plt.plot()绘制线性图
- 绘制单条线形图
- 绘制多条线形图
- 设置坐标系的比例plt.figure(figsize=(a,b))
- 设置图例legend()
- 设置轴的标识
- 图例保存
- fig = plt.figure()
- plt.plot(x,y)
- figure.savefig()
- 曲线的样式和风格
import matplotlib.pyplot as plt
import numpy as np
x = [1,2,3,4,5]
y = [5,4,3,2,1]
plt.plot(x,y)
[<matplotlib.lines.Line2D at 0x2496ac94908>]
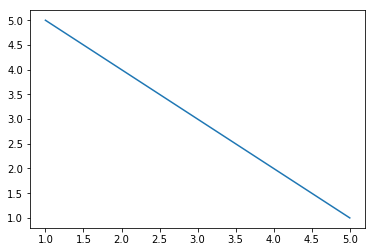
x = np.linspace(-np.pi,np.pi,40)
y = x**2
plt.plot(x,y)
[<matplotlib.lines.Line2D at 0x2496b05aba8>]
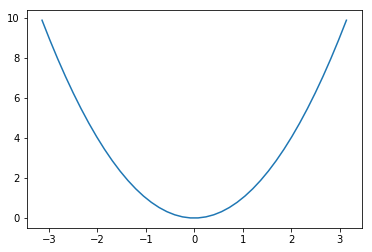
#在一个坐标系中绘制多条曲线
plt.plot(x,y)
plt.plot(x-1,y+2)
[<matplotlib.lines.Line2D at 0x2496b07b828>]
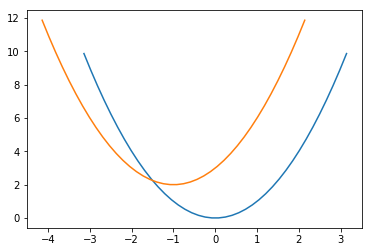
#给x,y设定标识
plt.plot(x,y)
plt.xlabel('bobo')
plt.ylabel('score')
plt.title('aaa')
Text(0.5,1,'aaa')
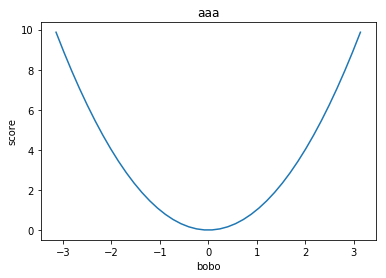
#设置图例大小
plt.figure(figsize=(10,10))
plt.plot(x,y)
[<matplotlib.lines.Line2D at 0x2496cd811d0>]
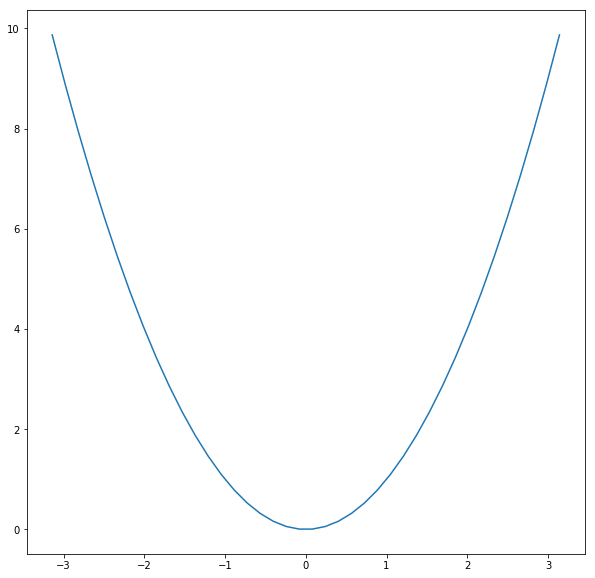
#设置图例legend()
plt.plot(x,y,label='hello')
plt.plot(x-1,y+2,label='hey')
plt.legend(loc=4)
<matplotlib.legend.Legend at 0x2496b940940>
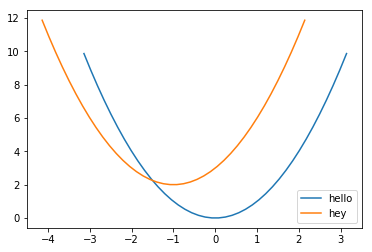
#保存图例
#1.实例化一个对象
fig = plt.figure()
#2.画图
plt.plot(x,y,label='hello')
plt.plot(x-1,y+2,label='hey')
plt.legend(loc=4)
#3.保存
fig.savefig('./123.png')
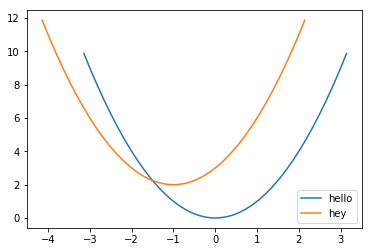
柱状图:plt.bar()
- 参数:第一个参数是索引。第二个参数是数据值。第三个参数是条形的宽度
x = [1,2,3,4,5]#x轴的刻度
y = [2,3,4,5,6]#柱子的高度
plt.bar(x,y)
<Container object of 5 artists>
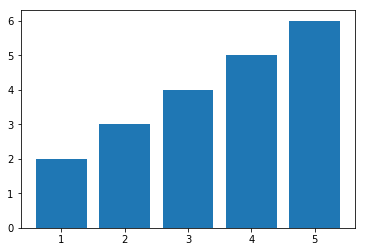
直方图
- 是一个特殊的柱状图,又叫做密度图
- plt.hist()的参数
- bins
可以是一个bin数量的整数值,也可以是表示bin的一个序列。默认值为10
- normed
如果值为True,直方图的值将进行归一化处理,形成概率密度,默认值为False
- color
指定直方图的颜色。可以是单一颜色值或颜色的序列。如果指定了多个数据集合,例如DataFrame对象,颜色序列将会设置为相同的顺序。如果未指定,将会使用一个默认的线条颜色
