---恢复内容开始---
这个作业属于那个课程 | C语言程序设计II |
这个作业要求在哪里 | https://edu.cnblogs.com/campus/zswxy/computer-scienceclass3-2018/homework/3237 |
我在这个课程的目标是 | 熟练运用指针函数 |
这个作业在那个具体方面帮助我实现目标 | 学好递归函数和指针函数 |
参考文献 | C语言程序设计II |
6-1 计算最长的字符串长度 (15 分)
本题要求实现一个函数,用于计算有n个元素的指针数组s中最长的字符串的长度。
函数接口定义:
int max_len( char *s[], int n );
其中n
个字符串存储在s[]
中,函数max_len
应返回其中最长字符串的长度。
裁判测试程序样例:
#include <stdio.h> #include <string.h> #include <stdlib.h> #define MAXN 10 #define MAXS 20 int max_len( char *s[], int n ); int main() { int i, n; char *string[MAXN] = {NULL}; scanf("%d", &n); for(i = 0; i < n; i++) { string[i] = (char *)malloc(sizeof(char)*MAXS); scanf("%s", string[i]); } printf("%d ", max_len(string, n)); return 0; } /* 你的代码将被嵌在这里 */
输入样例:
4 blue yellow red green
输出样例:
6
1,实验代码:
int max_len( char *s[], int n ) { int i=0,max=0; for(i=0;i<n;i++){ if(strlen(s[max])<strlen(s[i])){ max=i; } } return strlen(s[max]); }
2,流程图:
3,错误截图:
错误问题:第7行“i”与“max”反了
4,结果截图:
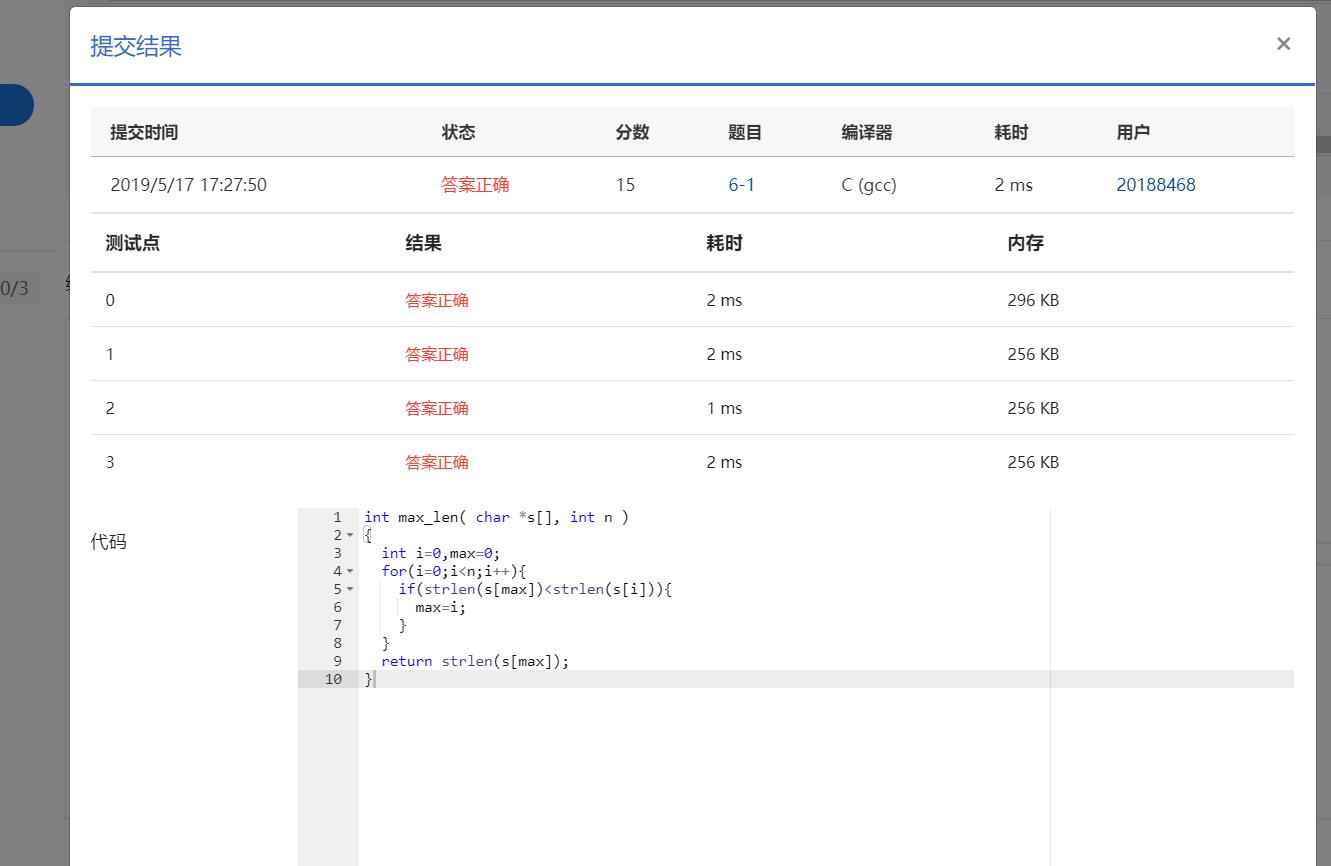
6-2 统计专业人数 (15 分)
本题要求实现一个函数,统计学生学号链表中专业为计算机的学生人数。链表结点定义如下:
struct ListNode { char code[8]; struct ListNode *next; };
这里学生的学号共7位数字,其中第2、3位是专业编号。计算机专业的编号为02。
函数接口定义:
int countcs( struct ListNode *head );
其中head
是用户传入的学生学号链表的头指针;函数countcs
统计并返回head
链表中专业为计算机的学生人数。
裁判测试程序样例:
#include <stdio.h> #include <stdlib.h> #include <string.h> struct ListNode { char code[8]; struct ListNode *next; }; struct ListNode *createlist(); /*裁判实现,细节不表*/ int countcs( struct ListNode *head ); int