1、要求:某商品管理系统的商品名称存储在一个字符串数组中,现需要自定义一个双向迭代器(MyIterator)实现对该商品名称数组的双向(向前和向后)遍历。使用Iterator模式来设计。
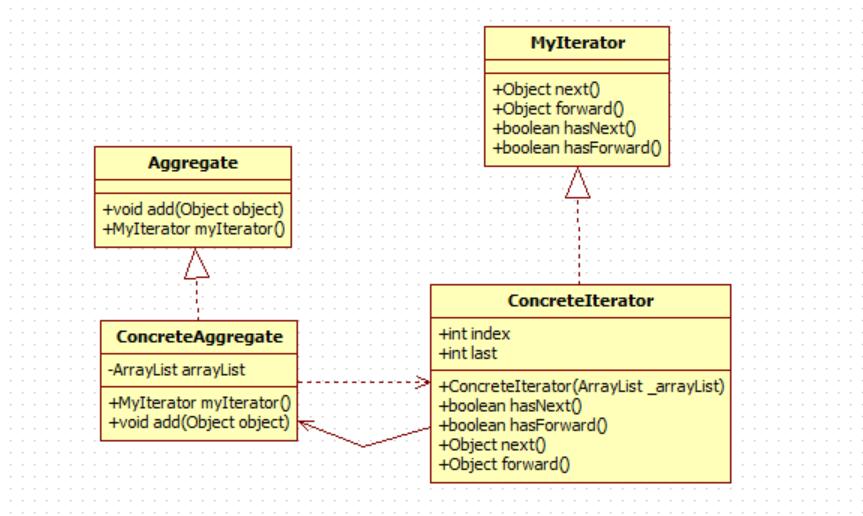
//抽象容器
public interface Aggregate {
public void add(Object object);
public MyIterator myIterator();
}
public class ConcreteAggregate implements Aggregate {
private ArrayList arrayList = new ArrayList();
//返回迭代器对象
public MyIterator myIterator(){
return new ConcreteIterator(this);
}
public void add(Object object){
this.arrayList.add(object);
}
public ArrayList getArrayList(){
return this.arrayList;
}
}
//抽象迭代器
public interface MyIterator {
//遍历下一个商品
public Object next();
//遍历上一个商品
public Object forward();
//是否已经遍历完
public boolean hasNext();
//从后面开始遍历
public boolean hasForward();
}
public class ConcreteIterator implements MyIterator {
private ArrayList arrayList = new ArrayList();
private ConcreteAggregate concreteAggregate;
public int index = 0;
public int last =0;
public ConcreteIterator(ConcreteAggregate concreteAggregate){
this.concreteAggregate = concreteAggregate;
this.arrayList = this.concreteAggregate.getArrayList();
last=this.arrayList.size()-1; }
public boolean hasNext(){
if (this.index == this.arrayList.size()){
return false;
}else {
return true;
}
}
public boolean hasForward(){
if (this.last<0){
return false;
}else {
return true;
}
}
//向下遍历
public Object next(){
Object result = null;
if (this.hasNext()){
result = this.arrayList.get(this.index++);
}else {
result = null;
}
return result;
}
//向上遍历
public Object forward(){
Object result = null;
if (this.hasForward()){
result = this.arrayList.get(this.last--);
}else {
result = null;
}
return result;
}
}
//测试类
public class Client {
public static void main(String[] args){
//声明出容器
Aggregate aggregate = new ConcreteAggregate();
aggregate.add("牙刷");
aggregate.add("杯子");
aggregate.add("糖果");
//遍历
MyIterator myIterator = aggregate.myIterator();
System.out.println("向下遍历");
while (myIterator.hasNext()){
System.out.println(myIterator.next());
}
System.out.println("向上遍历");
while (myIterator.hasForward()){
System.out.println(myIterator.forward());
}
}
}
输出结果:
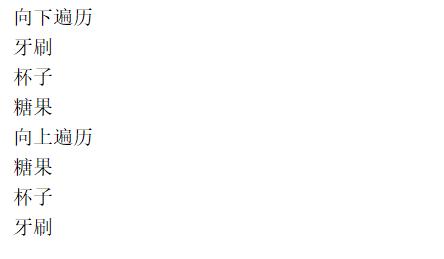
2、某公司欲开发一个基于Windows平台的公告板系统,系统提供主菜单(Menu)其中有菜单项(MenuItem)。通过Menu类的addMenuItem()方法增加菜单项。菜单项的打开方法是click(),每个菜单项包含一个抽象命令类,具体命令类包括OpenCommand()、CreateCommand()、EditCommand()等,命令类具有一个execute()方法,用于调用公告板系统界面类(BoardScreen())的open()、create()、edit())等方法。使用Command模式来设计。
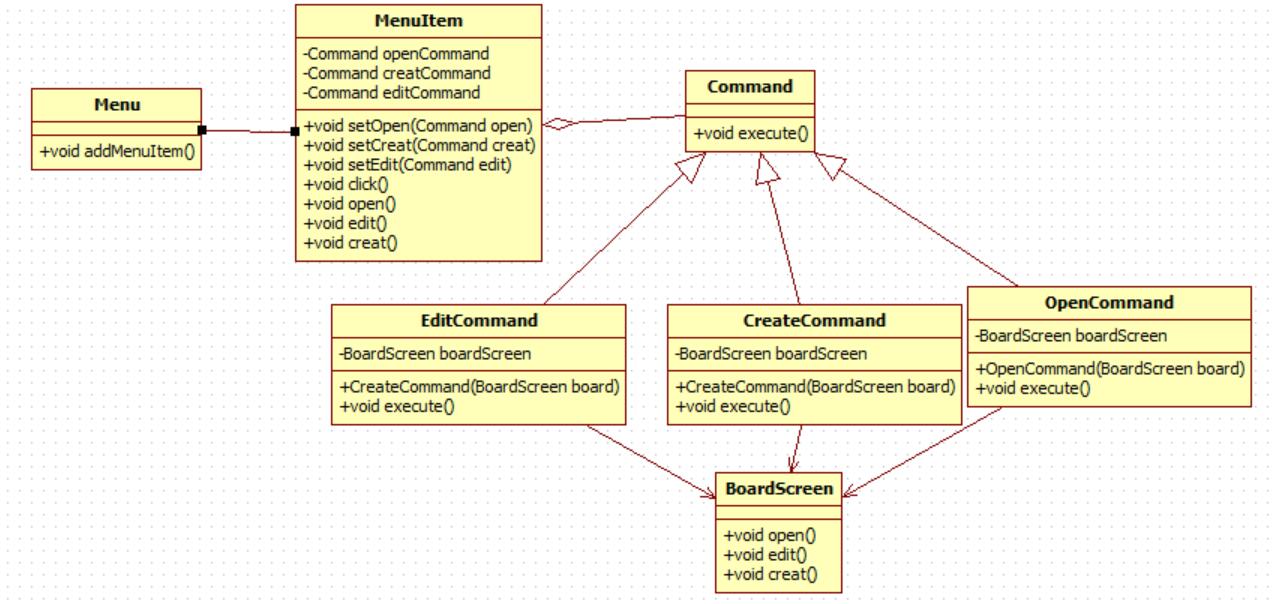
public class Menu {
public MenuItem addMenuItem(){
