Java的数据类型:
- 基本类型:int boolean float
- 引用类型:所有class类型
为一个基本类型int赋值为null,会提示“incompatible types”错误。
错误原始:基本类型是不能视为对象(引用类型)的.。
可以定义一个Integer类,包含一个实例字段int。这样可以把Integer视为int的包装类型wrapper。
包装类型本身是引用类型,只是其内部持有一个基本类型的变量。
JDK为每种基本类型都创建了对应的包装类型:
基本类型 | 对应的引用类型 |
---|---|
boolean | Boolean |
byte | Byte |
short | Short |
int | Integer |
long | Long |
float | Float |
double | Double |
char | Character |
使用JDK的Integer类传入null,语句不会再报错,只是执行时会抛出java.lang.NumberFormatException
Integer n = new Integer(null);
int i = n;
System.out.println(i);
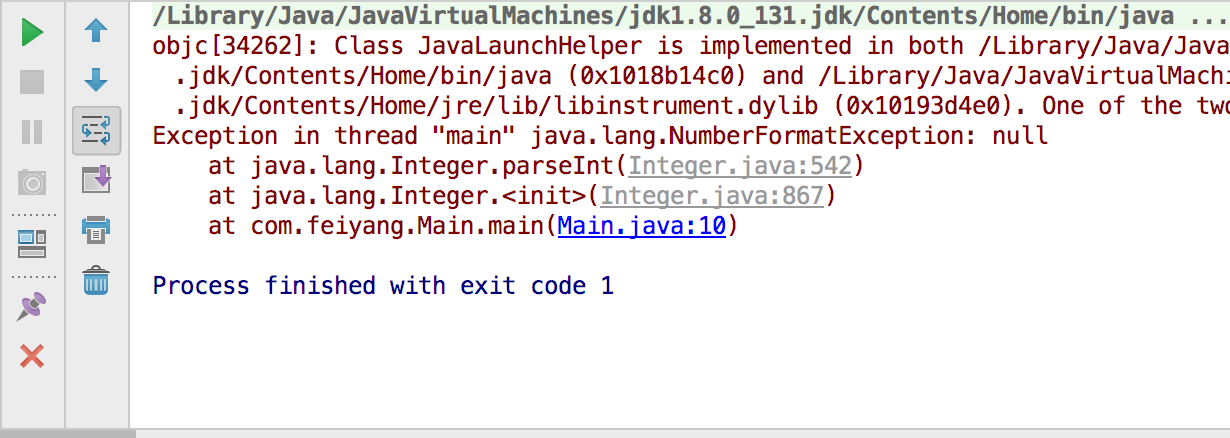
类型转换
int i = 100;
//把int、String转换为Integer
Integer n1 = new Integer(i);
Integer n2 = Integer.valueOf(i);
Integer n3 = Integer.valueOf("100");
//把Integer、String转换为int类型
int x1 = n1.intValue();
int x3 = Integer.parseInt("100");
//把Integer、int转换为String类型
String s1 = n1.toString();
String s2 = String.valueOf(i);
自动拆箱和自动装箱
编译器何以自动在int和Integer之间转型。
- 自动装箱auto boxing int -> Integer
Integer n = 99;//自动调用Integer.valueOf(99)
- 自动拆箱auto unboxing Integer -> int
int i = n;//n.intValue()
注意:
* 自动装箱和自动拆箱只发生在编译阶段
* 装箱和拆箱会影响执行效率
* 编译后的class代码是严格区分基本类型和引用类型的,没有自动装箱和拆箱
* Integer的null->int执行时会抛出<font color=#FF0000>java.lang.NumberFormatException</font>
包装类型定义了一些有用的静态变量
```#java
Boolean t = Boolean.TRUE;
Boolean f = Boolean.FALSE;
int max = Integer.MAX_VALUE;
int min = Integer.MIN_VALUE;
int sizeOfLong = Long.SIZE;
int bytesOfLong = Long.BYTES;
Java的包装类型全部继承自Number这个class。可以利用向上转型,把任意一种包装类型先变为Number对象,利用Number提供的Byte、Integer、Float变为任意的类型。
Integer n = 5 + Integer.valueOf(10);
Number m = n;
System.out.println(m.floatValue());//15.0
总结:
JDK的包装类型可以把基本类型包装为class
自动装箱和自动拆箱是编译器完成的 (JDK>= 1.5)
装箱和拆箱会影响执行效率
注意拆箱时kennel发生NullPointException