第七章 异常处理
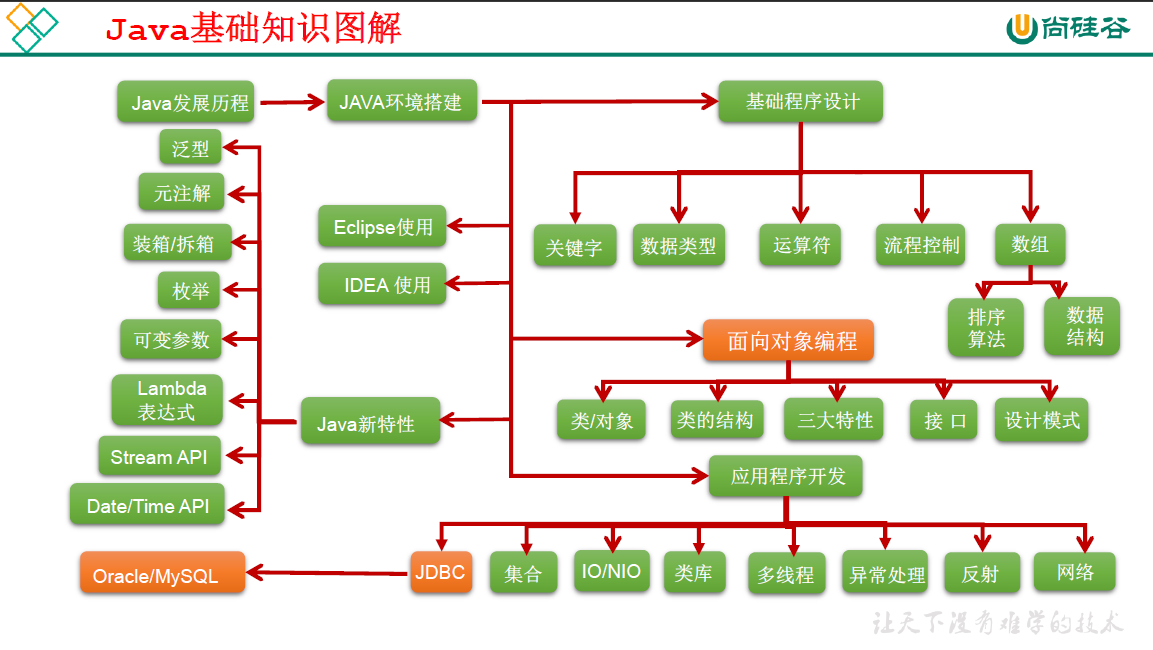
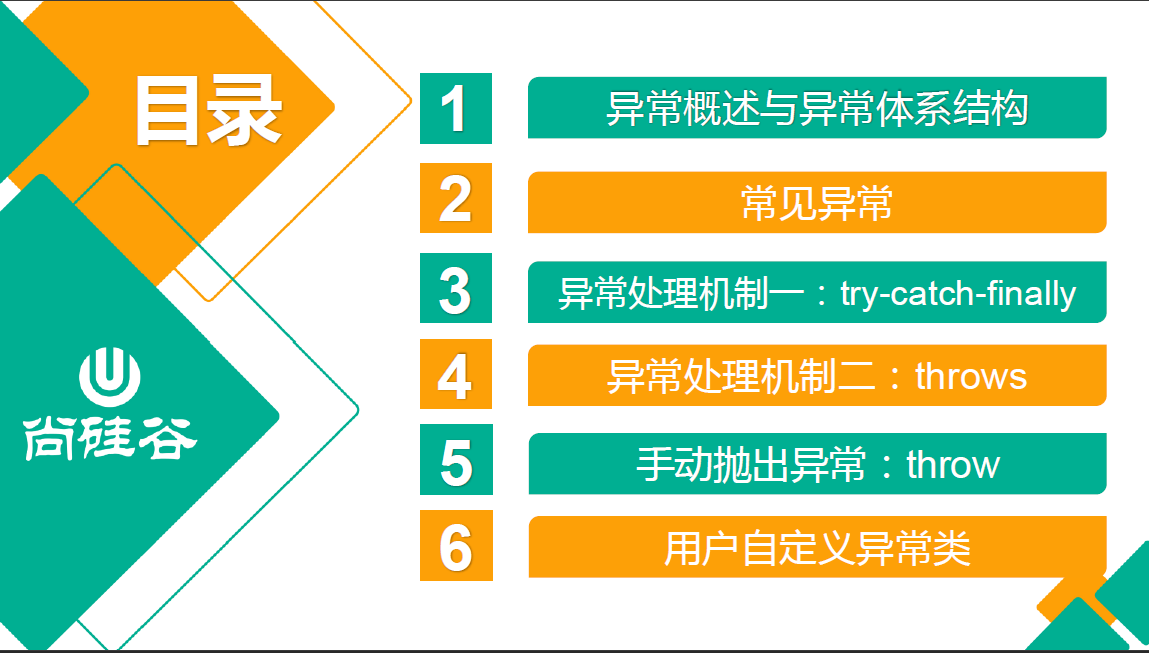
7.1 异常概述与异常体系结构
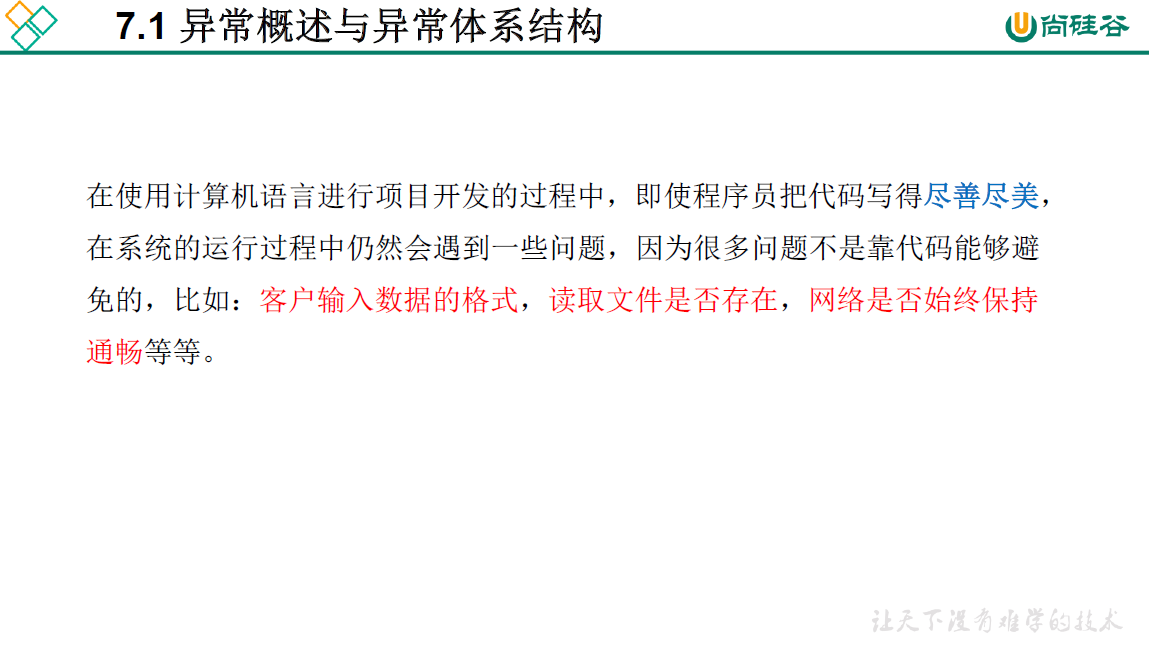
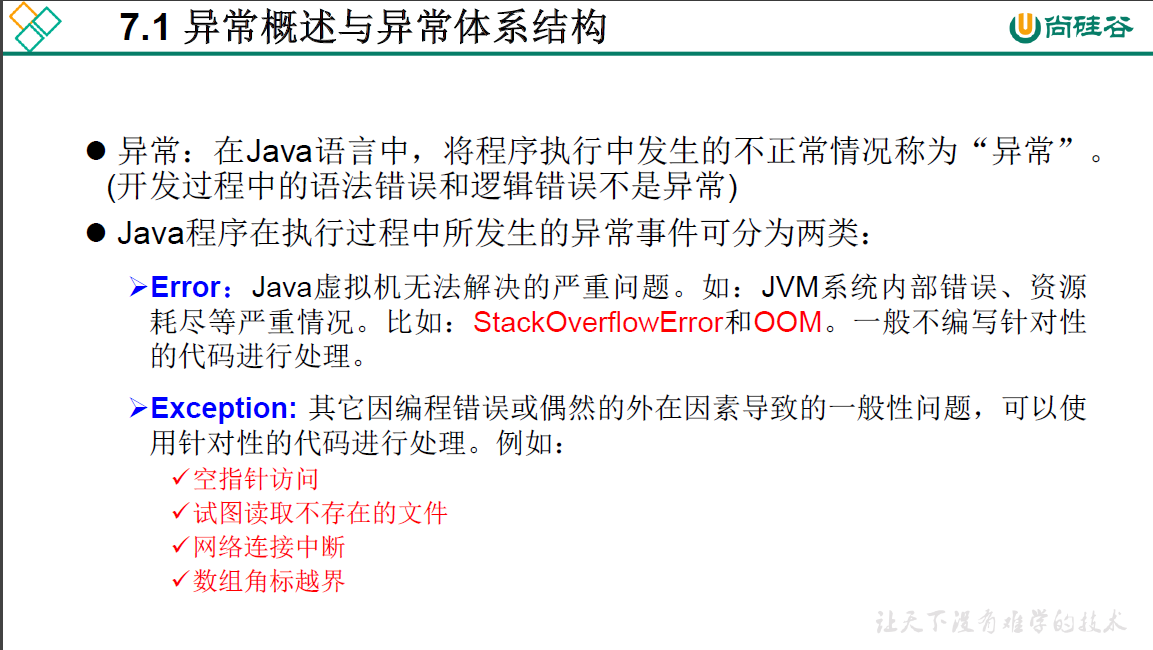
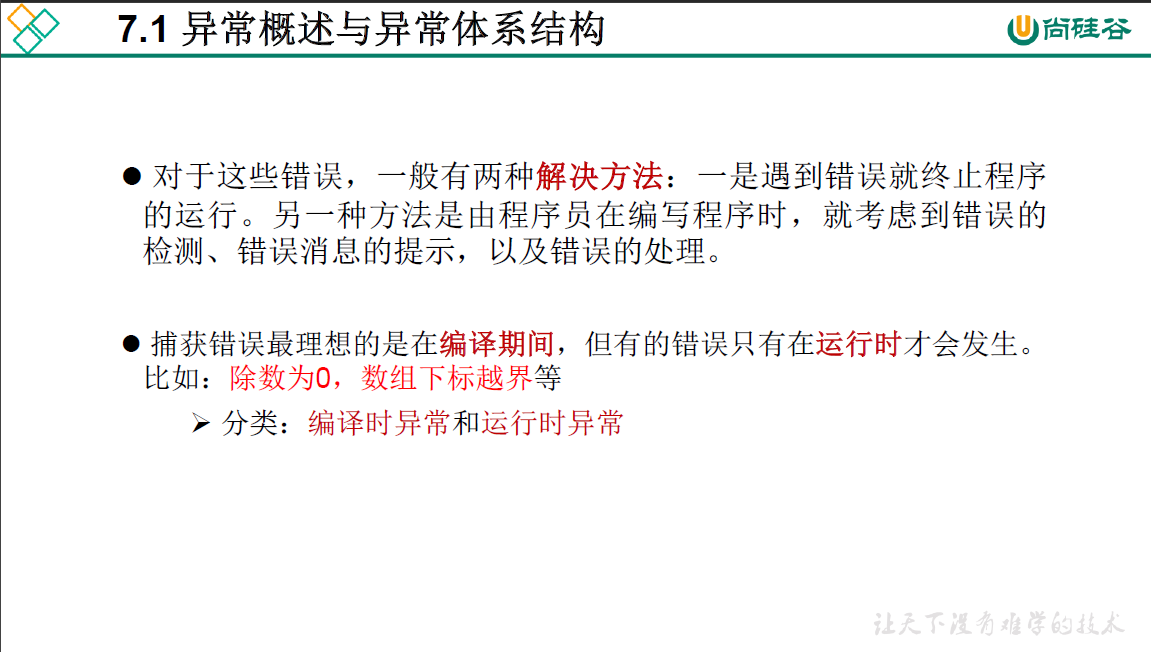
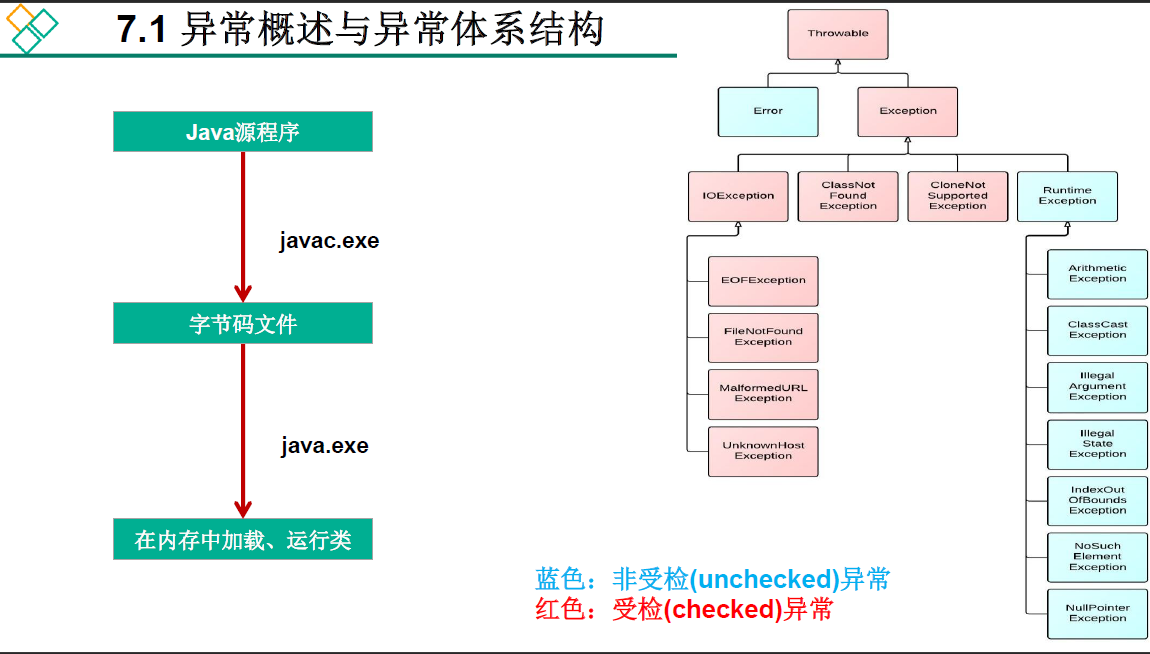
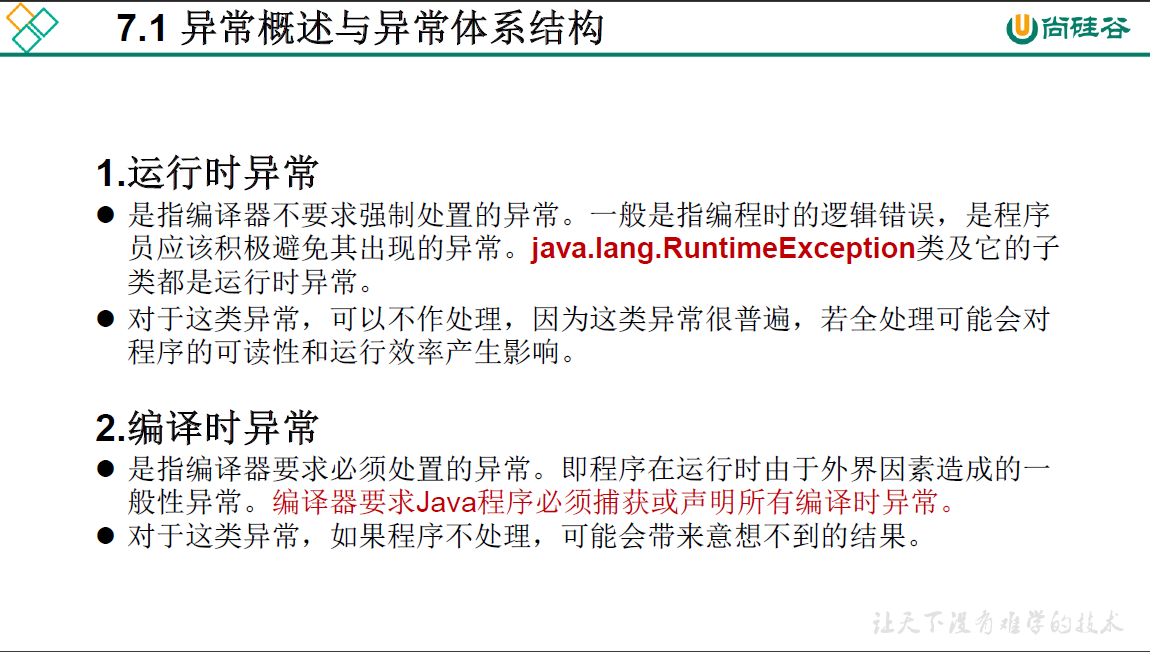
7.2 常见异常
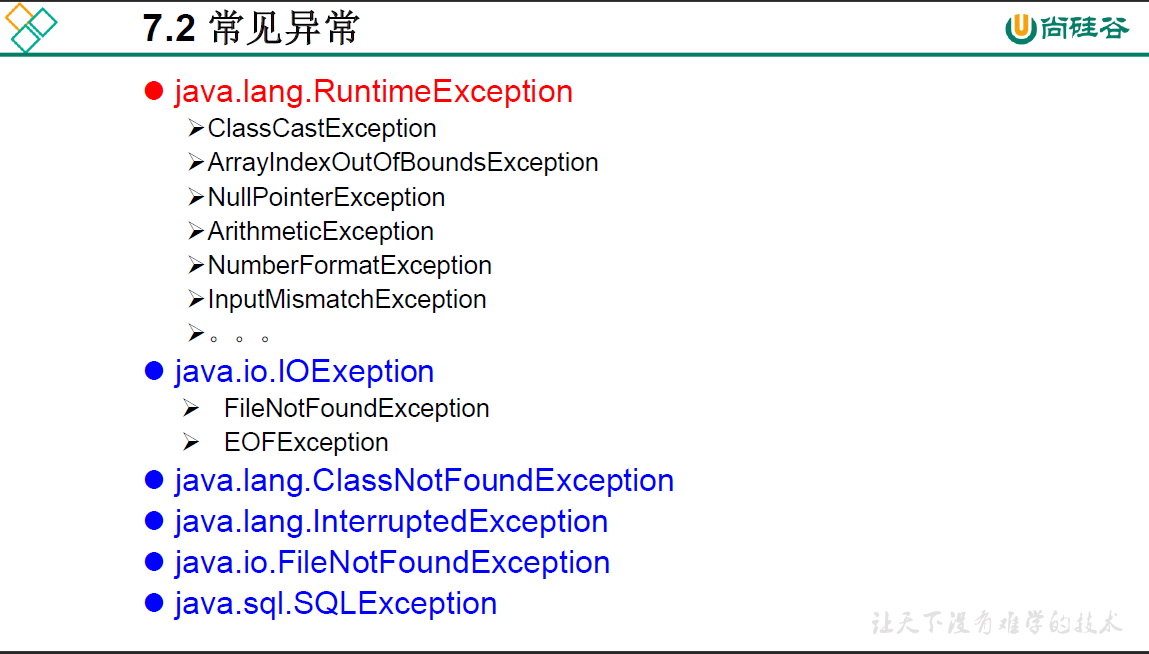
package exception.study;
/*
* java.lang.Throwable
* |-----java.lang.Error: 一般不编写针对性的代码进行处理。
* |-----java.lang.Exception: 可以进行异常的处理
* |-----编译时异常(checked)
* |-----IOException
* |-----FileNotFoundException
* |-----ClassNotFoundException
* |-----运行时异常(unchecked)
* |-----NullPointerException