return new MenuItem();
}
}
//Invoker 发送命令的人
public class MenuItem {
private Command openCommand;
private Command creatCommand;
private Command editCommand;
public void setOpen(Command open){
this.openCommand = open;
}
public void setCreat(Command creat){
this.creatCommand = creat;
}
public void setEdit(Command edit){
this.editCommand = edit;
}
public void click(){System.out.println("点击");}
public void open(){
openCommand.execute();
}
public void edit(){
editCommand.execute();
}
public void creat(){
creatCommand.execute();
}
}
public abstract class Command {
public abstract void execute();
}
public class CreateCommand extends Command {
private BoardScreen boardScreen;
public CreateCommand(BoardScreen board){
boardScreen = board;
}
public void execute(){
boardScreen.edit();
}
}
public class EditCommand extends Command {
private BoardScreen boardScreen;
public EditCommand(BoardScreen board){
boardScreen = board;
}
public void execute(){
boardScreen.crate();
}
}
public class OpenCommand extends Command {
private BoardScreen boardScreen;
public OpenCommand(BoardScreen board){
boardScreen =board;
}
public void execute(){
boardScreen.open();
}
}
public class BoardScreen {
public void open(){
System.out.println("打开");
}
public void crate(){
System.out.println("创建");
}
public void edit(){
System.out.println("编辑");
}
}
public class Client {
public static void main(String[] args){
Menu menu = new Menu();
//创建请求命令对象
MenuItem menuItem =menu.addMenuItem();
//创建接受者对象
BoardScreen boardScreen = new BoardScreen();
//创建命令对象
Command open = new OpenCommand(boardScreen);
Command creat = new CreateCommand(boardScreen);
Command edit= new EditCommand(boardScreen);
//创建请求者
menuItem.click();
menuItem.setCreat(creat);
menuItem.setEdit(edit);
menuItem.setOpen(open);
menuItem.creat();
menuItem.edit();
menuItem.open();
}
}
输出结果
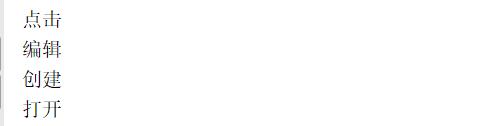

//抽象中介者
public abstract class AbstractChatRoom {
protected CommonMember commonMember;
protected DiamondMember diamondMember;
//构造函数
public AbstractChatRoom(){
commonMember = new CommonMember(this);
diamondMember = new DiamondMember(this);
}
//处理多个对象之间的关系
public abstract void execute(String str,String filter,Integer imgSize);
}
//具体中介者
public class ChatRoom extends AbstractChatRoom {
public void execute(String str,String filter,Integer imgSize){
if (str.equals("CommonMember")){
System.out.println("普通会员");
this.sentText(filter);
System.out.println();
}
if (str.equals("DiamondMember")){
