Sudoku
Time Limit : 4000/2000ms (Java/Other) Memory Limit : 131072/65536K (Java/Other)
Total Submission(s) : 15 Accepted Submission(s) : 12
Special Judge
Problem Description
Sudoku is a very simple task. A square table with 9 rows and 9 columns is divided to 9 smaller squares 3x3 as shown on the Figure. In some of the cells are written decimal digits from 1 to 9. The other cells are empty. The goal is to fill the empty cells with decimal digits from 1 to 9, one digit per cell, in such way that in each row, in each column and in each marked 3x3 subsquare, all the digits from 1 to 9 to appear. Write a program to solve a given Sudoku-task.
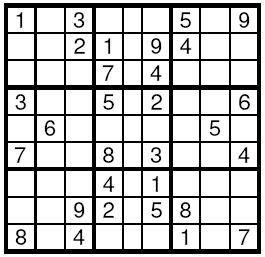
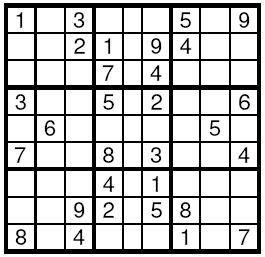
Input
The input data will start with the number of the test cases. For each test case, 9 lines follow, corresponding to the rows of the table. On each line a string of exactly 9 decimal digits is given, corresponding to the cells in this line. If a cell is empty it is represented by 0.
Output
For each test case your program should print the solution in the same format as the input data. The empty cells have to be filled according to the rules. If solutions is not unique, then the program may print any one of them.
Sample Input
1
103000509
002109400
000704000
300502006
060000050
700803004
000401000
009205800
804000107
Sample Output
143628579
572139468
986754231
391542786
468917352
725863914
237481695
619275843
854396127
Source
PKU
题意:
九宫格问题,也有人叫数独问题
把一个9行9列的网格,再细分为9个3*3的子网格
如图
要求每行、每列、每个子网格内都只能使用一次1~9中的一个数字,即每行、每列、每个子网格内都不允许出现相同的数字。
0是待填位置,其他均为已填入的数字。
要求填完九宫格并输出(如果有多种结果,则只需输出其中一种)
如果给定的九宫格无法按要求填出来,则输出原来所输入的未填的九宫格
思路:
判断某一个不为零的格子,看看它的本行本列以及相联系的一个宫已有那些数字,然后再把
没有数字依次往里面填,填好这个方格后继续填写下一个方格,如果走到后面走不通了,就应该返回来,把
刚刚它的那个数字还原为零,这样好下一次再继续填写另外的数,如果所有的方格都填完了,整个回溯也就结束了。
AC代码:

1 #include<cstdio> 2 #include<cstdlib> 3 #include<iostream> 4 #include<algorithm> 5 #include<cstring> 6 #include<string> 7 #include<cmath> 8 9 using namespace std; 10 11 12 int s[12][12]={0};//存储矩阵 13 bool row[12][12];//row[i][j]表示第i行有没有j这给数字 14 bool col[12][12];//col[i][j]表示第i列有没有j这给数字 15 bool grid[12][12];//grid[k][j]表示第k个九宫格有没有j这给数字 16 17 18 19 bool DFS(int x,int y) 20 { 21 if(x==10) 22 return true; 23 bool sgin=false; 24 if(s[x][y]!=0){ 25 if(y==9) 26 sgin=DFS(x+1,1); 27 else 28 sgin=DFS(x,y+1); 29 if(sgin) 30 return true; 31 else 32 return false; 33 } 34 else{ 35 int k=3*((x-1)/3)+(y-1)/3+1; 36 for(int i=1;i<=9;i++) 37 if(!row[x][i]&&!col[y][i]&&!grid[k][i]){//确定放入第x行,第y列,第k个九宫格都可以数 38 s[x][y]=i; 39 row[x][i]=true; 40 col[y][i]=true; 41 grid[k][i]=true; 42 if(y==9) 43 sgin=DFS(x+1,1); 44 else 45 sgin=DFS(x,y+1); 46 if(sgin) 47 return true; 48 else {//这个放入不可以,则返回上一层; 49 row[x][i]=false; 50 col[y][i]=false; 51 grid[k][i]=false; 52 s[x][y]=0; 53 } 54 } 55 } 56 return false; 57 } 58 59 60 61 62 int main() 63 { 64 // freopen("1.txt","r",stdin); 65 int test; 66 cin>>test; 67 char a; 68 while(test){ 69 memset(s,0,sizeof(s)); 70 memset(row,false,sizeof(row)); 71 memset(col,false,sizeof(col)); 72 memset(grid,false,sizeof(grid)); 73 for(int i=1;i<=9;i++){ 74 for(int j=1;j<=9;j++){ 75 cin>>a; 76 s[i][j]=a-'0'; 77 if(s[i][j]){//本来就不是0,进行排除 78 int k=((i-1)/3)*3+(j-1)/3+1; 79 row[i][s[i][j]]=true; 80 col[j][s[i][j]]=true; 81 grid[k][s[i][j]]=true; 82 } 83 } 84 } 85 DFS(1,1); 86 for(int i=1;i<=9;i++){ 87 for(int j=1;j<=9;j++){ 88 cout<<s[i][j]; 89 } 90 cout<<endl; 91 } 92 test--; 93 } 94 return 0; 95 }