前言:
苹果的网络请求有NSURLConnection和NSURLSession,而NSURLConnection在iOS9被宣布弃用。AFNetworking对NSURLSession进行了封装,另外,一般公司里用的网络库,也是在NSURLSession基础上进行封装,使得符合自己业务的开发需求,所以,我们很有必要对NSURLSession的使用进行了解。本文对NSURLSession进行简单的介绍。
正文:
一、NSURLSession简介
使用NSURLSession,共分两步:
- 第一步 通过NSURLSession的实例创建task
- 第二步 执行task
NSURLSessionTask的继承关系如下图所示:
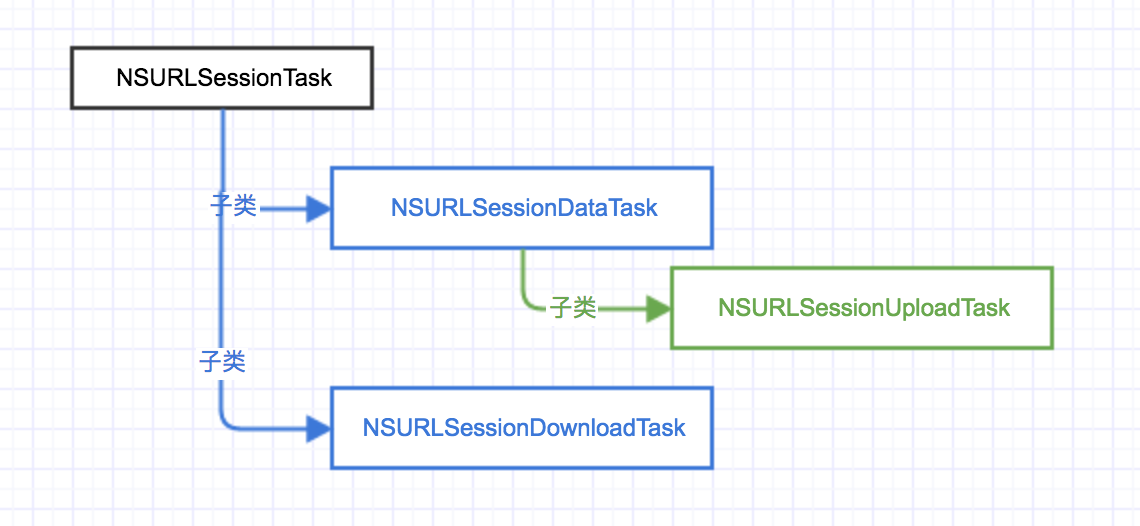
二、NSURLSessionTask的使用举例(分6种情况)
(1) NSURLSessionTask进行简单的Get请求
NSURLSession *session = [NSURLSession sharedSession]; NSURL *url = [NSURL URLWithString:@"https://xxx.yy.zz/check"]; NSURLSessionTask *task = [session dataTaskWithURL:url completionHandler:^(NSData *data, NSURLResponse *response, NSError *error){ if(!error){ NSDictionary *responseDic = [NSJSONSerialization JSONObjectWithData:data options:kNilOptions error:nil]; NSString *status = responseDic[@"status"]; if ([status isKindOfClass:[NSString class]] && [status isEqualToString:@"1"]) { self.needAutoCode = YES ; [self.tableView reloadData]; } }else { NSLog(@"%@", error); } }]; [task resume];
(2) NSURLSessionTask进行简单的Post请求
NSURL *url = [NSURL URLWithString:@"https://xxx.xxx.xx/accessToken"]; NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url]; request.HTTPMethod = @"POST"; NSString *param = [NSString stringWithFormat:@"code=%@&client_secret=3QmKoBbku7&client_id=apollo&grant_type=code",self.serviceTicket]; request.HTTPBody = [param dataUsingEncoding:NSUTF8StringEncoding]; NSURLSession *session = [NSURLSession sharedSession]; NSURLSessionTask *task = [session dataTaskWithRequest:request completionHandler:^(NSData *data, NSURLResponse *response, NSError *error){ if((!error) && ([(NSHTTPURLResponse *)response statusCode] == 200)) { NSError *error0 = nil; NSDictionary *jsonDic = [NSJSONSerialization JSONObjectWithData:data options:NSJSONReadingAllowFragments error:&error0]; if(!error0){ NSString *refreshToken = jsonDic[@"refresh_token"]; NSString *accessToken = jsonDic[@"access_token"]; //此处一些业务处理 }else{ NSLog(@"%@",error0.nv_message); } }else{ NSLog(@"%@",error.nv_message); } }]; [task resume];
(3)NSURLSessionDownloadTask进行简单的文件下载
NSURLSession *session = [NSURLSession sharedSession]; NSURL *url = [NSURL URLWithString:@"http://www.daka.com/resources/image/icon.png"] ; NSURLSessionDownloadTask *task = [session downloadTaskWithURL:url completionHandler:^(NSURL *location, NSURLResponse *response, NSError *error) { // location是沙盒中tmp文件夹下的一个临时url,文件下载后会存到这个位置,由于tmp中的文件随时可能被删除,所以我们需要自己需要把下载的文件挪到需要的地方 NSString *path = [[NSSearchPathForDirectoriesInDomains(NSCachesDirectory, NSUserDomainMask, YES) lastObject] stringByAppendingPathComponent:response.suggestedFilename]; // 剪切文件 [[NSFileManager defaultManager] moveItemAtURL:location toURL:[NSURL fileURLWithPath:path] error:nil]; }]; // 启动任务 [task resume];
(4)断点下载
