图书管理器
数据库设计代码
CREATE TABLE bookmanage(
b_id BIGINT AUTO_INCREMENT PRIMARY KEY NOT NULL,
b_name VARCHAR(40) NOT NULL,
b_author VARCHAR(40) NOT NULL,<br>b_date DATETIME NOT NULL,
b_type INT NOT NULL )ENGINE=INNODB DEFAULT CHARSET=utf8;
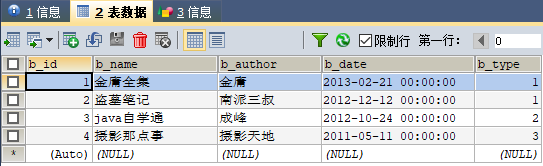
主界面:
<%@ page import="com.qkl.bean.BookManage"%>
<%@ page import="com.qkl.dao.BookManageDao"%>
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme() + "://"
+ request.getServerName() + ":" + request.getServerPort()
+ path + "/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>个人图书管理</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<h1>图书信息</h1>
<table border="1">
<tr>
<td>图书编号</td>
<td>图书名称</td>
<td>图书作者</td>
<td>购买时间</td>
<td>图书分类</td>
<td>操作</td>
</tr>
<%
BookManageDao book = new BookManageDao();
List<BookManage> list = book.selectBookAll();
for (int i = 0; i < list.size(); i++) {
%>
<tr>
<td><%=list.get(i).getB_id()%></td>
<td><%=list.get(i).getB_name()%></td>
<td><%=list.get(i).getB_author()%></td>
<td><%=list.get(i).getB_time()%></td>
<td><%int x = list.get(i).getB_type();
if (x == 1) {
%> <label>小说/文摘</label> <%
} else if (x == 2) {
%> <label>计算机/软件</label> <%
} else if (x == 3) {
%> <label>杂项</label> <%
}
%>
</td>
<td><a href="del.jsp?b_id=<%=list.get(i).getB_id()%>" onclick="del()">删除</a></td>
</tr>
<%
}
%>
</table>
<a href="newbook.jsp">新增图书信息</a>
</body>
<script type="text/javascript">
function del(){
if(confirm('确定要删除吗?')){
}else{
}
}
</script>
</html>
添加图书界面
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme() + "://"
+ request.getServerName() + ":" + request.getServerPort()
+ path + "/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>添加图书信息</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<form action="getnewbook.jsp" onsubmit="return validate_form(this)"
method="post">
<table>
<tr>
<th>新增图书信息</th>
</tr>
<tr>
<td>图书名称:</td>
<td><input type="text" name="newbookname">
</td>
</tr>
<tr>
<td>图书作者:</td>
<td><input type="text" name="newbookauthor">
</td>
</tr>
<tr>
<td>购买日期:</td>
<td><input type="text" name="newbookdate">
</td>
<td>yyyy-MM-dd格式</td>
</tr>
<tr>
<td>图书类别:</td>
<td><select id="select" name="select">
<option value="">选择所属分类</option>
<option value="1">小说/文摘</option>
<option value="2">计算机/软件</option>
<option value="3">杂项</option>
</select>
</tr>
</table>
<input type="submit" value="添加图书">
</form>
<script type="text/javascript">
function validate_required(field,alerttxt)
{
with (field)
{
if (value==null||value=="")
{alert(alerttxt);return false}
else {return true}
}
}
function validate_form(thisform)
{
var slt=document.getElementById("select");
if(slt.value==""){
alert("请选择分类");
return false;
}
with (thisform)
{
if (validate_required(newbookname,"书名不能为空")==false)
{newbookname.focus();return false}
if (validate_required(newbookauthor,"作者不能为空")==false)
{newbookauthor.focus();return false}
if (validate_required(newbookdate,"时间不能为空")==false)
{newbookdate.focus();return false}
}
}
</script>
</body>
</html>
添加图书内容收集界面
<%@ page import="com.qkl.bean.BookManage"%>
<%@ page import="com.qkl.dao.BookManageDao"%>
<%@ page import="java.util.*"%>
<%@ page import="java.text.*"%>
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme() + "://"
+ request.getServerName() + ":" + request.getServerPort()
+ path + "/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'getnewbook.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<%
request.setCharacterEncoding("utf-8");
BookManage m = new BookManage();
String b_name = request.getParameter("newbookname");
b_name.trim();
m.setB_name(b_name);
String b_author = request.getParameter("newbookauthor");
b_author.trim();
m.setB_author(b_author);
String b_date = request.getParameter("newbookdate");
SimpleDateFormat smt = new SimpleDateFormat("yyyy-MM-dd");
Date d_datein = smt.parse(b_date);
m.setB_time(d_datein);
String b_type = request.getParameter("select");
