Barney lives in NYC. NYC has infinite number of intersections numbered with positive integers starting from 1. There exists a bidirectional road between intersections i and 2i and another road between i and 2i + 1 for every positive integer i. You can clearly see that there exists a unique shortest path between any two intersections.
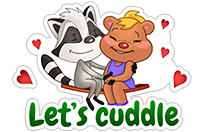
Initially anyone can pass any road for free. But since SlapsGiving is ahead of us, there will q consecutive events happen soon. There are two types of events:
1. Government makes a new rule. A rule can be denoted by integers v, u and w. As the result of this action, the passing fee of all roads on the shortest path from u to v increases by w dollars.
2. Barney starts moving from some intersection v and goes to intersection u where there's a girl he wants to cuddle (using his fake name Lorenzo Von Matterhorn). He always uses the shortest path (visiting minimum number of intersections or roads) between two intersections.
Government needs your calculations. For each time Barney goes to cuddle a girl, you need to tell the government how much money he should pay (sum of passing fee of all roads he passes).
The first line of input contains a single integer q (1 ≤ q ≤ 1 000).
The next q lines contain the information about the events in chronological order. Each event is described in form 1 v u w if it's an event when government makes a new rule about increasing the passing fee of all roads on the shortest path from u to v by w dollars, or in form 2 v u if it's an event when Barnie goes to cuddle from the intersection v to the intersection u.
1 ≤ v, u ≤ 1018, v ≠ u, 1 ≤ w ≤ 109 states for every description line.
For each event of second type print the sum of passing fee of all roads Barney passes in this event, in one line. Print the answers in chronological order of corresponding events.
7
1 3 4 30
1 4 1 2
1 3 6 8
2 4 3
1 6 1 40
2 3 7
2 2 4
94
0
32
In the example testcase:
Here are the intersections used:
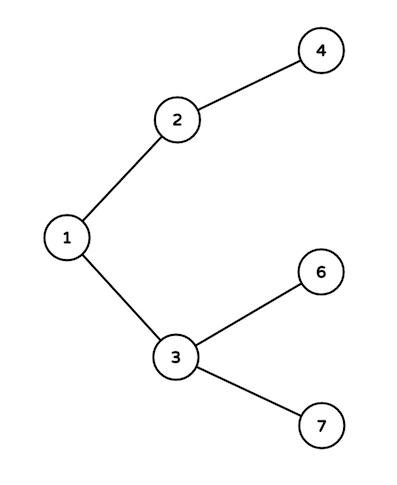
- Intersections on the path are 3, 1, 2 and 4.
- Intersections on the path are 4, 2 and 1.
- Intersections on the path are only 3 and 6.
- Intersections on the path are 4, 2, 1 and 3. Passing fee of roads on the path are 32, 32 and 30 in order. So answer equals to 32 + 32 + 30 = 94.
- Intersections on the path are 6, 3 and 1.
- Intersections on the path are 3 and 7. Passing fee of the road between them is 0.
7.Intersections on the path are 2 and 4. Passing fee of the road between them is 32 (increased by 30 in the first event and by 2 in the second).
题意:找树两点之间之间最短距离的花费;
思路:模拟,用map存每个节点当前的花费,表示这个节点到父亲节点的花费,查询或更新每个节点时,因为每个节点都是只有通过父亲节点才能到达的,所以只要向上找父亲节点记录,然后去掉两个点共同经过的节点,剩下的节点就是两个点之间的最短路。然更新或求费用。
复杂度log(n);
1 #include<stdio.h> 2 #include<algorithm> 3 #include<iostream> 4 #include<string.h> 5 #include<stdlib.h> 6 #include<queue> 7 #include<map> 8 #include<math.h> 9 using namespace std; 10 map<long long ,long long>my; 11 long long num1[100]; 12 long long num2[100]; 13 int x,y; 14 void findd(long long n) 15 { 16 while(n>=0) 17 { 18 num1[x++]=n; 19 if(n==0)break; 20 n=n-1; 21 n/=2; 22 23 } 24 } 25 void finddd(long long n) 26 { 27 28 while(n>=0) 29 { 30 num2[y++]=n; 31 if(n==0) 32 break; 33 n=n-1; 34 n/=2; 35 } 36 } 37 int main(void) 38 { 39 int i,j,k; 40 while(scanf("%d",&k)!=EOF) 41 { 42 x=0; 43 y=0; 44 my.clear(); 45 while(k--) 46 { 47 long long n,m,p,q; 48 scanf("%I64d",&p); 49 x=0,y=0; 50 if(p==1) 51 { 52 scanf("%I64d %I64d %I64d",&n,&m,&q); 53 n-=1; 54 m-=1; 55 findd(n); 56 finddd(m); 57 int t=x-1; 58 int tt=y-1; 59 while(num1[t]==num2[tt]&&t>=0&&tt>=0) 60 { 61 t--; 62 tt--; 63 } 64 for(j=0; j<=t; j++) 65 { 66 my[num1[j]]+=q; 67 68 } 69 for(j=0; j<=tt; j++) 70 { 71 my[num2[j]]+=q; 72 } 73 } 74 else if(p==2) 75 { 76 x=0; 77 y=0; 78 scanf("%I64d %I64d",&n,&m); 79 n-=1; 80 m-=1; 81 findd(n); 82 finddd(m); 83 long long ask=0; 84 int t=x-1; 85 int tt=y-1; 86 while(num1[t]==num2[tt]&&t>=0&&tt>=0) 87 { 88 t--; 89 tt--; 90 } 91 for(j=0; j<=t; j++) 92 { 93 ask+=my[num1[j]]; 94 95 } 96 for(j=0; j<=tt; j++) 97 { 98 99 ask+=my[num2[j]]; 100 } 101 printf("%I64d ",ask); 102 } 103 } 104 } 105 return 0; 106 }