Given a string, remove all leading/trailing/duplicated empty spaces.
Assumptions:
The given string is not null.
Examples:
“ a” --> “a”
“ I love MTV ” --> “I love MTV”
1 public String removeSpaces(String input) {
2 // Write your solution here
3 //corner case
4 if (input.isEmpty()){
5 return input ;
6 }
7 /*
8 return [0, i) j is the counter
9 * case 1: not space: input[i++] = input[j++]
10 * case 2: if space
11 * 2.1: leading space: skip
12 * i = 0
13 * 2.2: duplicate space: keep the first space
14 * j != j-1 : j一定是SPACE, J-1 如果不是的话,把当前的SPACE 给I 吃
15 * 2.3: trailing space : remove
16 * j to the end, and i = "", i--
17 * */
18 int slow = 0, fast = 0;
19 char[] chars = input.toCharArray();
20 while (fast < chars.length){
21 if (chars[fast] !=' ' ){
22 chars[slow++] = chars[fast++] ;
23 } else{
24 //非开头, 中间没连续_ 末尾可能进去一个 _
25 if (slow>0 && chars[fast] != chars[fast-1]){
26 chars[slow++] = chars[fast++] ;
27 } else{
28 fast++ ;
29 }
30 }
31 }
32 //post-processing: it is possible we still have one trailing ' '
33 if (slow>0 && chars[slow-1] == ' '){
34 return new String(chars, 0, slow-1) ;
35 }
36 return new String(chars, 0, slow) ;
37 }
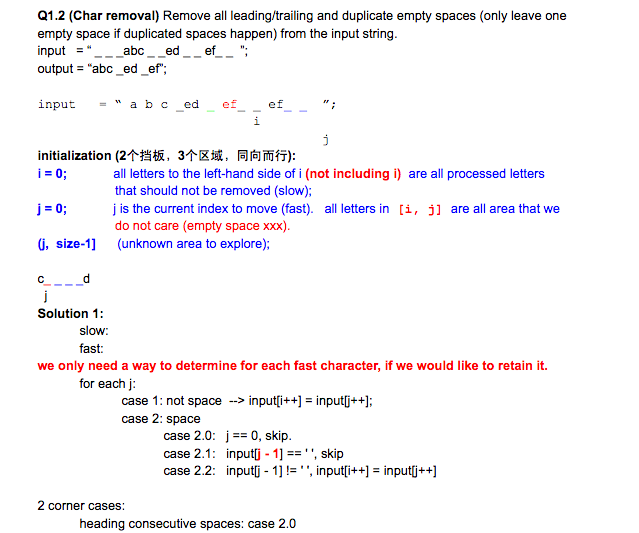
