Leonid wants to become a glass carver (the person who creates beautiful artworks by cutting the glass). He already has a rectangular wmm × h mm sheet of glass, a diamond glass cutter and lots of enthusiasm. What he lacks is understanding of what to carve and how.
In order not to waste time, he decided to practice the technique of carving. To do this, he makes vertical and horizontal cuts through the entire sheet. This process results in making smaller rectangular fragments of glass. Leonid does not move the newly made glass fragments. In particular, a cut divides each fragment of glass that it goes through into smaller fragments.
After each cut Leonid tries to determine what area the largest of the currently available glass fragments has. Since there appear more and more fragments, this question takes him more and more time and distracts him from the fascinating process.
Leonid offers to divide the labor — he will cut glass, and you will calculate the area of the maximum fragment after each cut. Do you agree?
The first line contains three integers w, h, n (2 ≤ w, h ≤ 200 000, 1 ≤ n ≤ 200 000).
Next n lines contain the descriptions of the cuts. Each description has the form H y or V x. In the first case Leonid makes the horizontal cut at the distance y millimeters (1 ≤ y ≤ h - 1) from the lower edge of the original sheet of glass. In the second case Leonid makes a vertical cut at distance x (1 ≤ x ≤ w - 1) millimeters from the left edge of the original sheet of glass. It is guaranteed that Leonid won't make two identical cuts.
After each cut print on a single line the area of the maximum available glass fragment in mm2.
4 3 4
H 2
V 2
V 3
V 1
8
4
4
2
7 6 5
H 4
V 3
V 5
H 2
V 1
28
16
12
6
4
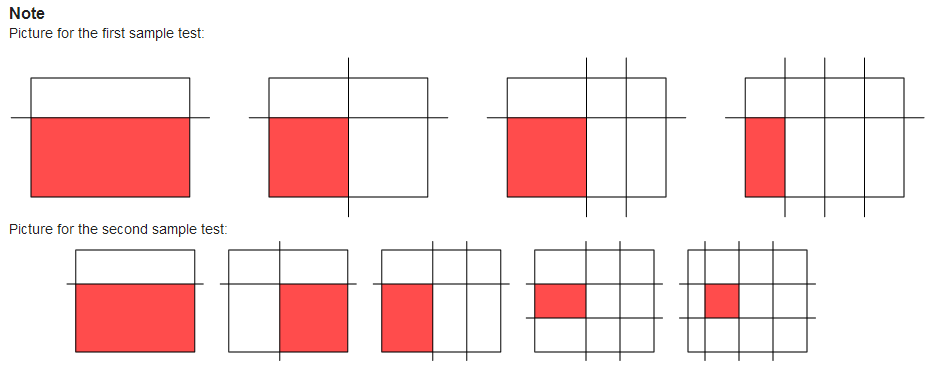
题意:给出一个n*m的矩阵,然后按照要求切线,问每切一条线分割后的最大矩形面积是多少?
