一、准备工作
在SqlServer上创建数据库:Element
模拟两个表并插入数据:SysUser(用户表)、SysRole(角色表)
CREATE TABLE [dbo].[SysUser](
[ID] [int] IDENTITY(1,1) NOT NULL,
[Name] [nchar](10) NOT NULL,
[RoleNum] [nchar](10) NOT NULL
) ON [PRIMARY]
CREATE TABLE [dbo].[SysRole](
[ID] [int] IDENTITY(1,1) NOT NULL,
[RoleName] [nchar](10) NOT NULL,
[RoleNum] [nchar](10) NOT NULL
) ON [PRIMARY]
插入数据:
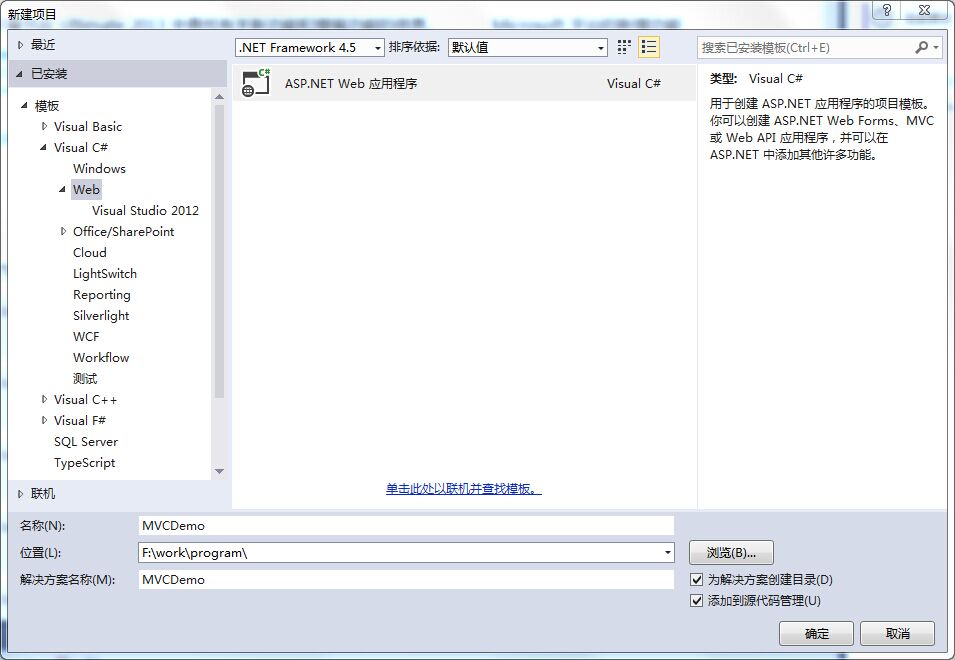


