Going Home
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 26212 | Accepted: 13136 |
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=4289
Description:
On a grid map there are n little men and n houses. In each unit time, every little man can move one unit step, either horizontally, or vertically, to an adjacent point. For each little man, you need to pay a $1 travel fee for every step he moves, until he enters a house. The task is complicated with the restriction that each house can accommodate only one little man.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates there is a little man on that point.
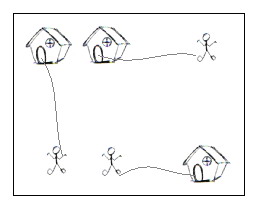
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Input:
There are one or more test cases in the input. Each case starts with a line giving two integers N and M, where N is the number of rows of the map, and M is the number of columns. The rest of the input will be N lines describing the map. You may assume both N and M are between 2 and 100, inclusive. There will be the same number of 'H's and 'm's on the map; and there will be at most 100 houses. Input will terminate with 0 0 for N and M.
Output:
For each test case, output one line with the single integer, which is the minimum amount, in dollars, you need to pay.
Sample Input:
2 2 .m H. 5 5 HH..m ..... ..... ..... mm..H 7 8 ...H.... ...H.... ...H.... mmmHmmmm ...H.... ...H.... ...H.... 0 0
Sample Output:
2 10 28
题意:
给出一个n*m的图,上面有数量相等的人和房子,同时有些空地,每个人每走一步都要给其1的金币,问当最后所有人都到房子时最小给出的金币是多少。
题解:
我一开始以为会BFS求每个人到房子的最短路径,后来发现没有障碍,直接人到房子的曼哈顿距离即是最短路径。这也就是每个人到相应房子应给的金钱。
因为一个房子只能容纳下一个人,考虑边权为1的最大流,然后跑个最小费用最大流就行了。
这里将每个人与所有房子连边,费用为其到房子的最短距离,边容量都为1。
代码如下:
#include <cstdio> #include <cstring> #include <algorithm> #include <iostream> #include <vector> #include <cmath> #include <queue> using namespace std; const int N = 105 ,INF = 99999999,t = 505; int n,m,cnt,tot,num; int map[N][N],d[N][N],head[N*N],dis[N*N],vis[N*N],a[N*N],p[N*N],pre[N*N]; int Dis(int x1,int y1,int x2,int y2){ return abs(x1-x2)+abs(y1-y2); } struct Edge{ int u,v,next,c,w; }e[N*N*N]; void adde(int u,int v,int w){ e[tot].v=v;e[tot].c=1;e[tot].w=w;e[tot].next=head[u];head[u]=tot++; e[tot].v=u;e[tot].c=0;e[tot].w=-w;e[tot].next=head[v];head[v]=tot++; } int BellmanFord(int s,int t,int &flow,int &cost){ for(int i=0;i<=t+5;i++) dis[i]=INF,a[i]=INF; queue <int> q;memset(vis,0,sizeof(vis));memset(p,-1,sizeof(p)); memset(pre,-1,sizeof(pre)); q.push(s);vis[s]=1;dis[s]=0;p[s]=0; while(!q.empty()){ int u=q.front();q.pop();vis[u]=0; for(int i=head[u];i!=-1;i=e[i].next){ int v=e[i].v; if(e[i].c>0 &&dis[v]>dis[u]+e[i].w){ dis[v]=dis[u]+e[i].w; a[v]=min(a[u],e[i].c); p[v]=u; pre[v]=i; if(!vis[v]){ vis[v]=1; q.push(v); } } } } if(dis[t]==INF) return 0; flow+=a[t]; cost+=a[t]*dis[t]; for(int i=t;i>0;i=p[i]){// e[pre[i]].c-=a[t]; e[pre[i]^1].c+=a[t]; } return 1; } int Min_cost(){ int flow=0,cost=0; while(BellmanFord(0,t,flow,cost)); return cost; } int main(){ while(~scanf("%d%d",&n,&m)){ if(n==0 && m==0) break; tot=0;num=0;cnt=0; memset(head,-1,sizeof(head)); vector <pair<int,int> > h; memset(map,0,sizeof(map)); for(int i=1;i<=n;i++){ char s[N]; scanf("%s",s); for(int j=0;j<m;j++){ if(s[j]=='H'){ map[i][j+1]=2; h.push_back(make_pair(i,j+1)); } if(s[j]=='m') map[i][j+1]=1,num++; } } for(int i=1;i<=num;i++) adde(0,i,0); for(int i=num+1;i<=2*num;i++) adde(i,t,0); for(int i=1;i<=n;i++){ for(int j=1;j<=m;j++){ if(map[i][j]!=1) continue ; cnt++;int tmp=0; for(int k=0;k<h.size();k++){ tmp++; pair <int,int> v = h[k]; adde(cnt,num+tmp,Dis(i,j,v.first,v.second)); } } } printf("%d ",Min_cost()); } return 0; }