分析:开4个set关联容器,其中sh存的是垂直线上的子区间长度,sw存的是水平线上的子区间长度,qh存的是划分的高度上的切割点,qw存的是划分宽度上的切割点,然后每次加入一条直线,先从q里面找出它的左右相邻的点,把该区间分割,然后用s维护子区间长度,每次把水平线上最大的子区间长度和垂直线上的最大子区间长度相乘即可
#include"cstdio" #include"cstring" #include"cstdlib" #include"cmath" #include"string" #include"map" #include"cstring" #include"algorithm" #include"iostream" #include"set" #include"queue" #include"stack" #define inf 0x3f3f3f3f #define M 200009 #define LL __int64 #define eps 1e-8 #define mod 1000000007 using namespace std; int w[M],h[M]; int main() { int W,H,n; while(scanf("%d%d%d",&W,&H,&n)!=-1) { set<int>sw,sh; set<int>qw,qh; sw.clear(); sh.clear(); memset(w,0,sizeof(w)); memset(h,0,sizeof(h)); sw.insert(W); sw.insert(-W); sh.insert(H); sh.insert(-H); qw.insert(0); qw.insert(W); qw.insert(-W); qh.insert(0); qh.insert(H); qh.insert(-H); w[W]++; h[H]++; char ch[3]; int x; while(n--) { scanf("%s%d",ch,&x); if(ch[0]=='H') { qh.insert(x); qh.insert(-x); int r=*(qh.upper_bound(x)); int l=-*(qh.upper_bound(-x)); if(h[r-l])//记录该长度出现的次数 h[r-l]--; if(!h[r-l]) { sh.erase(r-l); sh.erase(l-r); } sh.insert(x-l); h[x-l]++; sh.insert(r-x); h[r-x]++; sh.insert(l-x); sh.insert(x-r); } else { qw.insert(x); qw.insert(-x); int r=*(qw.upper_bound(x)); int l=-*(qw.upper_bound(-x)); if(w[r-l]) w[r-l]--; if(!w[r-l]) { sw.erase(r-l); sw.erase(l-r); } sw.insert(x-l); w[x-l]++; sw.insert(r-x); w[r-x]++; sw.insert(l-x); sw.insert(x-r); } set<int>::iterator r1,r2; r1=sh.begin(); r2=sw.begin(); printf("%I64d ",(LL)(*r1)*(*r2)); } } return 0; }
1 #include"cstdio" 2 #include"cstring" 3 #include"cstdlib" 4 #include"cmath" 5 #include"string" 6 #include"map" 7 #include"cstring" 8 #include"algorithm" 9 #include"iostream" 10 #include"set" 11 #include"queue" 12 #include"stack" 13 #define inf 0x3f3f3f3f 14 #define M 200009 15 #define LL __int64 16 #define eps 1e-8 17 #define mod 1000000007 18 using namespace std; 19 int w[M],h[M]; 20 int main() 21 { 22 int W,H,n; 23 while(scanf("%d%d%d",&W,&H,&n)!=-1) 24 { 25 set<int>sw,sh; 26 set<int>qw,qh; 27 sw.clear(); 28 sh.clear(); 29 memset(w,0,sizeof(w)); 30 memset(h,0,sizeof(h)); 31 sw.insert(W); 32 sw.insert(-W); 33 sh.insert(H); 34 sh.insert(-H); 35 qw.insert(0); 36 qw.insert(W); 37 qw.insert(-W); 38 qh.insert(0); 39 qh.insert(H); 40 qh.insert(-H); 41 w[W]++; 42 h[H]++; 43 char ch[3]; 44 int x; 45 while(n--) 46 { 47 scanf("%s%d",ch,&x); 48 if(ch[0]=='H') 49 { 50 qh.insert(x); 51 qh.insert(-x); 52 int r=*(qh.upper_bound(x)); 53 int l=-*(qh.upper_bound(-x)); 54 if(h[r-l])//记录该长度出现的次数 55 h[r-l]--; 56 if(!h[r-l]) 57 { 58 sh.erase(r-l); 59 sh.erase(l-r); 60 } 61 62 sh.insert(x-l); 63 h[x-l]++; 64 sh.insert(r-x); 65 h[r-x]++; 66 sh.insert(l-x); 67 sh.insert(x-r); 68 } 69 else 70 { 71 qw.insert(x); 72 qw.insert(-x); 73 int r=*(qw.upper_bound(x)); 74 int l=-*(qw.upper_bound(-x)); 75 if(w[r-l]) 76 w[r-l]--; 77 if(!w[r-l]) 78 { 79 sw.erase(r-l); 80 sw.erase(l-r); 81 } 82 83 84 sw.insert(x-l); 85 w[x-l]++; 86 sw.insert(r-x); 87 w[r-x]++; 88 sw.insert(l-x); 89 sw.insert(x-r); 90 } 91 set<int>::iterator r1,r2; 92 r1=sh.begin(); 93 r2=sw.begin(); 94 printf("%I64d ",(LL)(*r1)*(*r2)); 95 } 96 } 97 return 0; 98 }