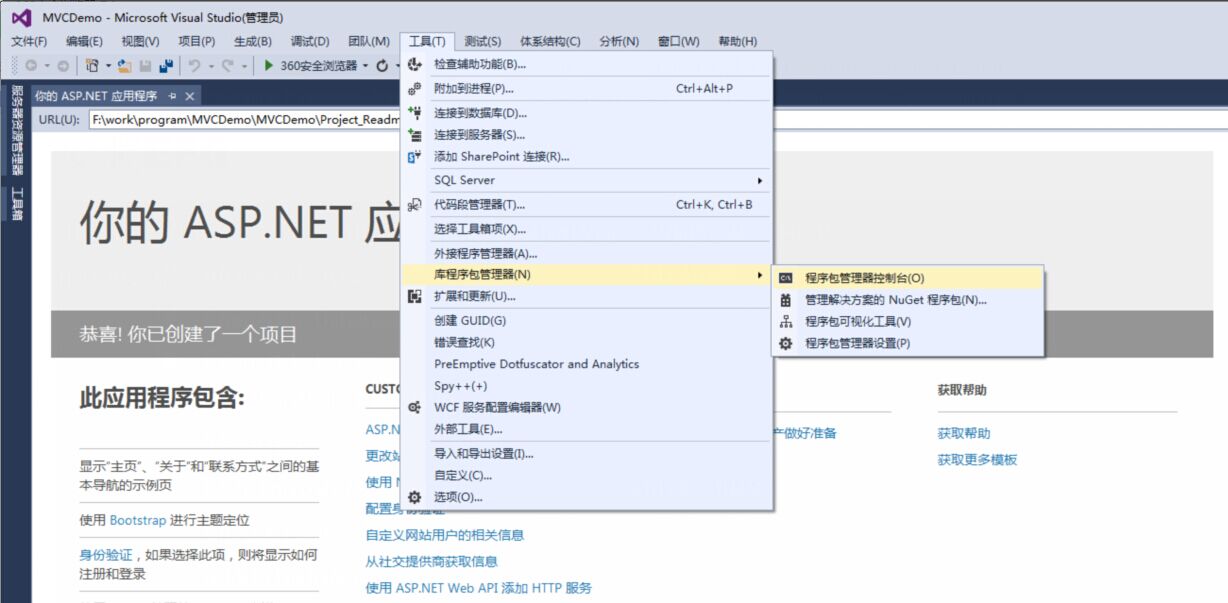

二、使用EF的Code First从原有数据库中生成Models

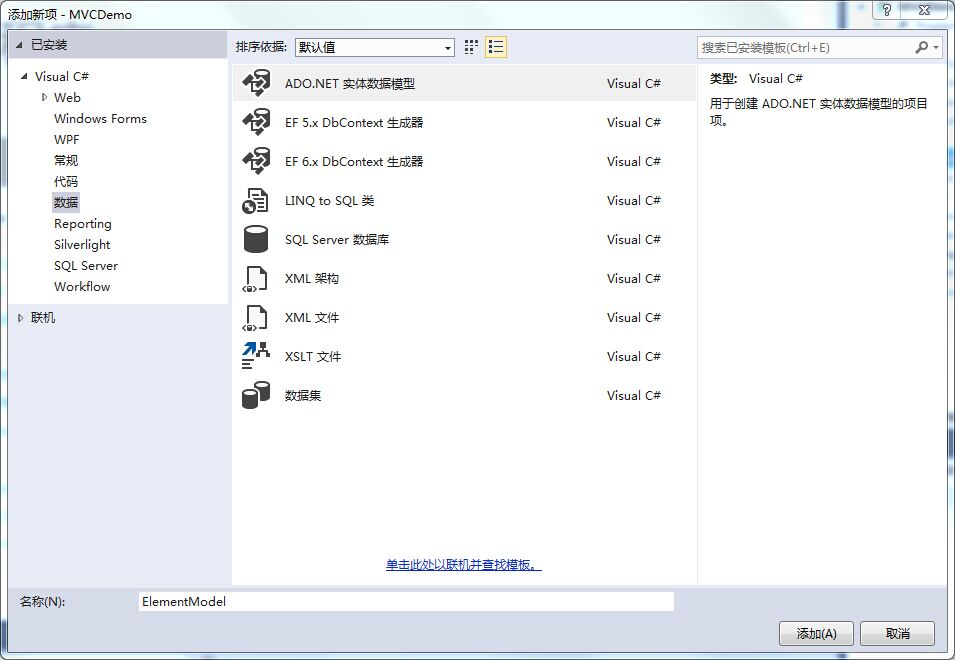
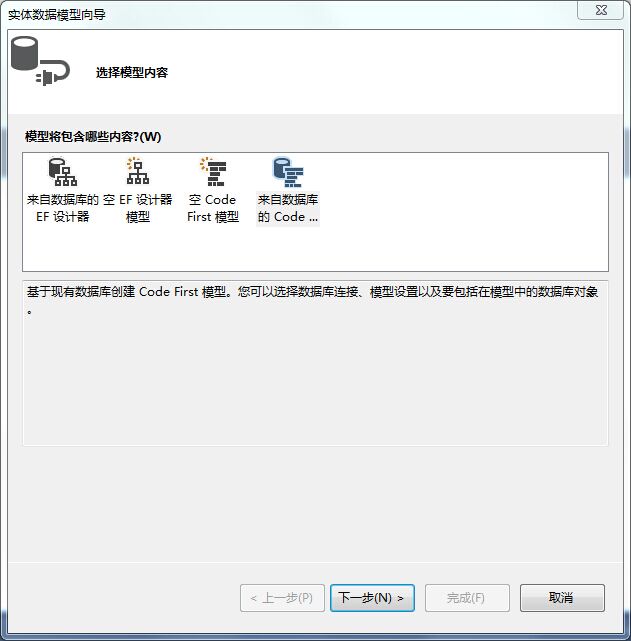
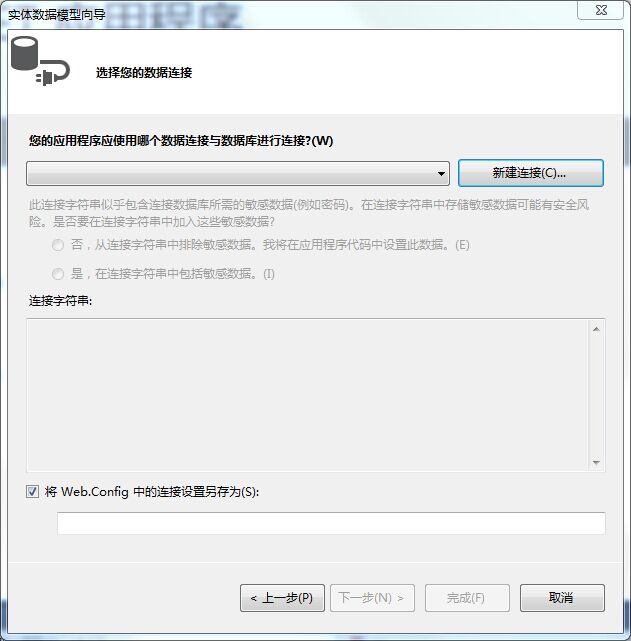
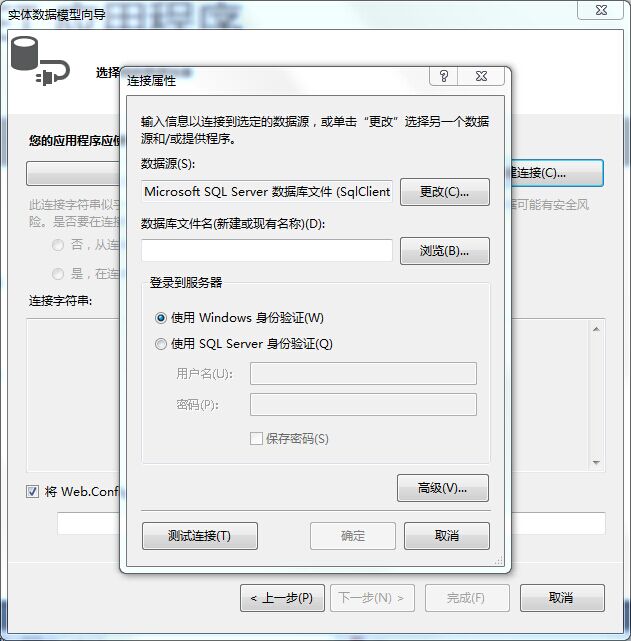

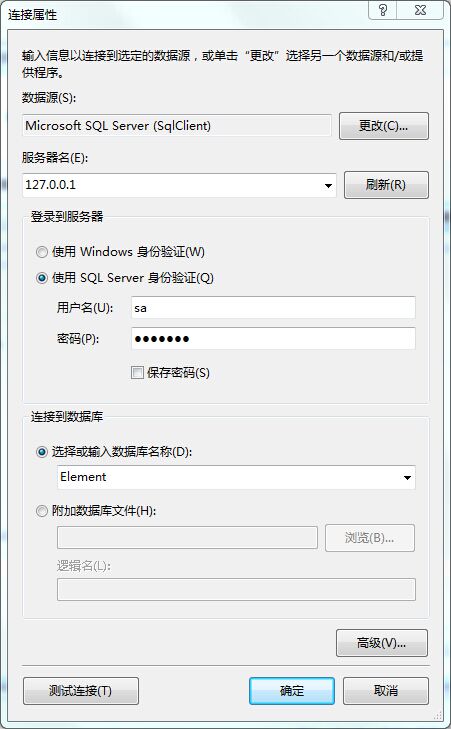
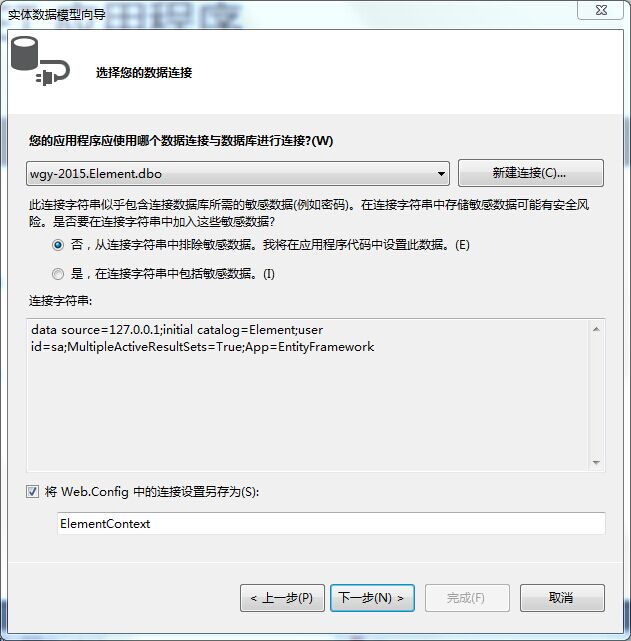

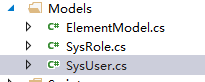
三、根据Model生成Controller及View
在Controllers文件夹上右键--添加--控制器
