我们使用的是永中的第三方服务、支持直接转换文档的线上地址,也可以直接把文档上传到官方服务器上
官方文档地址:https://www.yozodcs.com/page/help.html#link152
引入jar包:
commons-logging-1.1.jar,httpclient-4.5.jar,httpcore-4.4.1.jar,httpmime-4.5.jar
或者引入maven依赖
<!-- https://mvnrepository.com/artifact/commons-logging/commons-logging --> <dependency> <groupId>commons-logging</groupId> <artifactId>commons-logging</artifactId> <version>1.2</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpclient --> <dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpclient</artifactId> <version>4.5.12</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpcore --> <dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpcore</artifactId> <version>4.4.13</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpmime --> <dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpmime</artifactId> <version>4.5.12</version> </dependency>
调用方法类
package com.example.demo.controller; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.io.PrintWriter; import java.net.URL; import java.net.URLConnection; import org.springframework.stereotype.Controller; @Controller public class FileController { /** * 向指定 URL 发送POST方法的请求 * @param url 发送请求的 URL * @param param 请求参数,请求参数应该是 name1=value1&name2=value2 的形式。 * @return 远程资源的响应结果 */ public static String sendPost(String url, String param) { PrintWriter out = null; BufferedReader in = null; String result = ""; try { URL realUrl = new URL(url); // 打开和URL之间的连接 URLConnection conn = realUrl.openConnection(); conn.setRequestProperty("Accept-Charset", "UTF-8"); // 设置通用的请求属性 conn.setRequestProperty("accept", "*/*"); conn.setRequestProperty("connection", "Keep-Alive"); conn.setRequestProperty("user-agent", "Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1;SV1)"); // 发送POST请求必须设置如下两行 conn.setDoOutput(true); conn.setDoInput(true); // 获取URLConnection对象对应的输出流 out = new PrintWriter(conn.getOutputStream()); // 发送请求参数 out.print(param); // flush输出流的缓冲 out.flush(); // 定义BufferedReader输入流来读取URL的响应 in = new BufferedReader(new InputStreamReader(conn.getInputStream())); String line; while ((line = in.readLine()) != null) { result += line; } } catch (Exception e) { System.out.println("发送 POST 请求出现异常!" + e); e.printStackTrace(); } // 使用finally块来关闭输出流、输入流 finally { try { if (out != null) { out.close(); } if (in != null) { in.close(); } } catch (IOException ex) { ex.printStackTrace(); } } return result; } //调用方式 public static void main(String[] args) { /** * 网络地址转换 * http://dcs.yozosoft.com:80/onlinefile 这是永中转换的固定地址 * http://video.ch9.ms/build/2011/slides/TOOL-532T_Sutter.pptx 这是网上文档的绝对路径 * convertType 这是转换类型参数(必须) 具体参数详解见下方 */ String convertByUrl = sendPost("http://dcs.yozosoft.com:80/onlinefile", "downloadUrl=http://video.ch9.ms/build/2011/slides/TOOL-532T_Sutter.pptx&convertType=1"); System.out.println(convertByUrl); //返回json数据:{"result":0,"data":["http://dcs.yozosoft.com/view/2020/06/15/MjAwNjE1MTU3MzQ4MjE0.html"],"message":"转换成功","type":1} } }
转换成功后,直接浏览器打开 http://dcs.yozosoft.com/view/2020/06/15/MjAwNjE1MTU3MzQ4MjE0.html 这个链接即可(该链接时间长会失效,需要重新生成)
convertType参数取值说明
0-----文档格式到高清html的转换
1-----文档格式到html的转换
2-----文档格式到txt的转换
3-----文档格式到pdf的转换
4-----文档格式到gif的转换
5-----文档格式到png的转换
6-----文档格式到jpg的转换
7-----文档格式到tiff的转换
8-----文档格式到bmp的转换
9-----pdf文档格式到gif的转换
10----pdf文档格式到png的转换
11----pdf文档格式到jpg的转换
12----pdf文档格式到tiff的转换
13----pdf文档格式到bmp的转换
14----pdf文档格式到html的转换
15----html文档格式到微软文档格式的转换
16----文档转换多个SVG返回分页加载页面(模版)
17----tif文件转成html
18----文档转换多个SVG
19----压缩文件到html的转换(模版)
20----PDF文件到html的转换(模版)
21----ofd文件到html的转换(模版)
22----两个doc文档合并
23----图片到html的转换
24----pdf文档格式到txt的转换
25----文档按页转换(高清版)
26----文档按页转换(标准版)
27----获取文档页码(MS文档)
28----获取pdf页码(PDF文件)
29----文档到ofd的转换
30----文档到html(图片)的转换
31----多个pdf文档合并
32----图片到pdf的转换
33----文档到文档的转换
34----pdf到pdf的转换
35----tif到html的转换(模板)
返回参数说明:
result - 返回代码,0成功,其他失败
data - 请求需要返回的数据
message - 详细信息
成功状态下:{ "result":0,"data":"http://xxxx域名/文件地址","message":"转换成功" }
失败状态下: { "result":7, "":"上传失败" }
result - 返回状态码说明
0-----转换成功
1-----找不到指定文档
2-----无法打开指定文档
3-----转换失败
4-----转换的文档为加密文档,无法转换
5-----输出文档后缀错误
6-----授权文件过期
7-----转换超时,内容可能不完整
8-----无效参数
17-----上传失败
18-----下载文件失败
19-----文件过大
20-----下载成功
21-----删除失败
22-----获取文件信息失败
23-----URL编码失败
24-----生成文件名为空,有误或重名,请检查参数
25-----html转pdf转换失败
如果要对预览的文档加上防复制、水印等高级功能的话,可以购买永中DCS的私有化部署服务(适合对文档安全性要求比较高的企业),官网可以获取价格及测试包,也可以使用永中云转换的高级服务,使用非常简单便捷,如下:
浏览器打开:https://api.yozocloud.cn/index.html ,点击右上角的注册按钮进行注册,注册成功后,登录后台,再点击域名管理进行域名的绑定,绑定成功后,会生成一个域名key,这是后面调用接口的凭证
调用方式
http://dcsapi.com/?k=域名key&url=要预览的文件地址
例如:http://dcsapi.com/?k=1744232&url=http://58.215.166.234/example/doc/doctest.docx
注:其中文件的地址要和绑定的域名一样。
也可以在后面可以拼接四个可选参数:
1、noCache:Boolean格式。是否强制重新转换(忽略缓存),true为强制重新转换,false为不强制重新转换。
2、watermark:String格式。针对单文档设置水印内容。
3、isCopy:Integer格式(0否1是,默认为0。针对单文档设置是否防复制)。
4、pageStart:Integer格式;pageEnd:Integer格式。试读功能(转换页数的起始页和转换页数的终止页,拥有对应权限的域名才能调用)。
调用方式:http://api网址/ getPreview?k=(域名对应的用户Key)&url=(在线文档地址) &isCopy=(是否防复制1是0否,可选)&watermaerk=(水印文字内容,可选)
不过由于我们这个是免费版,所以不支持高级功能
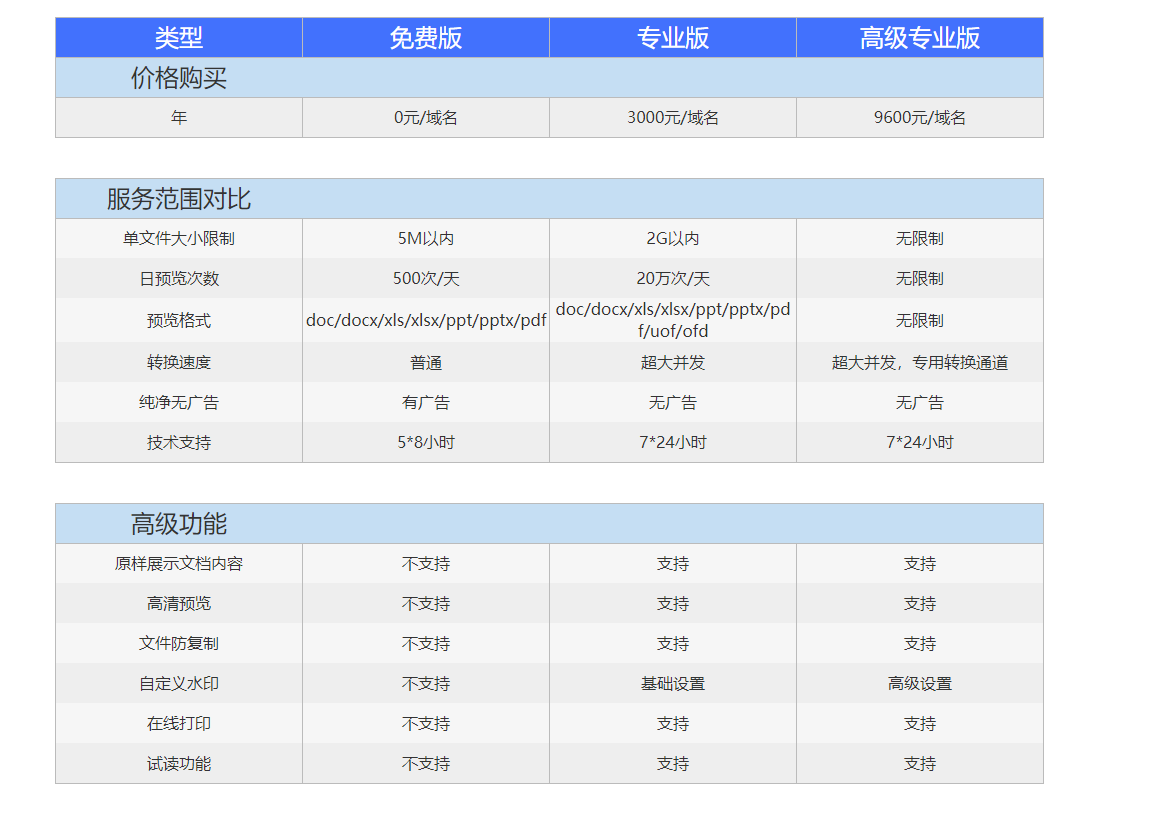
具体可以打开https://www.yozodcs.com/page/yun.html 查看详情