1.打开蓝牙适配器
openBluetoothAdapter() {
const that = this
wx.openBluetoothAdapter({
success(res) {
console.log('open blueFi success: ', res)
that.onBluetoothDeviceFound()
that.onBluetoothAdapterStateChange()
that.startBluetoothDevicesDiscovery()
},
fail(error) {
let errorRes = blueFi.fail(error)
console.log(errorRes)
}
})
},
2.打开蓝牙搜索功能
startBluetoothDevicesDiscovery() {
wx.startBluetoothDevicesDiscovery({
services: UUIDS,
success(res) {
console.log("打开蓝牙搜索功能成功")
}
})
}
3.监听寻找到新设备的事件
onBluetoothDeviceFound() {
const that = this
wx.onBluetoothDeviceFound((res) => {
var devices = res.devices;
that.setData({
devices: devices
})
})
},
4.监听本机蓝牙状态变化的事件
onBluetoothAdapterStateChange() {
wx.onBluetoothAdapterStateChange(function(res) {
console.log('adapterState changed, now is', res)
})
}
5.获取已发现的蓝牙设备 包括 已经和本机处于连接状态的设备
getBluetoothDevices(){
uni.getBluetoothDevices({
success(res) {
console.log(res)
if (res.devices[0]) {
console.log(ab2hex(res.devices[0].advertisData))
}
}
})
}
6.获取特征值
getBLEDeviceCharacteristics(){
uni.getBLEDeviceCharacteristics({
// 这里的 deviceId 需要已经通过 createBLEConnection 与对应设备建立链接
deviceId,
// 这里的 serviceId 需要在 getBLEDeviceServices 接口中获取
serviceId,
success(res) {
console.log('device getBLEDeviceCharacteristics:', res.characteristics)
}
})
}
7.开启订阅值
notifyBLECharacteristicValueChange(){
uni.notifyBLECharacteristicValueChange({
state: true, // 启用 notify 功能
// 这里的 deviceId 需要已经通过 createBLEConnection 与对应设备建立链接
deviceId,
// 这里的 serviceId 需要在 getBLEDeviceServices 接口中获取
serviceId,
// 这里的 characteristicId 需要在 getBLEDeviceCharacteristics 接口中获取