- orientation
通过设置orientation为horizontal创建水平直方图。默认值为vertical
x = [1,1,2,3,4,5,5,5,6,7,7,7,7,7,7,8]
plt.hist(x,bins=15)#柱子的个数
(array([2., 0., 1., 0., 1., 0., 1., 0., 3., 0., 1., 0., 6., 0., 1.]),
array([1. , 1.46666667, 1.93333333, 2.4 , 2.86666667,
3.33333333, 3.8 , 4.26666667, 4.73333333, 5.2 ,
5.66666667, 6.13333333, 6.6 , 7.06666667, 7.53333333,
8. ]),
<a list of 15 Patch objects>)
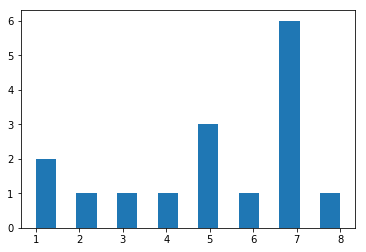
饼图
- pie(),饼图也只有一个参数x
- 饼图适合展示各部分占总体的比例,条形图适合比较各部分的大小
arr=[11,22,31,15]
plt.pie(arr)
([<matplotlib.patches.Wedge at 0x2496d446048>,
<matplotlib.patches.Wedge at 0x2496d446518>,
<matplotlib.patches.Wedge at 0x2496d446a20>,
<matplotlib.patches.Wedge at 0x2496d446f60>],
[Text(0.996424,0.465981,''),
Text(-0.195798,1.08243,''),
Text(-0.830021,-0.721848,''),
Text(0.910034,-0.61793,'')])
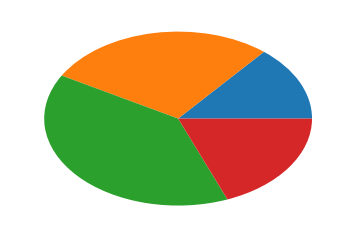
arr=[0.2,0.3,0.1]
plt.pie(arr)
([<matplotlib.patches.Wedge at 0x2496d0ccac8>,
<matplotlib.patches.Wedge at 0x2496d0ccf98>,
<matplotlib.patches.Wedge at 0x2496d1c6518>],
[Text(0.889919,0.646564,''),
Text(-0.646564,0.889919,''),
Text(-1.04616,-0.339919,'')])
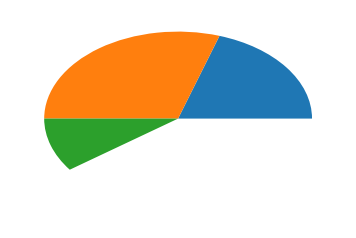
arr=[11,22,31,15]
plt.pie(arr,labels=['a','b','c','d'])
([<matplotlib.patches.Wedge at 0x2496d063240>,
<matplotlib.patches.Wedge at 0x2496d063710>,
<matplotlib.patches.Wedge at 0x2496d063c50>,
<matplotlib.patches.Wedge at 0x2496d0651d0>],
[Text(0.996424,0.465981,'a'),
Text(-0.195798,1.08243,'b'),
Text(-0.830021,-0.721848,'c'),
Text(0.910034,-0.61793,'d')])
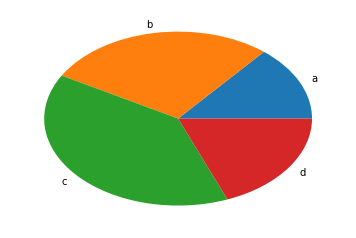
arr=[11,22,31,15]
plt.pie(arr,labels=['a','b','c','d'],labeldistance=0.3)
([<matplotlib.patches.Wedge at 0x2496d1dbe10>,