* |-----ArrayIndexOutOfBoundsException
* |-----ClassCastException
* |-----NumberFormatException
* |-----InputMismatchException
* |-----ArithmeticException
*
*/
import java.io.FileInputStream;
import java.util.Date;
import java.util.Scanner;
import javax.imageio.stream.FileImageInputStream;
import org.junit.Test;
//***************常见的异常************************
public class ExceptionTest {
//运行时异常
//NullPointerException
@Test
public void test1() {
String str = null;
System.out.println(str.charAt(3));
}
//ArrayIndexOutOfBoundsException
@Test
public void test2() {
int[] arr = new int[10];
System.out.println(arr[10]);
String str = "abc";
System.out.println(str.charAt(3));
}
//ClassCastException
@Test
public void test3() {
Object obj = new Date();
String str = (String)obj;
}
//NumberFormatException
@Test
public void test4() {
String str = "abc";
int num = Integer.parseInt(str);
System.out.println(num);
}
//InputMismatchException
@Test
public void test5() {
Scanner sc = new Scanner(System.in);
int score = sc.nextInt();
System.out.println(score);
}
//ArithmeticException
@Test
public void test6() {
int a = 10;
int b = 0;
System.out.println(a / b);
}
//编译时异常
//FileNotFoundException
@Test
public void test7() {
// File file = new File("hello.txt");
// FileInputStream fis = new FileImageInputStream(file);
