一:获取二维数组的行数与列数,截图
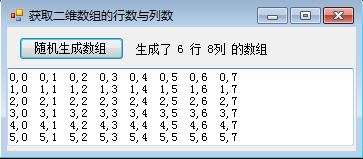
二:代码
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace ArrayRank
{
public partial class Frm_Main : Form
{
public Frm_Main()
{
InitializeComponent();
}
private string[,] G_str_array;//定义数组类型变量
private Random G_Random_Num = new Random();//生成随机数对象
private void btn_GetArray_Click(object sender, EventArgs e)
{
/*参数
minValue
类型:System.Int32
返回的随机数的下界(随机数可取该下界值)。
maxValue
类型:System.Int32
返回的随机数的上界(随机数不能取该上界值)。 maxValue 必须大于或等于 minValue。
返回值
类型:System.Int32
一个大于等于 minValue 且小于 maxValue 的 32 位带符号整数,即:返回的值范围包括 minValue 但不包括 maxValue。
如果 minValue 等于 maxValue,则返回 minValue。
*/
txt_display.Clear();//清空控件中的字符串
G_str_array = new string[//随机生成二维数组
G_Random_Num.Next(2, 10),
G_Random_Num.Next(2, 10)];
lab_Message.Text = string.Format(
"生成了 {0} 行 {1 }列 的数组",
/*GetUpperBound参数
dimension
类型:System.Int32
数组的从零开始的维度,其上限需要确定。
返回值
类型:System.Int32
数组中指定维度最后一个元素的索引,或 -1(如果指定维度为空)。
*/
G_str_array.GetUpperBound(0) + 1,//GetUpperBound(0) 返回 Array 的第一维的索引上限,GetUpperBound(Rank - 1) 返回 Array 的最后一维的上限。获取数组的行数
G_str_array.GetUpperBound(1) + 1);//获取数组的列数
DisplayArray();//调用显示数组方法
}
private void DisplayArray()
{
//使用循环赋值
for (int i = 0; i < G_str_array.GetUpperBound(0) + 1; i++)
{
for (int j = 0; j < G_str_array.GetUpperBound(1) + 1; j++)
{
G_str_array[i, j] = i.ToString() + "," + j.ToString() + " ";
}
}
//使用循环输出
for (int i = 0; i < G_str_array.GetUpperBound(0) + 1; i++)
{
for (int j = 0; j < G_str_array.GetUpperBound(1) + 1; j++)
{
txt_display.Text += G_str_array[i, j];
}
txt_display.Text += Environment.NewLine;
}
}
}
}
三:按指定条件在数组中检索元素,截图
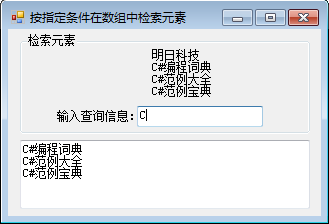
四:代码
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace FindStr
{
public partial class Frm_Main : Form
{
public Frm_Main()
{
InitializeComponent();
}
private string[] G_str_array;//定义字符串数组字段
private void Frm_Main_Load(object sender, EventArgs e)
{
G_str_array = new string[] {//为字符串数组字段赋值
"明日科技","C#编程词典","C#范例大全","C#范例宝典"};
for (int i = 0; i < G_str_array.Length; i++)//循环输出字符串
{
lab_Message.Text += G_str_array[i] + "
";
}
}
private void txt_find_TextChanged(object sender, EventArgs e)
{
if (txt_find.Text != string.Empty)//判断查找字符串是否为空
{
/*Array.FindAll类型参数
T
数组元素的类型。
参数
array
类型:T[]
要搜索的从零开始的一维 Array。
match
类型:System.Predicate<T>
Predicate<T> ,定义要搜索的元素的条件。
返回值
类型:T[]
如果找到一个其中所有元素均与指定谓词定义的条件匹配的 Array,则为该数组;否则为一个空 Array。
*/
string[] P_str_temp = Array.FindAll//使用FindAll方法查找相应字符串
(G_str_array, (s) => s.Contains(txt_find.Text));//表示一个方法。()中的是这个方法的参数,后面是方法的返回值。
if (P_str_temp.Length > 0)//判断是否查找到相应字符串
{
txt_display.Clear();//清空控件中的字符串
foreach (string s in P_str_temp)//向控件中添加字符串
{
txt_display.Text += s + Environment.NewLine;
}
}
else
{
txt_display.Clear();//清空控件中的字符串
txt_display.Text = "没有找到记录";//提示没有找到记录
}
}
else
{
txt_display.Clear();//清空控件中的字符串
}
}
}
}