一、落单的数
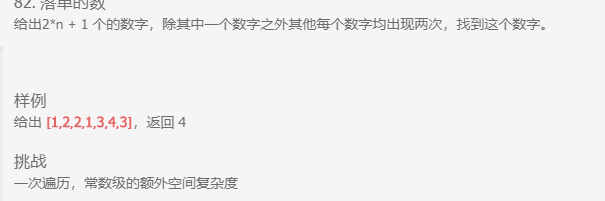
class Solution:
"""
@param A: An integer array
@return: An integer
"""
def singleNumber(self, A):
# write your code here
d= {}
for i in A:
if i in d:
d.pop(i)
else:
d[i] = None
return list(d.keys())[0]
二、 Majority Element
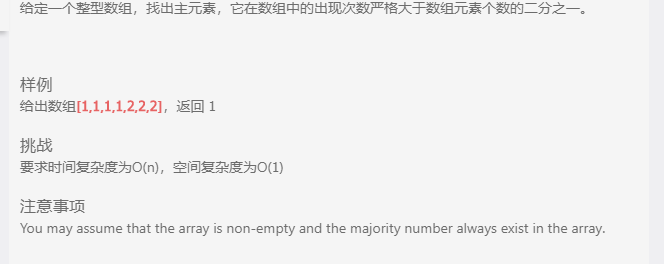
解法一:
def fun(A):
d= {}
for i in A:
if i in d:
d.pop(i)
else:
d[i] = None
return list(d.keys())[0]
解法二:
排序后,返回中间值
def fun(A):
A.sort()
return A[len(A)//2]
解法三:
思路:由于最多的数已经超过一半,若用最多的数去抵消其他的,最后剩下的就是目标值
class Solution:
"""
@param: nums: a list of integers
@return: find a majority number
"""
def majorityNumber(self, nums):
# write your code here
count = 1
result = nums[0]
for i in range(1, len(nums)):
if nums[i] == result or count == 0:
result = nums[i]
count += 1
else:
count -= 1
return result
加油站
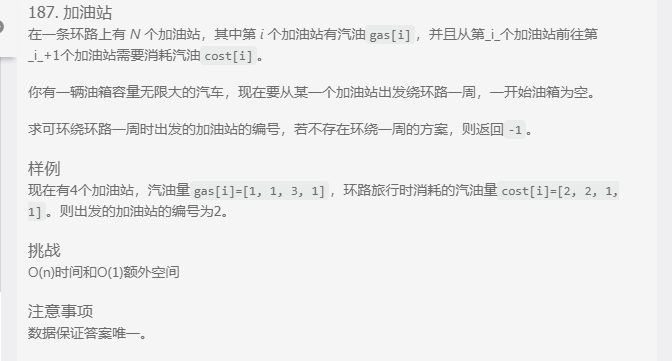
思路:其实这个题和求一个列表中最大的子列表和的思路类似,遍历列表,记录前面的累积和还有下标,当小于0就放弃当前下标,换成下一个大于0的小标。
class Solution:
"""
@param gas: An array of integers
@param cost: An array of integers
@return: An integer
"""
def canCompleteCircuit(self, gas, cost):
# write your code here
gas_account = 0
ret = -1
i = 0
for j in range( 2 * len(gas)):
if i == len(gas):
i = 0
if ret == i:
return ret
gas_account += gas[i] - cost[i]
if gas_account < 0:
gas_account = 0
ret = -1
else:
if ret == -1:
ret = i
i += 1
return -1

最大数
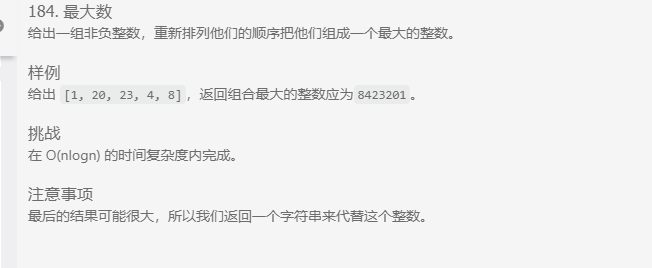
class Solution:
"""
@param nums: A list of non negative integers
@return: A string
"""
def largestNumber(self, nums):
# write your code here
from functools import cmp_to_key