// int data = fis.read();
// while (data != -1) {
// System.out.println((char)data);
// data = fis.read();
// }
}
}
7.3 异常处理机制一:try-catch-finally
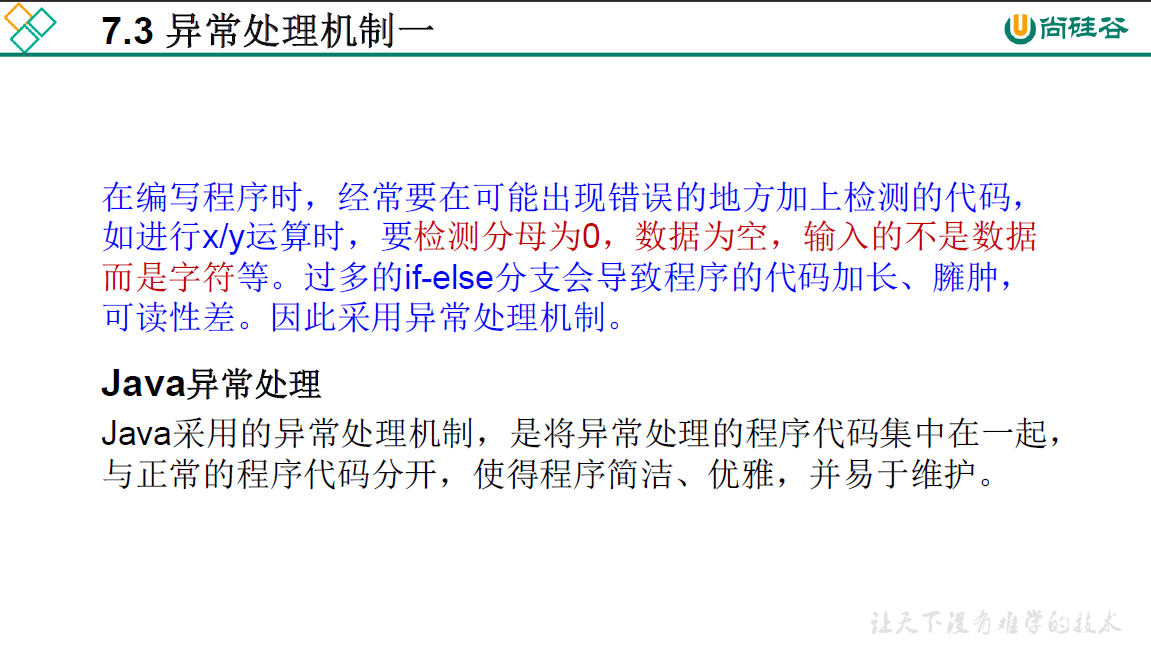
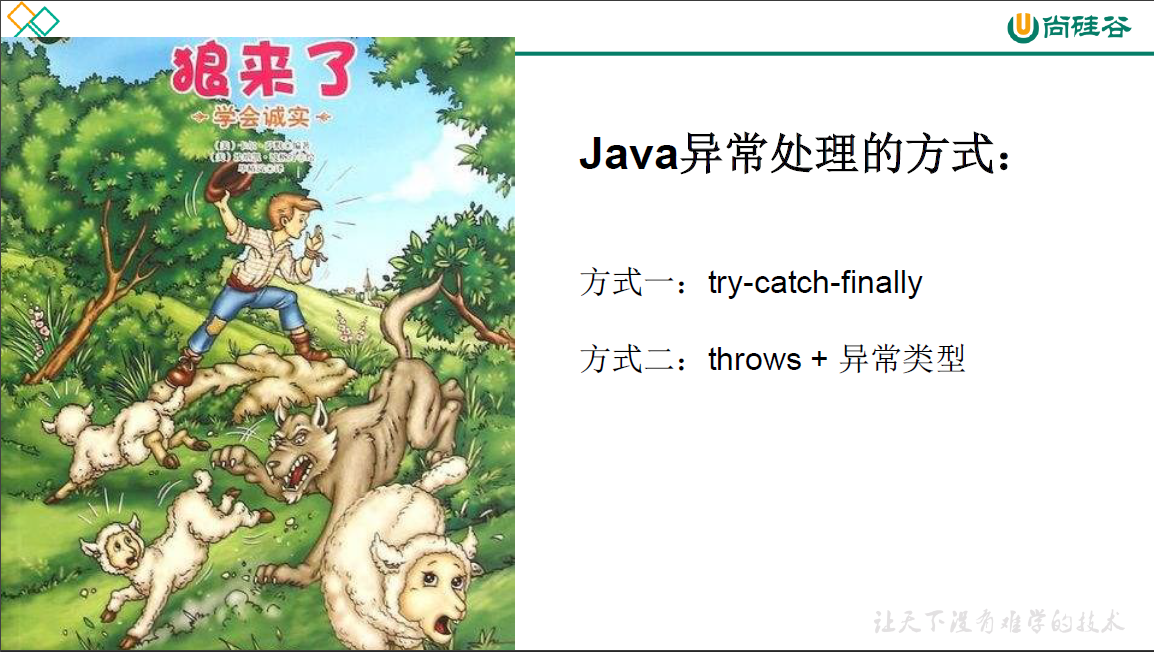
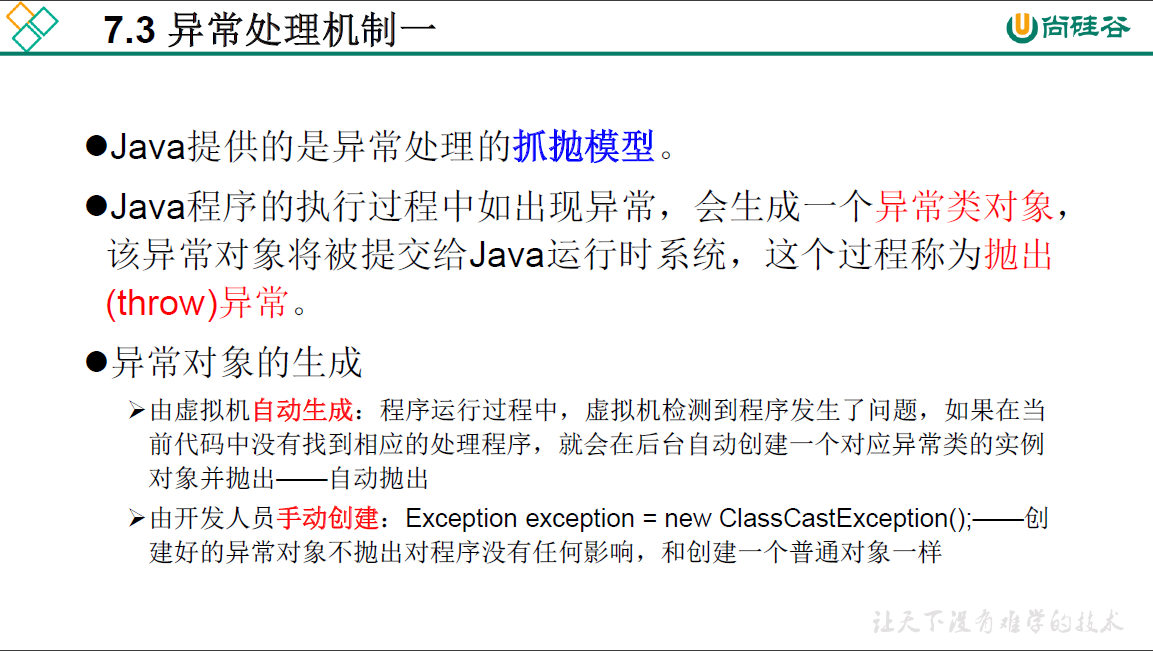
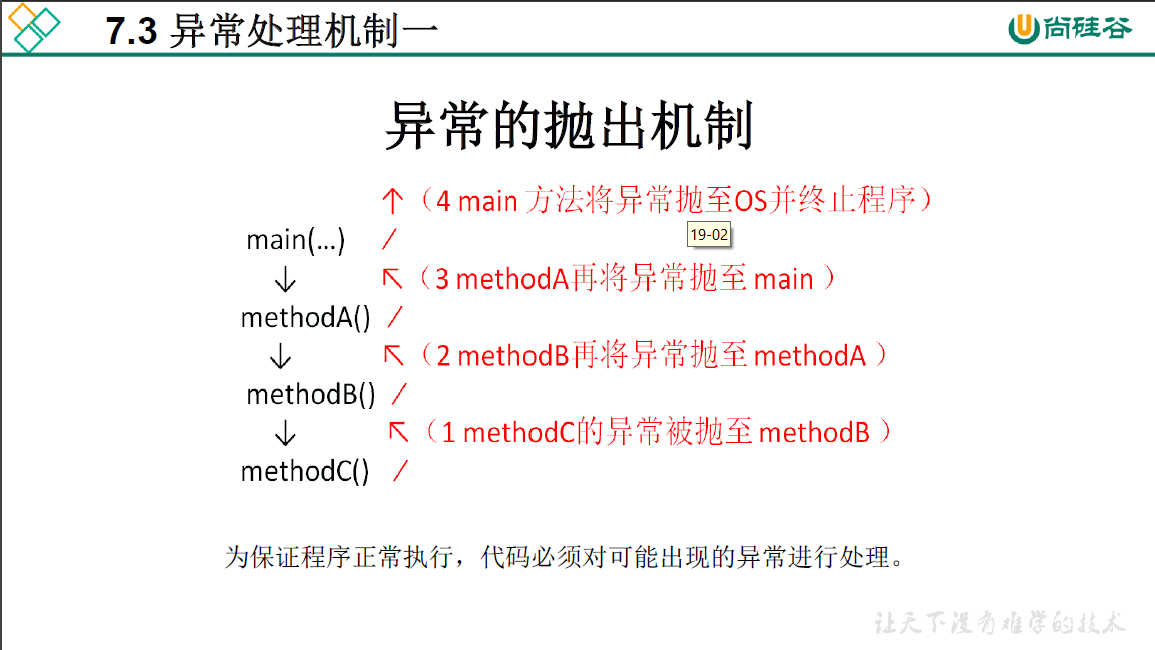
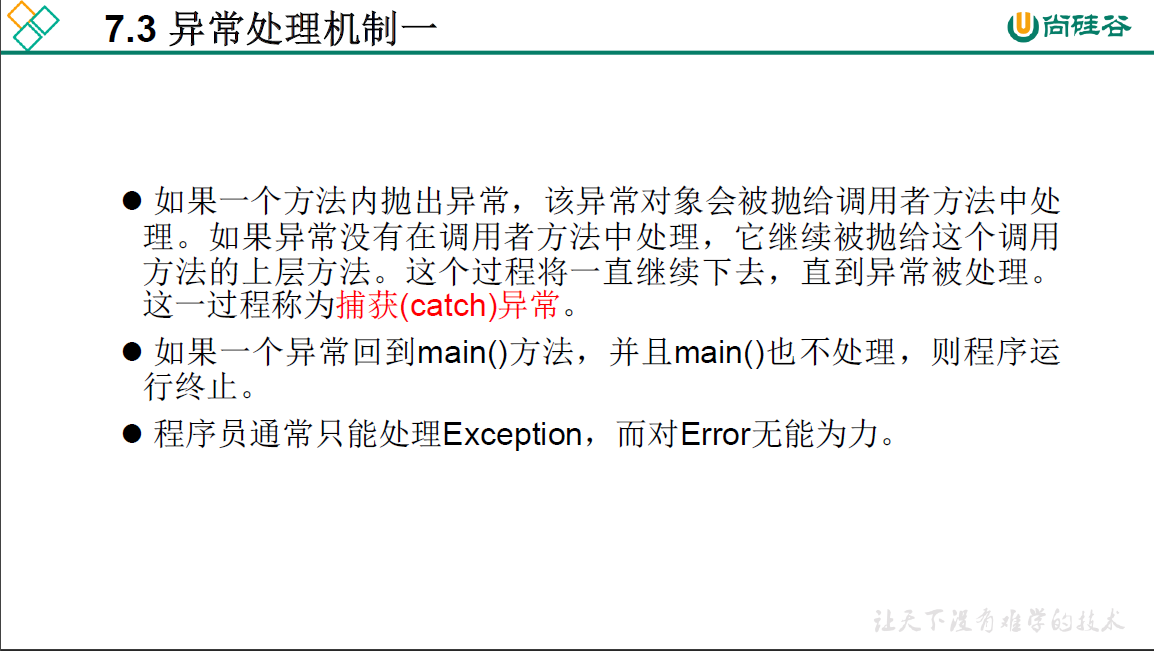
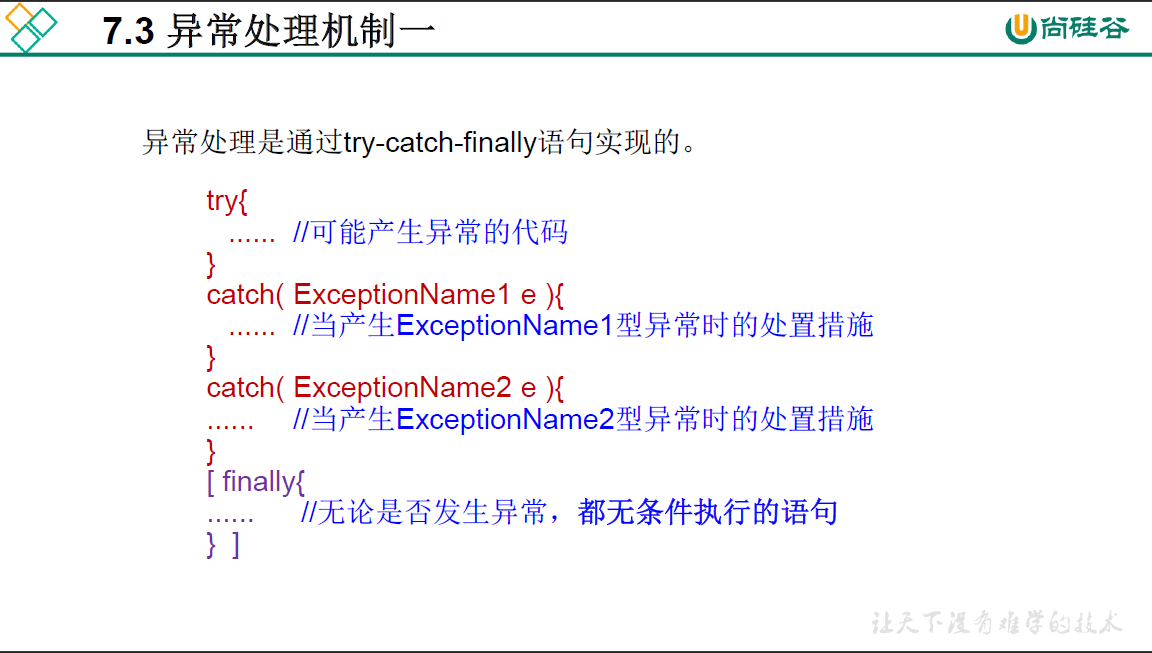
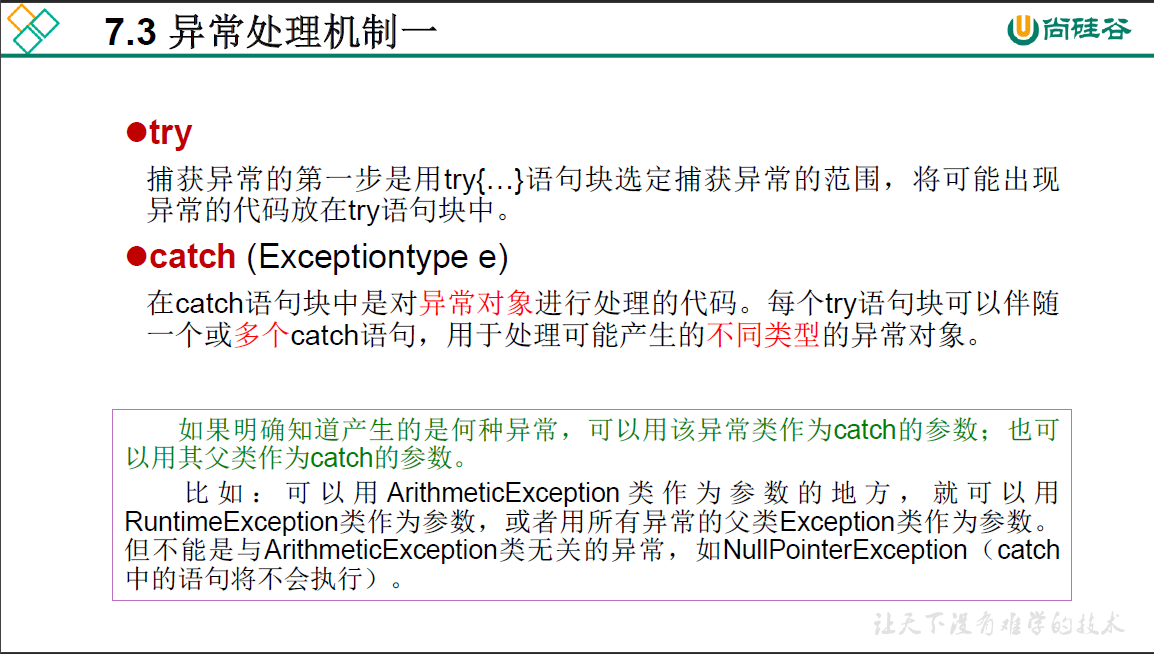
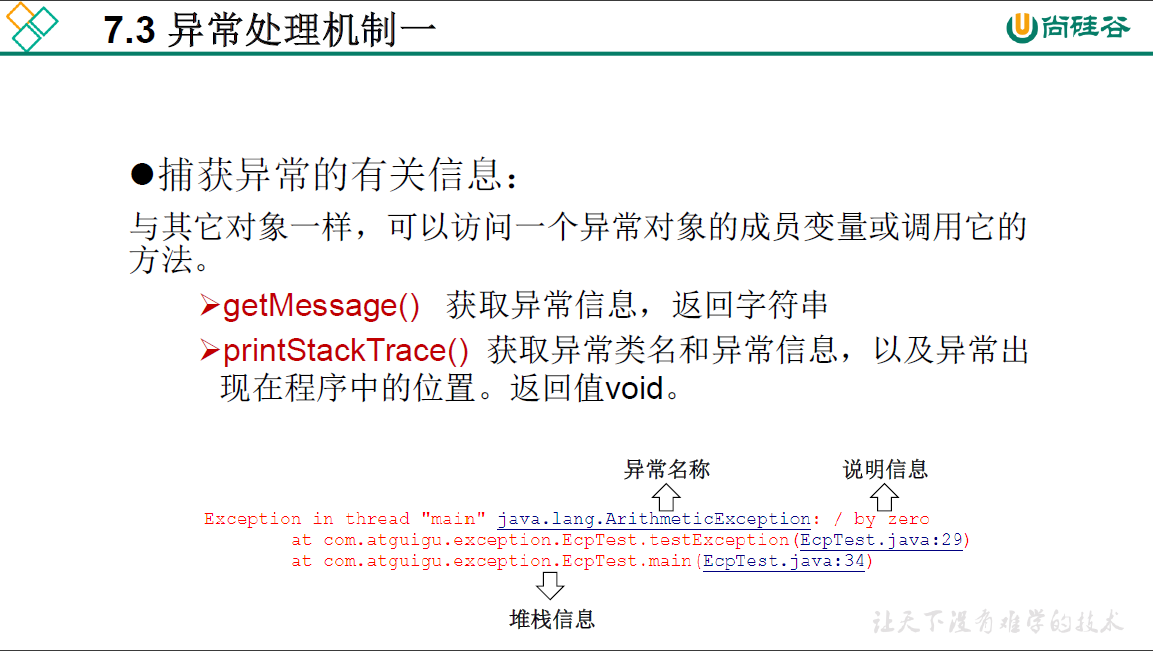
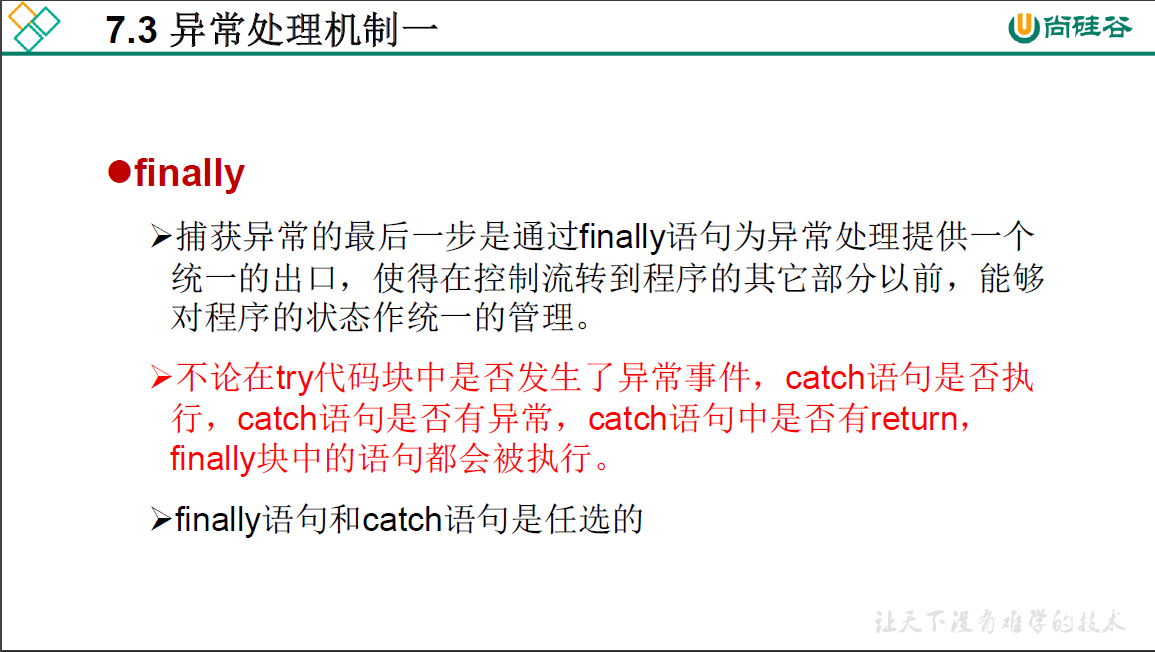
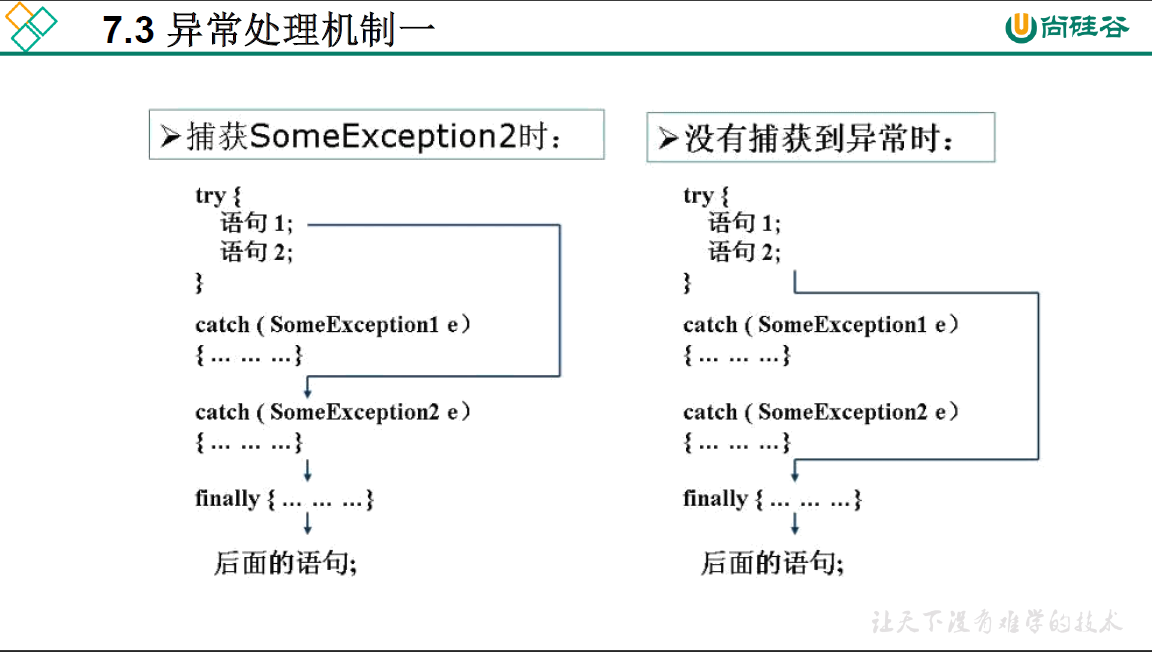
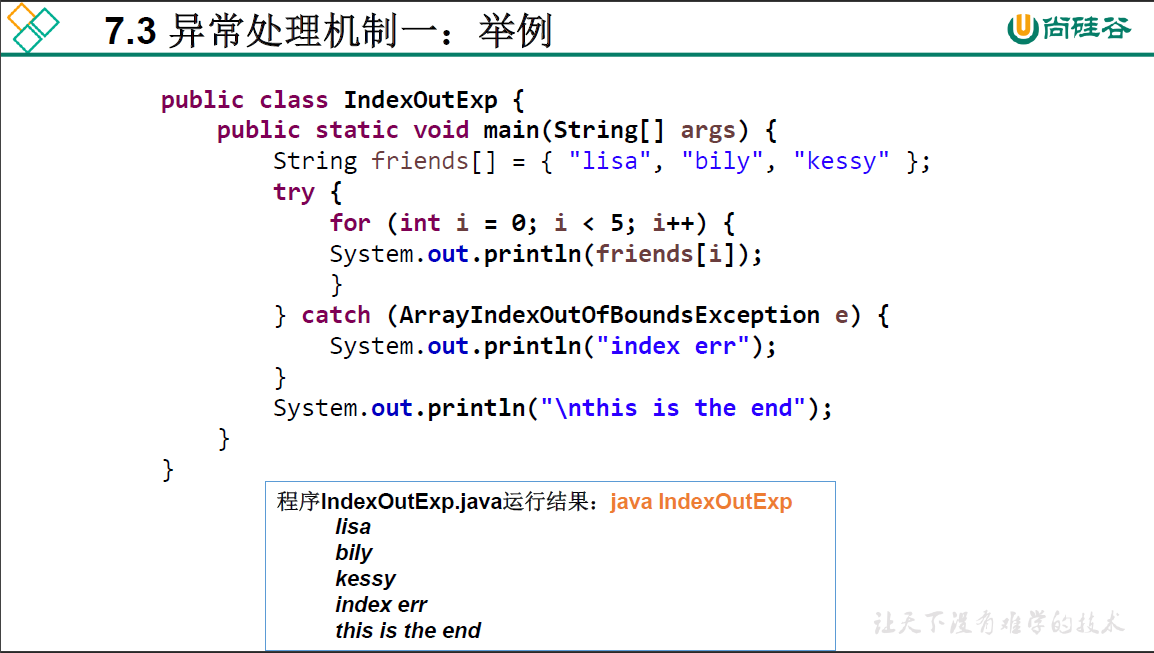
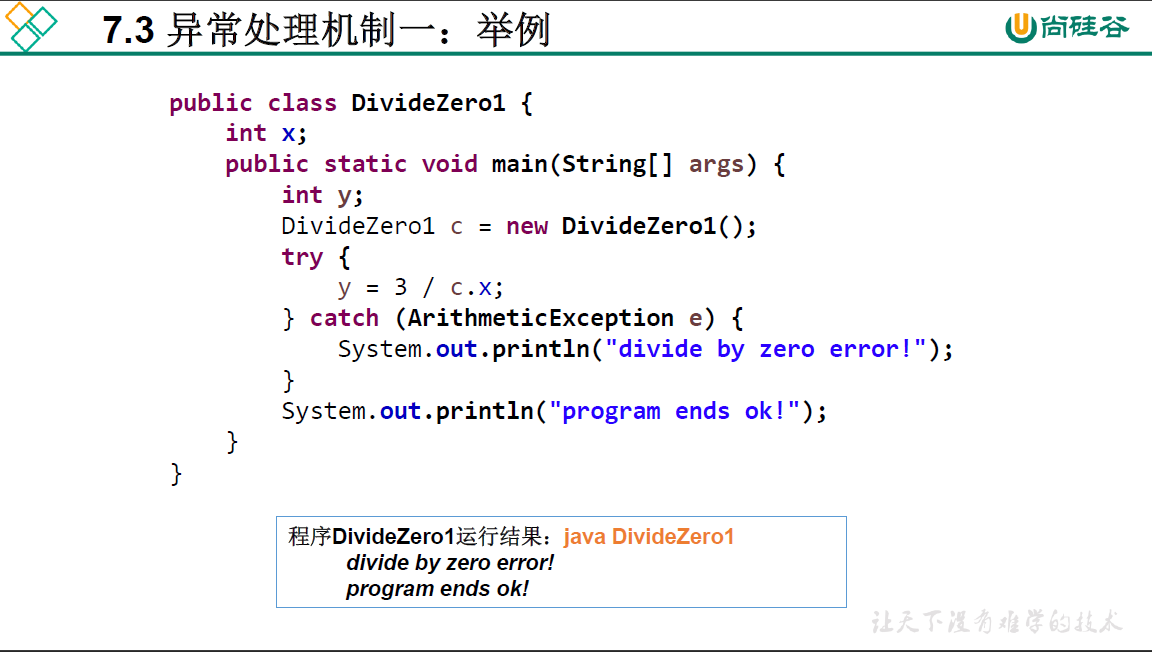
