Hibernate -- lazy加载
hibernate类级别懒加载:
lazy:true(默认)
//类级别懒加载 //load方法 //class lazy属性 //默认值:true load获得时,会返回代理对象,不查询数据库,使用时才查询 public void fun1() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- Customer load = (Customer) session.load(Customer.class, 17); System.out.println("----------------sql语句未发送"); System.out.println(load.getName()); //-------------------------------------- session.getTransaction().commit(); session.close(); }
lazy :false
//类级别懒加载 //load方法 //class lazy属性 //默认值:false load方法执行就会发送sql语句,和get方法一致 public void fun2() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- Customer load = (Customer) session.load(Customer.class, 19); System.out.println("----------------sql语句发送"); System.out.println(load.getName()); //-------------------------------------- session.getTransaction().commit(); session.close(); }
hibernate (一对多)加载策略
关联级别懒加载的配置以及查询方式:
lazy:false
//关联级别懒加载 //lazy:false public void fun2() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- Customer load = (Customer) session.get(Customer.class, 19); System.out.println("所有的--------sql语句发送"); for (Order o : load.getOrders()) { System.out.println(o.getName()); } //-------------------------------------- session.getTransaction().commit(); session.close(); }
fetch:
fetch:join / lazy:false 立刻使用select语句加载集合数据
fetch:join / lazy:true 立刻使用select语句加载集合数据
fetch:join / lazy:extra 立刻使用select语句加载集合数据
两种相同:会立刻加载出集合数据
fetch:join / lazy:ture 查询集合时使用表连接查询,会立刻加载出集合数据 //关联级别懒加载 //lazy:false //fetch :join public void fun3() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- Customer load = (Customer) session.get(Customer.class, 19); System.out.println("左外连接查询--------sql语句发送"); for (Order o : load.getOrders()) { System.out.println(o.getName()); } //-------------------------------------- session.getTransaction().commit(); session.close(); }
select:(默认)表连接语句查询集合数据 lazy:false
fetch:subselect / lazy:false 立刻使用select语句加载集合数据
一次加载多个customer的订单数据
//关联级别懒加载 //lazy:false //fetch :subselect public void fun4() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- List<Customer> list = session.createQuery("from com.huhu.domain.Customer").list(); for (Customer c : list) { System.out.println(c.getName() + c.getOrders().size()); } //-------------------------------------- session.getTransaction().commit(); session.close(); }
fetch:subselect / lazy:true 立刻使用select语句加载集合数据
//关联级别懒加载 //lazy:true //fetch :subselect public void fun5() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- List<Customer> list = session.createQuery("from com.huhu.domain.Customer").list(); System.out.println("使用order时才去数据库查询"); for (Customer c : list) { System.out.println(c.getName() + c.getOrders().size()); } //-------------------------------------- session.getTransaction().commit(); session.close(); }
fetch:select / lazy:true (默认)普通的查询语句
//关联级别懒加载 //lazy:true //fetch :select public void fun6() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- System.out.println("customer--------sql语句发送"); Customer load = (Customer) session.get(Customer.class, 17); System.out.println("order--------sql语句发送"); for (Order o : load.getOrders()) { System.out.println(o.getName()); } //-------------------------------------- session.getTransaction().commit(); session.close(); }
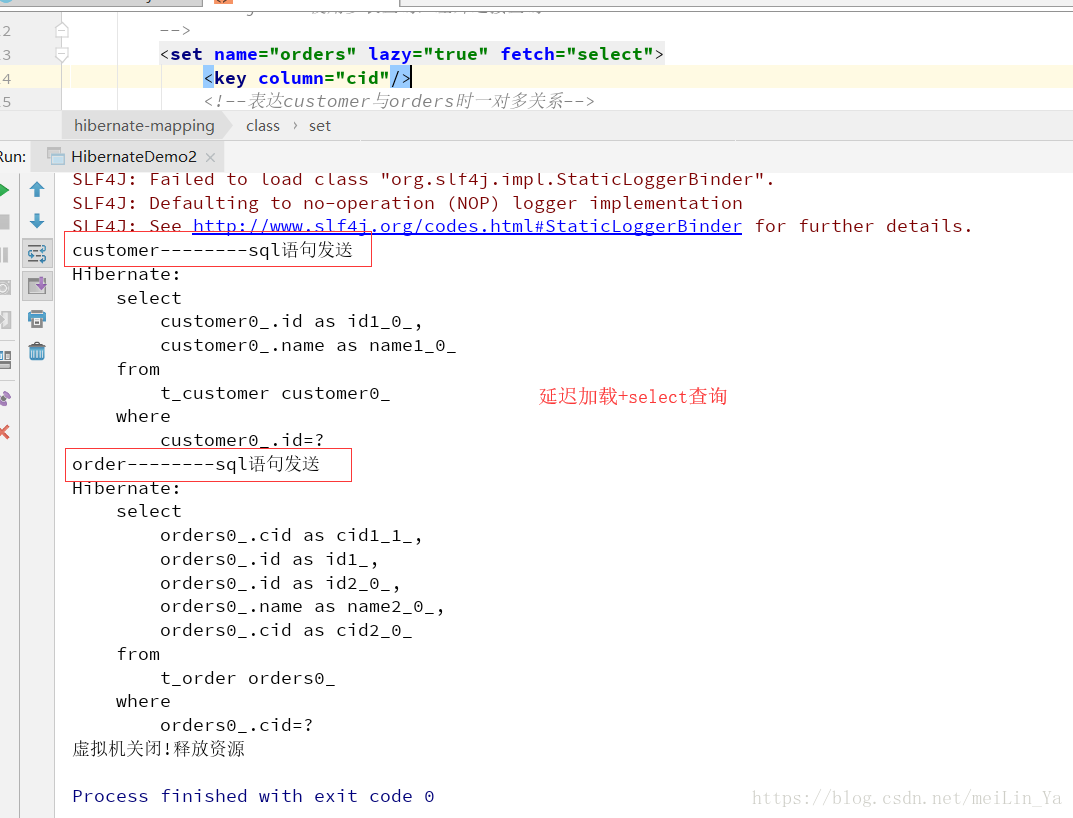
fetch:select / lazy:false 立刻使用select语句加载集合数据
