#define _CRT_SECURE_NO_DEPRECATE
#include<stdio.h>
#include<stdlib.h>
#include<windows.h>
typedef struct Node
{
int num;
Node *next;
}Node,*LinkNode;
bool LinkSameNum(LinkNode head, int x)//判断是否有相同的数
{
LinkNode p = head->next;
while (p)
{
if (p->num == x)
return true;
p = p->next;
}
return false;
}
void Creat(LinkNode &head, int n)//头插法建立链表
{
head = (LinkNode)malloc(sizeof(Node));
head->next = NULL;
LinkNode s;
int x;
for (int i = 0; i < n; i++)
{
x = rand() % 90 + 10;
if (LinkSameNum(head, x))//如果有相同的重新来过
{
i--;
continue;
}
s = (LinkNode)malloc(sizeof(Node));
s->num = x;
s->next = head->next;
head->next = s;
}
}
LinkNode JiaoJi(LinkNode head1, LinkNode head2)
{
LinkNode head=(LinkNode)malloc(sizeof(Node));
LinkNode tail=head;
tail->next = NULL;
LinkNode s,p1=head1->next, p2 = head2->next;
while (p1)
{
while (p2)
{
if (p2->num == p1->num)
{
s = (LinkNode)malloc(sizeof(Node));
s->num = p1->num;
s->next = NULL;
tail->next = s;
tail = s;
break;
}
p2 = p2->next;
}
p1 = p1->next;
p2 = head2->next;
}
return head;
}
LinkNode BingJi(LinkNode head1, LinkNode head2)
{
LinkNode head = (LinkNode)malloc(sizeof(Node));
head->next = NULL;
LinkNode tail = head;
LinkNode LinkJiaoji = JiaoJi(head1, head2);
LinkNode s, p1 = head1->next, p2 = head2->next,p3=LinkJiaoji->next;
while (p1)
{
s = (LinkNode)malloc(sizeof(Node));
s->num = p1->num;
s->next = tail->next;
tail->next = s;
tail = s;
p1 = p1->next;
}
while(p2)
{
while (p3)
{
if (p2->num == p3->num)
break;
p3 = p3->next;
}
if(!p3)
{
s = (LinkNode)malloc(sizeof(Node));
s->num = p2->num;
s->next = tail->next;
tail->next = s;
tail = s;
}
p3 = LinkJiaoji->next;
p2 = p2->next;
}
return head;
}
LinkNode ChaJi(LinkNode head1, LinkNode head2)
{
LinkNode head = (LinkNode)malloc(sizeof(Node));
head->next = NULL;
LinkNode tail = head;
LinkNode LinkJiaoji = JiaoJi(head1, head2);
LinkNode s, p1 = head1->next, p3 = LinkJiaoji->next;
while (p1)
{
while (p3)
{
if (p1->num == p3->num)
break;
p3 = p3->next;
}
if (!p3)
{
s = (LinkNode)malloc(sizeof(Node));
s->num = p1->num;
s->next = tail->next;
tail->next = s;
tail = s;
}
p3 = LinkJiaoji->next;
p1 = p1->next;
}
return head;
}
void Print(LinkNode head)
{
LinkNode p = head->next;
int count = 0;
if (p == NULL)
{
printf("空集!
");
return;
}
while (p)
{
printf("%3d", p->num);
count++;
p = p->next;
}
printf("
(共%d个数据)
",count);
}
int main()
{
LinkNode head1, head2,ListJiao,ListBing,ListCha1, ListCha2;
int m, n;
printf("分别输入两个链表的数据长度:");
scanf("%d %d", &m, &n);
Creat(head1, m);
Creat(head2, n);
printf("初始两个链表的数据
");
Print(head1);
Print(head2);
printf("
");
/** 交集**/
ListJiao = JiaoJi(head1, head2);
printf("交集链表的数据
");
Print(ListJiao);
printf("
");
/** 并集**/
ListBing = BingJi(head1, head2);
printf("并集链表的数据
");
Print(ListBing);
printf("
");
/** 差集**/
ListCha1 = ChaJi(head1, head2);
printf("差集head1-head2链表的数据
");
Print(ListCha1);
printf("
");
ListCha2 = ChaJi(head2, head1);
printf("差集head2-head1链表的数据
");
Print(ListCha2);
printf("
");
system("pause");
return 0;
}
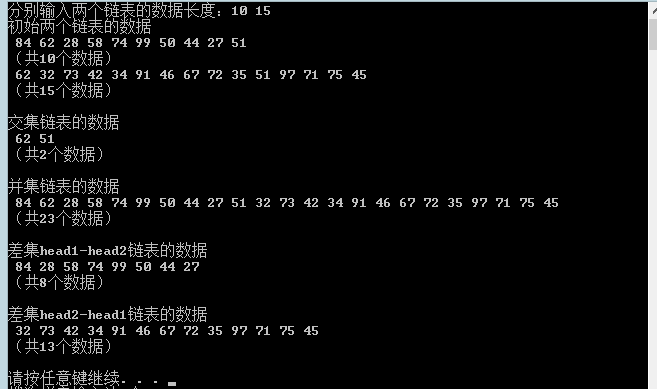