goal of the game from the given position. If the goal is initially achieved, then write 0. If it's impossible to achieve the goal, then write the word "Impossible" (without quotes).
Sample Input
bwwb
bbwb
bwwb
bwww
Sample Output
4
Source
题目大意:
有一个 4*4 的矩阵,然后现在每个格子有两个颜色,要么是黑色,要么是白色,当你的手按动一个格子的时候,这个格子的上、下、左、右都会
改变原来的颜色,即由黑变白,由白变黑,现在问你的是,经过最少几次翻转之后可以使得这个 4*4 的矩阵变为全是白色的或者是全是黑色的。
有一个 4*4 的矩阵,然后现在每个格子有两个颜色,要么是黑色,要么是白色,当你的手按动一个格子的时候,这个格子的上、下、左、右都会
改变原来的颜色,即由黑变白,由白变黑,现在问你的是,经过最少几次翻转之后可以使得这个 4*4 的矩阵变为全是白色的或者是全是黑色的。
解题思路:
1)使用DFS
每格棋子最多只可以翻转1次,
这是因为只要其中一格重复翻了2次(不论是连续翻动还是不连翻动),
那么它以及周边的棋子和没翻动时的状态是一致的。
算法停止条件就是当前翻转的棋子个数>=17个 以及 当前棋盘棋子为同一颜色
剪枝条件即为当前翻转棋子的个数>最优解bestx,则进行回溯
由于我们需要考虑的其实就是每一个棋子翻不翻转,所以对于已经考察过的点需要进行剪枝。
1 #include<iostream>
2 using namespace std;
3 int color[16];
4 int a[16];
5 int bestx;
6 bool checkColor()
7 {
8 int a = color[0];
9 for(int i=1;i<16;i++)
10 {
11 if(a != color[i])
12 {
13 return 0;//存在不同色
14 }
15 }
16 return 1;//同色
17 }
18 void BackTrack(int t,int pre)
19 {
20 if(checkColor() || t>=17)//如果同色
21 {//更新解
22 if(checkColor())
23 {
24 if(t-1<bestx)
25 bestx = t-1;
26 }
27 return;
28 } else{
29 for(int i=pre;i<16;i++)
30 {
31 if(a[i] == 0)//没有翻过
32 {
33 //进行翻牌
34 a[i] = 1;
35 color[i] = 1-color[i];
36 if(i-4>=0) color[i-4] = 1-color[i-4];
37 if(i+4<=15) color[i+4] = 1-color[i+4];
38 if(i-1>=0 && (i-1)%4!=3) color[i-1] = 1-color[i-1];
39 if(i+1<=15 && (i+1)%4!=0) color[i+1] = 1-color[i+1];
40
41 if(t<bestx)//剪枝
42 BackTrack(t+1,i+1);
43
44 a[i] = 0;//回溯
45 color[i] = 1-color[i];
46 if(i-4>=0) color[i-4] = 1-color[i-4];
47 if(i+4<=15) color[i+4] = 1-color[i+4];
48 if(i-1>=0 && (i-1)%4!=3) color[i-1] = 1-color[i-1];
49 if(i+1<=15 && (i+1)%4!=0) color[i+1] = 1-color[i+1];
50 }
51 }
52
53 }
54
55
56 }
57 int main()
58 {
59 while(true)
60 {
61 bestx=18;
62 for(int i=0;i<16;i++)
63 a[i]=0;
64 char c;
65 for(int i = 0;i<16;i++)
66 {
67 if(i % 4 == 0 && i!=0)
68 {
69 c = getchar();
70 }
71 c = getchar();
72 if(c == EOF) return 0;
73 if(c == 'b') color[i] = 1;
74 else if(c == 'w') color[i] = 0;
75
76 }
77 BackTrack(1,0);
78 if(bestx == 18)
79 cout<<"Impossible"<<endl;
80 else
81 cout<<bestx<<endl;
82 }
83
84 return 0;
85 }