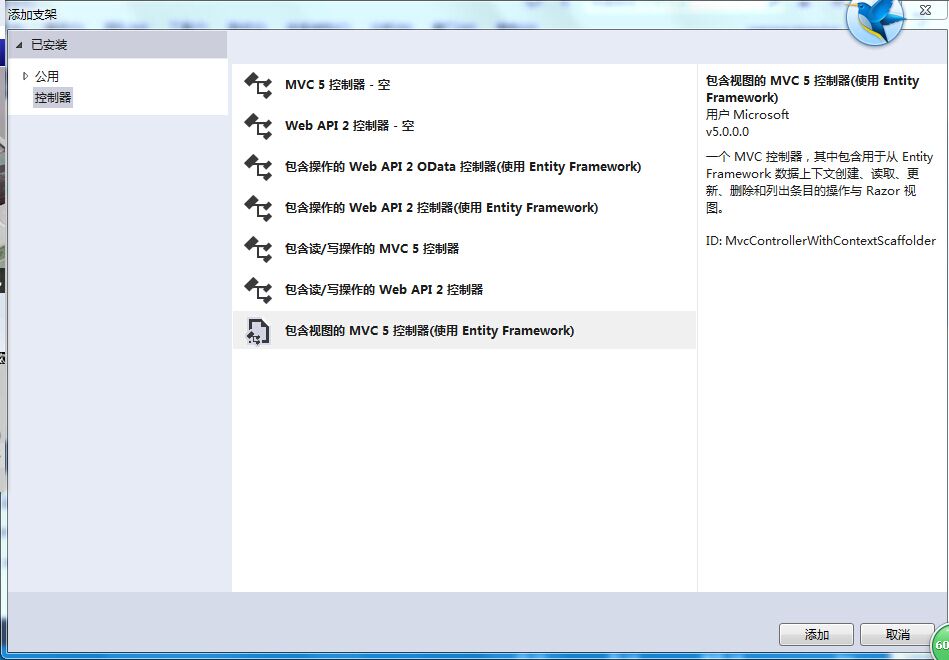
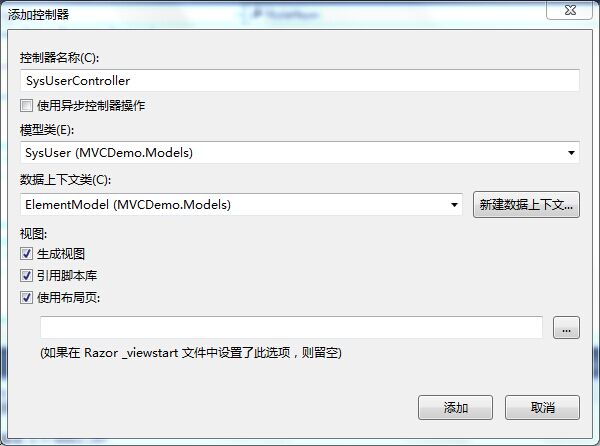
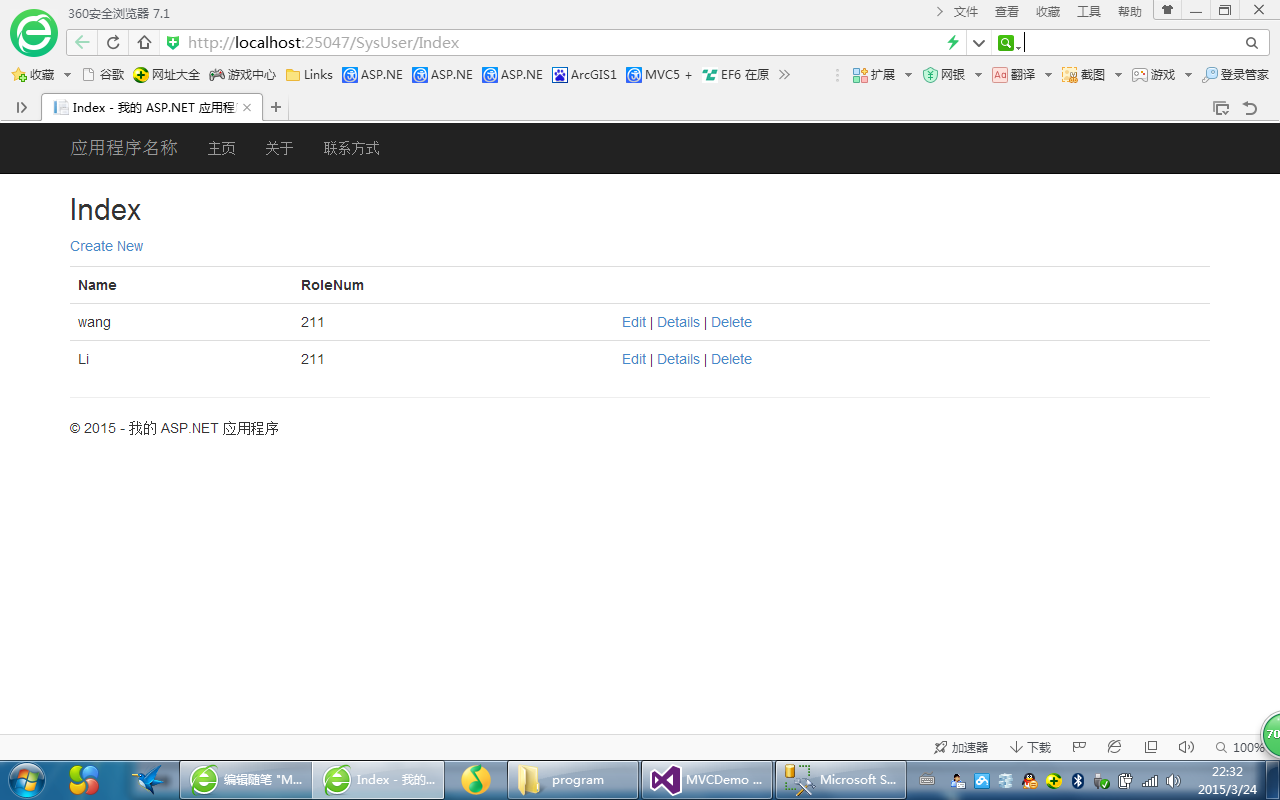
四、利用ViewModel显示多表联合查询

namespace MVCDemo.ViewModels
{
public class UserRole
{
public string userName { get; set; }
public string userRole { get; set; }
}
}
右键Controllers文件夹添加控制类,此类继承于Controller类
using System;
using System.Collections.Generic;
using System.Linq; using System.Web;
using System.Web.Mvc; using System.Data.Entity;
using MVCDemo.ViewModels;
using MVCDemo.Models;
namespace MVCDemo.Controllers
{
public class UserRoleController : Controller
{
ElementModel db = new ElementModel();
public ActionResult Index()
{
var userRoleList = from uu in db.SysUsers
join ud in db.SysRoles on uu.RoleNum equals ud.RoleNum
where uu.ID == 1
select new UserRole {userName = uu.Name,userRole = ud.RoleName}
return View(userRoleList);
}
}
}
@model IEnumerable<MVCDemo.ViewModels.UserRole>
@{
Layout = "~/Views/Shared/_Layout.cshtml";
}
<table class="table">
<tr>
<th>
@Html.DisplayNameFor(model=>model.userName)
</th>
<th>
@Html.DisplayNameFor(model => model.userRole)
</th>
<th></th>
</tr>
@foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.userName)
</td>
<td>
@Html.DisplayFor(modelItem => item.userRole)
</td>
</tr>
}
</table>