JSF入门第一篇,一个类似Hello world的演示,高手绕行~
测试环境:MyEclipse Blue 6.5 + JDK 1.6
第一步:新建一个项目
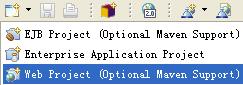
第二步:添加JSF引用(这个说法似乎有点太.NET)

第三步:建立两个JavaBean
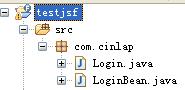
代码如下:
loginBean.java代码:

Code
1 package com.cinlap;
2
3 public class LoginBean {
4 private String user = "";
5 private String password = "";
6 private boolean logined = false;
7 public boolean isLogined() {
8 return logined;
9 }
10 public void setLogined(boolean logined) {
11 this.logined = logined;
12 }
13 public String getUser() {
14 return user;
15 }
16 public void setUser(String user) {
17 this.user = user;
18 }
19 public String getPassword() {
20 return password;
21 }
22 public void setPassword(String password) {
23 this.password = password;
24 }
25
26 public String login() {
27 Login login = new Login();
28 this.logined = login.LoginIn(this.user, this.password);
29 return "success";
30 }
31 }
32
login.java代码:

Code
1 package com.cinlap;
2
3 public class Login {
4 public boolean LoginIn(String user,String password) {
5 if ( user == "think8848" && password == "123456") {
6 return true;
7 }
8 else{
9 return false;
10 }
11 }
12 }
13
第四步:新建两个jsp页面

注意选择Default JSF template
界面和代码如下:
index.jsp界面:
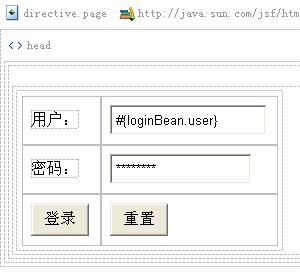
index.jsp代码:

Code
1 <%@ page language="java" pageEncoding="utf-8"%>
2 <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %>
3 <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %>
4
5 <%
6 String path = request.getContextPath();
7 String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
8 %>
9
10 <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
11 <html>
12 <head>
13 <base href="<%=basePath%>">
14
15 <title>My JSF 'index.jsp' starting page</title>
16
17 <meta http-equiv="pragma" content="no-cache">
18 <meta http-equiv="cache-control" content="no-cache">
19 <meta http-equiv="expires" content="0">
20 <meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
21 <meta http-equiv="description" content="This is my page">
22 <!--
23 <link rel="stylesheet" type="text/css" href="styles.css">
24 -->
25
26 </head>
27
28 <body>
29 <f:view>
30 <h:form>
31 <h:panelGrid columns="2">
32 <h:outputLabel value="用户:" />
33 <h:inputText id="txtUser" value="#{loginBean.user}" />
34 <h:outputLabel value="密码:" />
35 <h:inputSecret id="txtPassword" value="#{loginBean.password}" />
36 <h:commandButton id="btnSubmit" value="登录" action="#{loginBean.login}" />
37 <h:commandButton id="btnReset" value="重置" />
38 </h:panelGrid>
39 </h:form>
40 </f:view>
41 </body>
42 </html>
welcome.jsp界面:
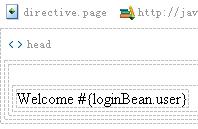
welcome.jsp代码

Code
1 <%@ page language="java" pageEncoding="utf-8"%>
2 <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %>
3 <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %>
4
5 <%
6 String path = request.getContextPath();
7 String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
8 %>
9
10 <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
11 <html>
12 <head>
13 <base href="<%=basePath%>">
14
15 <title>My JSF 'welcome.jsp' starting page</title>
16
17 <meta http-equiv="pragma" content="no-cache">
18 <meta http-equiv="cache-control" content="no-cache">
19 <meta http-equiv="expires" content="0">
20 <meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
21 <meta http-equiv="description" content="This is my page">
22 <!--
23 <link rel="stylesheet" type="text/css" href="styles.css">
24 -->
25
26 </head>
27
28 <body>
29 <f:view>
30 <h:form>
31 <h:outputLabel value="Welcome #{loginBean.user}" />
32 </h:form>
33 </f:view>
34 </body>
35 </html>
第五步:配置Managed Bean(Managed听起来舒服,但似乎和.NET的Manage没有丝毫关系)
将loginBean添加到Managed Beans中
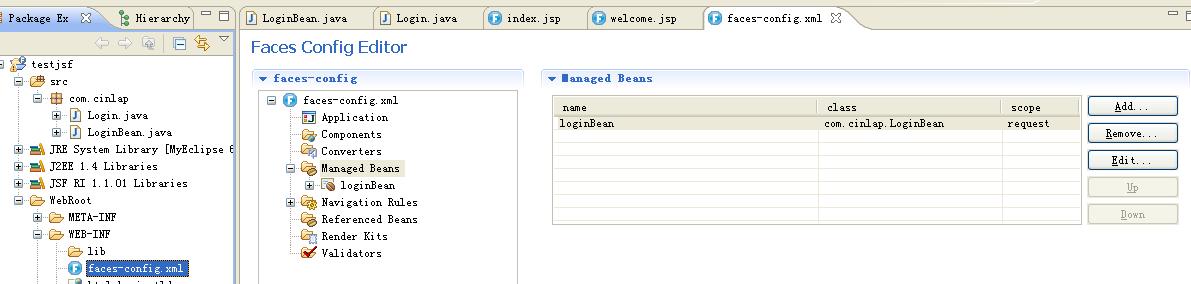
第六步:添加Navigation Rules
通俗点来说,就是添加页面跳转规则
注意这个from-outcome不是随便设置的,它和javaBean.java中的login方法的返回值相同(必须)
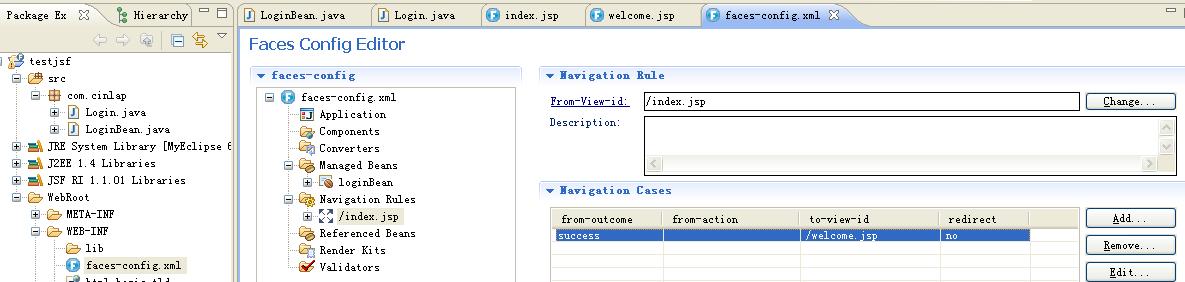
第七步:将该项目添加到运行环境中(我都忘了准确的说法了)
点击这个按钮之后的事我就不多讲了
第八步:启动MyEclipse Tomcat
略
第九步:在浏览器中输入http://localhost:8080/testjsf/index.faces

输入用户:think8848,密码:123456
第十步:点击登录
显示结果
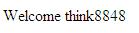