需求
- 导入文件,查看原始数据
- 将人口数据和各州简称数据进行合并
- 将合并的数据中重复的abbreviation列进行删除
- 查看存在缺失数据的列
- 找到有哪些state/region使得state的值为NaN,进行去重操作
- 为找到的这些state/region的state项补上正确的值,从而去除掉state这一列的所有NaN
- 合并各州面积数据areas
- 我们会发现area(sq.mi)这一列有缺失数据,找出是哪些行
- 去除含有缺失数据的行
- 找出2010年的全民人口数据
- 计算各州的人口密度
- 排序,并找出人口密度最高的州
1. 导入文件,查看原始数据
import pandas as pd from pandas import Series, DataFrame import numpy as np add = pd.read_csv('./data/state-abbrevs.csv') add.head() # 数据中state为州的全称,abbreviation为州的简称

pop = pd.read_csv('./data/state-population.csv') pop.head()
area = pd.read_csv('./data/state-areas.csv') area.head()
2. 将人口数据和各州简称数据进行合并
pop_add = pd.merge(add, pop, left_on='abbreviation', right_on='state/region', how='outer') pop_add.head()
3. 将合并的数据中重复的abbreviation列进行删除
pop_add.drop(labels='abbreviation', axis=1, inplace=True)
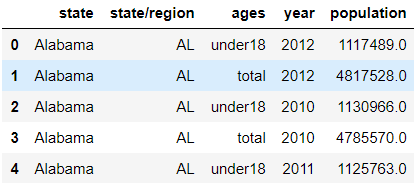
4. 查看存在缺失数据的列
pop_add.isnull().any(axis=0) state True state/region False ages False year False population True dtype: bool pop_add.info() <class 'pandas.core.frame.DataFrame'> Int64Index: 2544 entries, 0 to 2543 Data columns (total 5 columns): state 2448 non-null object state/region 2544 non-null object ages 2544 non-null object year 2544 non-null int64 population 2524 non-null float64 dtypes: float64(1), int64(1), object(3) memory usage: 119.2+ KB
补充俩个常用方法
- unique 查看存有哪些不同的元素 只能被Series调用
- value_counts 查看不同元素出现的次数 只能被Series调用
查看ages列中存有哪些不同的元素
pop_add['ages'].unique() array(['under18', 'total'], dtype=object)
查看ages列中不同元素出现的次数
pop_add['ages'].value_counts() total 1272 under18 1272 Name: ages, dtype: int64
5. 找到有哪些state/region(州简称)使得state(州全称)的值为NaN,进行去重操作
前提:由上题得知state列中存有空值数据
将state列中的空值对应的简称数据找出,且对这些找出的简称数据进行去重,去重后就可以得知到底是哪些简称对应的全称的值为空
# 1.将state中的空值找出 pop_add['state'].isnull() # 2.将步骤1获取的布尔值作为源数据的行索引,获得state为空值对应的行数据 pop_add.loc[pop_add['state'].isnull()] # 3.将步骤二获取的df中的简称列取出即可 pop_add.loc[pop_add['state'].isnull()]['state/region'] #4.去重 pop_add.loc[pop_add['state'].isnull()]['state/region'].unique() array(['PR', 'USA'], dtype=object) # 找到了PR,USA简称对应的全称为空
6. 为找到的这些state/region的state项补上正确的值,从而去除掉state这一列的所有NaN
# 1.将PR对应的行数据取出 pop_add['state/region'] == 'PR' pop_add.loc[pop_add['state/region'] == 'PR'] # 2.可以将上一步获取的临时表的行索引获取 # 行索引就是PR对应的空值对应的行索引 indexs = pop_add.loc[pop_add['state/region'] == 'PR'].index # 3.填充 pop_add.loc[indexs,'state'] = 'PPPRRR'
#1.将USA对应的行数据取出 pop_add['state/region'] == 'USA' pop_add.loc[apop_add['state/region'] == 'USA'] #2.获取需要填充空值的索引 indexs = pop_add.loc[pop_add['state/region'] == 'USA'].index #3.填充 pop_add.loc[indexs, 'state'] = 'United States America'
7. 合并各州面积数据areas
pop_add_area = pd.merge(pop_add, area, on='state', how='outer') pop_add_area.head()
8. 我们会发现area(sq.mi)这一列有缺失数据,找出是哪些行
indexs = pop_add_area.loc[pop_add_area['area (sq. mi)'].isnull()].index Int64Index([2448, 2449, 2450, 2451, 2452, 2453, 2454, 2455, 2456, 2457, 2458, 2459, 2460, 2461, 2462, 2463, 2464, 2465, 2466, 2467, 2468, 2469, 2470, 2471, 2472, 2473, 2474, 2475, 2476, 2477, 2478, 2479, 2480, 2481, 2482, 2483, 2484, 2485, 2486, 2487, 2488, 2489, 2490, 2491, 2492, 2493, 2494, 2495, 2496, 2497, 2498, 2499, 2500, 2501, 2502, 2503, 2504, 2505, 2506, 2507, 2508, 2509, 2510, 2511, 2512, 2513, 2514, 2515, 2516, 2517, 2518, 2519, 2520, 2521, 2522, 2523, 2524, 2525, 2526, 2527, 2528, 2529, 2530, 2531, 2532, 2533, 2534, 2535, 2536, 2537, 2538, 2539, 2540, 2541, 2542, 2543], dtype='int64')
9. 去除含有缺失数据的行
indexs = pop_add_area.loc[pop_add_area['area (sq. mi)'].isnull()].index pop_add_area.drop(labels=indexs,axis=0, inplace=True)
10. 找出2010年的全民人口数据 query 条件查询
pop_add_area.query('year==2010 and ages=="total"') pop_add_area.query('year == 2010 & ages == "total"')

11. 计算各州的人口密度
# 人口密度:人口/面积 pop_add_area['midu'] = pop_add_area['population'] / pop_add_area['area (sq. mi)'] # 这样做是不对的,缺少考虑,这里主要的目的是告诉你如何添加1列,下面是正确方法,但需要通过映射将数据添加到原数据

pop_add_area.query('ages == "total"')['population'] / pop_add_area.query('ages == "total"')['area (sq. mi)']
12. 排序,并找出人口密度最高的州 sort_values
by根据哪列,ascending默认是True表示升序,False为降序
# by根据哪列,ascending默认是True表示升序,False为降序 pop_add_area.sort_values(by='midu', axis=0, ascending=False)
