Crashing Robots
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 8124 | Accepted: 3528 |
Description
In a modernized warehouse, robots are used to fetch the goods. Careful planning is needed to ensure that the robots reach their destinations without crashing into each other. Of course, all warehouses are rectangular, and all robots occupy a circular floor space with a diameter of 1 meter. Assume there are N robots, numbered from 1 through N. You will get to know the position and orientation of each robot, and all the instructions, which are carefully (and mindlessly) followed by the robots. Instructions are processed in the order they come. No two robots move simultaneously; a robot always completes its move before the next one starts moving. A robot crashes with a wall if it attempts to move outside the area of the warehouse, and two robots crash with each other if they ever try to occupy the same spot.
Input
The first line of input is K, the number of test cases. Each test case starts with one line consisting of two integers, 1 <= A, B <= 100, giving the size of the warehouse in meters. A is the length in the EW-direction, and B in the NS-direction. The second line contains two integers, 1 <= N, M <= 100, denoting the numbers of robots and instructions respectively. Then follow N lines with two integers, 1 <= Xi <= A, 1 <= Yi <= B and one letter (N, S, E or W), giving the starting position and direction of each robot, in order from 1 through N. No two robots start at the same position.
Figure 1: The starting positions of the robots in the sample warehouse Finally there are M lines, giving the instructions in sequential order. An instruction has the following format: < robot #> < action> < repeat> Where is one of
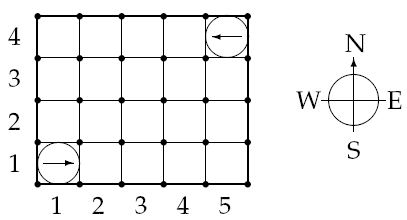
- L: turn left 90 degrees,
- R: turn right 90 degrees, or
- F: move forward one meter,
Output
Output one line for each test case:
- Robot i crashes into the wall, if robot i crashes into a wall. (A robot crashes into a wall if Xi = 0, Xi = A + 1, Yi = 0 or Yi = B + 1.)
- Robot i crashes into robot j, if robots i and j crash, and i is the moving robot.
- OK, if no crashing occurs.
Sample Input
4 5 4 2 2 1 1 E 5 4 W 1 F 7 2 F 7 5 4 2 4 1 1 E 5 4 W 1 F 3 2 F 1 1 L 1 1 F 3 5 4 2 2 1 1 E 5 4 W 1 L 96 1 F 2 5 4 2 3 1 1 E 5 4 W 1 F 4 1 L 1 1 F 20
Sample Output
Robot 1 crashes into the wall Robot 1 crashes into robot 2 OK Robot 1 crashes into robot 2
Source

1 #include<stdio.h> 2 #include<iostream> 3 #include<math.h> 4 #include<string.h> 5 using namespace std; 6 struct robot 7 { 8 int x , y ; 9 int dir ; 10 }a[200]; 11 12 struct instruction 13 { 14 int num ; 15 char act ; 16 int rep ; 17 }ins[200]; 18 19 int EW , NS ; 20 int n , m ; 21 bool flag ; 22 int map[150][150]; 23 24 void crash (int No) 25 { 26 int k = ins[No].rep ; 27 int t = ins[No].num ; 28 map[a[t].x][a[t].y] = 0 ; 29 switch (a[t].dir) 30 { 31 case 0 : 32 for (int i = 0 ; i < k && a[t].x && a[t].y ; i++) 33 if (map[a[t].x][++a[t].y]) { 34 flag = 1 ; 35 printf ("Robot %d crashes into robot %d " , t , map[a[t].x][a[t].y]) ; 36 break ; 37 } 38 break ; 39 case 1 : 40 for (int i = 0 ; i < k && a[t].x && a[t].y ; i++) 41 if (map[++a[t].x][a[t].y]) { 42 flag = 1 ; 43 printf ("Robot %d crashes into robot %d " , t , map[a[t].x][a[t].y]) ; 44 break ; 45 } 46 break ; 47 case 2 : 48 for (int i = 0 ; i < k && a[t].x && a[t].y ; i++) 49 if (map[a[t].x][--a[t].y]) { 50 flag = 1 ; 51 printf ("Robot %d crashes into robot %d " , t , map[a[t].x][a[t].y]) ; 52 break ; 53 } 54 break ; 55 case 3 : 56 for (int i = 0 ; i < k && a[t].x && a[t].y; i++) 57 if (map[--a[t].x][a[t].y]) { 58 flag = 1 ; 59 printf ("Robot %d crashes into robot %d " , t , map[a[t].x][a[t].y]) ; 60 break ; 61 } 62 break ; 63 } 64 map[a[t].x][a[t].y] = t ; 65 if (a[t].x == 0 || a[t].x >= EW + 1 || a[t].y == 0 || a[t].y >= NS + 1) { 66 printf ("Robot %d crashes into the wall " , t) ; 67 flag = 1 ; 68 } 69 } 70 void solve (int No) 71 { 72 int k = ins[No].num ; 73 switch (ins[No].act) 74 { 75 case 'L' : a[k].dir -= ins[No].rep ; a[k].dir %= 4 ; a[k].dir += 4 ; a[k].dir %= 4 ; break ; 76 case 'R' : a[k].dir += ins[No].rep ; a[k].dir %= 4 ; a[k].dir += 4 ; a[k].dir %= 4 ; break ; 77 case 'F' : crash (No) ; 78 } 79 } 80 int main () 81 { 82 // freopen ("a.txt" , "r" , stdin) ; 83 int T ; 84 scanf ("%d" , &T) ; 85 char temp ; 86 while (T--) { 87 memset (map , 0 , sizeof(map) ) ; 88 scanf ("%d%d" , &EW , &NS) ; 89 scanf ("%d%d" , &n , &m) ; 90 for (int i = 1 ; i <= n ; i++) { 91 cin >> a[i].x >> a[i].y >> temp ; 92 // cout << temp <<endl ; 93 map[a[i].x][a[i].y] = i ; 94 switch (temp) 95 { 96 case 'E' : a[i].dir = 1 ; break ; 97 case 'S' : a[i].dir = 2 ; break ; 98 case 'W' : a[i].dir = 3 ; break ; 99 case 'N' : a[i].dir = 0 ; break ; 100 } 101 // printf ("a[%d].dir=%d " , i , a[i].dir) ; 102 } 103 for (int i = 0 ; i < m ; i++) 104 cin >> ins[i].num >> ins[i].act >> ins[i].rep ; 105 106 flag = 0 ; 107 // printf ("a[1].dir=%d " , a[1].dir) ; 108 for (int i = 0 ; i < m ; i++) { 109 /* for (int i = 1 ; i <= EW ; i++) { 110 for (int j = 1 ; j <= NS ; j++) { 111 printf ("%d " , map[i][j]) ; 112 } 113 puts(""); 114 } 115 puts ("") ;*/ 116 solve (i) ; 117 /* for (int i = 1 ; i <= EW ; i++) { 118 for (int j = 1 ; j <= NS ; j++) { 119 printf ("%d " , map[i][j]) ; 120 } 121 puts(""); 122 } 123 printf (" ") ; */ 124 if (flag) 125 break ; 126 } 127 128 if (!flag) 129 puts ("OK") ; 130 } 131 return 0 ; 132 }
规规矩矩得模拟 robot 一步步走就行了