int bb_type= Integer.parseInt(b_type);
m.setB_type(bb_type);
BookManageDao sd = new BookManageDao();
if (sd.addbook(m) > 0) {
%><p>添加成功</p><a href="index.jsp">点击返回主页面</a>
<%
} else {
%>
<p>输入错误,请重新注册</p>
<%
}
%>
</body>
</html>
删除图书内容收集界面
<%@ page import="com.qkl.bean.BookManage"%>
<%@ page import="com.qkl.dao.BookManageDao"%>
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'del.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<%
request.setCharacterEncoding("utf-8");
int b_id=Integer.parseInt(request.getParameter("b_id"));
BookManageDao id=new BookManageDao();
id.delbook(b_id);
request.getRequestDispatcher("index.jsp").forward(request, response);
%>
</body>
</html>
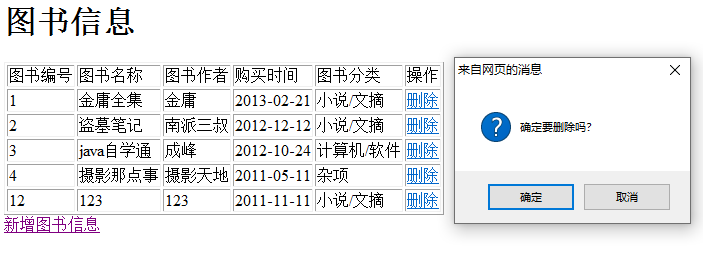
BookManage.java
package com.qkl.bean;
import java.util.Date;
public class BookManage {
private int b_id;
private String b_name;
private String b_author;
private Date b_time;
private int b_type;
public int getB_id() {
return b_id;
}
public void setB_id(int b_id) {
this.b_id = b_id;
}
public String getB_name() {
return b_name;
}
public void setB_name(String b_name) {
this.b_name = b_name;
}
public String getB_author() {
return b_author;
}
public void setB_author(String b_author) {
this.b_author = b_author;
}
public Date getB_time() {
return b_time;
}
public void setB_time(Date b_time) {
this.b_time = b_time;
}
public int getB_type() {
return b_type;
}
public void setB_type(int b_type) {
this.b_type = b_type;
}
public BookManage(int b_id, String b_name, String b_author, Date b_time,int b_type) {
super();
this.b_id = b_id;
this.b_name = b_name;
this.b_author = b_author;
this.b_time = b_time;
this.b_type = b_type;
}
public BookManage() {
super();
// TODO Auto-generated constructor stub
}
}
BookManageDao.java
package com.qkl.dao;
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
import com.jjy.bean.BookManage;
public class BookManageDao extends BaseDao {
//显示数据列表
public List<BookManage> selectBookAll() {
List<BookManage> list = new ArrayList<BookManage>();
Connection conn=getConnection();
String sql = "SELECT b_id,b_name,b_author,b_date,b_type FROM bookmanage";
PreparedStatement ps = null;
ResultSet rs=null;
try {
ps = conn.prepareStatement(sql);
rs=ps.executeQuery();
while(rs.next()) {
BookManage m = new BookManage();
m.setB_id(rs.getInt(1));
m.setB_name(rs.getString(2));
m.setB_author(rs.getString(3));
m.setB_time(rs.getDate(4));
m.setB_type(rs.getInt(5));
list.add(m);
}
} catch (SQLException e) {
e.printStackTrace();
}finally {
closeAll(conn,ps,rs);
}
return list;
}
//增加数据
public int addbook(BookManage m){
int i=0;
Connection conn=getConnection();
String sql="insert into bookmanage(b_name,b_author,b_date,b_type) values(?,?,?,?)";
PreparedStatement ps=null;
try {
ps = conn.prepareStatement(sql);
ps.setString(1, m.getB_name());
ps.setString(2, m.getB_author());
ps.setDate(3,new Date(m.getB_time().getTime()));
ps.setInt(4, m.getB_type());
i=ps.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
closeAll(conn, ps, null);
}
return i;
}
//删除数据
public void delbook(int id){
Connection con=getConnection();
PreparedStatement ps=null;
String sql="delete from bookmanage where b_id=?";
try {
ps=con.prepareStatement(sql);
ps.setInt(1, id);
ps.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
closeAll(con, ps, null);
}
}
}
BaseDao.java
package com.qkl.dao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class BaseDao {
//获取连接
protected Connection getConnection(){
Connection conn=null;
try {
Class.forName("com.mysql.jdbc.Driver");
// 2.建立连接
conn = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/jsp", "root", "root");
} catch (Exception e) {
e.printStackTrace();
}
return conn;
}
//关闭连接
protected void closeAll(Connection conn,PreparedStatement ps,ResultSet rs){
try {
if(rs != null)
rs.close();
if(ps != null)
ps.close();
if(conn != null)
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}