一、首先认识一下logger的作用以及级别
1、logger的作用
- 程序调试
- 了解程序运行是否正常
- 故障分析与问题定位
- 用户行为分析
2、logger的级别
- DEBUG:详细的信息,通常只出现在诊断问题上
- INFO:确认一切按预期运行
- WARNING(默认):一个迹象表明,一些意想不到的事情发生了,或表明一些问题在不久的将来(例如。磁盘空间低”)。这个软件还能按预期工作。
- ERROR:更严重的问题,软件没能执行一些功能
- CRITICAL:一个严重的错误,这表明程序本身可能无法继续运行
示例:
import logging
my_format= '%(asctime)s-%(filename)s-%(module)s-%(lineno)d'
logging.basicConfig(
filename='./my.log',
level=logging.INFO,
format=my_format
)
logging.info('infor')
logging.debug('debug')
logging.warning('waring')
logging.error('error')
logging.critical('critical')
二、logging模块的详细介绍
1、logging模块的使用
- 第一种方式就是使用logging提供的模块级别的函数
- 第二种方式就是使用Logging日志系统的四大组件
2、logging模块定义常用函数
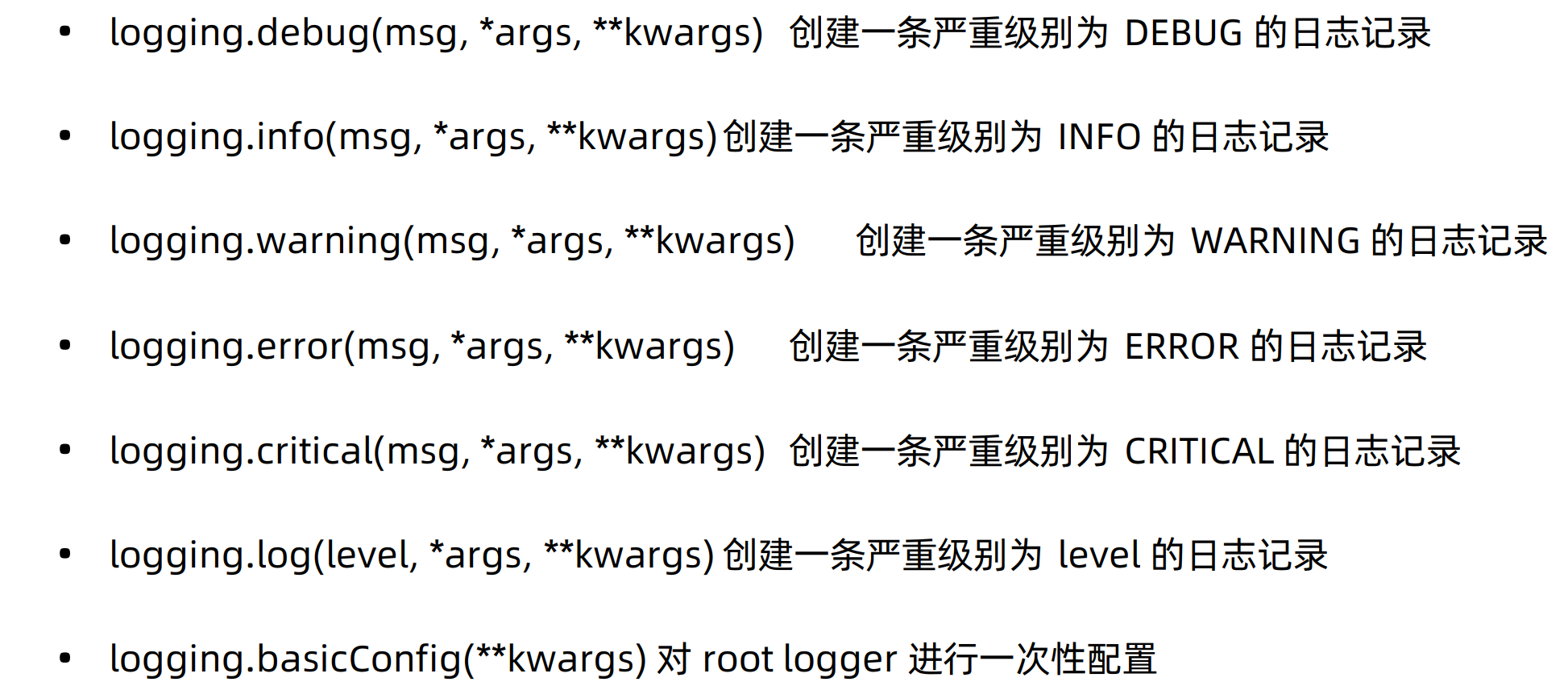
3、logging模块的四大组件
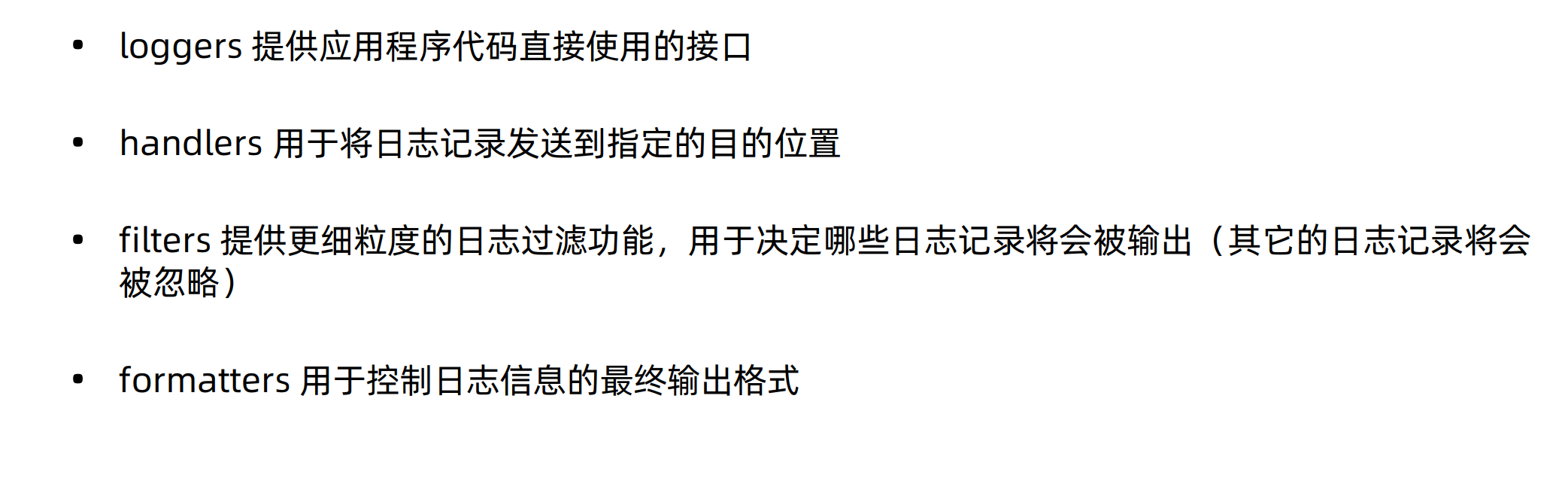
4、logging.basicConfig()函数说明
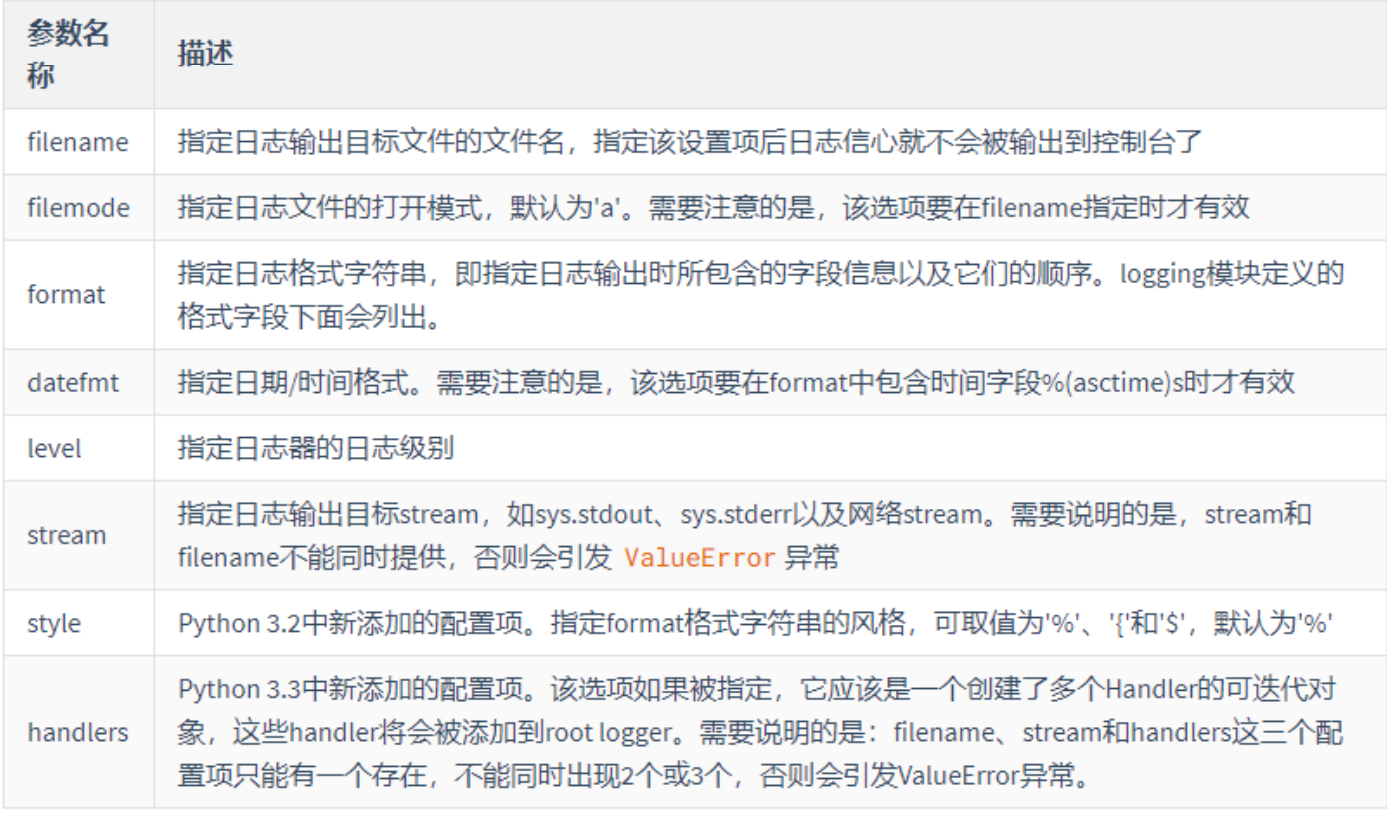
5、logging模块的格式字符串
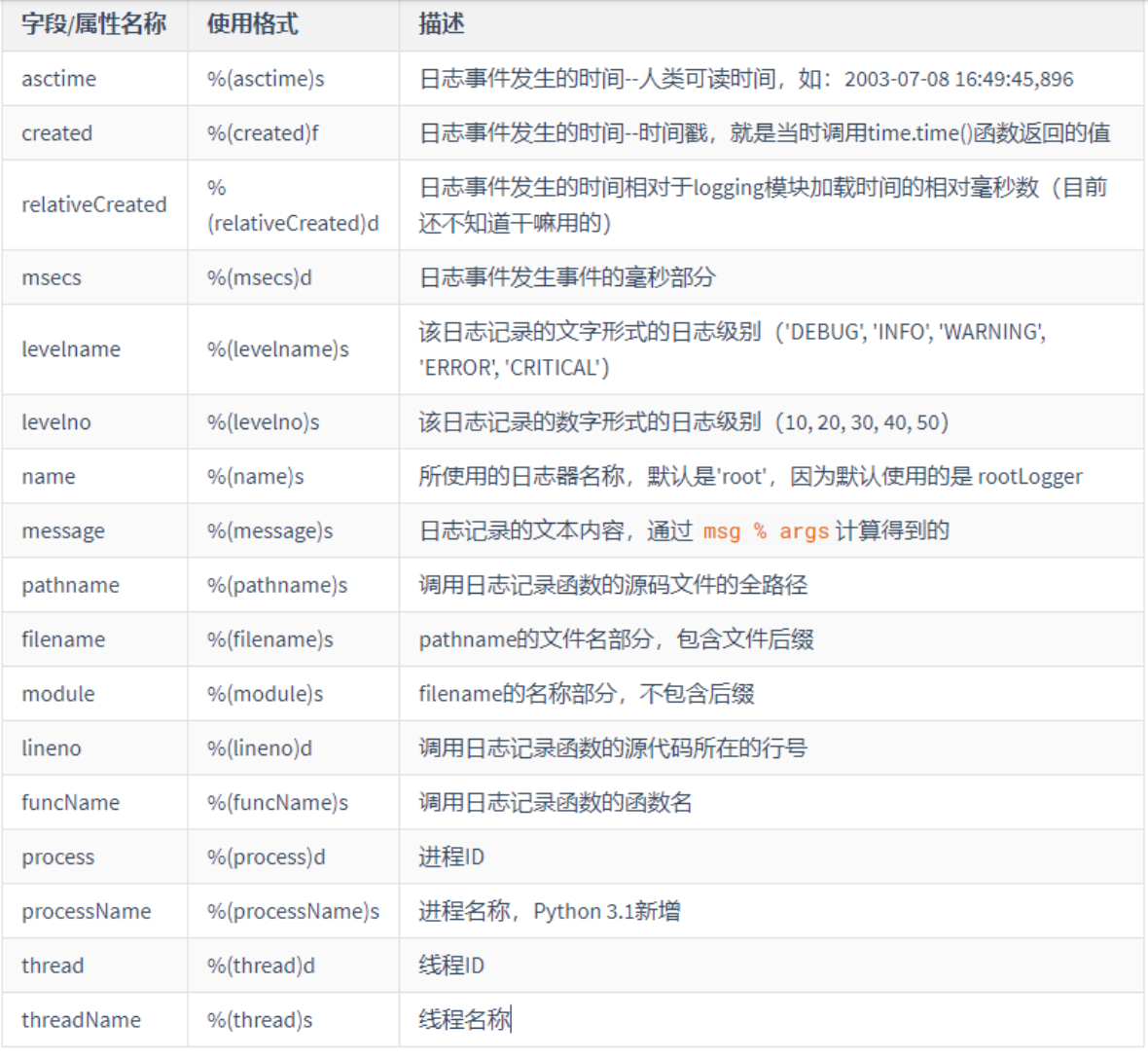
示例操作-封装: 新建util.py文件
import logging
import logging.handlers
import datetime
# 记录日志
def get_logger():
