GitHub地址,最新版本为2.0.2基于spring boot 2.x
https://github.com/codecentric/spring-boot-admin
注意:选分支版本每个版本还一样:本示例是基于1.5.x
开始整合的时候踩了很多坑,都是因为版本不兼容的问题,最后在gitHub上找到。按照官方版本
spring boot 用的是1.5.10
spring cloud 用的Edgware.SR4版本。版本太不兼容了,开始用SR1版本,但是spring cloud相关相关jar引入不进来报错,最后在spring cloud官方看,用了Edgware最后一个版本SR4。
示例:
新建maven项目如下结构:
parent pom.xml配置
主要配置spring boot 和spring cloud 版本,具体配置如下:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.niugang</groupId>
<artifactId>927.spring-boot-admin-simple</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>pom</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
<spring-cloud.version>Edgware.SR4</spring-cloud.version>
<spring-boot.version>1.5.10.RELEASE</spring-boot.version>
</properties>
<dependencyManagement>
<dependencies>
<!--引入springboot -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>${spring-boot.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<!--引入springcloud -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<modules>
<module>927.spring-boot-admin-simple-server</module>
<module>927..spring-boot-admin-simple-client</module>
</modules>
</project>
927.spring-boot-admin-simple-server
pom.xml
引入相关依赖配置
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.niugang</groupId>
<artifactId>927.spring-boot-admin-simple</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<properties>
<spring-boot-admin.version>1.5.7</spring-boot-admin.version>
</properties>
<artifactId>927.spring-boot-admin-simple-server</artifactId>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--安全 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<!--spring boot admin server -->
<dependency>
<groupId>de.codecentric</groupId>
<artifactId>spring-boot-admin-starter-server</artifactId>
<version>${spring-boot-admin.version}</version>
</dependency>
<dependency>
<groupId>de.codecentric</groupId>
<artifactId>spring-boot-admin-server-ui-login</artifactId>
<version>${spring-boot-admin.version}</version>
</dependency>
<!-- 当时没引报错 -->
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>19.0</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
SecurityConfig.java
安全认证相关配置
package org.niugang.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
/**
* 基于安全认证的spring boot admin
*
* @author niugang
*
*/
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
// Page with login form is served as /login.html and does a POST on /login
http.formLogin().loginPage("/login.html").loginProcessingUrl("/login").permitAll();
// The UI does a POST on /logout on logout
http.logout().logoutUrl("/logout");
// The ui currently doesn't support csrf
http.csrf().disable();
// Requests for the login page and the static assets are allowed
//允许登录页面和静态资源的请求
http.authorizeRequests()
.antMatchers("/login.html", "/**/*.css", "/img/**", "/third-party/**")
.permitAll();
// ... and any other request needs to be authorized
//这点重要:所有请求都需要认证
http.authorizeRequests().antMatchers("/**").authenticated();
// Enable so that the clients can authenticate via HTTP basic for registering
