Description: Given the root
of a binary tree, imagine yourself standing on the right side of it, return the values of the nodes you can see ordered from top to bottom.
Link: 199. Binary Tree Right Side View
Examples:
Example 1:
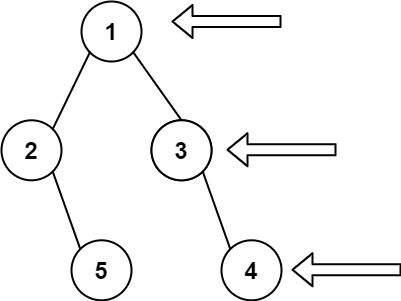
Input: root = [1,2,3,null,5,null,4] Output: [1,3,4] Example 2: Input: root = [1,null,3] Output: [1,3] Example 3: Input: root = [] Output: []
思路: 逐层遍历,取每层最后一个元素。所以逐层遍历既可以用DFS, 也可以用BFS。
class Solution(object): def rightSideView(self, root): """ :type root: TreeNode :rtype: List[int] """ if not root: return [] res = [] self.dfs(root, 0, res) return [l[-1] for l in res] def dfs(self, root, level, res): if not root: return if len(res) == level: res.append([]) res[level].append(root.val) if root.left: self.dfs(root.left, level+1, res) if root.right: self.dfs(root.right, level+1, res)
BFS:
class Solution(object): def rightSideView(self, root): """ :type root: TreeNode :rtype: List[int] """ if not root: return [] res = [] que = collections.deque() que.append(root) while que: size = len(que) level = [] for _ in range(size): node = que.popleft() if not node: continue level.append(node.val) que.append(node.left) que.append(node.right) res.append(level) # print(res[:-1]) return [l[-1] for l in res[:-1]]
日期: 2021-03-26 阴天