characteristicId,
success(res) {
var value = that.ab2hex(res.value); // ArrayBuffer转16进度字符串示例
console.log('notifyBLECharacteristicValueChange success', res.errMsg)
}
})
}
8. 连接设备
createBLEConnection(){
uni.getBLEDeviceServices({
// 这里的 deviceId 需要已经通过 createBLEConnection 与对应设备建立链接
deviceId,
success(res) {
console.log('device services:', res.services)
this.getBLEDeviceCharacteristics() //获取特征值
setTimeout(function() {
that.notifyBLECharacteristicValue() // 开启订阅
let wifi = "chenKKK";
let newWifi = stringToUint8Array(wifi ) // string 转化为 unit8array的函数
that.writeBLECharacteristicValue('53',5,newWifi ) //发送写入数据 // 指令, 长度, 值 , 这里根据设备的协议发
}, 2000)
}
})
}
9.写入数据
writeBLECharacteristicValue(typeNum,subCMD, data) {
let that = this;
let deviceId = this.bindDeviceMes.deviceId;
let serviceId = this.bindDeviceMes.serviceId;
let characteristicId = this.bindDeviceMes.writeId;
var beffer = new ArrayBuffer(20)
var bufView = new Uint8Array(beffer)
bufView[0] = typeNum // 指令1
bufView[1] = subCMD ? bufView[1] = subCMD : bufView[1] = data.length // 指令2
for(let i = 0; i < 18; i++){ // 3-18位值(一共20,我这里不满20位补0)
let item = data[i]
if(!item){
bufView[2+i] = 0
}else{
bufView[2 +i] = data[i]
}
}
console.log('发送写入指令:' + String(bufView))
// plus.bluetooth.writeBLECharacteristicValue({ //12.25更改
uni.writeBLECharacteristicValue({
// 这里的 deviceId 需要在 getBluetoothDevices 或 onBluetoothDeviceFound 接口中获取
deviceId,
// 这里的 serviceId 需要在 getBLEDeviceServices 接口中获取1
serviceId,
// 这里的 characteristicId 需要在 getBLEDeviceCharacteristics 接口中获取
characteristicId,
// 这里的value是ArrayBuffer类型