http.httpBasic();
}
}
NotifierConfig.java
通知消息相关重写
package org.niugang.config;
import java.util.concurrent.TimeUnit;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import org.springframework.scheduling.annotation.Scheduled;
import de.codecentric.boot.admin.notify.LoggingNotifier;
import de.codecentric.boot.admin.notify.Notifier;
import de.codecentric.boot.admin.notify.RemindingNotifier;
import de.codecentric.boot.admin.notify.filter.FilteringNotifier;
import org.springframework.scheduling.annotation.EnableScheduling;
/**
*
* 重新配置消息通知
* @author niugang
*
*/
@Configuration
@EnableScheduling
public class NotifierConfig {
/**
*
提醒将每5分钟发送一次。
* @return
*/
@Bean
@Primary
public RemindingNotifier remindingNotifier() {
RemindingNotifier notifier = new RemindingNotifier(filteringNotifier(loggerNotifier()));
notifier.setReminderPeriod(TimeUnit.SECONDS.toMillis(5));
return notifier;
}
/**
* 每60秒发送一次应有的提醒。
*/
@Scheduled(fixedRate = 60_000L)
public void remind() {
remindingNotifier().sendReminders();
}
@Bean
public FilteringNotifier filteringNotifier(Notifier delegate) {
return new FilteringNotifier(delegate);
}
@Bean
public LoggingNotifier loggerNotifier() {
return new LoggingNotifier();
}
}
启动类
package org.niugang;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import de.codecentric.boot.admin.config.EnableAdminServer;
/**
*
* Copyright: Copyright (c) 2018 863263957@qq.com
*
* @ClassName: SpringBootAdminApplication.java
* @Description: 启动
* @author: niugang
* @date: 2018年9月9日 下午1:43:02
*
* Modification History:
* Date Author Version Description
*---------------------------------------------------------*
* 2018年9月9日 niugang v1.0.0 修改原因
*/
@SpringBootApplication
@EnableAdminServer
public class SpringBootAdminApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootAdminApplication.class, args);
}
}
application.properties
server.port=8080
#关闭原始的spring security 认证,不关闭的话,浏览器打开就会跳出弹出框
security.basic.enabled=false
#spring boot actuator某些端点的访问时需要权限的
management.security.enabled=false
#spring boot default user.name='user'
security.user.name=root
#spring boot dafault user.password 在项目启动时打印在控制台中
security.user.password=root
启动springboot启动类,访问http://localhost:8080/login.html
用户名和密码都是上面配置的root
以上完成还没有client端,以下新建client端demo
927.spring-boot-admin-simple-client
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.niugang</groupId> <artifactId>927.spring-boot-admin-simple</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <artifactId>927.spring-boot-admin-simple-client</artifactId> <dependencies> <!--每个要注册的应用程序都必须包含Spring Boot Admin Client。 --> <dependency> <groupId>de.codecentric</groupId> <artifactId>spring-boot-admin-starter-client</artifactId> <version>1.5.7</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies> </project>
启动类
