我们在以前学习
Spring
的时候,其所有的配置信息都写在
applicationContext.xml
里,大致示例如下:
java代码:
- <beans>
- <bean name="ds" class="org.apache.commons.dbcp.BasicDataSource">
- <property name="driverClassName" value="oracle.jdbc.driver.OracleDriver"/>
- <property name="url" value="jdbc:oracle:thin:@localhost :1521:wangbin"/>
- <property name="username" value="tech37"/>
- <property name="password" value="tech37"/>
- </bean>
- <bean name="txManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
- <property name="dataSource" ref="ds"/>
- </bean>
- <tx:advice id="txAdvice" transaction-manager="txManager">
- <tx:attributes>
- <tx:method name="get*" read-only="true"/>
- <tx:method name="*"/>
- </tx:attributes>
- </tx:advice>
- <aop:config>
- <aop:advisor advice-ref="txAdvice"
- pointcut="execution(* cn.javass..business.ebo.*Ebo.*(..))"/>
- </aop:config>
- </beans>
在上面的示例中,我们可以典型的看到Spring的三种功能:
1、IoC容器,如:
<bean …>
<property…/>
</bean>
2、AOP
<aop:config/>
3、事务
<tx:advice/>
首先我们学习如何使用注解来构造IoC容器。
用注解来向Spring容器注册Bean。需要在applicationContext.xml中注册<context:component-scan base-package=“cn.javass”/>。表明cn.javass包及其子包中,如果某个类的头上带有特定的注解【@Component/@Repository/@Service/@Controller】,就会将这个对象作为Bean注册进Spring容器。
以上的4个注解,用法完全一摸一样,只有语义上的区别。
@Component 是所有受Spring 管理组件的通用形式,Spring 还提供了更加细化的注解形式: @Repository 、@Service 、@Controller ,它们分别对应数据层Bean ,业务层Bean ,和表现层Bean 。
其中,@Component不推荐使用。
这四个注解都可以放在类的头上,如果不指定其value【@Service】,则默认的bean名字为这个类的简单类名首字母小写;如果指定value【@Service(“dao”)】,则使用value作为ban名字。
注意:如果cn.javass.SampleDao和cn.javass1.SampleDao都只用@Service注解,而不指定value会发生什么事?
除了注册Bean之外,还可以通过在<bean>上设置属性来控制Bean的其他属性,比如:
<bean name="" class=""
lazy-init=“true” //是否延迟初始化
scope=“prototype” //bean的生命周期
depends-on=“其他bean“ //依赖其他bean
/>
在Spring中也有相应的注解去对应
@Lazy
@Scope
@DependsOn
他们都是放在类的头上。
除了注册Bean之外,还可以通过在<bean>上设置属性来控制Bean的初始化方法和析构方法,比如:
<bean name="" class=""
init-method=“init“ //初始化方法
destroy-method=“close“ //析构方法
/>
在Spring中也有相应的Bean去对应,当然,这两个注解是jdk里内置的
@PostConstruct
@PreDestroy
这两个注解放在方法头上。
@Autowired 根据bean 类型从spring 上下文中进行查找。我们需要从以下几方面来学习这个注解:
1、它都能放在什么头上?
它可以放在属性、方法和构造方法头上。
2、根据什么注入?
2.1、如果某个接口的实现类在Spring容器中唯一的话,仅使用@Autowired就可以正确注入,如:
@Autowired
private SampleDao dao;
2.2、如果某个接口的实现类在Spring容器中不唯一
2.2.1、用@Qualifier来指定注入Bean的名字,如
@Autowired
@Qualifier(“sampleDaoImpl”)
private SampleDao dao;
2.2.2、用@Primary在多个Bean之中指定哪个为最优先者,注意它不是跟@Autowired配合使用,而是跟@Service配合使用,如
@Service @Primary SampleDaoImpl
2.2.3、用属性名、方法参数名、构造方法参数名来设置注入哪个 Bean,如
public class SampleDaoImpl implements SampleDao
public class SampleDaoImpl1 implements SampleDao
对应
@Autowired
private SampleDao sampleDaoImpl;
注意:属性名在编译后是一定存在的;但是方法参数名和构造方法参数名必须指定相应的编译选项才能保留。
3、@Autowired只有一个选项, boolean required() default true;是否必须,且默认为true。因此,如果仅仅使用@Autowired,就必须要能注入,否则会报错。
@Resource拥有和@Autowired类似的作用。
Spring还支持使用@Configuration,把一个类作为一个IoC容器,它的某个方法头上如果注册了@Bean,就会作为这个Spring容器中的Bean。
java代码:
- @Configuration("ctx")
- public class JavaApplicationContext {
- @Bean
- public String hello(){
- return "hello";
- }
- @Bean
- public int max(){
- return 9;
- }
- }
使用AnnotationConfigApplicationContext获得Spring容器
ApplicationContext context = new AnnotationConfigApplicationContext(JavaApplicationContext.class);
使用@ImportResource (“classpath:applicationContext.xml”)可以把其他容器导入到这个容器中。
Spring使用的AOP注解分为三个层次:
1、@Aspect放在类头上,把这个类作为一个切面,但是这个类一定要显式的注册在Spring容器中。
2、 @Pointcut放在方法头上,定义一个可被别的方法引用的切入点表达式。
3、5种通知。
3.1、@Before,前置通知,放在方法头上。
3.2、@After,后置【finally】通知,放在方法头上。
3.3、@AfterReturning,后置【try】通知,放在方法头上,使用returning来引用方法返回值。
3.4、@AfterThrowing,后置【catch】通知,放在方法头上,使用throwing来引用抛出的异常。
3.5、@Around,环绕通知,放在方法头上,这个方法要决定真实的方法是否执行,而且必须有返回值。
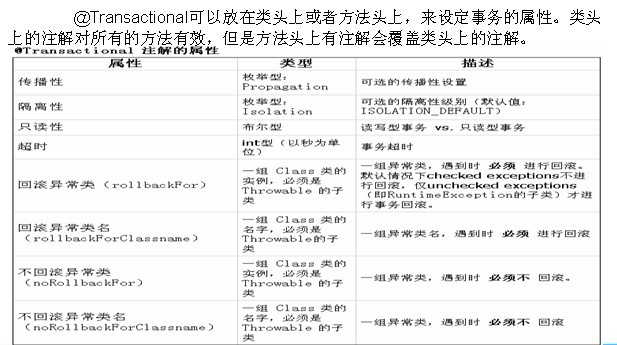