value: beffer,
success(res) {
console.log('写入成功' + JSON.stringify(res))
that.testList.push('写入数据成功' + JSON.stringify(res)) //test4
},
fail(res) {
that.testList.push('写入数据失败' + JSON.stringify(res)) //test4
console.log("写入数据失败" + res);
}
});
},
10. 断开蓝牙连接
closeBluetoothAdapter() {
let that = this;
that.reConnectNum = 0
let deviceId = this.bindDeviceMes.deviceId;
uni.closeBLEConnection({
deviceId,
success(res) {
that.testList.push('断开蓝牙模块连接') //test4
}
})
uni.closeBluetoothAdapter({
success: res => {
that.testList.push('断开蓝牙模块成功') //test4
console.log('断开蓝牙模块成功');
}
});
that.blueHadConnected = false;
},
11.ArrayBuffer转16进度字符串示例 (接受蓝牙返回时使用)
function ab2hex(buffer) {
const hexArr = Array.prototype.map.call(
new Uint8Array(buffer),
function (bit) {
return ('00' + bit.toString(16)).slice(-2)
}
)
return hexArr.join('')
}
12. 将string 转化为 unit8array的函数
const stringToUint8Array = function(string) {
let pos = 0;
const len = string.length;
const out = [];
let at = 0; // output position
let tlen = Math.max(32, len + (len >> 1) + 7); // 1.5x size
let target = new Uint8Array((tlen >> 3) << 3); // ... but at 8 byte offset
while (pos < len) {
let value = string.charCodeAt(pos++);
if (value >= 0xd800 && value <= 0xdbff) {
// high surrogate
if (pos < len) {
const extra = string.charCodeAt(pos);
if ((extra & 0xfc00) === 0xdc00) {
++pos;
value = ((value & 0x3ff) << 10) + (extra & 0x3ff) + 0x10000;
}
}
if (value >= 0xd800 && value <= 0xdbff) {
continue; // drop lone surrogate
}
}
// expand the buffer if we couldn't write 4 bytes
if (at + 4 > target.length) {