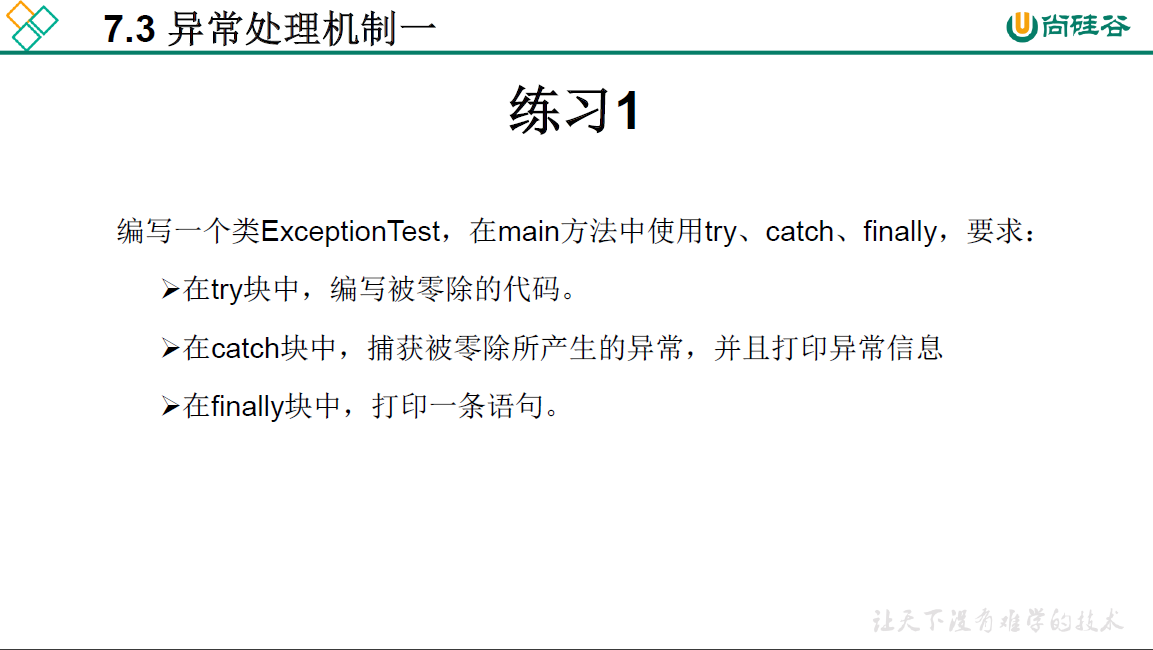
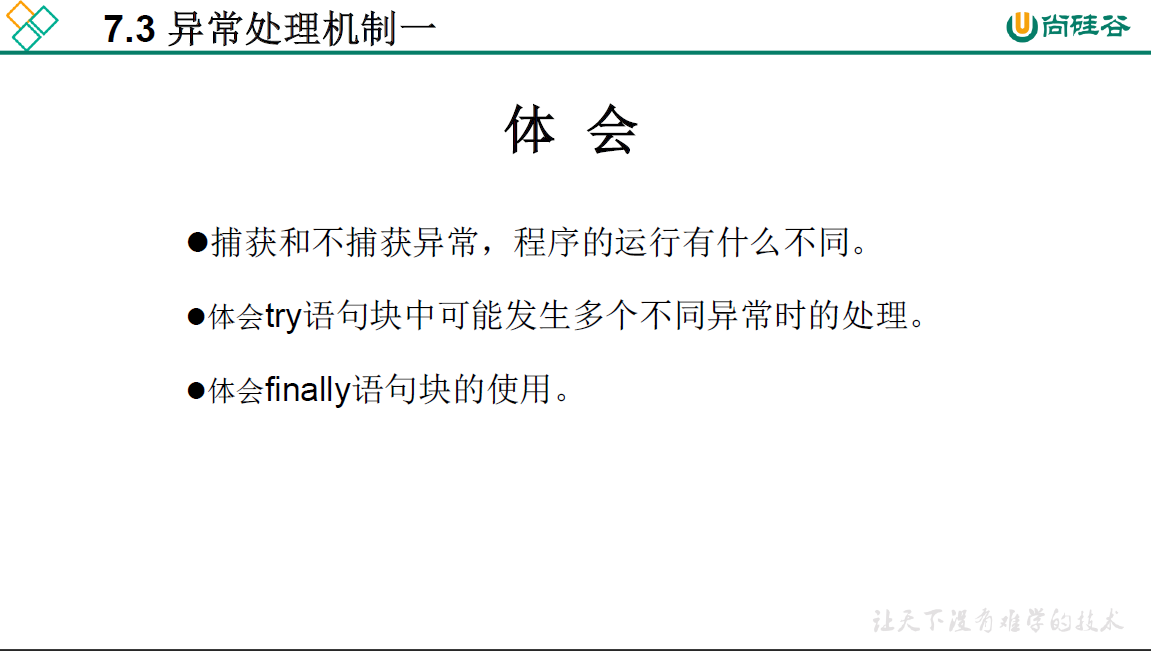
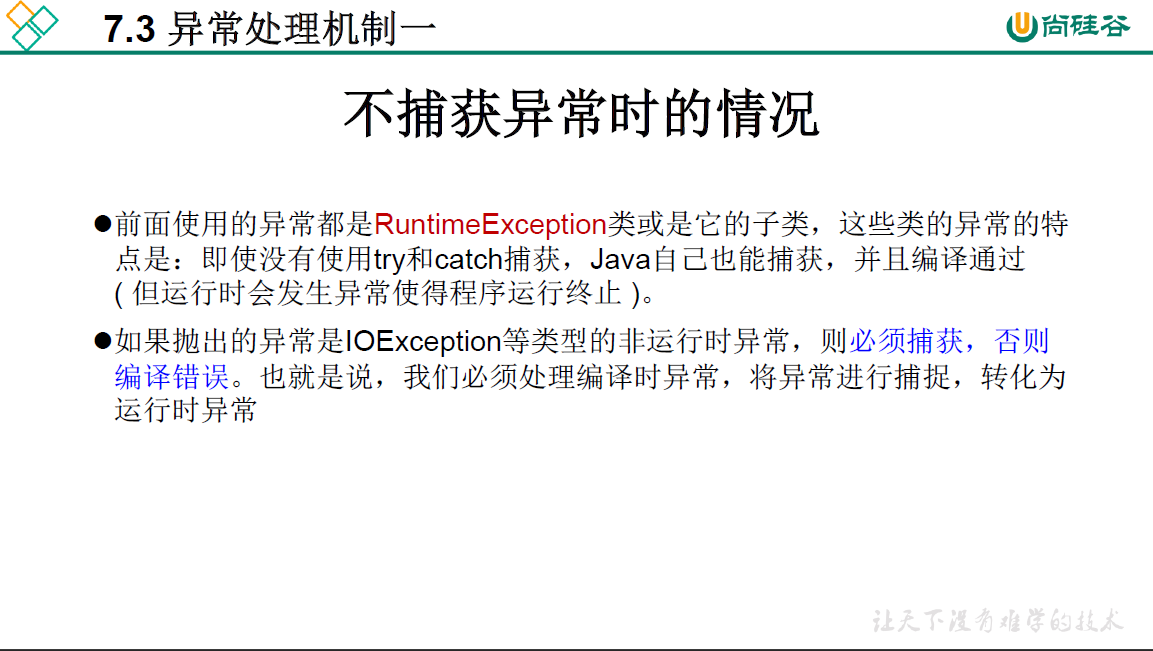
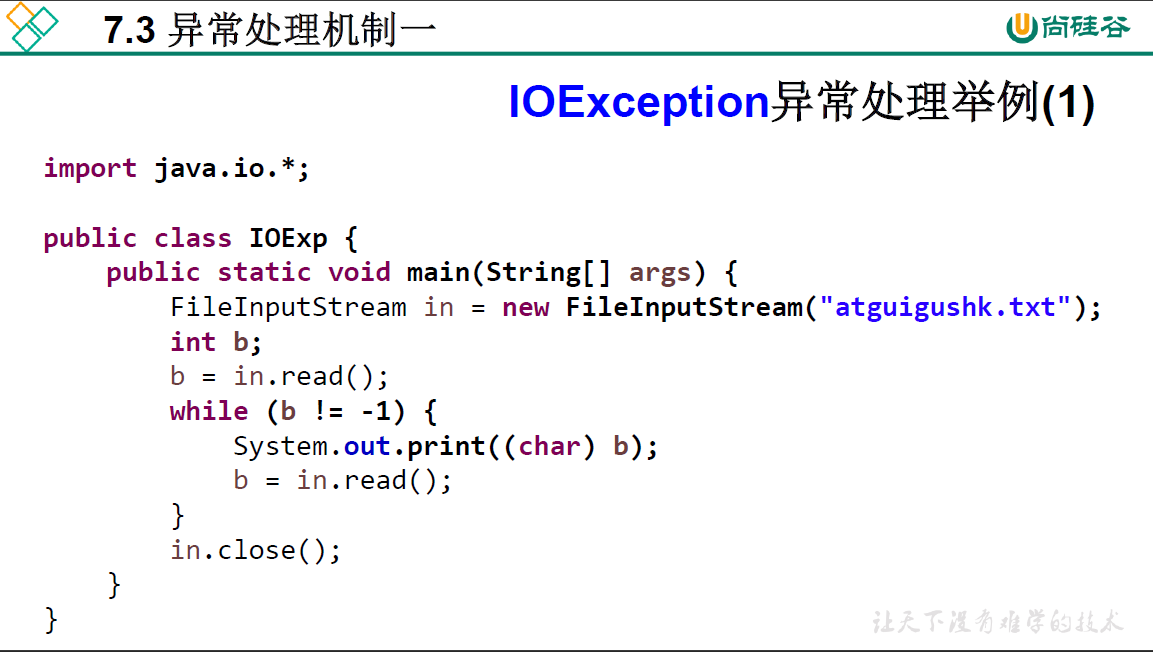
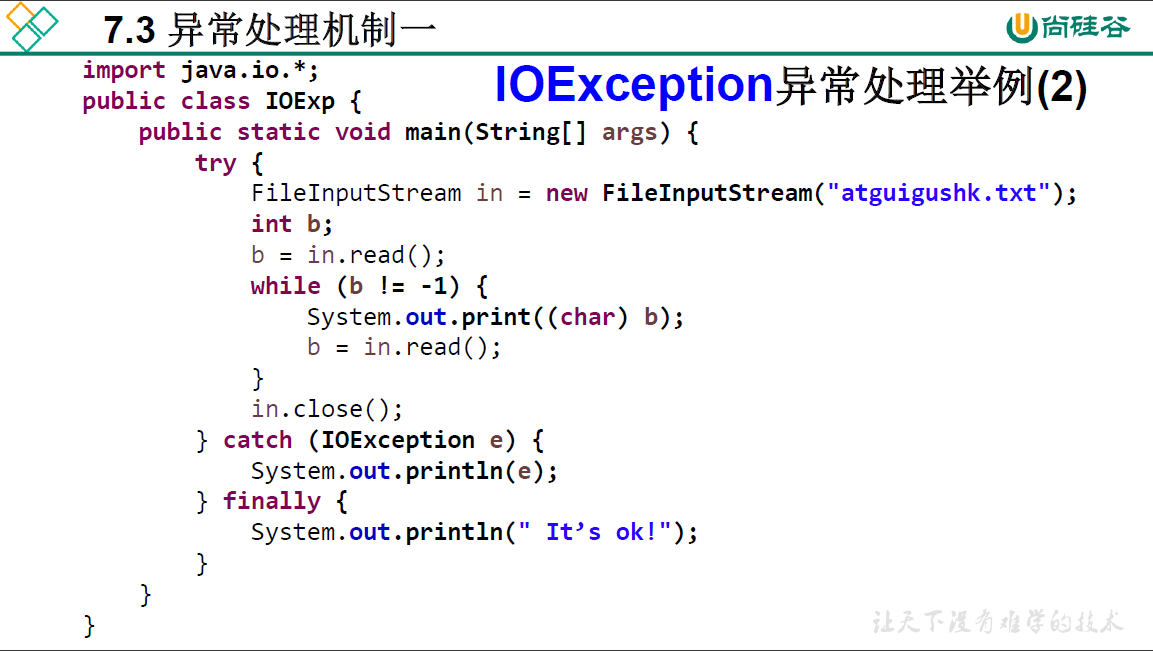
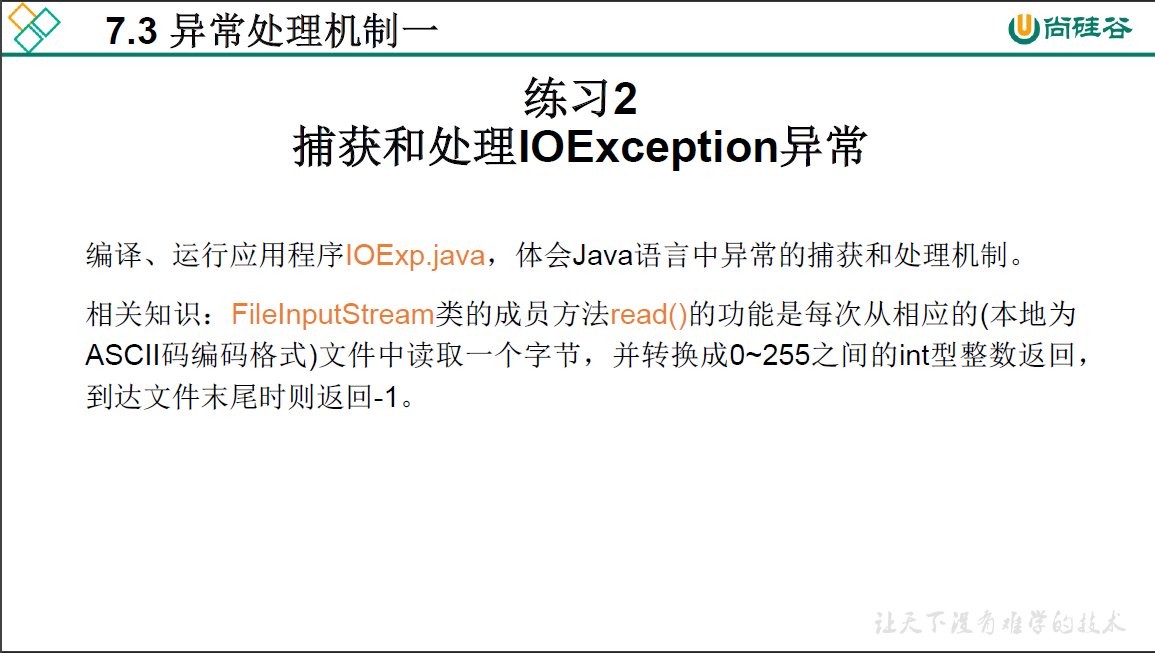
package exception.study;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import org.junit.Test;
/*
* 一、异常的处理:抓抛模型
* 过程一: "抛": 程序在正常执行的过程中,一旦出现异常,就会在异常代码处生成一个对应异常类的对象。并将此对象抛出。
* 一旦抛出对象以后,其后的代码就不再执行。
* 过程二: "抓": 可以理解为异常的处理方式: try-catch-finally throws
*
* 二、try-catch-finally的使用
*
* try{
* //可能出现异常的代码
* }catch(异常类型1 变量名1){
* //异常处理的方式1
* }catch(异常类型2 变量名2){
* //异常处理的方式2
* }catch(异常类型3 变量名3){
* //异常处理的方式3
* }
* ......
