一:微信授权
//用户授权
public function is_weixin(){
$url = "https://open.weixin.qq.com/connect/oauth2/authorize?appid=xxxxxxxxxxxxxxx&redirect_uri=http://xxx.xxxxx.com/index.php/privilege/getWeixinUser&response_type=code&scope=snsapi_userinfo&state=1#wechat_redirect";
redirect($url);
}
上面的$url 中有5个get参数,前面2个get参数的值由我们指定,即appid 和 redirect_uri
appid="微信APP_ID"
redirect_uri="回调地址"
以上例子中 is_weixin 方法执行后浏览器会访问 redirect_uri所填写的地址,也就是会执行privilege中的getWeixinUser方法
public function getWeixinUser(){
$appid = "xxxxxxxxxxxxxxxxxxxxxxx";
$secret = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx";
$code = $_GET["code"];
$get_token_url = 'https://api.weixin.qq.com/sns/oauth2/access_token?appid='.$appid.'&secret='.$secret.'&code='.$code.'&grant_type=authorization_code';
$ch = curl_init();
curl_setopt($ch,CURLOPT_URL,$get_token_url);
curl_setopt($ch,CURLOPT_HEADER,0);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1 );
curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 10);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, FALSE);
$res = curl_exec($ch);
curl_close($ch);
$json_obj = json_decode($res,true);
//根据openid和access_token查询用户信息
$access_token = $json_obj['access_token'];
$openid = $json_obj['openid'];
$get_user_info_url = 'https://api.weixin.qq.com/sns/userinfo?access_token='.$access_token.'&openid='.$openid.'&lang=zh_CN';
$ch = curl_init();
curl_setopt($ch,CURLOPT_URL,$get_user_info_url);
curl_setopt($ch,CURLOPT_HEADER,0);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1 );
curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 10);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, FALSE);
$res = curl_exec($ch);
curl_close($ch);
//解析json
$user_obj = json_decode($res,true);
// array(
// [openid] => o3ushwi5OiaBfCNA2F187BKPdnfU
// [nickname] => xxx
// [sex] => 1
// [language] => zh_CN
// [city] => 深圳
// [province] => 广东
// [country] => 中国
// [headimgurl] => http://wx.qlogo.cn/mmopen/qibdIUkiaxRnic3D9icdBOonZxI3HibH1sP1xKchqhlDOnQibVuxhfNxHVvRJCrfz9jOkR5uZxsWiaToMIQQ0spkRNfG325j8NaGO67/0
// );
}
getWeixinUser方法中最终得到的$user_obj就是一个包含了用户微信基本信息的数组。
二:QQ授权
第一步,跳转到QQ授权页面,获取(Authorization) Code值
/*QQ登陆*/
public function qqlogin(){
$appid = 'xxxxxxxxxxx';
$appkey = 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx';
$scope = "get_user_info";
//成功授权后的回调地址,需要进行urlencode
$my_url = "http://www.nightlostk.com/index.php/privilege/callback";
set_sess('state',md5(uniqid(rand(), TRUE)));
$login_url = "https://graph.qq.com/oauth2.0/authorize?response_type=code&client_id=".$appid."&redirect_uri=" .urlencode($my_url)."&state=".get_sess('state')."&scope=".$scope;
redirect($login_url);
}
代码运行后会跳转到类似如下页面:
用户点击QQ头像即表示授权并登录。页面将会跳转到 上面代码中的回调地址
//成功授权后的回调地址,需要进行urlencode
$my_url = "http://www.nightlostk.com/index.php/privilege/callback";
实际上回调地址会附带一些上get参数。 实际的地址为: http://www.nightlostk.com/index.php/privilege/callback?code=xxxxxxxxxxxx&state=xxxxxxxxxxxxxx
可以理解为第一步就是为了获取到返回的get参数 code的值
第二步, 通过Authorization Code获取Access Token->openid->用户基本信息
在第一步中,页面最后跳转到
http://www.nightlostk.com/index.php/privilege/callback?code=xxxxxxxxxxxx&state=xxxxxxxxxxxxxx
在PHP中会执行 privilege中的 callback方法
/*QQ登陆回调地址*/
public function callback(){
$appid = '101261269';
$appkey = 'adcaacfadd912ecc5991aa6936aafe0d';
$my_url = "http://m.etripbon.com/index.php/privilege/callback";
//Step2:通过Authorization Code获取Access Token
if($_REQUEST['state'] == get_sess('state')) {
$code = $_REQUEST["code"];
//拼接URL
$token_url = "https://graph.qq.com/oauth2.0/token?grant_type=authorization_code&"."client_id=".$appid."&redirect_uri=".urlencode($my_url)."&client_secret=".$appkey."&code=".$code;
$response = $this->https_request($token_url);
//Step3:使用Access Token来获取用户的OpenID
$params = array();
parse_str($response, $params);
$graph_url = "https://graph.qq.com/oauth2.0/me?access_token=".$params['access_token'];
$access_token = $params["access_token"];
$str = $this->https_request($graph_url);
$user = json_decode($str);$openid = $user->openid;
$url = "https://graph.qq.com/user/get_user_info?access_token=".$access_token."&oauth_consumer_key=".$appid."&openid=".$openid;
$output = $this->https_request($url);
$jsoninfo = json_decode($output, true);
}else{
echo("The state does not match. You may be a victim of CSRF.");
}
}
public function https_request($url,$data = null){ if(function_exists('curl_init')){ $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, FALSE); curl_setopt($curl, CURLOPT_SSLVERSION, CURL_SSLVERSION_TLSv1); if (!empty($data)){ curl_setopt($curl, CURLOPT_POST, 1); curl_setopt($curl, CURLOPT_POSTFIELDS, $data); } curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); $output = curl_exec($curl); curl_close($curl); return $output; }else{ return false; } }
在callback方法中,通过第一步获取的code值来组装好url
https://graph.qq.com/oauth2.0/token?grant_type=authorization_code&"."client_id=".$appid."&redirect_uri=".urlencode($my_url)."&client_secret=".$appkey."&code=".$code
请求这个url 获取到 Access Token
Access Token 有了以后组装出如下url
$graph_url = "https://graph.qq.com/oauth2.0/me?access_token=".$params['access_token']
请求这个url 获取到用户的 openid
openid 有了以后组装出如下url
$url = "https://graph.qq.com/user/get_user_info?access_token=".$access_token."&oauth_consumer_key=".$appid."&openid=".$openid;
请求这个url 获取到用户的基本信息,到此为止,用户的QQ基本信息就获取到了。后续则是根据自己项目的业务需求来处理这些信息即可。
返回的QQ用户基本信息如下图所示
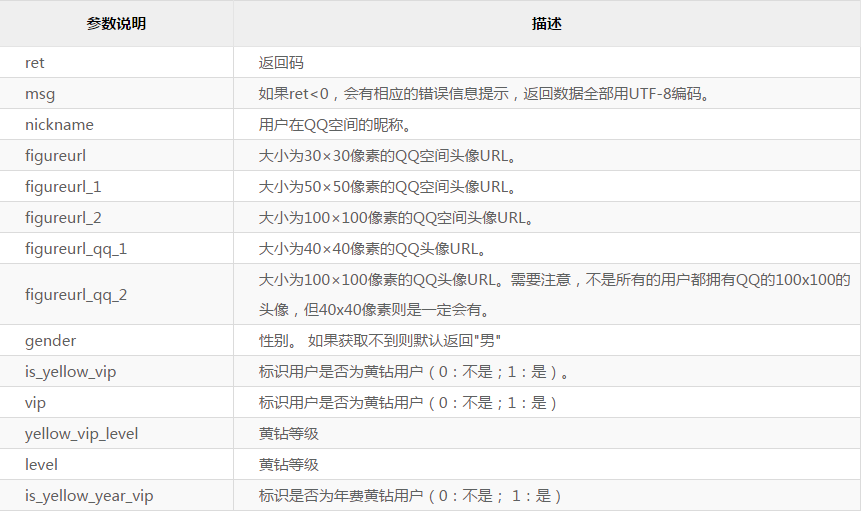