1. Description: 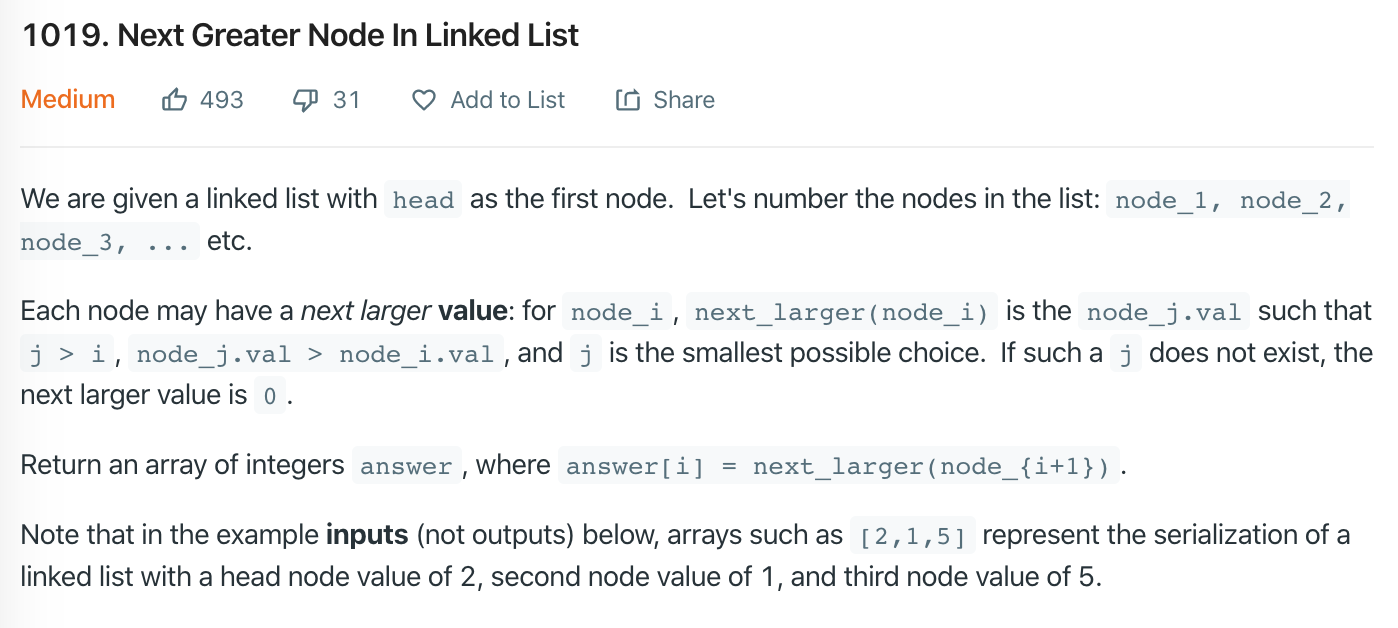
Notes:
2. Examples: 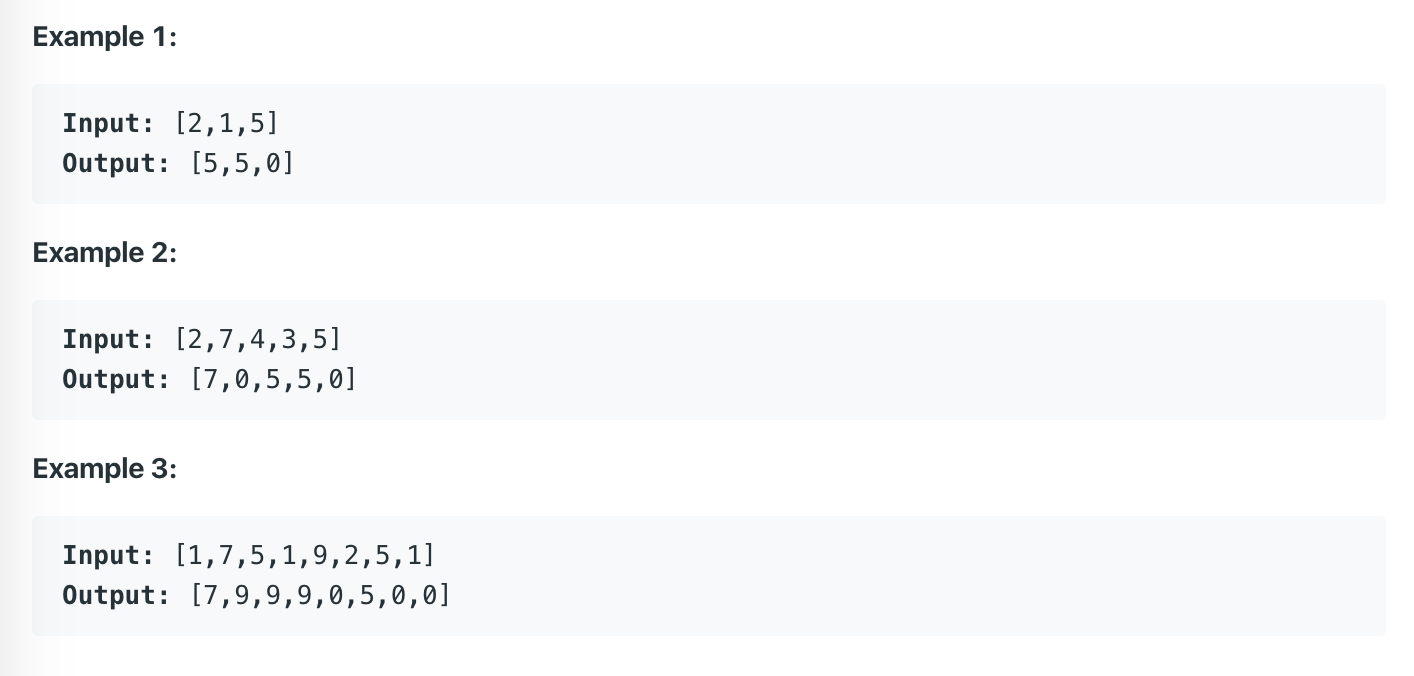
3.Solutions:
1 /** 2 * Created by sheepcore on 2019-05-09 3 * Definition for singly-linked list. 4 * public class ListNode { 5 * int val; 6 * ListNode next; 7 * ListNode(int x) { val = x; } 8 * } 9 */ 10 class Solution { 11 public int[] nextLargerNodes(ListNode head) { 12 ArrayList<Integer> A = new ArrayList<>(); 13 for (ListNode node = head; node != null; node = node.next) 14 A.add(node.val); 15 int[] res = new int[A.size()]; 16 Stack<Integer> stack = new Stack<>(); 17 for (int i = 0; i < A.size(); ++i) { 18 while (!stack.isEmpty() && A.get(stack.peek()) < A.get(i)) 19 res[stack.pop()] = A.get(i); 20 stack.push(i); 21 } 22 return res; 23 } 24 }