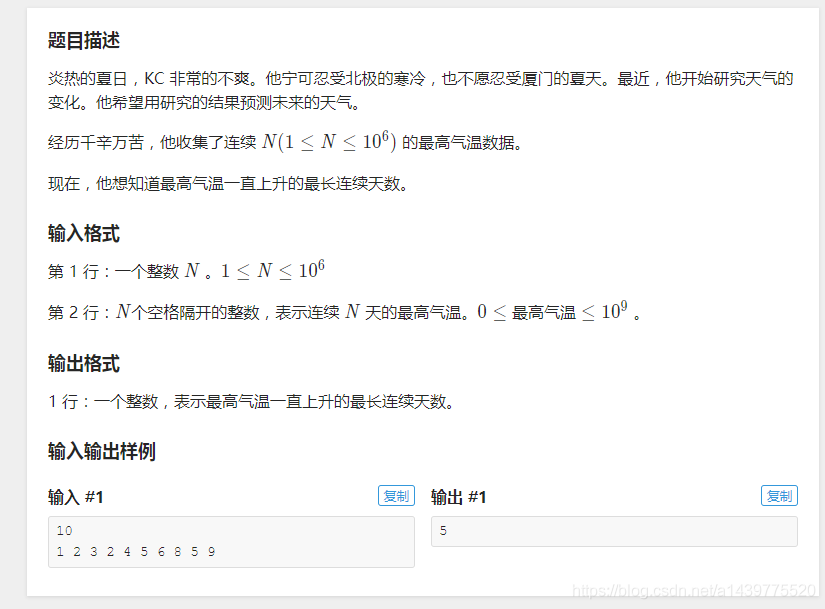
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.StringTokenizer;
public class Main {
private static CustomizedReader cin;
public static void main(String args[]) throws Exception {
cin = new CustomizedReader(System.in);
int n = cin.nextInt();
if(n<=0 || n>Math.pow(10, 7)) {
return;
}
int historyRecord = 0;
int newRecord = 0;
int startTemprature = -1;
int MAX = (int) Math.pow(10, 9);
for (int i = 0; i < n; i++) {
int t = cin.nextInt();
if(t>=0&&t<=MAX) {
if(t > startTemprature) {
newRecord++;
startTemprature = t;
}else {
if(newRecord > historyRecord) {
historyRecord = newRecord;
}
newRecord = 1;
startTemprature = t;
}
}
}
if(newRecord > historyRecord) {
historyRecord = newRecord;
}
System.out.println(historyRecord);
}
}
class CustomizedReader {
private BufferedReader reader = null;
private StringTokenizer tokenizer;
CustomizedReader(InputStream source){
reader = new BufferedReader(new InputStreamReader(source) );
tokenizer = new StringTokenizer("");
}
public String next() {
while ( ! tokenizer.hasMoreTokens() ) {
//TODO add check for eof if necessary
try {
tokenizer = new StringTokenizer(reader.readLine() );
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return tokenizer.nextToken();
}
public int nextInt() {
return Integer.parseInt(next());
}
public double nextDouble() {
return Double.parseDouble(next());
}
public double nextLong() {
return Long.parseLong(next());
}
public double nextFloat() {
return Float.parseFloat(next());
}
}