1.什么是Mybatis
<?xml version="1.0" encoding="utf-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!--引入外部配置文件,如数据库连接的属性文件--> <properties resource="jdbc.properties"/> <!--为实体类配置别名--> <typeAliases> <typeAlias type="com.mybatisdemo.pojo.User" alias="user"/> <typeAlias type="com.mybatisdemo.pojo.Role" alias="role"/> </typeAliases> <!--数据库的描述信息--> <environments default="development"> <environment id="development"> <transactionManager type="JDBC"></transactionManager> <!--配置数据库,POOLED表示这里使用了连接池--> <dataSource type="POOLED"> <property name="driver" value="${jdbc.driver}"/> <property name="url" value="${jdbc.url}"/> <property name="username" value="${jdbc.username}"/> <property name="password" value="${jdbc.password}"/> </dataSource> </environment> </environments> <!--引入映射器--> <mappers> <mapper resource="mappings/UserMapper.xml"/> <mapper resource="mappings/RoleMapper.xml" /> </mappers> </configuration>
生成SqlSessionFactory
// 生成SqlSessionFactory实例,采用单例模式 public class SqlSessionFactoryUtil { private static SqlSessionFactory sqlSessionFactory = null; private static String resource = "mybatis-config.xml"; private SqlSessionFactoryUtil(){ } public static SqlSessionFactory getSqlSessionFactory(){ if(sqlSessionFactory == null){ try { sqlSessionFactory = new SqlSessionFactoryBuilder().build(Resources.getResourceAsStream(resource)); } catch (IOException e) { e.printStackTrace(); } } return sqlSessionFactory; } }
将配置文件mybatis-config.xml文件用流的方式读取,然后通过SqlSessionFactoryBuilder的builder方法去生成SqlSessionFactory就可以了
5.Mybatis 获取SqlSession
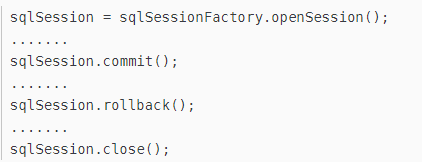
注意:sqlSession在使用完之后要及时关闭,以免浪费连接资源
6.Mybatis 映射
1)xml方式:
映射器接口:
public interface UserMapper { // 根据id查找用户 User getUserById(long id); // 根据用户名查找用户 List<User> getUserByName(String userName); }
创建映射器:
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.mybatisdemo.mapper.UserMapper"> <resultMap id="userMap" type="user"> <id property="userId" column="user_id"/> <result property="userName" column="user_name"/> <result property="password" column="password"/> <result property="realName" column="real_name"/> <result property="sex" column="sex" typeHandler="org.apache.ibatis.type.EnumOrdinalTypeHandler"/> <result property="userImg" column="user_img" typeHandler="org.apache.ibatis.type.BlobInputStreamTypeHandler"/> <result property="roleId" column="role_id"/> </resultMap> <select id="getUserById" parameterType="long" resultMap="userMap"> select * from t_user where user_id=#{id} </select> <select id="getUserByName" parameterType="string" resultMap="userMap"> select * from t_user where user_name like concat('%',#{userName},'%') </select> </mapper>
SqlSession获取映射器:
public void test(){ SqlSession sqlSession = SqlSessionFactoryUtil.getSqlSession(); UserMapper userMapper = sqlSession.getMapper(UserMapper.class); User user = userMapper.getUserById(1L); sqlSession.close(); }
2)注解形式:
public interface UserMapper { // 根据id查找用户 @Select("select * from t_user where user_id=#{id}") User getUserById(long id); }
7.Mybatis Sql语句中 #{} ${}
#{}是预编译处理 #在底层会转换为 jdbc 中使用 PreparedStatement 时?预编译
${}是字符串替换 $转换为 Statement 的字符串拼接
SELECT * FROM t_usr WHERE name like '%${name}%'
SELECT * FROM t_usr WHERE name like CONCAT('%',#{name},'%'))
9.Mybatis 缓存技术
在没有配置的默认情况下,它只支持一级缓存(一级缓存只相对于同一个 SqlSession 而言)。
1)一级缓存:使用了 HashMap 的存储方式,key 为 hashCode+sqlid+SQL 语句,value 为从查询出来映射的 java 对象。每次使用同一个 SqlSession 发起请求时,检查 sql 语句是否已经存在,如果存在则从缓存中返回映射的 java 对象,所以多次查询同一条 sql语句避免访问多次数据库;在BaseExecutor中实现
2)二级缓存:SqlSessionFactory 的缓存,默认不开启。如果要开启需要在 xxx.mapper.xml 中配置。mybatis 要求返回的 POJO 对象必须是可序列化的(实现 Serializable 接口);在CachingExecutor中实现
注意: eviction:缓存回收策略
10.Mybatis 映射文件的常用标签
11.Mybatis 获取自动生成的主键(id)
<insert id=”insertname” usegeneratedkeys=”true” keyproperty=”id”> insert into names (name) values (#{name}) </insert>
12.Mybatis 映射文件中namespace标签
Actor selectActorById(Long id);
<select id="selectActorById" resultType="canger.study.chapter04.bean.Actor"> select actor_id as id, first_name as firstName ,last_name as lastName from acto where actor_id=#{abc} </select>
Actor actor = mapper.selectActorById(1L)
2)@Param
Boolean insertActorByParamString(@Param("firstName")String var1, @Param("lastName")String var2);
<insert id="insertActorByParamString"> insert into actor(first_name,last_name) values (#{firstName},#{lastName}) </insert>
Boolean result = mapper.insertActorByParamString("James", "Harden");
System.out.println(result)
14.Mybatis 多表操作
1)一对多
2)多对一
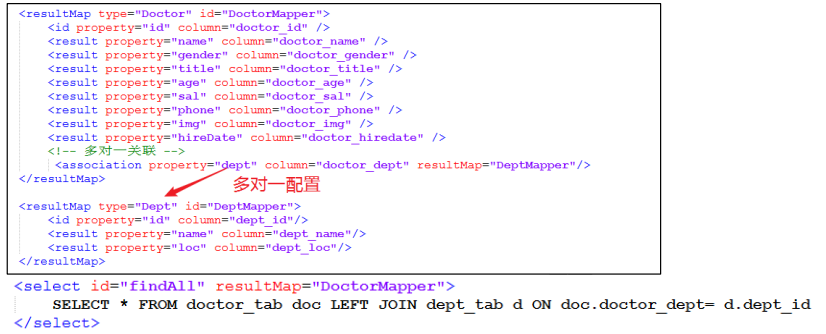
14.Mybatis 是否支持延迟加载
15.Mybatis 执行器(executor)