A. Stock Arbitraging
Welcome to Codeforces Stock Exchange! We're pretty limited now as we currently allow trading on one stock, Codeforces Ltd. We hope you'll still be able to make profit from the market!
In the morning, there are nn opportunities to buy shares. The ii-th of them allows to buy as many shares as you want, each at the price of sisibourles.
In the evening, there are mm opportunities to sell shares. The ii-th of them allows to sell as many shares as you want, each at the price of bibibourles. You can't sell more shares than you have.
It's morning now and you possess rr bourles and no shares.
What is the maximum number of bourles you can hold after the evening?
The first line of the input contains three integers n,m,rn,m,r (1≤n≤301≤n≤30, 1≤m≤301≤m≤30, 1≤r≤10001≤r≤1000) — the number of ways to buy the shares on the market, the number of ways to sell the shares on the market, and the number of bourles you hold now.
The next line contains nn integers s1,s2,…,sns1,s2,…,sn (1≤si≤10001≤si≤1000); sisi indicates the opportunity to buy shares at the price of sisi bourles.
The following line contains mm integers b1,b2,…,bmb1,b2,…,bm (1≤bi≤10001≤bi≤1000); bibi indicates the opportunity to sell shares at the price of bibibourles.
Output a single integer — the maximum number of bourles you can hold after the evening.
3 4 11 4 2 5 4 4 5 4
26
2 2 50 5 7 4 2
50
In the first example test, you have 1111 bourles in the morning. It's optimal to buy 55 shares of a stock at the price of 22 bourles in the morning, and then to sell all of them at the price of 55 bourles in the evening. It's easy to verify that you'll have 2626 bourles after the evening.
In the second example test, it's optimal not to take any action.
代码:

#include <bits/stdc++.h> using namespace std; const int maxn = 50; int N, M, R; int a[maxn], b[maxn]; int main() { scanf("%d%d%d", &N, &M, &R); for(int i = 1; i <= N; i ++) scanf("%d", &a[i]); for(int i = 1; i <= M; i ++) scanf("%d", &b[i]); sort(a + 1, a + 1 + N); sort(b + 1, b + 1 + M); int minn = a[1], maxx = b[M]; if(minn >= maxx) printf("%d ", R); else { int t = R / minn; t *= maxx; printf("%d ", R % minn + t); } return 0; }
B. Tiling Challenge
One day Alice was cleaning up her basement when she noticed something very curious: an infinite set of wooden pieces! Each piece was made of five square tiles, with four tiles adjacent to the fifth center tile:

Alice started wondering whether she could fill the board completely using the pieces she had found. Of course, each piece has to cover exactly five distinct cells of the board, no two pieces can overlap and every piece should fit in the board entirely, without some parts laying outside the board borders. The board however was too large for Alice to do the tiling by hand. Can you help determine if it's possible to fully tile the board?
The first line of the input contains a single integer nn (3≤n≤503≤n≤50) — the size of the board.
The following nn lines describe the board. The ii-th line (1≤i≤n1≤i≤n) contains a single string of length nn. Its jj-th character (1≤j≤n1≤j≤n) is equal to "." if the cell in the ii-th row and the jj-th column is free; it is equal to "#" if it's occupied.
You can assume that the board contains at least one free cell.
Output YES if the board can be tiled by Alice's pieces, or NO otherwise. You can print each letter in any case (upper or lower).
3 #.# ... #.#
YES
4 ##.# #... #### ##.#
NO
5 #.### ....# #.... ###.# #####
YES
5 #.### ....# #.... ....# #..##
NO
The following sketches show the example boards and their tilings if such tilings exist:
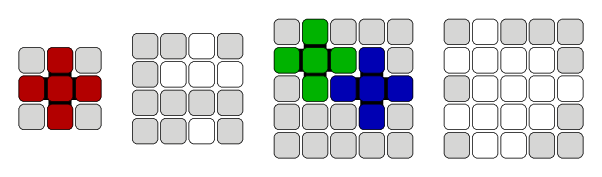
代码(改了半个小时没发现图输入错我会说??):

