testNG是java中的一套测试框架,它基于JUnit思想并强化了测试注解,可以灵活应用于各种测试场景。
maven依赖
<!-- https://mvnrepository.com/artifact/org.testng/testng -->
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>6.14.3</version>
<scope>test</scope>
<dependency>
用例的编写
@BeforeGroups:此测试组内所有测试之前
@AfterGroups:此测试组内所有测试都运行之后
@BeforeClass:测试类之前运行,用于测试前的准备工作
@AfterClass:测试类之后运行,用于测试后清理工作
@BeforeTest:测试方法前运行,只运行一次
@AfterTest:测试方法后运行,只运行一次
@BeforeMethod:每个测试方法之前都运行
@AfterMethod:每个测试方法之后都运行
import org.testng.annotations.*;
public class Lesson1 {
@BeforeClass
public void testBeforeClass(){
System.out.println("before class");
}
@Test(groups = "a")
public void test_a(){
System.out.println("test_a");
}
@BeforeTest
public void beforeTest(){
System.out.println("before test");
}
@BeforeMethod
public void beforeMethod(){
System.out.println("before method");
}
@Test
public void test_b(){
System.out.println("test_b");
}
@BeforeGroups(groups = "a")
public void beforeGroup(){
System.out.println("before group a");
}
}
执行结果:
before test
before class
before group a
before method
test_a
before method
test_b
===============================================
Default Suite
Total tests run: 2, Failures: 0, Skips: 0
Test方法的细节
分组和执行次数,用例级别
public class Lesson1 {
@BeforeClass
public void testBeforeClass(){
System.out.println("before class");
}
@Test(groups = "a",priority = 5) //设置级别,数字小的优先执行
public void test_a(){
System.out.println("test_a");
}
@BeforeTest
public void beforeTest(){
System.out.println("before test");
}
@BeforeMethod
public void beforeMethod(){
System.out.println("before method");
}
@Test(groups = "b",invocationCount = 5,priority = 2,threadPoolSize = 3) //设置线程组,1次执行3个
public void test_b(){
System.out.println("test_b");
}
@BeforeGroups(groups = "a")
public void beforeGroup(){
System.out.println("before group a");
}
}
before test
before class
before method
before method
before method
test_b
test_b
test_b
before method
before method
test_b
test_b
before group a
before method
test_a
===============================================
Default Suite
Total tests run: 6, Failures: 0, Skips: 0
用例的管理
通过testNG.xml可以对用例进行管理。https://testng.org/doc/documentation-main.html#testng-xml
1.执行指定包的测试用例
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Suite1" verbose="1" >
<test name="Nopackage" >
<packages>
<package name="date89"/>
</packages>
</test>
</suite>
2.执行指定类
#xml
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Suite1" verbose="1" >
<test name="part1" >
<classes>
<class name="date89.Lesson1" />
</classes>
</test>
<test name="part2">
<classes>
<class name="date89.Lesson2"/>
</classes>
</test>
</suite>
3.执行指定组
注意:在组后要指定执行的类或包,否则结果为空,如
<suite name="Regression1" verbose="1">
<test name="Lesson1" >
<groups>
<run>
<include name="chen" />
</run>
</groups>
</test>
</suite>```