main() { struct ListNode *head; head = createlist(); printf("%d ", countcs(head)); return 0; } /* 你的代码将被嵌在这里 */
输入样例:
1021202 2022310 8102134 1030912 3110203 4021205 #
输出样例:
3
1,实验代码:
int countcs( struct ListNode *head ) { int sum=0; while(head!=NULL){ if(head->code[1]=='0'&&head->code[2]=='2') sum++; head=head->next; } return sum; }
2,流程图:
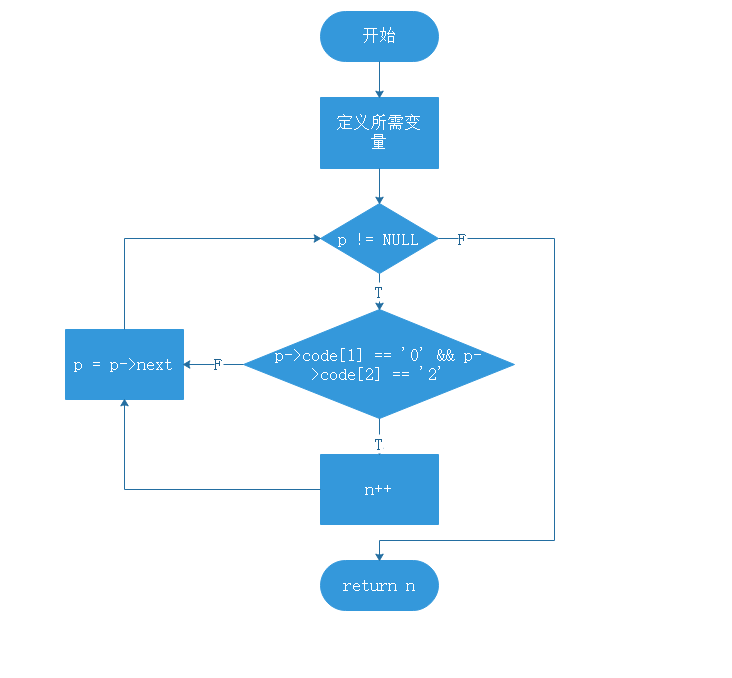
3,错误截图:
错误问题:第五行中的“1”写错了
改正方法:“1”改为“0”
4,结果截图:
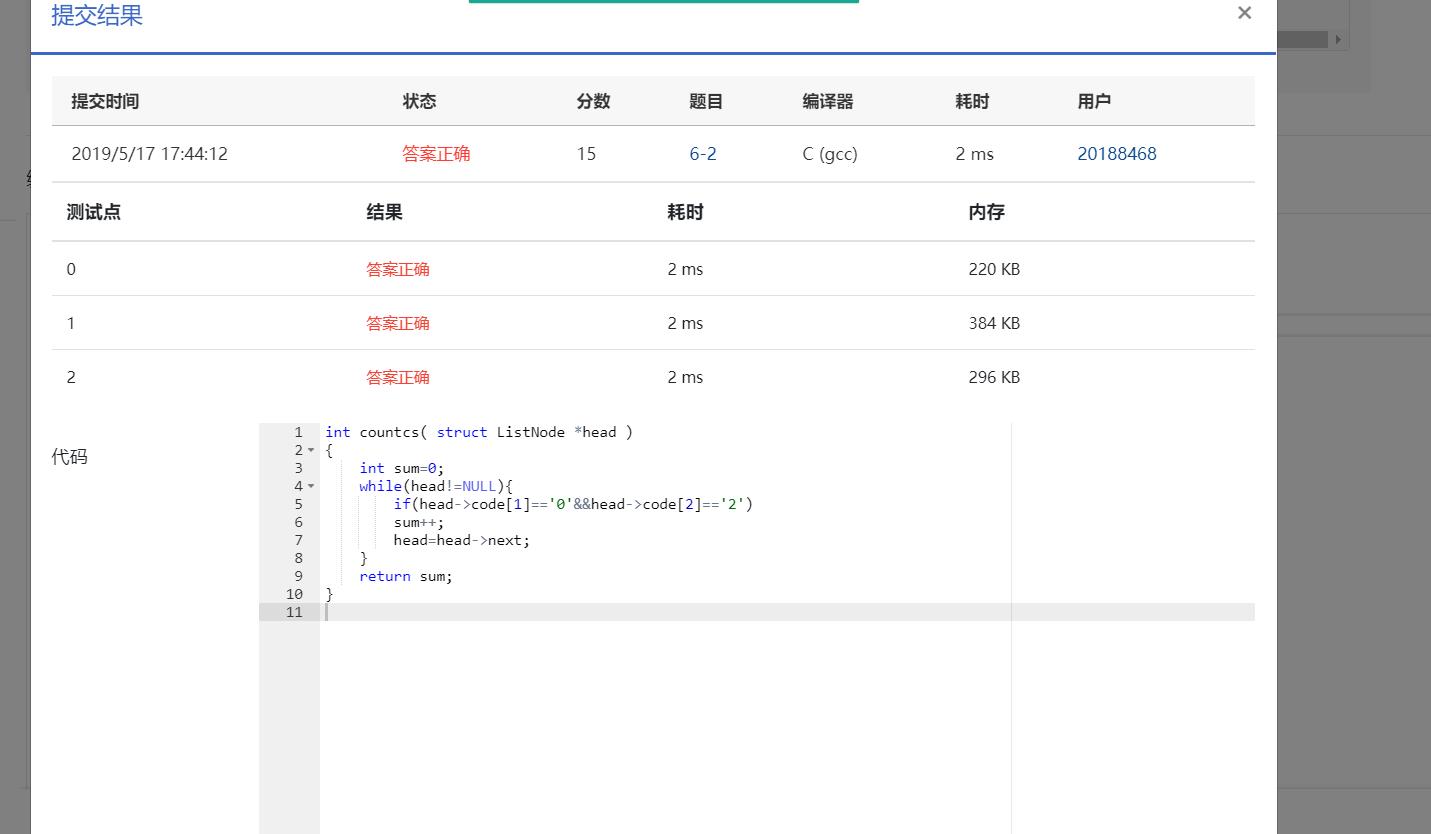
6-3 删除单链表偶数节点 (20 分)
本题要求实现两个函数,分别将读入的数据存储为单链表、将链表中偶数值的结点删除。链表结点定义如下:
struct ListNode { int data; struct ListNode *next; };
函数接口定义:
struct ListNode *createlist(); struct ListNode *deleteeven( struct ListNode *head );
函数createlist