* finally{
* //一定会执行的代码
* }
*
* 说明:
* 1. finally是可选的,不一定非要写。
* 2.使用try将可能出现异常代码包装起来,在执行过程中,一旦出现异常,就会生成一个对应异常类的对象,根据此对彭的类型,去catch中进行匹配
* 3.一旦try中的异常对象匹配到某一个catch时,就进入catch中进行异常的处理。一旦处理完成,就跳出当前的try-catch结构(在没有写finally的情况)。继续执行其后的代码
* 4. catch中的异常类型如果没有子父类关系,则谁声明在上,谁声明在下无所谓。
* catch中的异常类型如果满足子父类关系,则要求子类一定声明在父类的上面。否则,报错
* 5. 常用的异常对象处理的方式: ① String getMessage() ② printstackTrace()
* 6. 在try结构中声明的变量,再出了try结构以后,就不能再被调用
*
* 体会1: 使用try-catch-finally处理编译时异常,是得程序在编译时就不再报错,但是运行时仍可能报错。
* 相当于我们使用try-catch-finally将一个编译时可能出现的异常,延迟到运行时出现。
* 体会2: 开发中,由于运行时异常比较常见,所以我们通常就不针对运行时异常编写try-catch-finally了。
* 针对于编译时异常,我们一定要考虑异常的处理。
*
*/
public class ExceptionTest1 {
//运行时异常
//NumberFormatException
@Test
public void test1() {
String str = "abc";
try {
int num = Integer.parseInt(str); // 在try结构中声明的变量num,再出了try结构以后,就不能再被调用
}catch(NullPointerException e) {
System.out.println("出现空指针异常");
}catch(NumberFormatException e){
// System.out.println("出现数值转换异常");
System.out.println(e.getMessage()); //常用的异常对象处理的方式1
e.printStackTrace(); //常用的异常对象处理的方式2
}catch(Exception e) {
System.out.println("出现异常");
}
System.out.println("hello");
}
//编译时异常
//FileNotFoundException
@Test
public void test2() {
try {
File file = new File("hello.txt");
FileInputStream fis = new FileInputStream(file);
int data = fis.read();
while (data != -1) {
System.out.println((char)data);
data = fis.read();
}
}catch(FileNotFoundException e) {
System.out.println(e.getMessage());
}catch(IOException e) {
System.out.println(e.getMessage());
}
System.out.println("hello world");
}
}
finally的使用
package exception.study;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import org.junit.Test;
/*
* try-catch-finally中finally的使用:
* 1. finally是可选的
* 2. finally中声明的是一定会被执行的代码。即使catch中又出现异常了,try中有return语句
* catch中有return语句等情况。
*
*/
public class FinallyTest {
@Test
public void test1() {
try {
int a = 10;
int b = 0;
System.out.println(a / b);
}catch(ArithmeticException e) {
int[] arr = new int[10];
System.out.println(arr[10]);
}catch(Exception e) {
e.printStackTrace();
}finally {
System.out.println("hello finally");
}
}
@Test
public void test2() {
int num = method();
System.out.println(num);
}
@Test
public void test3() {
FileInputStream fis = null;
try {
File file = new File("hello.txt");
fis = new FileInputStream(file);
int data = fis.read();
while (data != -1) {
System.out.print((char)data);
data = fis.read();
}
}catch(FileNotFoundException e) {
System.out.println(e.getMessage());
}catch(IOException e) {
System.out.println(e.getMessage());
}finally {
try {
if (fis != null)
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public int method() {
try {
int[] arr = new int[10];
System.out.println(arr[10]);
return 1;
}catch(IndexOutOfBoundsException e) {
e.printStackTrace();
return 2;
}finally {
System.out.println("我一定会执行");
return 3;
}
}
}
7.4 异常处理机制二:throws
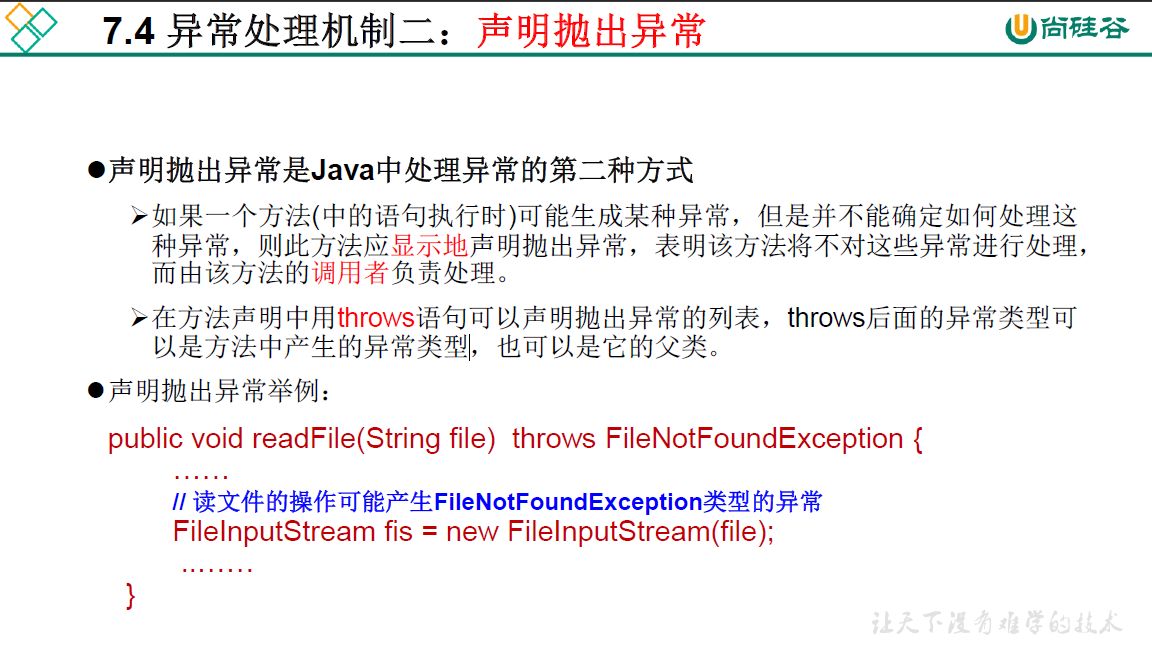
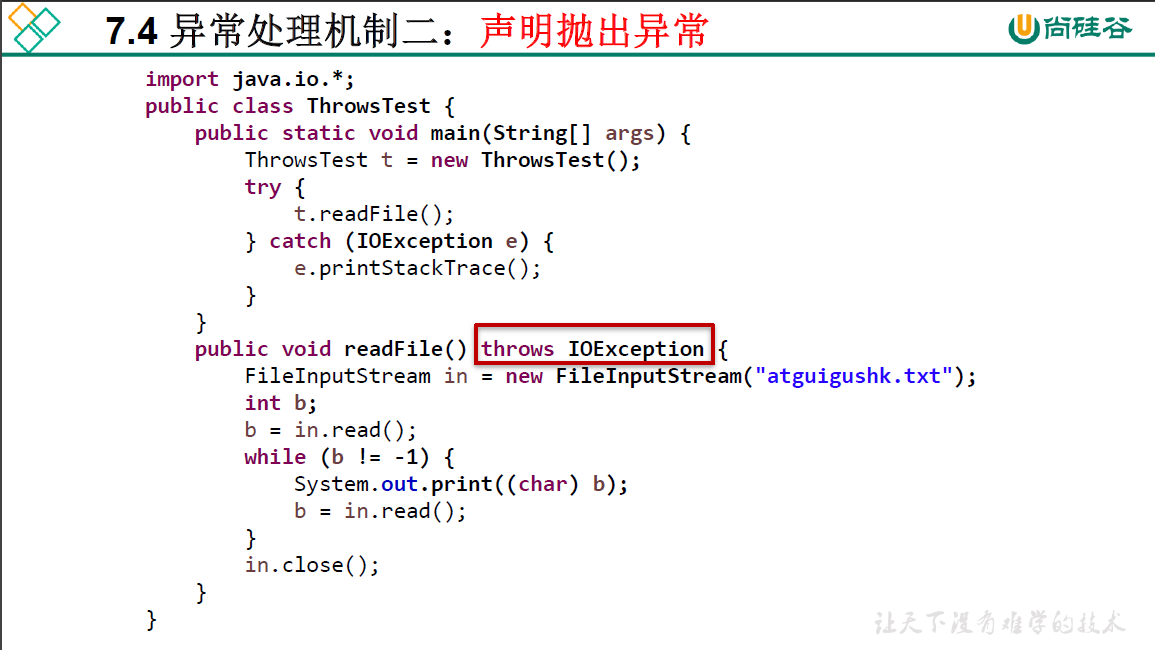
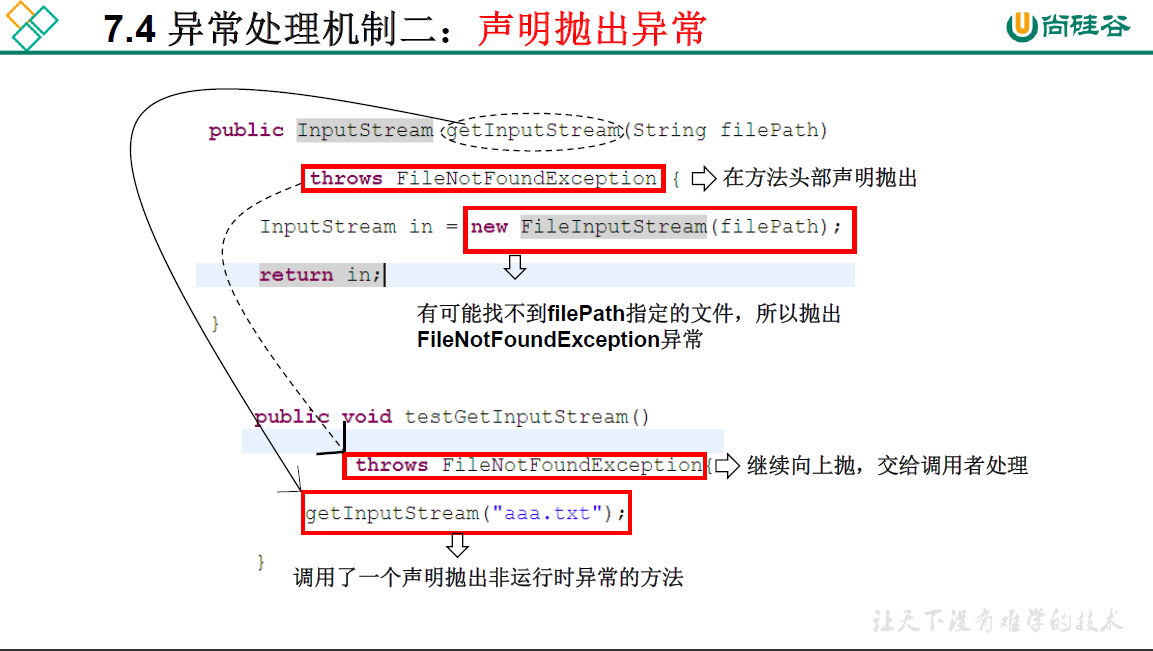
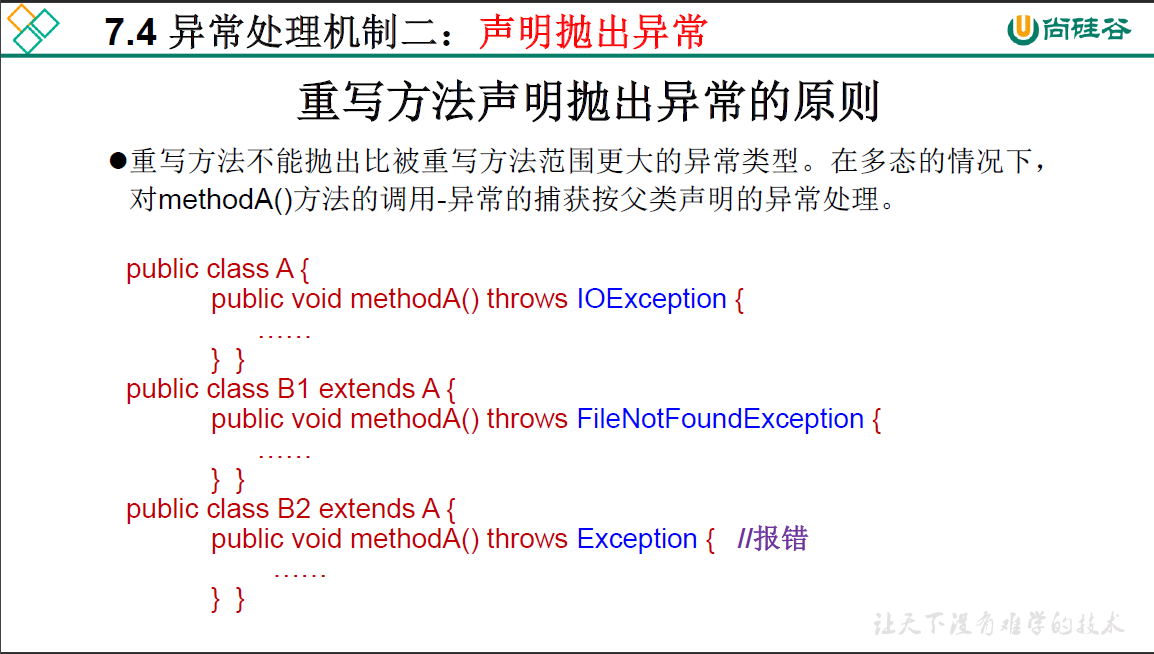
package exception.study;
/*
* 异常处理的方式二: throws + 异常类型
* 1. "throws +异常类型"写在方法的声明处。指明此方法执行时,可能会抛出的异常类型。
* 一旦当方法体执行时,出现异常,仍会在异常代码处生成一个异常类的对象,此对象满足throws后异常类型时,就会被抛出
* 异常代码后续的代码,就不再执行!
