Median Filter
Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 1092 Accepted Submission(s): 269
Problem Description
Median filter is a cornerstone of modern image processing and is used extensively in
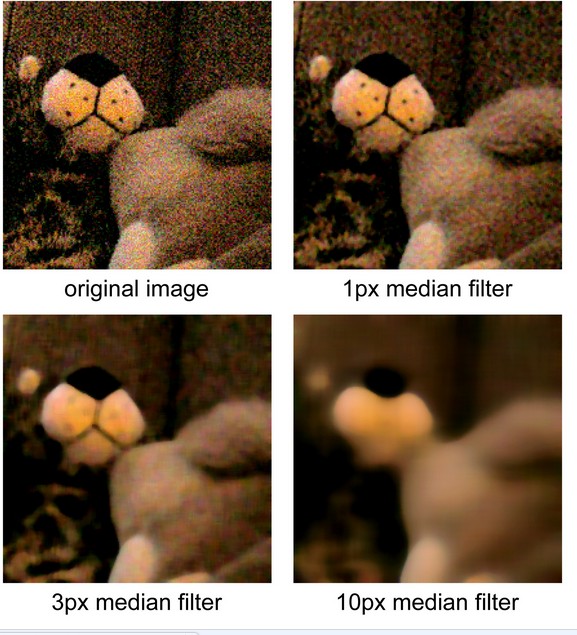
Given a black and white image of n by n pixels, the algorithm works as follow:
For each pixel p at the i‐th row and the j‐th column (1+ r <= i, j <= n – r), its gray level g[i,j] is replace by the median of gray levels of pixels in the (2r+1) by (2r+1) square centered at p. The square is called the filtering window and r is its radius.
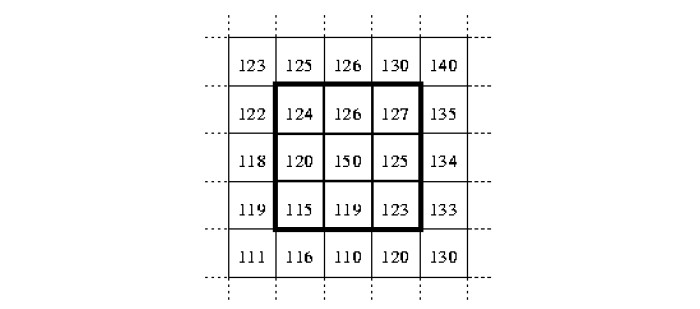
Considering the above example, the gray level of the pixel at center will be changed from 150 to 124, which is the median of a filtering window of radius 1.
Note that the algorithm won’t be applied on the pixels near the boundary, for the filtering window lies outside the image. So you are actually asked to output the filtered sub-image which contains the pixels from (r+1, r+1) to (n-r, n-r).
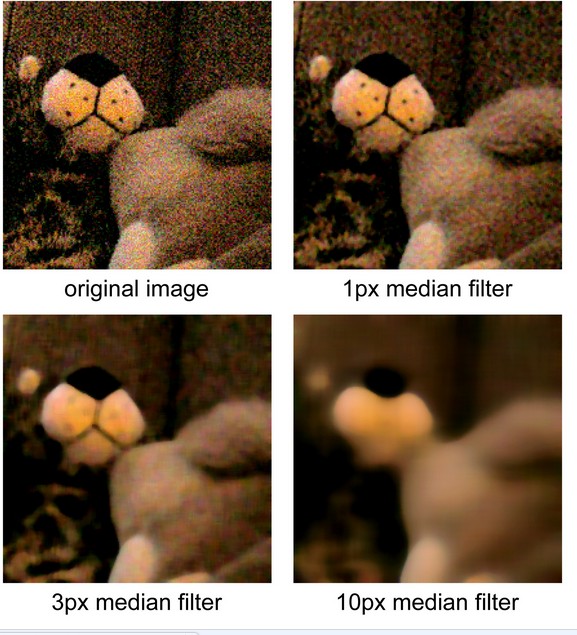
Given a black and white image of n by n pixels, the algorithm works as follow:
For each pixel p at the i‐th row and the j‐th column (1+ r <= i, j <= n – r), its gray level g[i,j] is replace by the median of gray levels of pixels in the (2r+1) by (2r+1) square centered at p. The square is called the filtering window and r is its radius.
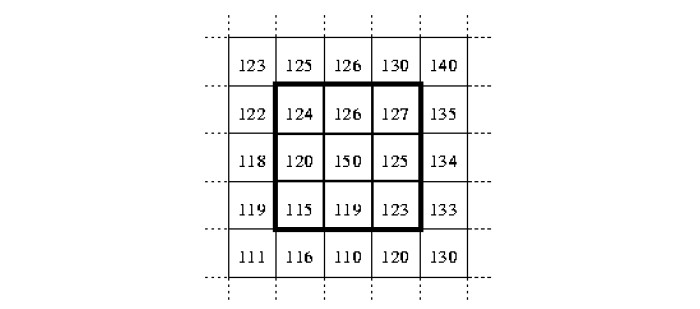
Considering the above example, the gray level of the pixel at center will be changed from 150 to 124, which is the median of a filtering window of radius 1.
Note that the algorithm won’t be applied on the pixels near the boundary, for the filtering window lies outside the image. So you are actually asked to output the filtered sub-image which contains the pixels from (r+1, r+1) to (n-r, n-r).
Input
The input contains several test cases.