从标准输入读入一系列正整数,按照读入顺序建立单链表。当读到−时表示输入结束,函数应返回指向单链表头结点的指针。
函数deleteeven
将单链表head
中偶数值的结点删除,返回结果链表的头指针。
裁判测试程序样例:
#include <stdio.h> #include <stdlib.h> struct ListNode { int data; struct ListNode *next; }; struct ListNode *createlist(); struct ListNode *deleteeven( struct ListNode *head ); void printlist( struct ListNode *head ) { struct ListNode *p = head; while (p) { printf("%d ", p->data); p = p->next; } printf(" "); } int main() { struct ListNode *head; head = createlist(); head = deleteeven(head); printlist(head); return 0; } /* 你的代码将被嵌在这里 */
输入样例:
1 2 2 3 4 5 6 7 -1
输出样例:
1 3 5 7
1,实验代码:
struct ListNode *createlist(){ struct ListNode * p ,*head=NULL,*tail; int size = sizeof (struct ListNode); int n,z; scanf("%d",&n); for (z=0;n!=-1;z++) { p=(struct ListNode *) malloc(size); p->data = n; if(head==NULL){ head=p; tail=p; } else { tail->next = p; } tail = p; scanf("%d",&n); } p->next=NULL; return head; } struct ListNode *deleteeven( struct ListNode *head ) { struct ListNode *p,*p1,*p2; while(head && head->data %2 ==0){ p1=head; head = head->next; free(p1); } p1=head; while(p1 && p1 ->next !=NULL) { while(p1->next && p1->next->data%2==0) { p2=p1->next; p1->next=p2->next; } p1=p1->next; } return head; }
2,流程图:
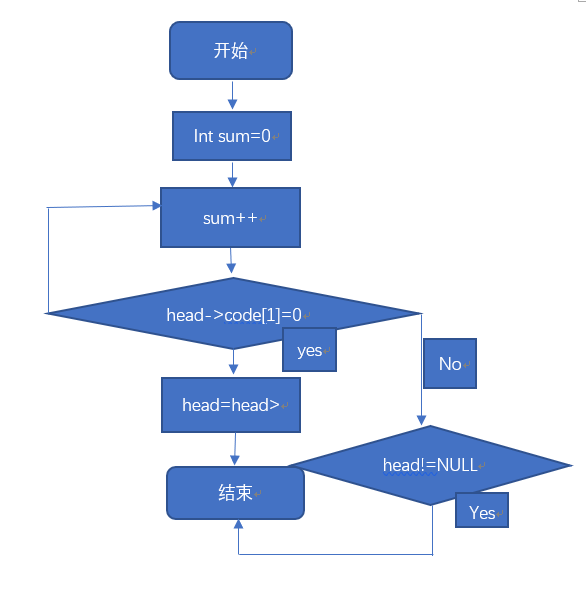
3,错误截图:
错误原因:第41行p1写成了p2
改正方法:
p1=p2->next改为p1=p1->next;
4,结果截图:
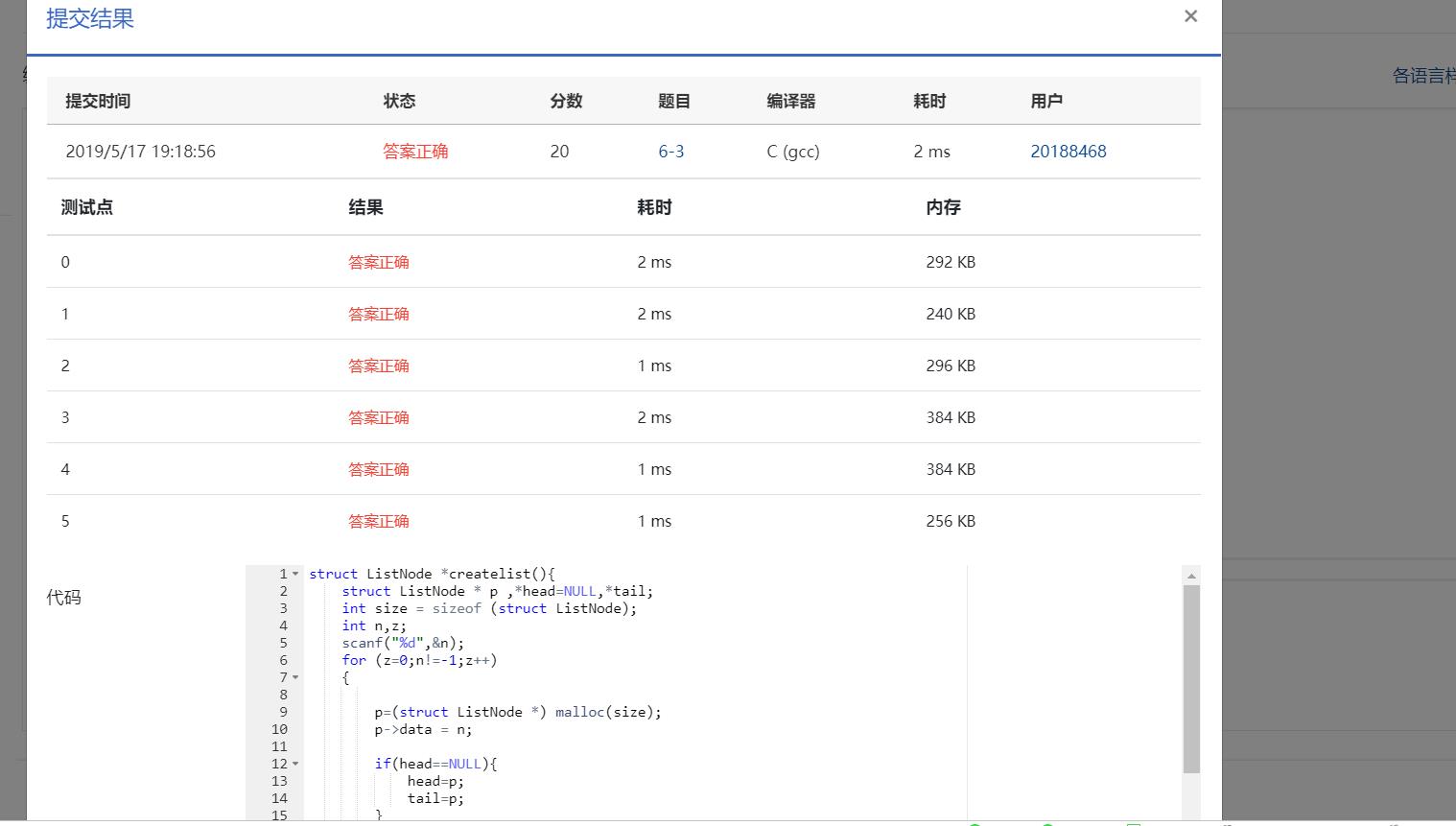
思想感悟:
本周作业题目好多,但是前面几题的难度还比较简单,有的题我们已经涉及过了,感觉我们要学的结构指针,指针函数,二次指针挺有意思的,挺期待的。不过我对挑战题疑问很大(弱小可怜又无助QAQ)。
---恢复内容结束---