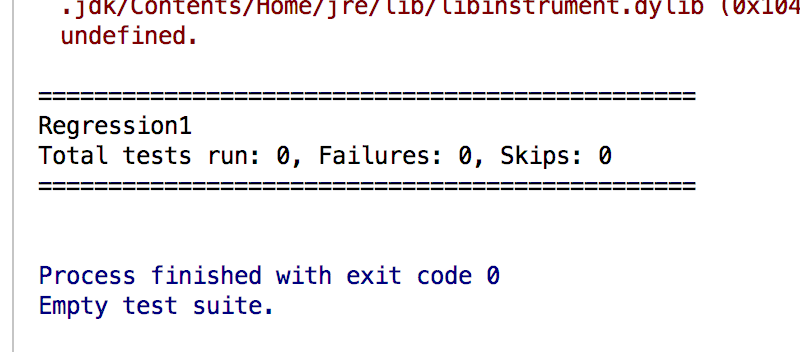
``` #xml
<suite name="Regression1" verbose="1">
<test name="Lesson1" >
<groups>
<run>
<include name="chen" />
</run>
</groups>
<classes>
<class name="date89.Lesson1"></class>
</classes>
</test>
</suite> ```
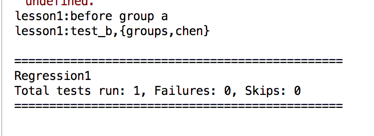
``` #xml
<suite name="Regression1" verbose="1">
<test name="Lesson1" >
<groups>
<run>
<include name="chen" />
</run>
</groups>
<packages>
<package name="date89" />
</packages>
</test>
</suite> ```
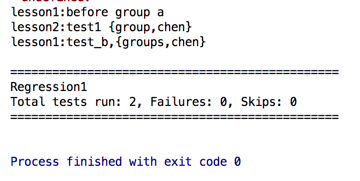
```#xml 重新定义组
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Regression1" verbose="1">
<test name="Lesson1" >
<groups>
<define name="new">
<include name="chen"/>
<include name="a"/>
</define>
<run>
<include name="chen" />
</run>
</groups>
<packages>
<package name="date89"/>
</packages>
</test>
</suite>
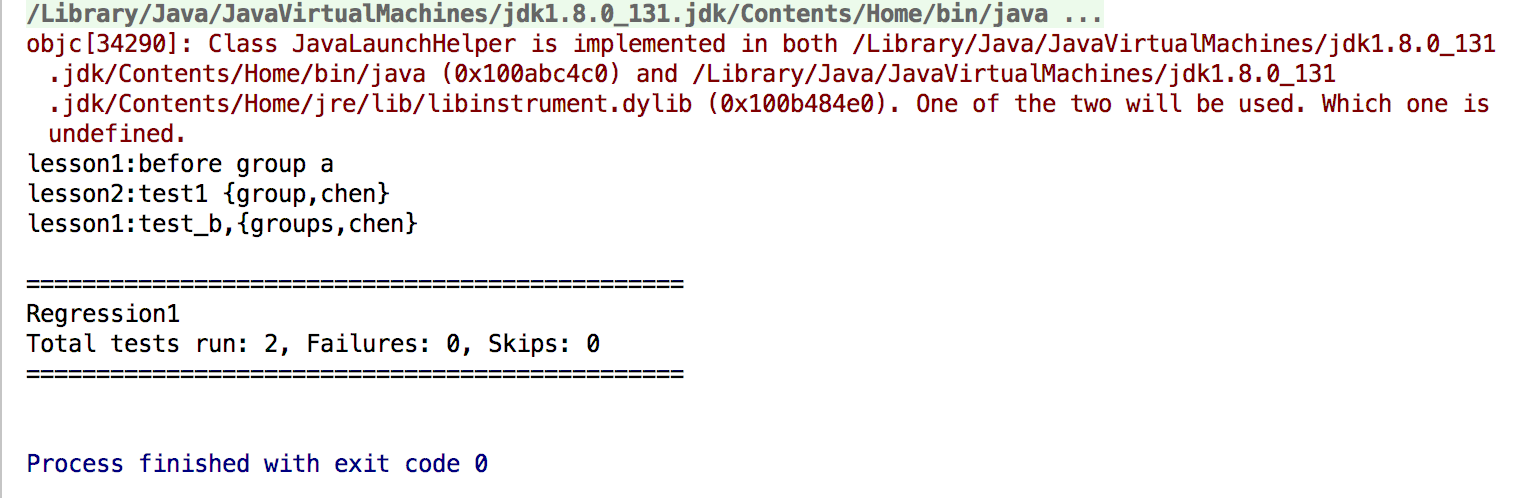
## 4.执行指定某个测试用例
``` #xml
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Regression1" verbose="1">
<test name="part1">
<classes>
<class name="date89.Lesson2">
<methods>
<include name="lesson2Test2"/>
<exclude name="lesson2Test1"/>
</methods>
</class>
</classes>
</test>
</suite>
#java
//Lesson1.java
package date89;
import com.google.common.base.StandardSystemProperty;
import org.testng.annotations.*;
public class Lesson1 {
@BeforeClass
public void lesson1BeforeClass(){
System.out.println("lesson1:before class");
}
@Test(groups = "a",priority = 5)
public void test_a(){
System.out.println("lesson1:test_a,{groups,a}");
}
@BeforeTest
public void beforeTest(){
System.out.println("lesson1:before test");
}
@BeforeMethod
public void lesson1BeforeMethod(){
System.out.println("lesson1:before method");
}
@Test(groups = {"chen"},priority = 2)
public void lesson1Test_b(){
System.out.println("lesson1:test_b,{groups,chen}");
}
@BeforeGroups(groups = {"chen"})
public void lesson1BeforeGroup(){
System.out.println("lesson1:before group a");
}
}
//Lesson2
package date89;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
import sun.java2d.pipe.ShapeSpanIterator;
public class Lesson2 {
@BeforeTest
public void lesson2BeforeTest1(){
System.out.println("lesson2:before test");
}
@BeforeClass
public void lesson2BeforeClass1(){
System.out.println("lesson2:before class");
}
@Test(groups = {"chen"})
public void lesson2Test1(){
System.out.println("lesson2:test1 {group,chen}");
}
@Test
public void lesson2Test2(){
System.out.println("lesson2:test2");
}
}
//Lesson3.java
package date89;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
public class lesson3 {
@BeforeTest
public void lesson3BeforeTest1(){
System.out.println("lesson3:before test");
}
@BeforeClass
public void lesson3BeforeClass1(){
System.out.println("lesson3:before class");
}
@Test
public void lesson3Test(){
System.out.println("lesson3:test");
}
}