* 2.体会: try-catch-finally: 真正的将异常给处理掉了。
* throws的方式只是将异常抛给了方法的调用者。并投有真正将异常处理掉。
*/
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class ExceptionTest2 {
public static void main(String[] args) {
try {
method2();
}catch(IOException e) {
e.printStackTrace();
}
// method3();
}
public static void method3() {
try {
method2();
}catch(IOException e) {
e.printStackTrace();
}
}
public static void method2() throws IOException{
method1();
}
public static void method1() throws FileNotFoundException, IOException{
File file = new File("hello1.txt");
FileInputStream fis = new FileInputStream(file);
int data = fis.read();
while (data != -1) {
System.out.print((char)data);
data = fis.read();
}
fis.close();
}
}
package exception.study;
/*
* 方法重写的规则之一:
* 子类重写的方法抛出的异常类型不大于父类被重写的方法抛出的异常类型
*
* 3.开发中如何选择使用try-catch-finally 还是使用throws?
* 3.1 如果父类中被重写的方法没有throws方式处理异常,则子类重写的方法也不能使用throws,意味着如果
* 子类重写的方法中有异常,必须使用try-catch-finally方式处理。
* 3.2 执行的方法a中,先后又调用了另外的几个方法,这几个方法是递进关系执行的。我们建议这几个方法使用throw的方式进行处理。
* 而执行的方法a可以考虑使用try-catch-finally方式进行处理。
*
*/
import java.io.FileNotFoundException;
import java.io.IOException;
public class OverrideTest {
public static void main(String[] args) {
OverrideTest t = new OverrideTest();
t.display(new SubClass());
}
public void display(SuperClass s) {
try {
s.show();
} catch (IOException e) {
e.printStackTrace();
}
}
}
class SuperClass{
public void show() throws IOException{
System.out.println("SperClass Exception");
}
}
class SubClass extends SuperClass{
public void show() throws FileNotFoundException{
System.out.println("SubClass Exception");
}
}
7.5 手动抛出异常
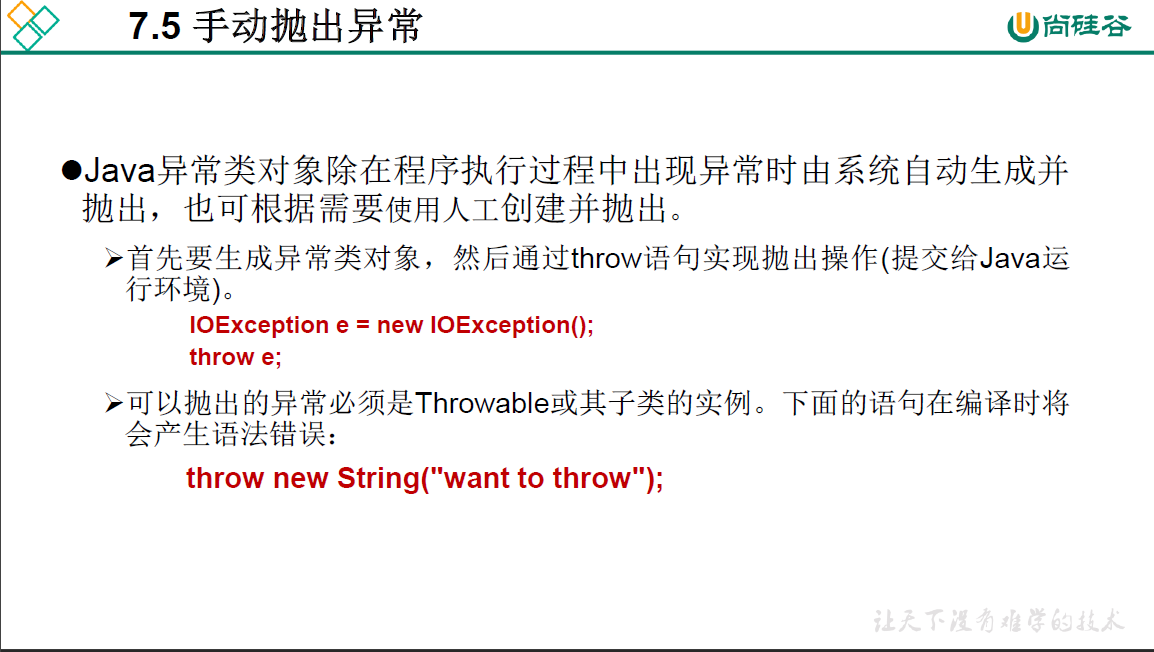
package exception.study;
/*
* 手动抛出异常
*/
public class ExceptionTest3 {
public static void main(String[] args) {
try {
Student s = new Student();
s.regist(-100);