tlen += 8; // minimum extra
tlen *= (1.0 + (pos / string.length) * 2); // take 2x the remaining
tlen = (tlen >> 3) << 3; // 8 byte offset
const update = new Uint8Array(tlen);
update.set(target);
target = update;
}
if ((value & 0xffffff80) === 0) { // 1-byte
target[at++] = value; // ASCII
continue;
} else if ((value & 0xfffff800) === 0) { // 2-byte
target[at++] = ((value >> 6) & 0x1f) | 0xc0;
} else if ((value & 0xffff0000) === 0) { // 3-byte
target[at++] = ((value >> 12) & 0x0f) | 0xe0;
target[at++] = ((value >> 6) & 0x3f) | 0x80;
} else if ((value & 0xffe00000) === 0) { // 4-byte
target[at++] = ((value >> 18) & 0x07) | 0xf0;
target[at++] = ((value >> 12) & 0x3f) | 0x80;
target[at++] = ((value >> 6) & 0x3f) | 0x80;
} else {
// FIXME: do we care
continue;
}
target[at++] = (value & 0x3f) | 0x80;
}
return target.slice(0, at);
}
13. unit8array转化为 string的方法
function Unit8ArrayToStr(array) {
var out, i, len, c;
var char2, char3;
out = "";
len = array.length;
i = 0;
while (i < len) {
c = array[i++];
switch (c >> 4) {
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
case 6:
case 7:
// 0xxxxxxx
out += String.fromCharCode(c);
break;
case 12:
case 13:
// 110x xxxx 10xx xxxx
char2 = array[i++];
out += String.fromCharCode(((c & 0x1F) << 6) | (char2 & 0x3F));
break;
case 14:
// 1110 xxxx 10xx xxxx 10xx xxxx
char2 = array[i++];
char3 = array[i++];
out += String.fromCharCode(((c & 0x0F) << 12) |
((char2 & 0x3F) << 6) |
((char3 & 0x3F) << 0));
break;
}
}
return out;
}
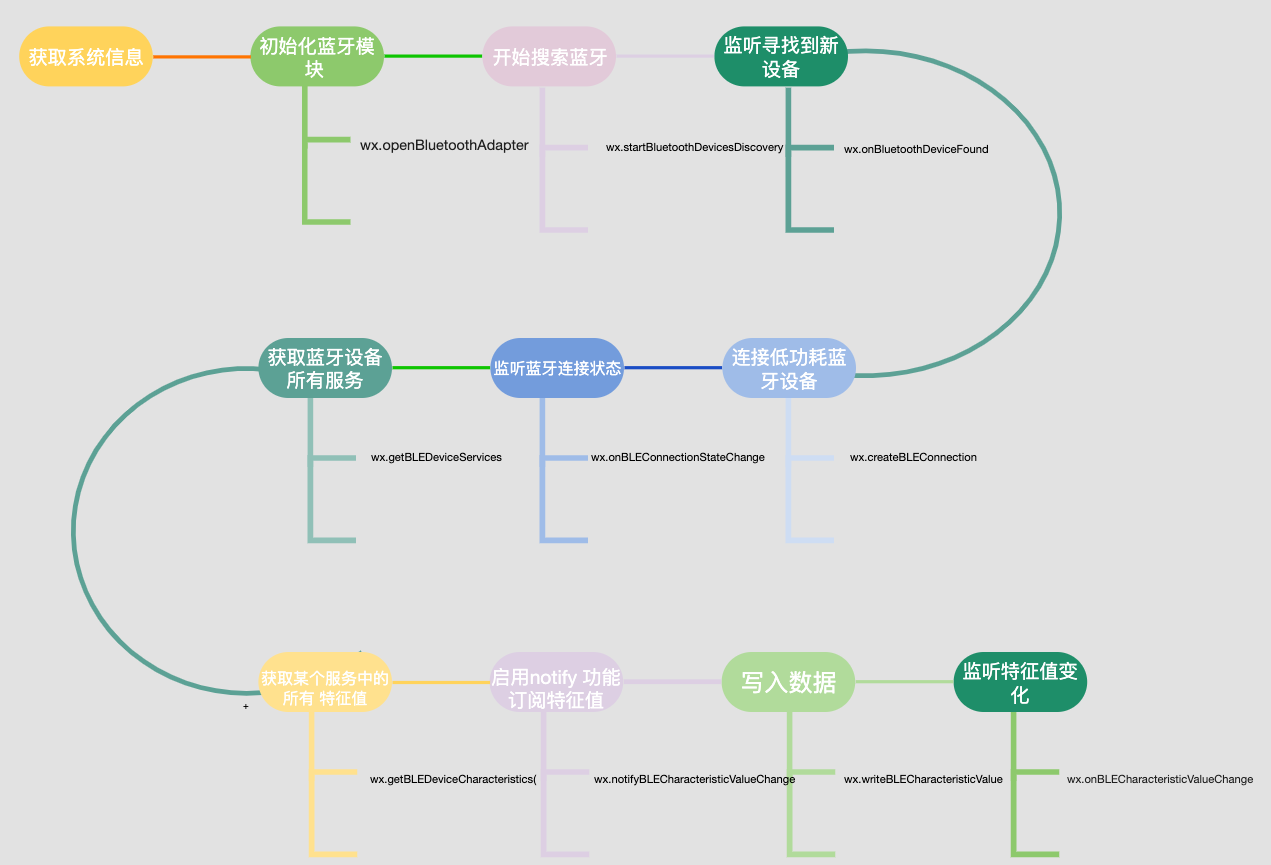
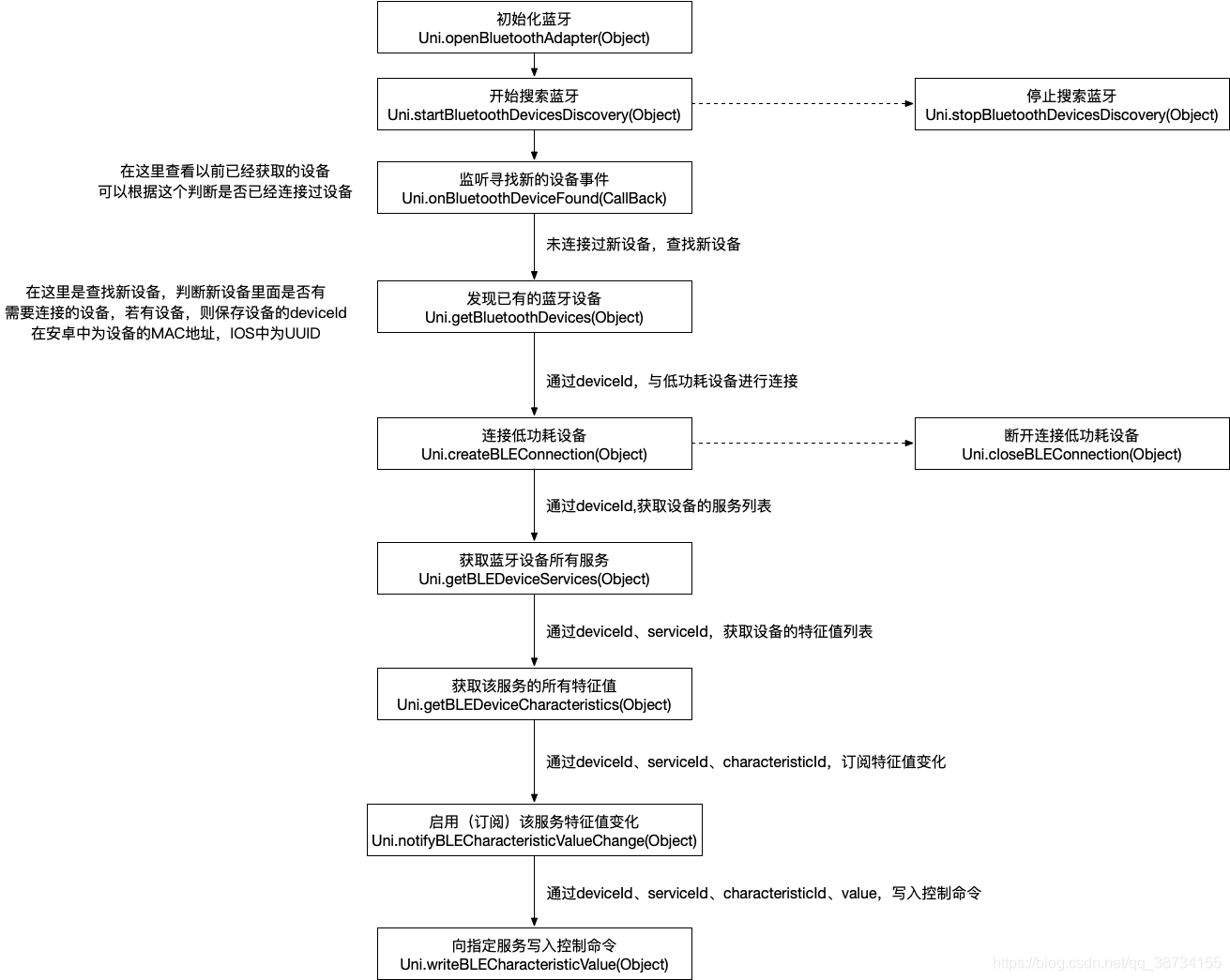