res = ''.join(sorted(map(str, nums), key=cmp_to_key(self.cmp), reverse=True))
if int(res) == 0:
return '0'
return res
def cmp(self, x, y):
if x + y > y + x:
return 1
elif x + y < y + x:
return -1
else:
return 0
删除数字
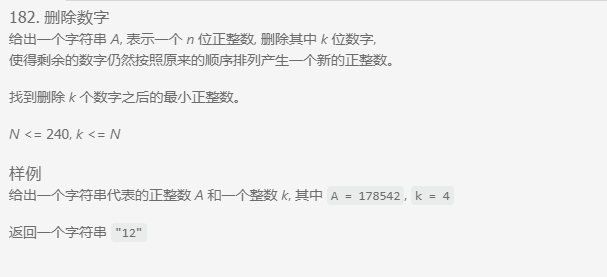
思路:当存在降序子串时,删除降序子串第一个字符,当不存在的时候,删除最后的字符。
坑:最后返回的时候不能是0开头
class Solution:
"""
@param A: A positive integer which has N digits, A is a string
@param k: Remove k digits
@return: A string
"""
def DeleteDigits(self, A, k):
A = list(A)
i = 0
while i < k:
flag = False
for j in range(len(A) - 1):
if A[j] > A[j + 1]:
del A[j]
flag = True
break
if not flag:
break
i += 1
if i == k:
ret= ''.join(A)
else:
ret=''.join(A[:(len(A)-k+i)])
return ret.lstrip('0')
跳跃游戏
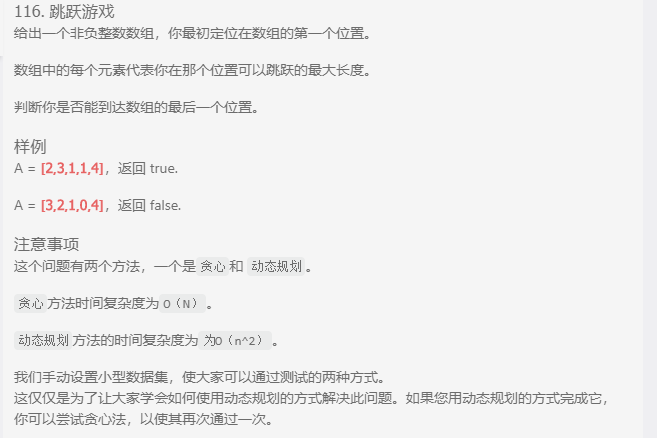
思路:先抛弃跳跃的思想,每走一步都让剩余步数最大化,也就是上判断上次剩余的步数和当前步数比,取其大者。
class Solution:
"""
@param A: A list of integers
@return: A boolean
"""
def canJump(self, A):
# write your code here
save = A[0]
for i in range(len(A)):
if save < A[i]:
save = A[i]
if save == 0 and i != len(A) - 1:
return False
save -= 1
return True
下一个排列
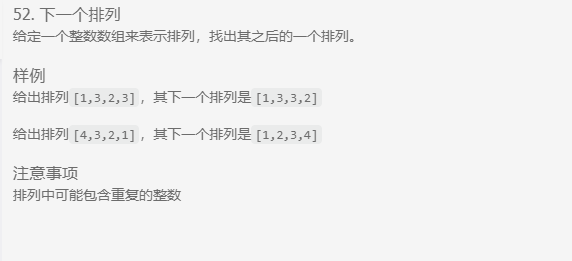
思路:从尾部向前遍历,如果有出现降序,那么从前面升序的序列中找出比出现降序的值大的最小的,然后交换位置,然后后面全部倒序
class Solution:
"""
@param nums: A list of integers
@return: A list of integers
"""
def nextPermutation(self, nums):
# write your code here
for i in range(len(nums) - 1, 0, -1):
if nums[i] > nums[i - 1]:
for j in range(len(nums) - 1, i - 1, -1):
if nums[j] > nums[i - 1]:
nums[j], nums[i - 1] = nums[i - 1], nums[j]
nums[i:] = nums[i:][::-1]
return nums
return nums[::-1]