//关联级别懒加载 //lazy:false //fetch :select public void fun7() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- Customer load = (Customer) session.get(Customer.class, 17); System.out.println("所有的--------sql语句发送"); for (Order o : load.getOrders()) { System.out.println(o.getName()); } //-------------------------------------- session.getTransaction().commit(); session.close(); }
关联级别懒加载:
由两个属性控制,在关联属性<set>中的两个属性:lazy和fecth,他们的对应值分别为 true,false,extra/select,subselect,join,这样一共有9中方法。仔细看看关于extra。
true/select:(默认值)会在使用集合是加载,普通的select语句
true/subselect:会在使用集合时加载,子查询语句,是延迟加载
true/join和false/join和extra/join:查询时使用表链接查询,会立刻加载集合数据,都没有延时加载
false/select:立刻使用select语句加载集合数据
false/subselect:会在查询customer时, 立即使用子查询加载客户的订单数据
extra:特别懒惰,如果时用集合时,之调用size()方法查询数量时,Hibernate发送count语句,只查询数量,不查询集合内数据
//关联级别懒加载 //lazy:extra //fetch :select public void fun8() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- Customer load = (Customer) session.get(Customer.class, 17); System.out.println("-------查询数量count()------"); System.out.println(load.getOrders().size()); System.out.println("----------查询customer--------"); for (Order o : load.getOrders()) { System.out.println(o.getName()); } //-------------------------------------- session.getTransaction().commit(); session.close(); }
extra/subselect
//关联级别懒加载 //lazy:extra //fetch :subselect public void fun9() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- List<Customer> list = session.createQuery("from com.huhu.domain.Customer").list(); for (Customer c : list) { System.out.println(c.getName() + "------------" + c.getOrders().size()); } for (Customer cs : list) { for (Order o : cs.getOrders()) { System.out.println(cs.getName() + "------------" + o.getName()); } } //-------------------------------------- session.getTransaction().commit(); session.close(); }
hibernate:加载策略多对一
多对一:
lazy
false 加载订单时,会立即加载客户
proxy 看客户对象的类加载策略来决定
no-proxy : 不做研究.
fetch=
select : (默认值)使用普通select加载
join : 使用表链接加载数据
测试:
package com.huhu.b_lazy; import com.huhu.domain.Customer; import com.huhu.domain.Order; import com.huhu.utils.HibernateUtils; import org.hibernate.Session; import java.util.List; /** * 加载策略:多对一 */ public class HibernateDemo3 { //fetch:select //lazy:false //默认:与我关联的数据时,在使用时才会加载。 public void fun1() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- Order order = (Order) session.get(Order.class, 17); System.out.println(order.getCustomer().getName()); //-------------------------------------- session.getTransaction().commit(); session.close(); } //fetch:select //lazy:proxy //Customer类加载策略:lazy :false //默认:与我关联的数据时,在使用时才会加载。 public void fun2() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- Order order = (Order) session.get(Order.class, 17); //-------------------------------------- session.getTransaction().commit(); session.close(); } //fetch:select //lazy:proxy //Customer类加载策略:lazy :true //默认:与我关联的数据时,在使用时才会加载。 public void fun3() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- Order order = (Order) session.get(Order.class, 19); System.out.println(order.getCustomer().getName()); //-------------------------------------- session.getTransaction().commit(); session.close(); } //fetch:join //lazy:proxy|false //Customer类加载策略:lazy :true //默认:与我关联的数据时,在使用时才会加载。 public void fun4() { Session session = HibernateUtils.openSession(); session.beginTransaction(); //-------------------------------------- Order order = (Order) session.get(Order.class, 19); //-------------------------------------- session.getTransaction().commit(); session.close(); } public static void main(String[] args) { HibernateDemo3 d = new HibernateDemo3(); d.fun4(); } }
如果想看代码得话可以复制以上代码测试。