Description
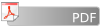
Matrix Chain Multiplication |
Suppose you have to evaluate an expression like A*B*C*D*E where A,B,C,D and E are matrices. Since matrix multiplication is associative, the order in which multiplications are performed is arbitrary. However, the number of elementary multiplications needed strongly depends on the evaluation order you choose.
For example, let A be a 50*10 matrix, B a 10*20 matrix and C a 20*5 matrix. There are two different strategies to compute A*B*C, namely (A*B)*C and A*(B*C).
The first one takes 15000 elementary multiplications, but the second one only 3500.
Your job is to write a program that determines the number of elementary multiplications needed for a given evaluation strategy.
Input Specification
Input consists of two parts: a list of matrices and a list of expressions.
The first line of the input file contains one integer n ( ), representing the number of matrices in the first part. The next n lines each contain one capital letter, specifying the name of the matrix, and two integers, specifying the number of rows and columns of the matrix.
The second part of the input file strictly adheres to the following syntax (given in EBNF):
SecondPart = Line { Line } <EOF> Line = Expression <CR> Expression = Matrix | "(" Expression Expression ")" Matrix = "A" | "B" | "C" | ... | "X" | "Y" | "Z"
Output Specification
For each expression found in the second part of the input file, print one line containing the word "error" if evaluation of the expression leads to an error due to non-matching matrices. Otherwise print one line containing the number of elementary multiplications needed to evaluate the expression in the way specified by the parentheses.
Sample Input
9 A 50 10 B 10 20 C 20 5 D 30 35 E 35 15 F 15 5 G 5 10 H 10 20 I 20 25 A B C (AA) (AB) (AC) (A(BC)) ((AB)C) (((((DE)F)G)H)I) (D(E(F(G(HI))))) ((D(EF))((GH)I))
Sample Output
0 0 0 error 10000 error 3500 15000 40500 47500 15125
按照题目的意思,不能进行乘法运算的自然是输出error,然而能进行乘法运算的,可以遵循下面条件进行进栈出栈即可:
1、左括号直接入栈,此处用x为-1值表示为左括号
2、右括号,让栈顶元素向下移动一格,即删除原顶部第二个的左括号。右括号不入栈。操作完成对栈顶开始能两两乘法运算的矩阵进行乘法结合。
3、矩阵,如果其能与栈顶元素进行乘法运算,就进行乘法结合。
ps:注意特判top为0的情况,在运算的同时记下乘法运算的个数。
代码:
#include <iostream> #include <cstdio> #include <cstdlib> #include <cstring> #include <cmath> #include <algorithm> #include <set> #include <map> #include <vector> #include <queue> #include <string> #define inf 0x3fffffff #define eps 1e-10 using namespace std; struct node { int x, y; }; node a[26], Stack[10000]; int top; int n; void Input() { char ch; int xx, yy; for (int i = 0; i < n; ++i) { getchar(); scanf("%c", &ch); scanf("%d%d", &a[ch-'A'].x, &a[ch-'A'].y); } } void No() { char ch; for (;;) { ch = getchar(); if (ch == ' ') break; } } int work() { node k; int ans = 0; top = 0; char ch; for (;;) { if ((ch = getchar()) == EOF) return -2; if (ch == ' ') return ans; if (ch == '(') { Stack[top].x = -1; top++; continue; } if (ch == ')') { Stack[top-2] = Stack[top-1]; top--; while (top > 1 && Stack[top-2].x != -1) { if (Stack[top-2].y != Stack[top-1].x) { No(); return -1; } ans += Stack[top-2].x * Stack[top-1].x * Stack[top-1].y; Stack[top-2].y = Stack[top-1].y; top--; } continue; } //'A'^'Z' if (top == 0) { Stack[top].y = a[ch-'A'].y; continue; } if (Stack[top-1].x == -1) { Stack[top++] = a[ch-'A']; continue; } if (Stack[top-1].y != a[ch-'A'].x) { No(); return -1; } ans += Stack[top-1].x * a[ch-'A'].x * a[ch-'A'].y; Stack[top-1].y = a[ch-'A'].y; } } void qt() { int ans; for (;;) { ans = work(); if (ans == -2) break; if (ans == -1) printf("error "); else printf("%d ", ans); } } int main() { //freopen ("test.txt", "r", stdin); scanf("%d", &n); Input(); getchar(); qt(); return 0; }