logger = logging.getLogger('mylogger')
logger.setLevel(logging.DEBUG)
rf_handler = logging.handlers.TimedRotatingFileHandler('D:TestSoftwarePyCharmPyCharmProjectJpressLogs\all.log', when='midnight', interval=1, backupCount=7,atTime=datetime.time(0, 0, 0, 0))
rf_handler.setFormatter(logging.Formatter('%(%(asctime)s-%(levelname)s-%(message)s'))
f_handler = logging.FileHandler('D:TestSoftwarePyCharmPyCharmProjectJpressLogserror.log')
f_handler.setLevel(logging.ERROR)
rf_handler.setFormatter(logging.Formatter('%(asctime)s-%(levelname)s-%(filename)s[:%(lineno)d - %(message)s]'))
logger.addHandler(rf_handler)
logger.addHandler(f_handler)
return logger
三、为管理员登录添加日志
from selenium import webdriver
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.support.wait import WebDriverWait
from Utils import Utils
from time import sleep
import pytest
import allure
# 管理员登录
@allure.feature('管理员登录页面')
class TestAdminLogin(object):
def setup_class(self):
self.driver = webdriver.Chrome()
self.driver.get("http://192.168.242.101:8080/jpress/admin/login")
self.driver.maximize_window()
self.logger = Utils.get_logger()
self.logger.info('测试用户登录')
@allure.title('管理员登录失败')
@allure.step('输入错误的账号密码登录')
@pytest.mark.skip()
def test_admin_login_err(self):
username = 'admin'
pwd = '123456'
captcha = '666'
excepted = '验证码不正确,请重新输入'
self.driver.find_element_by_name('username').send_keys(username)
self.logger.debug('输入用户名称: %s', username)
self.driver.find_element_by_name('pwd').send_keys(pwd)
self.logger.debug('输入密码: %s', pwd)
self.driver.find_element_by_name('captcha').send_keys(captcha)
self.logger.debug('输入验证码: %s', captcha)
self.driver.find_element_by_class_name('btn').click()
self.logger.debug('点击登录')
WebDriverWait(self.driver,5).until(EC.alert_is_present())
alert = self.driver.switch_to.alert
try:
assert alert.text == excepted
except AssertionError as ae:
self.logger.error('管理员登录失败:%s', 'test_admin_login_err',exc_info=1)
alert.accept()
sleep(3)
@allure.title('管理员登录成功')
@allure.step('输入正确的账号密码登录')
@pytest.mark.dependency(name='admin_login')
def test_admin_login_success(self):
username = 'admin'
pwd = 'admin'
captcha = '666'
excepted = 'JPress后台'
self.driver.find_element_by_name('username').clear()
self.driver.find_element_by_name('username').send_keys(username)
self.logger.debug('输入用户名称:%s', username)
self.driver.find_element_by_name('pwd').clear()
self.driver.find_element_by_name('pwd').send_keys(pwd)
self.logger.debug('输入用户名称:%s', pwd)
# 登录验证码的识别应该修改为Xpath //*[@id="form"]/div[3]/img
captcha = Utils.get_code(self.driver, 'captchaimg')
self.driver.find_element_by_name('captcha').clear()
self.driver.find_element_by_name('captcha').send_keys(captcha)
self.logger.debug('输入验证码: %s', captcha)
self.driver.find_element_by_class_name('btn').click()
self.logger.debug('管理员-登录成功!!!')
WebDriverWait(self.driver, 5).until(EC.alert_is_present())
alert = self.driver.switch_to.alert
assert excepted == self.driver.title
sleep(3)