1. dao层增加更新店铺的方法
package com.csj2018.o2o.dao;
import com.csj2018.o2o.entity.Shop;
public interface ShopDao {
/**
* 新增店铺
* @param shop
* @return 返回影响的行数;-1插入失败
*/
int insertShop(Shop shop);
/**
* 更新店铺信息
* @param shop
* @return 返回影响的行数
*/
int updateShop(Shop shop);
}
2.mapper增加update方法的配置
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<!-- useGeneratedKeys="true",一旦数据插入成功,使用JDBC的getGeneratedKeys获取数据库自增主键值 -->
<mapper namespace="com.csj2018.o2o.dao.ShopDao">
<insert id="insertShop" useGeneratedKeys="true"
keyColumn="shop_id" keyProperty="shopId">
insert into
tb_shop(owner_id, area_id, shop_category_id, shop_name,
shop_desc, shop_addr, phone, shop_img, priority,
create_time, last_edit_time, enable_status, advice)
values
(#{owner.userId}, #{area.areaId}, #{shopCategory.shopCategoryId}, #{shopName},
#{shopDesc}, #{shopAddr}, #{phone}, #{shopImg}, #{priority},
#{createTime}, #{lastEditTime}, #{enableStatus}, #{advice});
</insert>
<update id="updateShop" parameterType="com.csj2018.o2o.entity.Shop">
update tb_shop
<set>
<if test="shopName != null">shop_name=#{shopName},</if><!--Mybatis的另外一个优势是支持动态SQL的生成-->
<if test="shopDesc != null">shop_desc=#{shopDesc},</if><!--<if test="属性 != null ">表字段=#{属性},</if>不是最后一行要加 ' , '-->
<if test="shopAddr != null">shop_addr=#{shopAddr},</if>
<if test="phone != null">phone=#{phone},</if>
<if test="shopImg != null">shop_img=#{shopImg},</if>
<if test="priority != null">priority=#{priority},</if>
<if test="lastEditTime != null">last_edit_time=#{lastEditTime},</if>
<if test="enableStatus != null">enable_status=#{enableStatus},</if>
<if test="advice != null">advice=#{advice},</if>
<if test="area != null">area_id=#{area.areaId},</if>
<if test="shopCategory != null">shop_category_id=#{shopCategory.shopCategoryId}</if>
</set>
where shop_id=#{shopId}
</update>
</mapper>
2.1 为什么需要动态SQL呢?
假设某一张表只有A和B两列,有时只需要更新A,有时只需要更新B,有时A和B都要更新。
如果不支持动态语句的话,就需要写3条update语句才能满足数据的更新,如果支持动态SQL,只需要写一个方法就可以了
<if test="shopName != null">shop_name=#{shopName},</if>
<!--先判断是否传入了shopName,如果传入,shopName不等于null,条件成立,将shop_name=#{shopName}添加到更新语句-->
3.测试类编写测试方法
package com.csj2018.o2o.dao;
import static org.junit.Assert.assertEquals;
import java.util.Date;
import org.junit.Ignore;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import com.csj2018.o2o.BaseTest;
import com.csj2018.o2o.entity.Area;
import com.csj2018.o2o.entity.PersonInfo;
import com.csj2018.o2o.entity.Shop;
import com.csj2018.o2o.entity.ShopCategory;
public class ShopDaoTest extends BaseTest{
@Autowired
private ShopDao shopDao;
@Test
@Ignore
public void testInsertShop() {}
@Test
public void testUpdateShop() {
Shop shop = new Shop();
shop.setShopId(1L);
shop.setShopDesc("江南皮革厂");
shop.setShopAddr("皮革厂路1号");
shop.setPhone("0571-3770571");
shop.setLastEditTime(new Date());
int effectedNum = shopDao.updateShop(shop);
assertEquals(1,effectedNum);
System.out.print(effectedNum);
}
}
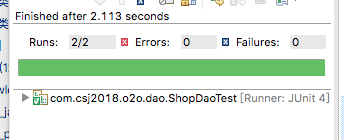