<matplotlib.patches.Wedge at 0x2496d1e52b0>,
<matplotlib.patches.Wedge at 0x2496d1e57f0>,
<matplotlib.patches.Wedge at 0x2496d1e5d30>],
[Text(0.271752,0.127086,'a'),
Text(-0.0533994,0.295209,'b'),
Text(-0.226369,-0.196868,'c'),
Text(0.248191,-0.168526,'d')])
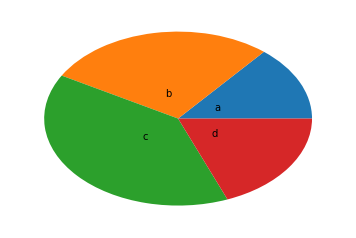
arr=[11,22,31,15]
plt.pie(arr,labels=['a','b','c','d'],labeldistance=0.3,autopct='%.6f%%')
([<matplotlib.patches.Wedge at 0x2496d227940>,
<matplotlib.patches.Wedge at 0x2496d230080>,
<matplotlib.patches.Wedge at 0x2496d2307f0>,
<matplotlib.patches.Wedge at 0x2496d230f60>],
[Text(0.271752,0.127086,'a'),
Text(-0.0533994,0.295209,'b'),
Text(-0.226369,-0.196868,'c'),
Text(0.248191,-0.168526,'d')],
[Text(0.543504,0.254171,'13.924050%'),
Text(-0.106799,0.590419,'27.848101%'),
Text(-0.452739,-0.393735,'39.240506%'),
Text(0.496382,-0.337053,'18.987341%')])
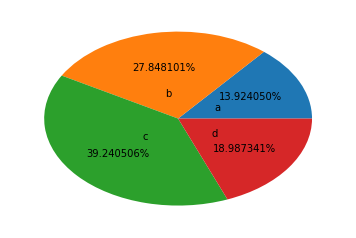
arr=[11,22,31,15]
plt.pie(arr,labels=['a','b','c','d'],labeldistance=0.3,shadow=True,explode=[0.2,0.3,0.2,0.4])
([<matplotlib.patches.Wedge at 0x2496d26ee48>,
<matplotlib.patches.Wedge at 0x2496d277630>,
<matplotlib.patches.Wedge at 0x2496d277e48>,
<matplotlib.patches.Wedge at 0x2496d27f6a0>],
[Text(0.45292,0.21181,'a'),
Text(-0.106799,0.590419,'b'),
Text(-0.377282,-0.328113,'c'),
Text(0.579113,-0.393228,'d')])
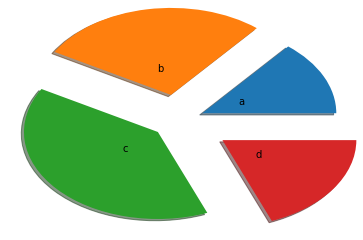
散点图scatter()
x = np.array([1,3,5,7,9])
y = x ** 2
plt.scatter(x,y)
<matplotlib.collections.PathCollection at 0x2496eb66940>
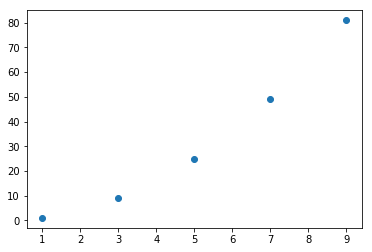
x = np.random.random((60,))
y = np.random.random((60,))
plt.scatter(x,y)
<matplotlib.collections.PathCollection at 0x2496eba82b0>
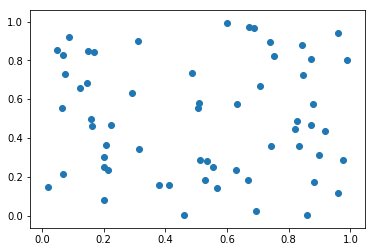