The string "PAYPALISHIRING"
is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility)
P A H N A P L S I I G Y I RAnd then read line by line:
"PAHNAPLSIIGYIR"
Write the code that will take a string and make this conversion given a number of rows:
string convert(string text, int nRows);
convert("PAYPALISHIRING", 3)
should return "PAHNAPLSIIGYIR"
.分析
可以从zig-zag数组中找到数学规律:
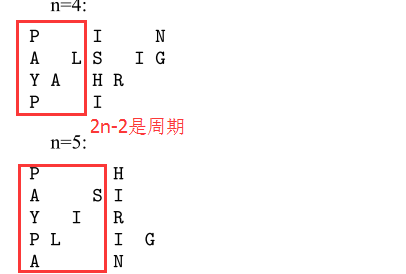
i 代表行index,j代表第几个周期
(i,j) 代表的就是第i行,第j周期的字母
当i是首行和最后一行,只有垂直线的一个字母,
其他情况,会有两个字母:垂直线上一个,斜线上一个,二者在源字符串的index为:
i + j * (2n - 2)
i + j * (2n - 2) + 2(n - 1 - i)
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | class Solution { public : string convert(string s, int numRows) { if (numRows <= 1 || s.size() <= 1 ) return s; string result; for ( int i = 0; i < numRows; ++i){ for ( int j = 0,index = i; index < s.size(); ++j, index = i + (2*numRows-2)*j){ result.append(1,s[index]); if (i != 0 && i != numRows - 1) { index += 2*(numRows-1-i); if (index < s.size()) result.append(1,s[index]); } } } return result; } }; |