package org.niugang; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; /** * * Copyright: Copyright (c) 2018 863263957@qq.com * * @ClassName: SpringBootAdminClientApplication.java * @Description: 客户端启动类 * @author: niugang * @date: 2018年9月9日 下午1:54:36 * * Modification History: * Date Author Version Description *---------------------------------------------------------* * 2018年9月9日 niugang v1.0.0 修改原因 */ @SpringBootApplication public class SpringBootAdminClientApplication { public static void main(String[] args) { SpringApplication.run(SpringBootAdminClientApplication.class, args); } }
application.properties
server.port=8081 spring.application.name=spring-boot-admin-client #要注册的Spring Boot Admin Server的URL spring.boot.admin.url=http://localhost:8080 #从Spring Boot 1.5.x开始,默认情况下所有端点都是安全的。 为简洁起见,我们暂时禁用了安全性。 查看有关如何处理安全端点的安全性部分。 management.security.enabled=false spring.boot.admin.client.metadata.user.name=root spring.boot.admin.client.metadata.user.password=root #如果保护/api/applications端点,请不要忘记使用spring.boot.admin.username和spring.boot.admin.password在SBA客户端上配置用户名和密码【否则你的client端信息注册不到server端上】 spring.boot.admin.username=root spring.boot.admin.password=root
启动client端
通知:
刷新浏览器
源码地址:https://gitee.com/niugangxy/springcloud 在 spring-cloud-learn-code文件下中
官方文档对Spring-Boot-Admin安全的介绍
保护Spring Boot Admin Server
由于解决分布式Web应用程序中的身份验证和授权有多种方法,因此Spring Boot Admin不提供默认方法。 如果在依赖项中包含spring-boot-admin-server-ui-login,它将提供登录页面和注销按钮。
Spring Security配置可能如下所示:
@Configuration public static class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { // Page with login form is served as /login.html and does a POST on /login http.formLogin().loginPage("/login.html").loginProcessingUrl("/login").permitAll(); // The UI does a POST on /logout on logout http.logout().logoutUrl("/logout"); // The ui currently doesn't support csrf http.csrf().disable(); // Requests for the login page and the static assets are allowed http.authorizeRequests() .antMatchers("/login.html", "/**/*.css", "/img/**", "/third-party/**") .permitAll(); // ... and any other request needs to be authorized http.authorizeRequests().antMatchers("/**").authenticated(); // Enable so that the clients can authenticate via HTTP basic for registering http.httpBasic(); } }
注意:
如果保护/api/applications端点,请不要忘记使用spring.boot.admin.username和spring.boot.admin.password在SBA客户端上配置用户名和密码【否则你的client端信息注册不到server端上】。
官方示例地址:https://github.com/codecentric/spring-boot-admin/blob/1.5.x/spring-boot-admin-samples
保护客户端Actuator端点
使用HTTP基本身份验证保护Actuator点时,SBA服务器需要凭据才能访问它们。 注册应用程序时,您可以在元数据中提交凭据。 然后,BasicAuthHttpHeaderProvider使用此元数据添加Authorization标头以访问应用程序的执行器端点。 您可以提供自己的HttpHeadersProvider来改变行为(例如添加一些解密)或添加额外的标头。
使用SBA客户端提交凭据:
application.yml
spring.boot.admin:
url: http://localhost:8080
client:
metadata:
user.name: ${security.user.name}
user.password: ${security.user.password}
使用Eureka提交凭据:
application.yml
eureka:
instance:
metadata-map:
user.name: ${security.user.name}
user.password: ${security.user.password}
注意:SBA服务器屏蔽HTTP接口中的某些元数据,以防止泄漏敏感信息。
在通过元数据提交凭据时,应为SBA服务器或(服务注册表)配置HTTPS。
使用Spring Cloud Discovery时,您必须意识到任何可以查询服务注册表的人都可以获取凭据。
微信公众号
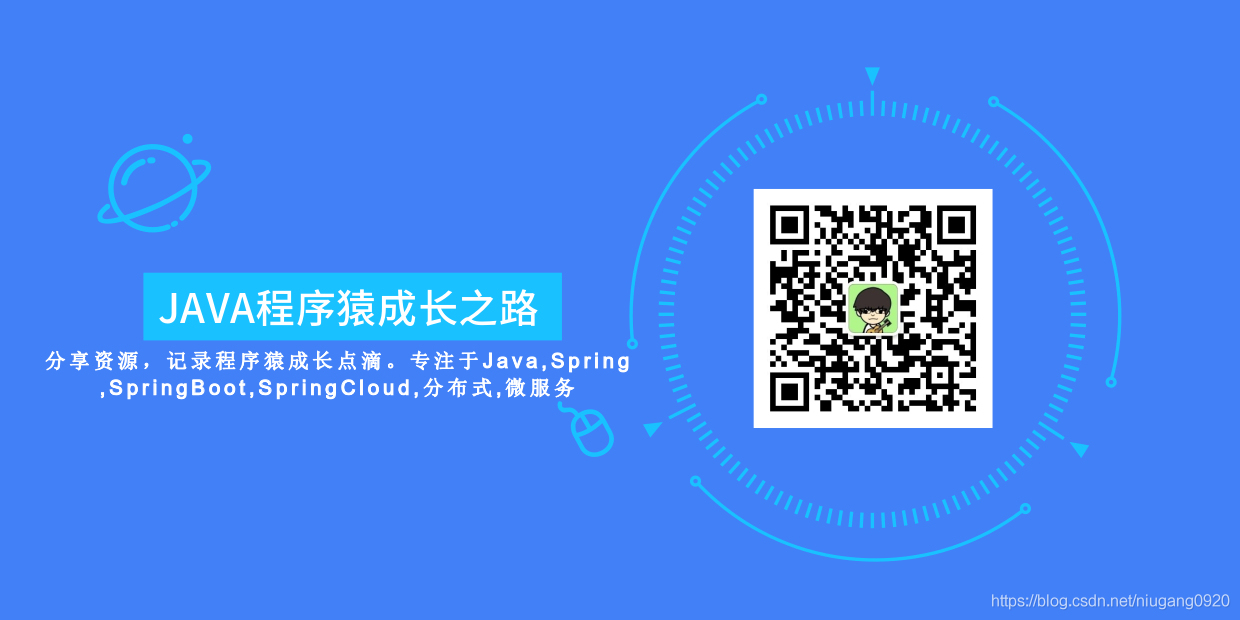