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
class Student{
private int id;
public void regist(int id) throws Exception {
if (id > 0)
this.id = id;
else {
//手动抛出异常
// throw new RuntimeException( "输入的数据非法");
throw new Exception("输入的数据非法"); //生成一个异常对象
}
}
}
7.6 用户自定义异常类
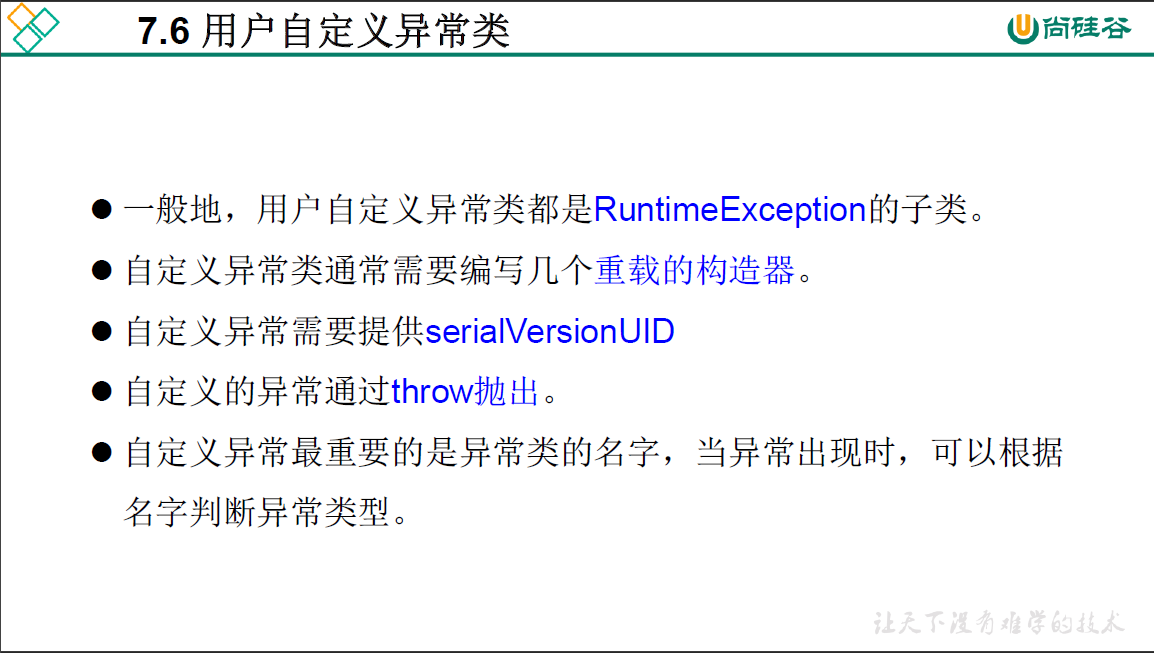
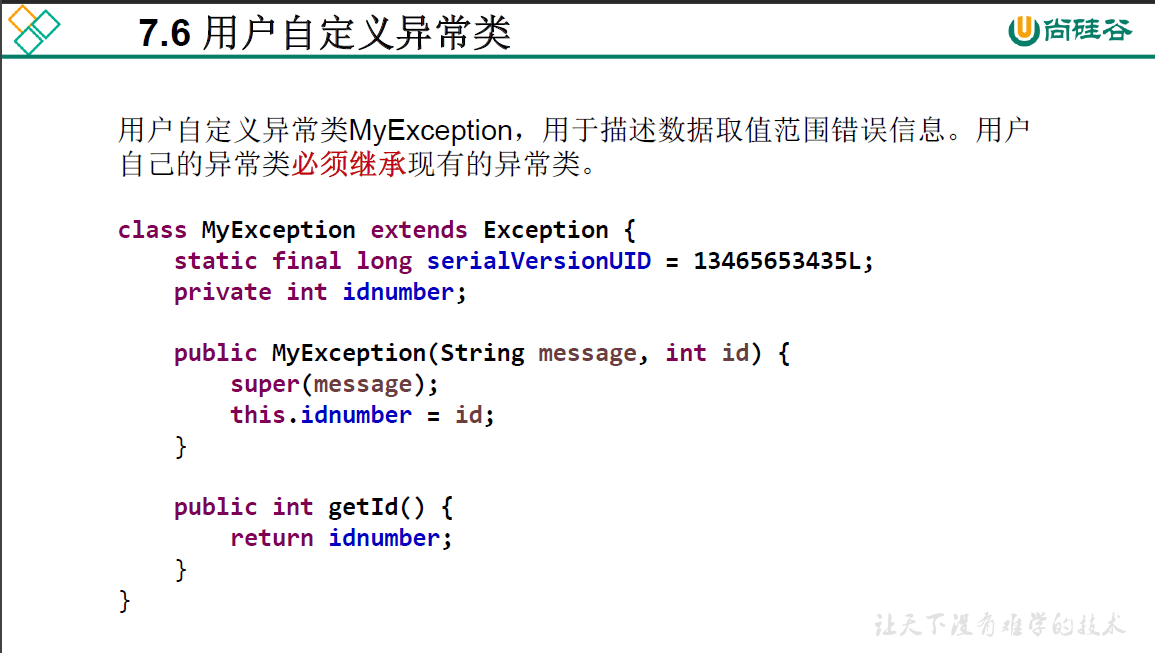
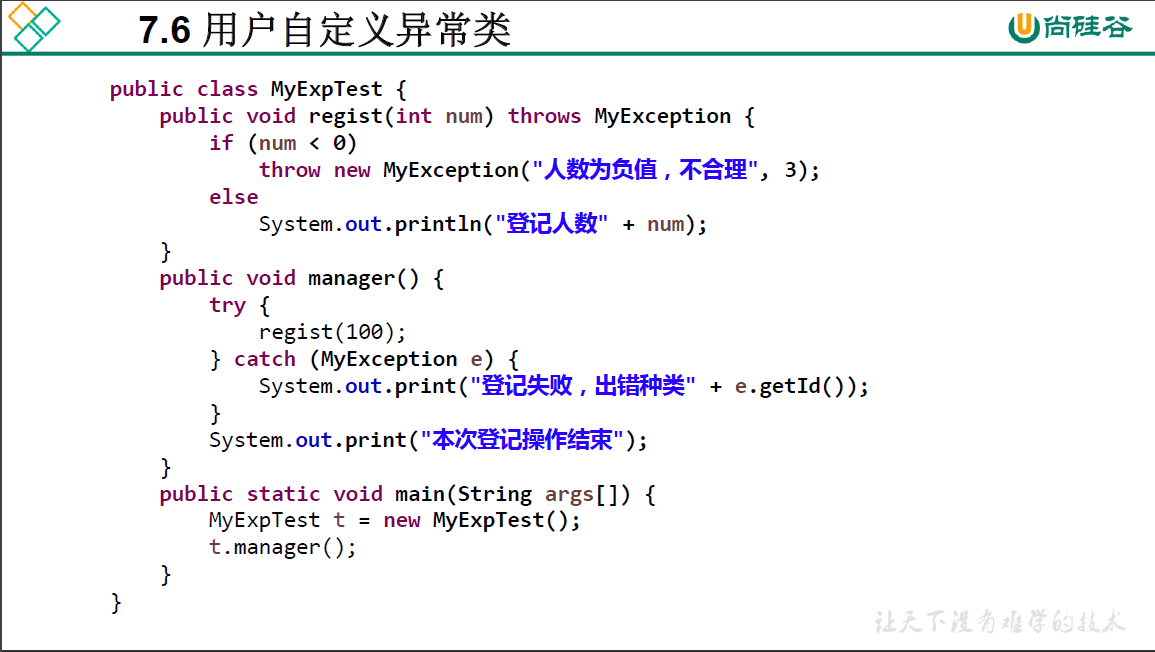
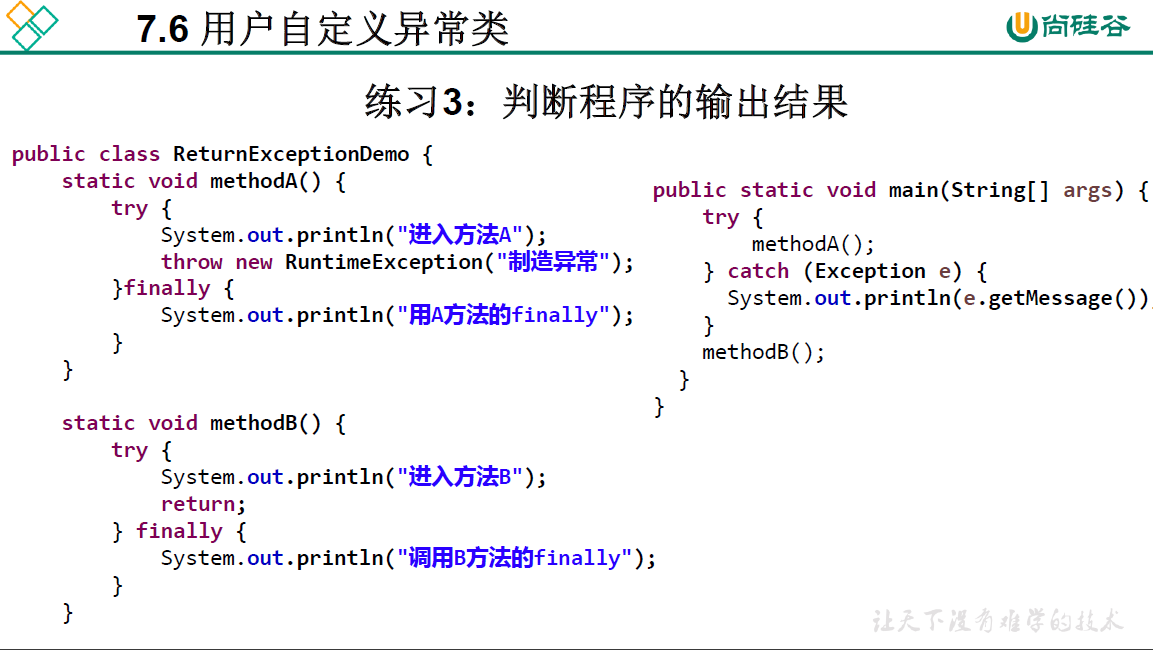
package exception.study;
public class ReturnExceptionDemo {
public static void main(String[] args) {
try {
methodA();
}catch (Exception e) {
System.out.println(e.getMessage());
}
methodB();
}
static void methodA() {
try {
System.out.println("进入方法A");
throw new RuntimeException("制造异常");
}finally {
System.out.println("用A方法的finally");
}
}
static void methodB() {
try {
System.out.println("进入方法B");
return;
}finally {
System.out.println("用B方法的finally");
}
}
}
//输出结果
进入方法A
用A方法的finally
制造异常
进入方法B
用B方法的finally
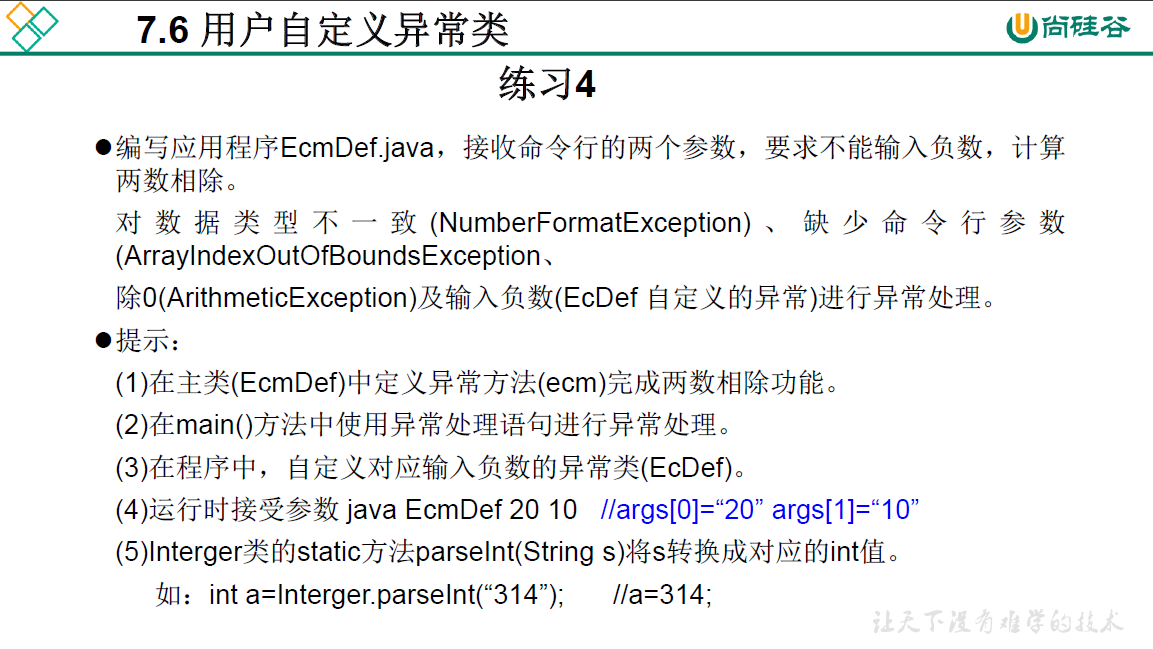
练习4
package exception.study;
public class EcmDef {
public static void main(String[] args) {
try {
int i = Integer.parseInt(args[0]);
int j = Integer.parseInt(args[1]);
double result = ecm(i, j);
System.out.println(result);
}catch (NumberFormatException e) {
System.out.println("数据格式不匹配");
}catch (ArrayIndexOutOfBoundsException e) {
System.out.println("参数个数不匹配");
}catch (ArithmeticException e) {
System.out.println("除0异常");
}catch (EcDef e) {
System.out.println(e.getMessage());
}
}
public static double ecm(int i, int j) throws EcDef {
if (i < 0 || j < 0) {
throw new EcDef("分子或分母为负数");
}
return i / j;
}
}
class EcDef extends Exception{
static final long serialVersionNID = -223334456578934L;
public EcDef() {
}
public EcDef(String msg) {
super(msg);
}
}
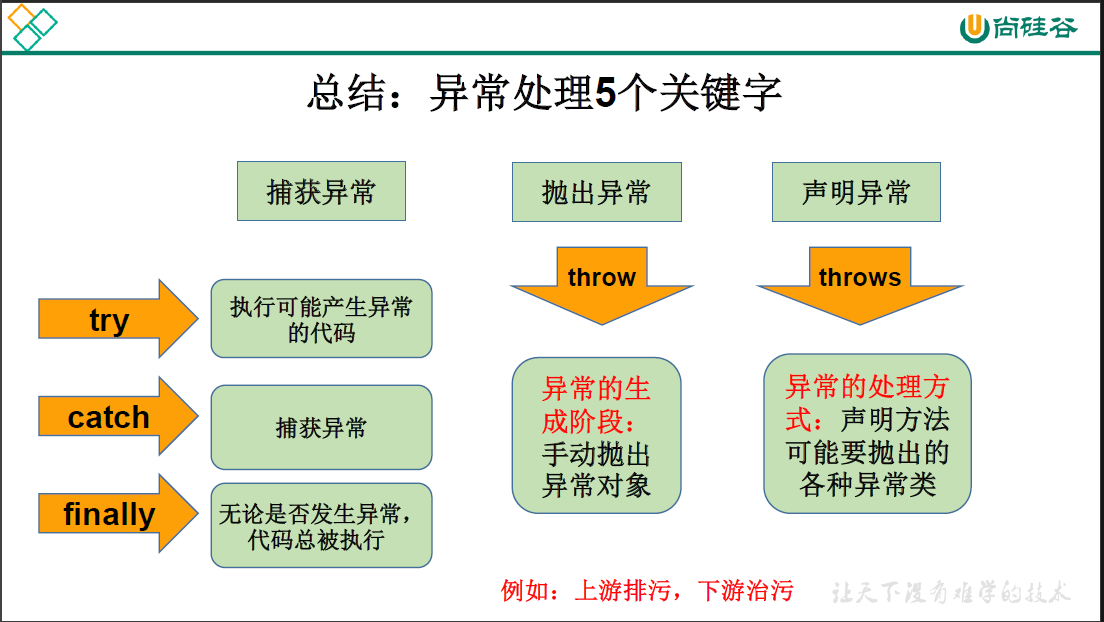
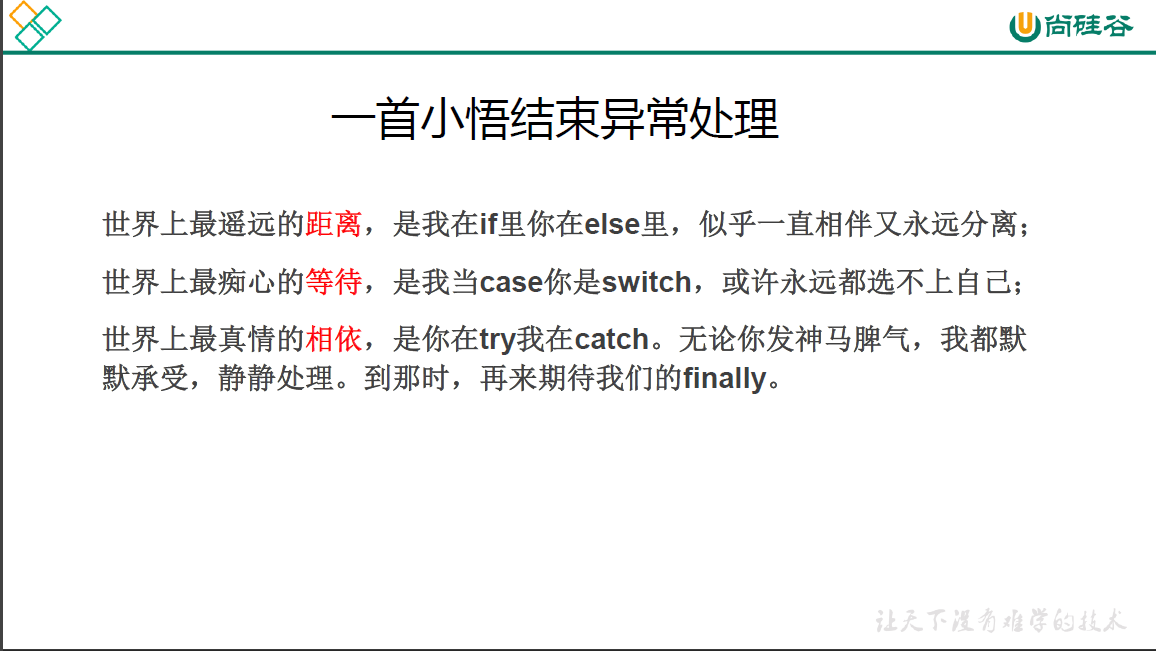