1.栈的定义
栈Stack是一种后进先出(LIFO: Last In First Out)的数据结构,可以看作一端封闭的容器,先进去的元素永远在底部,最后出来。
栈有2个重要的方法:
- push(E e):把元素压栈
- pop(E e):把栈顶的元素弹出
2.Qeque
用Deque可以实现Stack的功能。Deque接口有3个方法,push、pop、peek,只需要调用这3个方法就可以,不需要调用对应的addFirst、removeFirst、peekFirst。
- push(E e) : addFirst(E e)
- pop() : removeFirst()
- peek() : peekFirst()
为什么Java的集合类没有单独的Stack接口呢?
有一个遗留的class名字就叫Stack,出于兼容性的考虑,就没有创建Stack的接口了
3.Stack的作用:
3.1方法(函数)的嵌套使用
Java在处理Java方法调用的时候,就会通过栈这种数据结构来维护方法的调用层次,如通过main方法调用foo方法,foo方法调用bar方法。
static void main(String[] args) {
foo(123);
}
static String foo(int x){
return "F-" + bar(x+1);
}
static int bar(int x){
return x << 2;
}
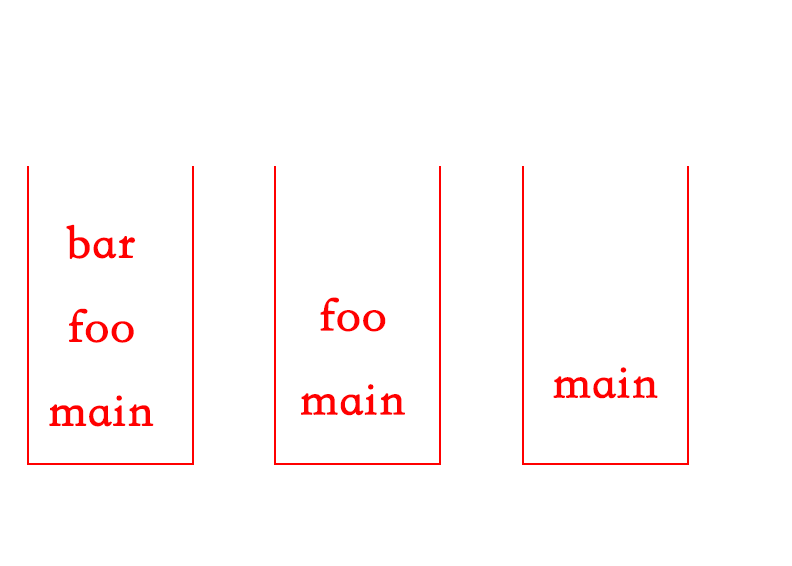
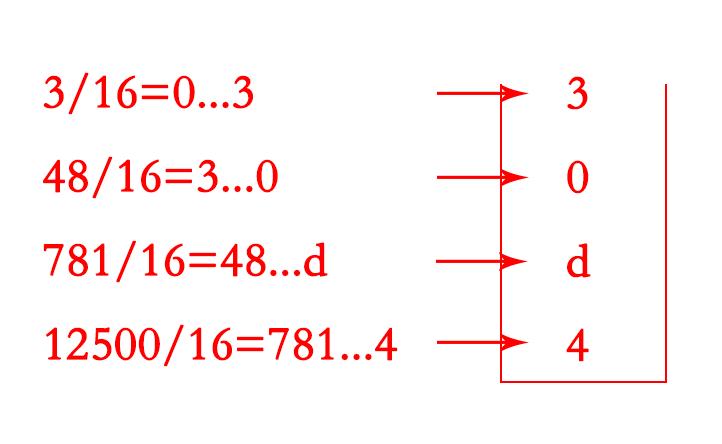
public class Main {
public static void main(String[] args) {
int x = 12500;
System.out.println(x+" 转换为十六进制字符串-->"+shang(x));
}
static String shang(Integer x){
Deque
Map map1 = new HashMap();
map1.put("10","a");
map1.put("11","b");
map1.put("12","c");
map1.put("13","d");
map1.put("14","e");
map1.put("15","f");
while(x != 0 ){
Integer result = x%16;
if (result > 9){
String index = String.valueOf(result);
String resultValue = (String) map1.get(index);
stack.push(String.valueOf(resultValue));
}else{
String resultValue = String.valueOf(result);
stack.push(String.valueOf(resultValue));
}
x = x / 16;
}
String result = "0x";
while(stack.size()>0){
result = result + stack.pop();
}
return result;
}
}
<img src="https://img2018.cnblogs.com/blog/1418970/201903/1418970-20190324170553019-943809392.png" width="500" />
### 3.4将中缀表达式编译为后缀表达式
中缀表达式:1 + 2 * (9 - 5)
后缀表达式:1 2 9 5 - * +
## 4.总结
* 栈Stack是一种后进先出的数据结构
* 操作栈的元素的方法:
* push(E e) 压栈
* pop() 出栈
* peek() 取栈顶元素但不出栈
* Java使用Deque实现栈的功能,注意只调用push/pop/peek,避免调用Deque的其他方法
* 不要使用遗留类Stack