Given a list of points that form a polygon when joined sequentially, find if this polygon is convex (Convex polygon definition). Note: There are at least 3 and at most 10,000 points. Coordinates are in the range -10,000 to 10,000. You may assume the polygon formed by given points is always a simple polygon (Simple polygon definition). In other words, we ensure that exactly two edges intersect at each vertex, and that edges otherwise don't intersect each other. Example 1: [[0,0],[0,1],[1,1],[1,0]] Answer: True
Explanation:
Example 2: [[0,0],[0,10],[10,10],[10,0],[5,5]]
Answer: False
Explanation:
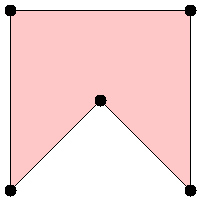
https://discuss.leetcode.com/topic/70706/beyond-my-knowledge-java-solution-with-in-line-explanation
The key observation for convexity is that vector pi+1-pi always turns to the same direction to pi+2-pi formed by any 3 sequentially adjacent vertices, i.e., cross product (pi+1-pi) x (pi+2-pi) does not change sign when traversing sequentially along polygon vertices.
Note that for any 2D vectors v1, v2,
- v1 x v2 = det([v1, v2])
which is the determinant of 2x2 matrix [v1, v2]. And the sign of det([v1, v2]) represents the positive z-direction of right-hand system from v1 to v2. So det([v1, v2]) ≥ 0 if and only if v1 turns at most 180 degrees counterclockwise to v2.
1 public class Solution { 2 public boolean isConvex(List<List<Integer>> points) { 3 // For each set of three adjacent points A, B, C, find the cross product AB · BC. If the sign of 4 // all the cross products is the same, the angles are all positive or negative (depending on the 5 // order in which we visit them) so the polygon is convex. 6 boolean gotNegative = false; 7 boolean gotPositive = false; 8 int numPoints = points.size(); 9 int B, C; 10 for (int A = 0; A < numPoints; A++) { 11 // Trick to calc the last 3 points: n - 1, 0 and 1. 12 B = (A + 1) % numPoints; 13 C = (B + 1) % numPoints; 14 15 int crossProduct = 16 crossProductLength( 17 points.get(A).get(0), points.get(A).get(1), 18 points.get(B).get(0), points.get(B).get(1), 19 points.get(C).get(0), points.get(C).get(1)); 20 if (crossProduct < 0) { 21 gotNegative = true; 22 } 23 else if (crossProduct > 0) { 24 gotPositive = true; 25 } 26 if (gotNegative && gotPositive) return false; 27 } 28 29 // If we got this far, the polygon is convex. 30 return true; 31 } 32 33 // Return the cross product AB x BC. 34 // The cross product is a vector perpendicular to AB and BC having length |AB| * |BC| * Sin(theta) and 35 // with direction given by the right-hand rule. For two vectors in the X-Y plane, the result is a 36 // vector with X and Y components 0 so the Z component gives the vector's length and direction. 37 private int crossProductLength(int Ax, int Ay, int Bx, int By, int Cx, int Cy) 38 { 39 // Get the vectors' coordinates. 40 int ABx = Bx - Ax; 41 int ABy = By - Ay; 42 int BCx = Cx - Bx; 43 int BCy = Cy - By; 44 45 // Calculate the Z coordinate of the cross product. 46 return (ABx * BCy - ABy * BCx); 47 } 48 }