// 使用这种方式取消下载可以得到将来用来恢复的数据,保存起来 [self.task cancelByProducingResumeData:^(NSData *resumeData) { self.resumeData = resumeData; }]; // 由于下载失败导致的下载中断会进入此协议方法,也可以得到用来恢复的数据 - (void)URLSession:(NSURLSession *)session task:(NSURLSessionTask *)task didCompleteWithError:(NSError *)error{ // 保存恢复数据 self.resumeData = error.userInfo[NSURLSessionDownloadTaskResumeData]; } // 恢复下载时接过保存的恢复数据 self.task = [self.session downloadTaskWithResumeData:self.resumeData]; // 启动任务 [self.task resume];
(5)文件上传
NSURLSessionUploadTask *uploadtask1 = [self.session uploadTaskWithRequest:request fromFile:fileName completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {}]; //或者,出于安全性考虑,通常使用POST方式进行文件上传 NSURLSessionUploadTask *uploadtask2 = [self.session uploadTaskWithRequest:request fromData:body completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) { NSLog(@"-------%@", [NSJSONSerialization JSONObjectWithData:data options:kNilOptions error:nil]);}];
(6) 使用代理NSURLSessionDataDelegate
NSURLSession提供了block方式处理返回数据的简便方式,但如果想要在接收数据过程中做进一步的处理,仍然可以调用相关的协议方法.NSURLSession的代理方法分为接收响应、接收数据、请求完成几个阶段。
NSURLSessionDelegate的继承关系如下图所示:
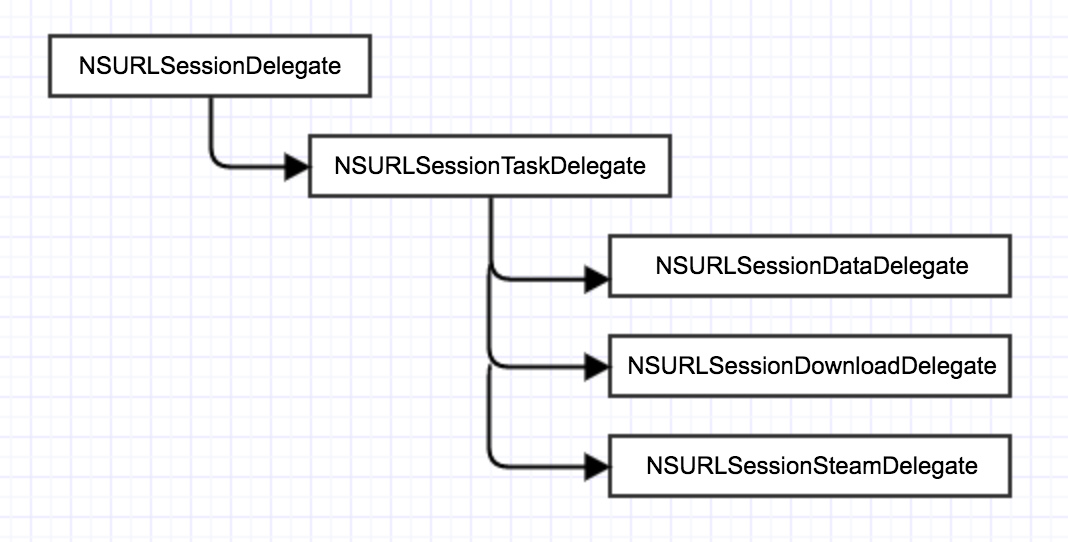
//使用代理方法需要设置代理,但是session的delegate属性是只读的,要想设置代理只能通过这种方式创建session self.session = [NSURLSession sessionWithConfiguration:[NSURLSessionConfiguration defaultSessionConfiguration] delegate:self delegateQueue:nil]; NSURLRequest *request = [NSURLRequest requestWithURL:[NSURL URLWithString:@""]]; // 创建任务(因为要使用代理方法,就不需要block方式的初始化了)和启动任务 //(1)一般请求任务 NSURLSessionDataTask *task = [self.session dataTaskWithRequest:request]; [task resume]; //(2)上传任务 NSURLSessionUploadTask *uploadtask = [self.session uploadTaskWithRequest:request fromData:request.HTTPBody]; [uploadtask resume]; //(3)下载任务 NSURLSessionDownloadTask *downloadTask = [self.session downloadTaskWithRequest:request]; [downloadTask resume];
//对应的代理方法如下:
# pragma mark NSURLSessionTaskDelegate // 请求成功或者失败(如果失败,error有值) - (void)URLSession:(NSURLSession *)session task:(NSURLSessionTask *)task didCompleteWithError:(NSError *)error { // 请求完成,成功或者失败的处理 if (self.completion) { NSData *data = nil; if (self.receivedData) { data = [self.receivedData copy]; self.receivedData = nil; } self.completion(self.cmd, task.response, data, error,error); } [self.session invalidateAndCancel]; } - (void)URLSession:(NSURLSession *)session task:(NSURLSessionTask *)task didSendBodyData:(int64_t)bytesSent totalBytesSent:(int64_t)totalBytesSent totalBytesExpectedToSend:(int64_t)totalBytesExpectedToSend { if (self.uploadBlock) { int64_t totalUnitCount = totalBytesExpectedToSend; if(totalUnitCount == NSURLSessionTransferSizeUnknown) { NSString *contentLength = [task.originalRequest valueForHTTPHeaderField:@"Content-Length"]; if(contentLength) { totalUnitCount = (int64_t) [contentLength longLongValue]; } } NSInteger totalBytesSentInt = [[NSNumber numberWithLongLong:totalBytesSent] integerValue]; NSInteger totalUnitCountInt = [[NSNumber numberWithLongLong:totalUnitCount] integerValue]; self.uploadBlock(totalBytesSentInt,totalUnitCountInt); } } - (void)URLSession:(NSURLSession *)session task:(NSURLSessionTask *)task willPerformHTTPRedirection:(NSHTTPURLResponse *)response newRequest:(NSURLRequest *)request completionHandler:(void (^)(NSURLRequest * __nullable))completionHandler{ //禁止302 301重定向 completionHandler(nil); } # pragma mark NSURLSessionDataDelegate // 接收到服务器的响应 - (void)URLSession:(NSURLSession *)session dataTask:(NSURLSessionDataTask *)dataTask didReceiveResponse:(NSURLResponse *)response completionHandler:(void (^)(NSURLSessionResponseDisposition))completionHandler { // 允许处理服务器的响应,才会继续接收服务器返回的数据 completionHandler(NSURLSessionResponseAllow); } // 接收到服务器的数据(可能调用多次) - (void)URLSession:(NSURLSession *)session dataTask:(NSURLSessionDataTask *)dataTask didReceiveData:(NSData *)data { // 处理每次接收的数据 [self.receivedData appendData:data]; } # pragma mark NSURLSessionDownloadDelegate // 每次写入调用(会调用多次) - (void)URLSession:(NSURLSession *)session downloadTask:(NSURLSessionDownloadTask *)downloadTask didWriteData:(int64_t)bytesWritten totalBytesWritten:(int64_t)totalBytesWritten totalBytesExpectedToWrite:(int64_t)totalBytesExpectedToWrite { if (self.downloadBlock) { NSInteger bytesWrittenInt = [[NSNumber numberWithLongLong:totalBytesWritten] integerValue]; NSInteger totalBytesExpectedToWriteInt = [[NSNumber numberWithLongLong:totalBytesExpectedToWrite] integerValue]; self.downloadBlock(bytesWrittenInt,totalBytesExpectedToWriteInt); } //另外, 可在这里通过已写入的长度和总长度算出下载进度 CGFloat progress = 1.0 * totalBytesWritten / totalBytesExpectedToWrite; NSLog(@"%f",progress); } // 下载完成调用 - (void)URLSession:(NSURLSession *)session downloadTask:(NSURLSessionDownloadTask *)downloadTask didFinishDownloadingToURL:(NSURL *)location { self.receivedData = [[NSData dataWithContentsOfURL:location.filePathURL] mutableCopy]; }
三、其他
task拥有下面三个方法:
- (void)suspend; - (void)resume; - (void)cancel;
suspend可以让当前的任务暂停;
resume方法不仅可以启动任务,还可以唤醒suspend状态的任务;
cancel方法可以取消当前的任务,你也可以向处于suspend状态的任务发送cancel消息,任务如果被取消便不能再恢复到之前的状态.
NSURLSessionConfiguration配置有三种类型:
+ (NSURLSessionConfiguration *)defaultSessionConfiguration; + (NSURLSessionConfiguration *)ephemeralSessionConfiguration; + (NSURLSessionConfiguration *)backgroundSessionConfigurationWithIdentifier:(NSString *)identifier
默认的配置会将缓存存储在磁盘上,第二种瞬时会话模式不会创建持久性存储的缓存,第三种后台会话模式允许程序在后台进行上传下载工作.
配置可以设置session的一些配置信息,如下举例:
NSURLSessionConfiguration *config = [NSURLSessionConfiguration defaultSessionConfiguration]; // 超时时间 config.timeoutIntervalForRequest = 10; // 是否允许使用蜂窝网络(后台传输不适用) config.allowsCellularAccess = YES; // 还有很多可以设置的属性
特别说明,本文是对简书《使用NSURLSession》的学习与记录,在使用方面做了一些调研和实验。