For each test case:
(a) The first line contains two integers, n and r, meaning that the size of image is n * n (3 <= n <= 500) and the radius of filtering window is r ( 3 <= 2r + 1 <= n).
(b) The following n lines contains the n by n gray level matrix presenting the image
(c) The gray level ranges from 0 to 1000000 The input ends by double 0s.
For each test case:
(a) The first line contains two integers, n and r, meaning that the size of image is n * n (3 <= n <= 500) and the radius of filtering window is r ( 3 <= 2r + 1 <= n).
(b) The following n lines contains the n by n gray level matrix presenting the image
(c) The gray level ranges from 0 to 1000000 The input ends by double 0s.
Output
For each test case output a (n – 2r) by (n – 2r) matrix presenting the sub-image after filtered.
Sample Input
3 1
1 1 1
1 1 1
1 1 1
3 1
1 9 6
4 5 2
3 7 8
3 1
0 0 0
255 255 255
0 255 0
5 1
0 0 1 1 0
1 0 1 0 1
0 0 1 1 1
1 1 1 0 1
1 0 0 0 1
0 0
Sample Output
1
5
0
0 1 1
1 1 1
1 0 1
Hint
The definition of “median”: One type of average, found by arranging the values in order and then selecting the one in the middle.
If the total number of values in the sample is even, then the median is the mean of the two middle numbers.
The median is a useful number in cases where the distribution has very large extreme values which would otherwise skew the data.
Source
Recommend
题意:
中值滤波,求在n*n的矩阵中,内(n-2*r)*(n-2*r)的中值滤波后的子矩阵。
中值滤波的方式就是对于每一个可以取到的以(i,j)为矩阵中心的(2*r+1)*(2*r+1)的子矩阵,将子矩阵中所有数排序后的中位数作为新值,新值不影响后面的值。
做法:
树状数组
这是一题比较有趣的题吧,一开始暴力试一下是TLE的,明显算法复杂都会很大。子矩阵的处理如果用到排序应该都会超时,因此看到了一个很高效的算法,就是利用树状数组和一个在树状树组中求第k大的数的函数,后来在采用S行遍历,代码因此有点长,还要注意一下输出。
1 //1781MS 6196K 2399 B G++ 2 #include<stdio.h> 3 #include<string.h> 4 #define N 505 5 int g[N][N]; 6 int ans[N][N]; 7 int c[1<<20]; 8 int n,r,M; 9 inline int lowbit(int k) 10 { 11 return k&(-k); 12 } 13 int getsum(int k) 14 { 15 int s=0; 16 for(;k>0;k-=lowbit(k)) 17 s+=c[k]; 18 return s; 19 } 20 void insert(int k,int detal) 21 { 22 for(;k<=M;k+=lowbit(k)) 23 c[k]+=detal; 24 } 25 int find_kth(int k) //树状数组找第k大的数 26 { 27 int sum=0,pos=0; 28 for(int i=20;i>=0;i--){ 29 pos+=(1<<i); 30 if(pos>M || sum+c[pos]>=k) 31 pos-=(1<<i); 32 else sum+=c[pos]; 33 } 34 return pos+1; 35 } 36 void solve() 37 { 38 int R=2*r+1; 39 int k=R*R/2+1; 40 for(int i=1;i<=R;i++) 41 for(int j=1;j<=R;j++) 42 insert(g[i][j],1); 43 //for(int i=1;i<=2*(k-1);i++) printf("*%d ",c[i]); 44 for(int i=r+1;i<=n-r;i++){ 45 if((i-r)&1){ //奇数行,向右遍历 46 if(i!=r+1){ 47 for(int j=1;j<=R;j++){ 48 insert(g[i-r-1][j],-1); 49 insert(g[i+r][j],1); 50 } 51 } 52 ans[i][r+1]=find_kth(k); 53 for(int j=r+2;j<=n-r;j++){ 54 for(int ii=i-r;ii<=i+r;ii++){ 55 insert(g[ii][j-r-1],-1); 56 insert(g[ii][j+r],1); 57 } 58 ans[i][j]=find_kth(k); 59 } 60 }else{ //偶数行,向左遍历 61 for(int j=n;j>=n-R+1;j--){ 62 insert(g[i-r-1][j],-1); 63 insert(g[i+r][j],1); 64 } 65 ans[i][n-r]=find_kth(k); 66 for(int j=n-r-1;j>=r+1;j--){ 67 for(int ii=i-r;ii<=i+r;ii++){ 68 insert(g[ii][j+r+1],-1); 69 insert(g[ii][j-r],1); 70 } 71 ans[i][j]=find_kth(k); 72 } 73 } 74 } 75 } 76 int main(void) 77 { 78 while(scanf("%d%d",&n,&r),n+r) 79 { 80 M=0; 81 memset(c,0,sizeof(c)); 82 for(int i=1;i<=n;i++) 83 for(int j=1;j<=n;j++){ 84 scanf("%d",&g[i][j]); 85 g[i][j]++; 86 if(g[i][j]>M) M=g[i][j]; 87 } 88 solve(); 89 for(int i=r+1;i<=n-r;i++) 90 for(int j=r+1;j<=n-r;j++) 91 printf(j==n-r?"%d ":"%d ",ans[i][j]-1); 92 } 93 return 0; 94 } 95 96 /* 97 98 3 1 99 1 9 6 100 4 5 2 101 3 7 8 102 103 5 1 104 0 0 1 1 0 105 1 0 1 0 1 106 0 0 1 1 1 107 1 1 1 0 1 108 1 0 0 0 1 109 110 */