功能介绍:
1. 角色切换(快递员和普通用户)

快递员功能:存快递,删除快递,修改快递,查看所有快递
普通用户功能:取快递
2. 存快递
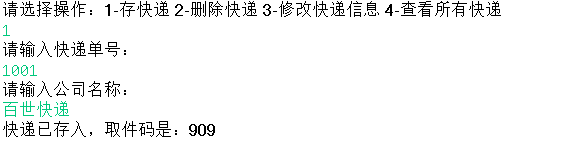
3.删除快递
4.修改快递
5.查看所有快递
6.取快递
代码:
1 import java.util.Random; 2 import java.util.Scanner; 3 4 public class Express { 5 6 private static Scanner scanner = new Scanner(System.in); 7 static Express5[] ex = new Express5[10]; 8 public static void add() { 9 System.out.println("请输入快递单号:"); 10 int tracking0 = scanner.nextInt(); 11 System.out.println("请输入公司名称:"); 12 String company0 = scanner.next(); 13 Express5 e = new Express5(); 14 int c = code(); 15 e.setCode(c); 16 e.setCompany(company0); 17 e.setNumber(tracking0); 18 for (int i = 0; i < ex.length; i++) { 19 if (ex[i] == null) { 20 ex[i]=e; 21 break; 22 } 23 } 24 System.out.println("快递已存入,取件码是:"+c); 25 } 26 public static int code() { 27 Random random = new Random(); 28 return random.nextInt(899) + 100; 29 } 30 public static void delete() { 31 System.out.println("请输入要删除的快递单号:"); 32 int tracking1 = scanner.nextInt(); 33 for(int i = 0;i < ex.length;i ++) { 34 if(ex[i] == null) { 35 System.out.println("未找到快递"); 36 break; 37 }else if(ex[i].getNumber() == tracking1) { 38 ex[i] = null; 39 System.out.println("删除成功"); 40 break; 41 } 42 } 43 } 44 public static void change() { 45 System.out.println("请输入要修改的快递单号:"); 46 int tracking1 = scanner.nextInt(); 47 for(int i = 0;i < ex.length;i ++) { 48 Express5 e = new Express5(); 49 if(ex[i] == null) { 50 System.out.println("未找到快递"); 51 break; 52 }else if(ex[i].getNumber() == tracking1) { 53 System.out.println("请输入新的快递单号:"); 54 int tracking3 = scanner.nextInt(); 55 System.out.println("请输入新的公司名称:"); 56 String company1 = scanner.next(); 57 e.setCode(code()); 58 e.setNumber(tracking3); 59 e.setCompany(company1); 60 ex[i] = e; 61 System.out.println("修改成功"); 62 break; 63 } 64 } 65 } 66 67 public static void find() { 68 System.out.println("所有快递信息如下:"); 69 System.out.println("快递单号 公司名称 取件码"); 70 for (int i = 0; i < ex.length; i++) { 71 if (ex[i] != null) { 72 System.out.println(ex[i].number + " " + ex[i].company + " " + ex[i].code); 73 } 74 } 75 } 76 77 public static void delete2() { 78 System.out.println("请输入取件码:"); 79 int tracking1 = scanner.nextInt(); 80 for(int i = 0;i < ex.length;i ++) { 81 if(ex[i] == null) { 82 System.out.println("未找到快递"); 83 break; 84 }else if(ex[i].getCode() == tracking1) { 85 ex[i] = null; 86 System.out.println("取件成功"); 87 break; 88 } 89 } 90 } 91 92 public static void main(String[] args) { 93 while(true) { 94 System.out.println("=====欢迎使用快递柜====="); 95 System.out.println("请输入您的身份:1-快递员,2-用户"); 96 Scanner scanner = new Scanner(System.in); 97 int a = scanner.nextInt(); 98 if(a == 1) { 99 System.out.println("请选择操作:1-存快递 2-删除快递 3-修改快递信息 4-查看所有快递"); 100 int b = scanner.nextInt(); 101 if (b == 1) { 102 add(); 103 } else if (b == 2) { 104 delete(); 105 } else if (b == 3) { 106 change(); 107 } else if (b == 4) { 108 find(); 109 } else { 110 System.out.println("输入有误,请重新输入"); 111 } 112 }else if(a == 2) { 113 delete2(); 114 } 115 } 116 117 } 118 } 119 120 121 122 class Express5 { 123 124 int code; 125 String company; 126 int number; 127 128 public int getCode() { 129 return code; 130 } 131 132 public void setCode(int code) { 133 this.code = code; 134 } 135 136 public String getCompany() { 137 return company; 138 } 139 140 public void setCompany(String company) { 141 this.company = company; 142 } 143 144 public int getNumber() { 145 return number; 146 } 147 148 public void setNumber(int number) { 149 this.number = number; 150 } 151 }