System.out.println("钻石会员");
this.sentText(filter);
this.setPicture(imgSize);
}
}
public void sentText(String filter){
System.out.println("发送文本信息");
if (filter.equals("TMD")){
System.out.println("过滤不雅字符");
}
}
public void setPicture(Integer imgSize){
System.out.println("可以发送图片信息");
if(imgSize>3){
System.out.println("对图片大小进行控制");
}
}
}
//抽象同事类
public abstract class AbstractMember {
protected AbstractChatRoom chatRoom;
public AbstractMember(AbstractChatRoom _ab){
this.chatRoom = _ab;
}
}
public class CommonMember extends AbstractMember{
public CommonMember(AbstractChatRoom _chatRoom){
super(_chatRoom);
}
public void chat(String filter){
super.chatRoom.execute( "CommonMember",filter,null);
}
}
public class DiamondMember extends AbstractMember {
public DiamondMember(AbstractChatRoom _chatRoom){
super(_chatRoom);
}
public void chat(String filter,Integer imgSize){
super.chatRoom.execute("DiamondMember",filter,imgSize);
}
}
public class Client {
public static void main(String[] args){
//声明中介者
AbstractChatRoom chatRoom = new ChatRoom();
//声明同事
CommonMember commonMember = new CommonMember(chatRoom);
commonMember.chat("TMD");
DiamondMember diamondMember = new DiamondMember(chatRoom);
diamondMember.chat("TMD",4);
}
}
输出结果
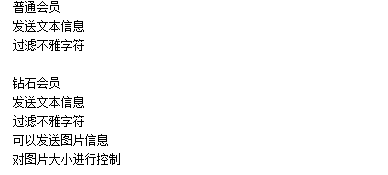
4、要求:设计一个网上书店,对系统中所有的计算机类图书(ComputerBook)每本都有10%的折扣,语言类图书(LanguageBook)每本都有2元的折扣,小说类图书(NovelBook)每100元有15元的折扣。使用Strategy模式来设计。
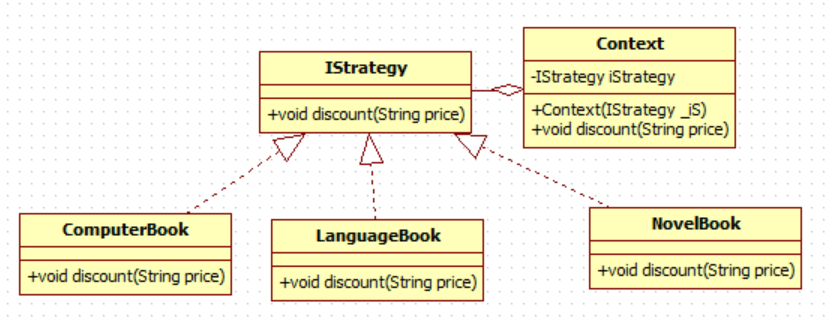
public interface IStrategy {
public void discount(String price);
}
public class ComputerBook implements IStrategy {
public void discount(String price){
System.out.println("计算机类图书每本有"+price+"的折扣");
}
}
public class LanguageBook implements IStrategy {
public void discount(String price){
System.out.println("语言类图书每本有"+price+"元的折扣");
}
}
public class NovelBook implements IStrategy {
public void discount(String price){
System.out.println("小说类图书每100元有"+price+"元的折扣");
}
}
public class Context {
private IStrategy iStrategy;
public Context(IStrategy _iS){
this.iStrategy = _iS;
}
public void discount(String price){
this.iStrategy.discount(price);
}
}
public class Client {
public static void main(String[] args){
Context context;
context = new Context(new ComputerBook());
context.discount("10%");
System.out.println("----------");
context = new Context(new LanguageBook());
context.discount("2");
System.out.println("----------");
context = new Context(new NovelBook());
context.discount("10");
}
}
输出结果
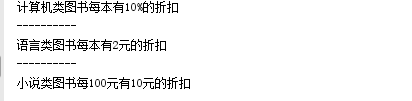