1: 完成弹出式对话框;
2: 完成个性化时间进度条;
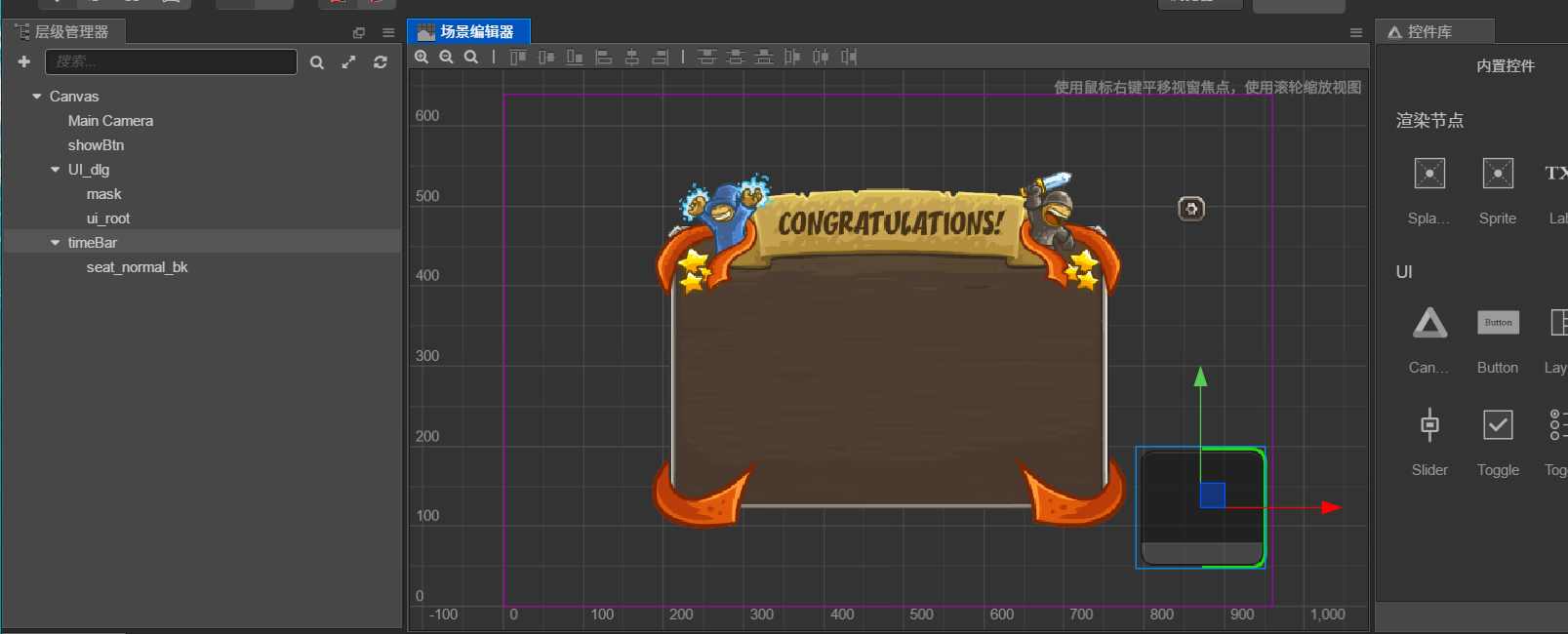
弹出式对话框
1:对话框的结构:
根节点 -->
mask: 全屏的单色精灵,监听事件,关闭对话框;
dlg 与它的孩子: 对话框的内容,监听事件,挡住不让他传递到mask节点上;
弹出式动画:
mask: 渐变进来;
对话框内容缩放,并加上easing缓动对象;
收起式动画:
mask: 渐变出去;
对话框内容缩小,并加上easing 缓动对象;
2: 对话框组件脚本
(1)show_dlg
(2)hide_dlg
1 // popup_dlg.js 2 cc.Class({ 3 extends: cc.Component, 4 5 properties: { 6 // foo: { 7 // default: null, // The default value will be used only when the component attaching 8 // to a node for the first time 9 // url: cc.Texture2D, // optional, default is typeof default 10 // serializable: true, // optional, default is true 11 // visible: true, // optional, default is true 12 // displayName: 'Foo', // optional 13 // readonly: false, // optional, default is false 14 // }, 15 // ... 16 mask: { 17 type: cc.Node, 18 default: null, 19 }, 20 21 mask_opacity: 128, 22 23 content: { 24 type: cc.Node, 25 default: null, 26 }, 27 28 }, 29 30 // use this for initialization 31 onLoad: function () { 32 33 }, 34 35 show_dlg: function() { 36 this.node.active = true; 37 // mask 渐变出来; 38 this.mask.opacity = 0; 39 var fin = cc.fadeTo(0.3, this.mask_opacity); 40 this.mask.runAction(fin); 41 // dlg由小到大 42 43 this.content.scale = 0; 44 var s = cc.scaleTo(0.4, 1).easing(cc.easeBackOut()); 45 this.content.runAction(s); 46 }, 47 48 hide_dlg: function() { 49 // 50 var fout = cc.fadeOut(0.3); 51 this.mask.runAction(fout); 52 53 var s = cc.scaleTo(0.3, 0).easing(cc.easeBackIn()); 54 var end_func = cc.callFunc(function() { 55 this.node.active = false; 56 }.bind(this)); 57 58 var seq = cc.sequence([s, end_func]); 59 this.content.runAction(seq); 60 }, 61 // called every frame, uncomment this function to activate update callback 62 // update: function (dt) { 63 64 // }, 65 });