#include <bits/stdc++.h> using namespace std; const int maxn = 60; int N; char mp[maxn][maxn]; int vis[maxn][maxn]; int dx[5] = {1, 0, -1, 0}; int dy[5] = {0, 1, 0, -1}; bool f(int x, int y) { int cnt = 0; for(int i = 0; i < 4; i ++) { int xx = x + dx[i], yy = y + dy[i]; if(xx >= 0 && xx < N && yy >= 0 && yy < N) { if(mp[xx][yy] == '.' && vis[xx][yy] == 0) cnt ++; } } if(cnt == 4) return true; return false; } int main() { scanf("%d", &N); getchar(); for(int i = 0; i < N; i ++) { scanf("%s", mp[i]); } memset(vis, 0, sizeof(vis)); for(int i = 0; i < N; i ++) { for(int j = 0; j < N; j ++) { if(mp[i][j] == '.' && vis[i][j] == 0) { if(f(i, j)) { vis[i][j] = 1; for(int k = 0; k < 4; k ++) { int xx = i + dx[k], yy = j + dy[k]; vis[xx][yy] = 1; } } } } } bool flag = true; for(int i = 0; i < N; i ++) { if(!flag) break; for(int j = 0; j < N; j ++) { if(mp[i][j] == '.' && vis[i][j] == 0) flag = false; } } if(flag) printf("YES "); else printf("NO "); return 0; }
C. Prefix Sum Primes
We're giving away nice huge bags containing number tiles! A bag we want to present to you contains nn tiles. Each of them has a single number written on it — either 11 or 22.
However, there is one condition you must fulfill in order to receive the prize. You will need to put all the tiles from the bag in a sequence, in any order you wish. We will then compute the sums of all prefixes in the sequence, and then count how many of these sums are prime numbers. If you want to keep the prize, you will need to maximize the number of primes you get.
Can you win the prize? Hurry up, the bags are waiting!
The first line of the input contains a single integer nn (1≤n≤2000001≤n≤200000) — the number of number tiles in the bag. The following line contains nn space-separated integers a1,a2,…,ana1,a2,…,an (ai∈{1,2}ai∈{1,2}) — the values written on the tiles.
Output a permutation b1,b2,…,bnb1,b2,…,bn of the input sequence (a1,a2,…,an)(a1,a2,…,an) maximizing the number of the prefix sums being prime numbers. If there are multiple optimal permutations, output any.
5 1 2 1 2 1
1 1 1 2 2
9 1 1 2 1 1 1 2 1 1
1 1 1 2 1 1 1 2 1
The first solution produces the prefix sums 1,2,3,5,71,2,3,5,7 (four primes constructed), while the prefix sums in the second solution are 1,2,3,5,6,7,8,10,111,2,3,5,6,7,8,10,11 (five primes). Primes are marked bold and blue. In each of these cases, the number of produced primes is maximum possible.
代码:

#include <bits/stdc++.h> using namespace std; const int maxn = 2e5 + 10; int N; int a[maxn]; int one = 0, two = 0; int main() { scanf("%d", &N); for(int i = 0; i < N; i ++) { scanf("%d", &a[i]); if(a[i] == 1) one ++; else two ++; } vector<int> ans; if(one == 0) { for(int i = 0; i < N; i ++) ans.push_back(2); } else if(two == 0) { for(int i = 0; i < N; i ++) ans.push_back(1); } else { ans.push_back(2); ans.push_back(1); two --, one --; while(two --) ans.push_back(2); while(one --) ans.push_back(1); } for(int i = 0; i < ans.size(); i ++) printf("%d%s", ans[i], i != ans.size() - 1 ? " " : " "); return 0; }
D. Three Religions
代码:

#include <bits/stdc++.h> using namespace std; const int maxn = 1e6 + 10; int nx[maxn][30]; int dp[300][300][300]; int leen[5]; char d[5][300]; int N, Q, len; char s[maxn]; void init() { for(int i = 0; i < 26; i ++) nx[N][i] = nx[N + 1][i] = N + 1; for(int i = N - 1; i >= 0; i --) { for(int j = 0; j < 26; j ++) nx[i][j] = nx[i + 1][j]; nx[i][s[i + 1] - 'a'] = i + 1; } } int main() { scanf("%d%d", &N, &Q); scanf("%s", s + 1); init(); while(Q --) { string op, c; int x; cin >> op >> x; if(op == "-") leen[x] --; else { leen[x] ++; cin >> c; d[x][leen[x]] = c[0]; for(int i = x == 1 ? leen[x] : 0; i <= leen[1]; i ++) { for(int j = x == 2 ? leen[x] : 0; j <= leen[2]; j ++) { for(int k = x == 3 ? leen[x] : 0; k <= leen[3]; k ++) { dp[i][j][k] = N + 1; if(i) dp[i][j][k] = min(dp[i][j][k], nx[dp[i - 1][j][k]][d[1][i] - 'a']); if(j) dp[i][j][k] = min(dp[i][j][k], nx[dp[i][j - 1][k]][d[2][j] - 'a']); if(k) dp[i][j][k] = min(dp[i][j][k], nx[dp[i][j][k - 1]][d[3][k] - 'a']); } } } } if(dp[leen[1]][leen[2]][leen[3]] <= N) printf("YES "); else printf("NO "); } return 0; }
序列自动机 + dp
dp[i][j][k] 代表三个字符串长度分别是 i j k 的时候用到最短的 s 的长度 nx[i][j] 代表第 i 位后 j 字母第一次出现的位置
怎么这么难的 我要去撸 Leetcode 的 dp 了 希望会进步