Problem Description
Astronomers often examine star maps where stars are represented by points on a plane and each star has Cartesian coordinates. Let the level of a star be an amount of the stars that are not higher and not to the right of the given star. Astronomers want to know
the distribution of the levels of the stars.
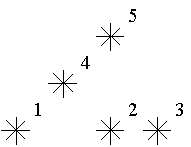
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level 0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
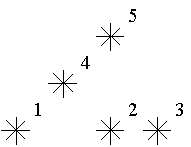
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level 0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
Input
The first line of the input file contains a number of stars N (1<=N<=15000). The following N lines describe coordinates of stars (two integers X and Y per line separated by a space, 0<=X,Y<=32000). There can be only one star at one point of the plane. Stars
are listed in ascending order of Y coordinate. Stars with equal Y coordinates are listed in ascending order of X coordinate.
Output
The output should contain N lines, one number per line. The first line contains amount of stars of the level 0, the second does amount of stars of the level 1 and so on, the last line contains amount of stars of the level N-1.
Sample Input
5
1 1
5 1
7 1
3 3
5 5
Sample Output
1
2
1
10
这题是简单的线段树应用,好像也可以用树状数组做,以后学了再做一次(笑)。因为题目中的坐标是先按纵坐标大小,再按横坐标大小排列的,所以一个左边的值就是比它横坐标小的值再加上它下面的坐标。可以用线段树维护区间的小于等于区间右端点的横坐标的个数,这里查询和更新放在了一起,从根节点到叶子节点是查询,再从叶子节点递归上来是询问。
#include<iostream>
#include<stdio.h>
#include<string.h>
#include<math.h>
#include<vector>
#include<map>
#include<queue>
#include<stack>
#include<string>
#include<algorithm>
using namespace std;
#define maxn 32005
int num,vis[15005];
struct node{
int l,r,num;
}b[8*maxn];
void build(int l,int r,int i)
{
int mid;
b[i].l=l;b[i].r=r;b[i].num=0;
if(l==r)return;
mid=(l+r)/2;
build(l,mid,i*2);
build(mid+1,r,i*2+1);
}
void update(int id,int i)
{
int mid;
if(b[i].l==b[i].r){
num+=b[i].num;
b[i].num++;return;
}
if(b[i*2].r>=id){
update(id,i*2);
}
else{
num+=b[i*2].num;
update(id,i*2+1);
}
b[i].num=b[i*2].num+b[i*2+1].num;
}
int main()
{
int n,m,i,j,c,d;
while(scanf("%d",&n)!=EOF)
{
memset(vis,0,sizeof(vis));
build(0,32005,1);
for(i=1;i<=n;i++){
scanf("%d%d",&c,&d);
num=0;
update(c,1);
vis[num]++;
}
for(i=0;i<=n-1;i++){
printf("%d
",vis[i]);
}
}
return 0;
}