// game_scene.js var popup_dlg = require("piopup_dlg"); cc.Class({ extends: cc.Component, properties: { // foo: { // // ATTRIBUTES: // default: null, // The default value will be used only when the component attaching // // to a node for the first time // type: cc.SpriteFrame, // optional, default is typeof default // serializable: true, // optional, default is true // }, // bar: { // get () { // return this._bar; // }, // set (value) { // this._bar = value; // } // }, dlg : { type : popup_dlg, default : null }, }, // LIFE-CYCLE CALLBACKS: // onLoad () {}, start () { }, on_show_dlg_click: function(){ this.dlg.showDlg(); }, // update (dt) {}, });
个性化时间进度条
1: 编写脚本, 来使用sprite的扇形来显示当前的进度:
属性:
time_sec: 定时器的时间
clockwise: 是否为顺时针或逆时针;
reverse: 是否反转
start_clock_action: 开始累积时间,看时间过去的百分比,来改变精灵显示的百分比;
stop_clock_action: 停止计时累积;
// timebar.js cc.Class({ extends: cc.Component, properties: { // foo: { // default: null, // The default value will be used only when the component attaching // to a node for the first time // url: cc.Texture2D, // optional, default is typeof default // serializable: true, // optional, default is true // visible: true, // optional, default is true // displayName: 'Foo', // optional // readonly: false, // optional, default is false // }, // ... action_time: 15, clockwise: false, // 是否为顺时针 reverse: false, // false, 由少变多,否者的话的就是由多变少; play_onload: true, // 是否在加载的时候开始倒计时 }, // use this for initialization onLoad: function () { this.now_time = 0; this.is_running = false; this.node.active = false; this.sprite = this.getComponent(cc.Sprite); if (this.play_onload) { this.start_clock_action(this.action_time); } }, start_clock_action: function(action_time, end_func) { if (action_time <= 0) { return; } this.end_func = end_func; this.node.active = true; this.action_time = action_time; this.now_time = 0; this.is_running = true; }, stop_clock_action: function() { this.node.active = false; this.is_running = false; }, // called every frame, uncomment this function to activate update callback update: function (dt) { if (!this.is_running) { return; } this.now_time += dt; var per = this.now_time / this.action_time; if (per > 1) { // 结束了,超时了 per = 1; this.is_running = false; if (this.end_func) { this.end_func(); } } if (this.reverse) { per = 1 - per; } if (this.clockwise) { per = -per; } this.sprite.fillRange = per; }, });