Best Time to Buy and Sell Stock II
题目等级:Easy
题目描述:
Say you have an array for which the ith element is the price of a given stock on day i.
Design an algorithm to find the maximum profit. You may complete as many transactions as you like (i.e., buy one and sell one share of the stock multiple times).
Note: You may not engage in multiple transactions at the same time (i.e., you must sell the stock before you buy again).
Example 1:
Input: [7,1,5,3,6,4]
Output: 7
Explanation: Buy on day 2 (price = 1) and sell on day 3 (price = 5), profit = 5-1 = 4.
Then buy on day 4 (price = 3) and sell on day 5 (price = 6), profit = 6-3 = 3.
Example 2:
Input: [1,2,3,4,5]
Output: 4
Explanation: Buy on day 1 (price = 1) and sell on day 5 (price = 5), profit = 5-1 = 4.
Note that you cannot buy on day 1, buy on day 2 and sell them later, as you are
engaging multiple transactions at the same time. You must sell before buying again.
Example 3:
Input: [7,6,4,3,1]
Output: 0
Explanation: In this case, no transaction is done, i.e. max profit = 0.
题意:给定一个数组,它的第 i 个元素是一支给定股票第 i 天的价格。设计一个算法来计算所能获取的最大利润。你可以尽可能地完成更多的交易(多次买卖一支股票),必须在再次购买前出售掉之前的股票。
解题思路(贪心策略):
本题和上一题相比,唯一的区别是:这里对买卖次数没有限制。
这里我们可以采用贪心策略来解决:首先我们需要理解一点,第i天买入,第j天卖出得到的收益和第i天买入,第i+p天卖出,第i+p天再买入,第j天卖出得到的收益是相同的。比如[1,2,3,4,5],很明显我们知道最大收益是4,可以看作是第一天买入,第五天卖出,但是也可以看作是第1天买入,第二天卖出,同时买入,第三天又卖出,同时买入······
理解了这一点,我们就清楚这里为什么能用贪心的思想了,从第一天开始买入,只要有收益就可以直接卖出,接下来再买入,同样一旦有收益就可以卖出,这是一种典型的贪心思想,局部最优达到全局最优。
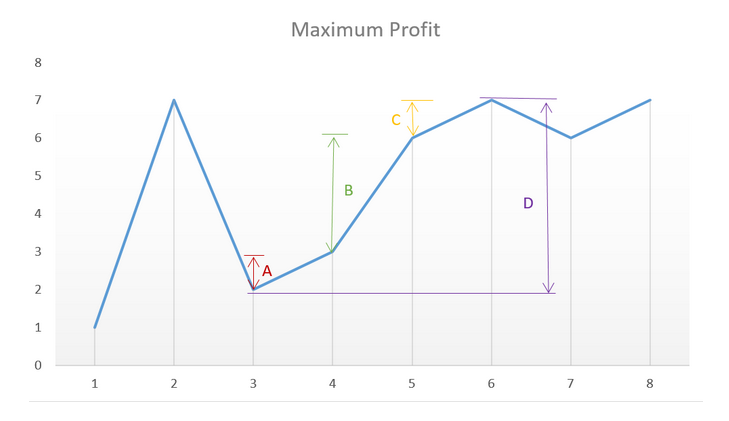
从代码角度来说,我们只需要累加后一天和前一天的差(后一天大于前一天的情况下)即可。
public int maxProfit(int[] prices) {
//贪心法
if(prices==null || prices.length==0)
return 0;
int profit=0;
for(int i=1;i<prices.length;i++){
if(prices[i]>prices[i-1])
profit+=(prices[i]-prices[i-1]);
}
return profit;
}
可以看出来,代码实现很简洁,时间复杂度:O(n),空间复杂度O(1)。